Mastering Coding Challenges: Your Path to Programming Excellence
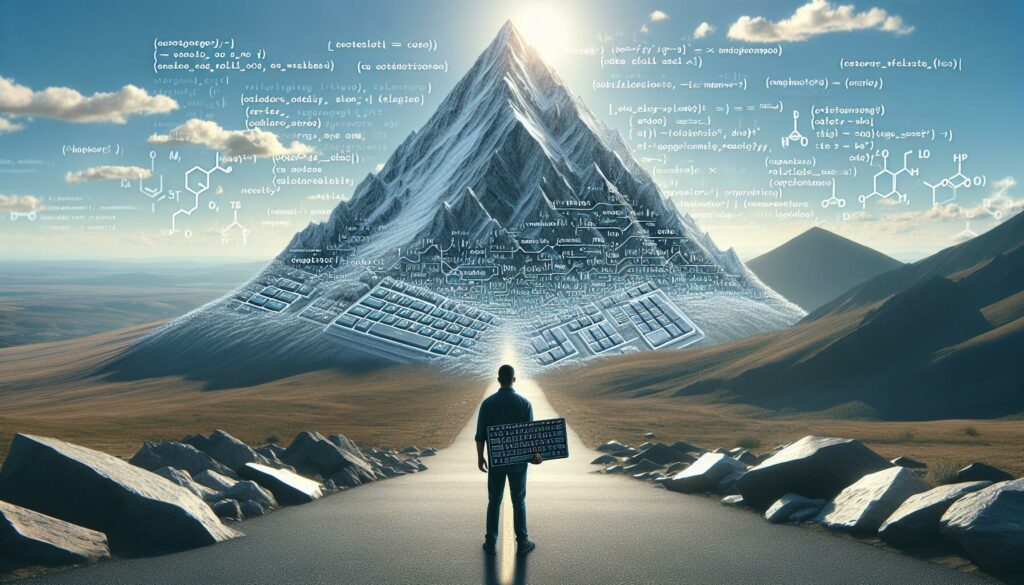
In today’s competitive tech landscape, mastering coding challenges has become an essential skill for aspiring programmers and seasoned developers alike. Whether you’re a beginner looking to sharpen your skills or a professional aiming to crack those coveted FAANG (Facebook, Amazon, Apple, Netflix, Google) interviews, understanding and conquering coding challenges is crucial. This comprehensive guide will walk you through the world of coding challenges, their importance, and how platforms like AlgoCademy can help you excel in your programming journey.
Understanding Coding Challenges
Coding challenges are problem-solving exercises designed to test a programmer’s ability to write efficient, clean, and functional code. These challenges often simulate real-world scenarios or focus on specific algorithmic concepts. They serve multiple purposes:
- Skill assessment for job interviews
- Personal skill development
- Competitive programming contests
- Enhancing problem-solving abilities
Platforms like AlgoCademy have revolutionized the way we approach these challenges by providing interactive environments, real-time feedback, and a structured learning path.
The Importance of Coding Challenges in Tech Careers
For those aspiring to work at top tech companies, particularly FAANG, coding challenges are more than just practice exercises—they’re a rite of passage. Here’s why they matter:
1. Interview Preparation
Many tech companies use coding challenges as a primary screening tool for potential hires. Mastering these challenges can significantly improve your chances of landing your dream job.
2. Skill Demonstration
Coding challenges provide a practical way to demonstrate your problem-solving skills, coding proficiency, and ability to work under pressure.
3. Continuous Learning
Regular practice with coding challenges keeps your skills sharp and exposes you to a wide range of programming concepts and best practices.
4. Building Confidence
As you solve more challenges, you build confidence in your abilities, which is crucial for technical interviews and real-world problem-solving.
Types of Coding Challenges
Coding challenges come in various forms, each testing different aspects of your programming skills:
1. Algorithm Challenges
These focus on implementing efficient algorithms to solve specific problems, often involving data structures, sorting, searching, and optimization.
2. Data Structure Challenges
These test your ability to use and manipulate data structures like arrays, linked lists, trees, and graphs effectively.
3. System Design Challenges
More common in senior-level interviews, these challenges assess your ability to design scalable software systems.
4. Debugging Challenges
These involve finding and fixing errors in existing code, testing your ability to read, understand, and optimize other people’s code.
5. Time-Limited Challenges
Often used in competitive programming, these challenges test your ability to solve problems quickly and efficiently under time constraints.
Strategies for Tackling Coding Challenges
To excel in coding challenges, consider the following strategies:
1. Understand the Problem
Before diving into coding, ensure you fully understand the problem statement. Identify the inputs, expected outputs, and any constraints.
2. Plan Your Approach
Sketch out your solution on paper or whiteboard before coding. This helps organize your thoughts and can reveal potential issues early.
3. Start with a Brute Force Solution
If you’re stuck, begin with the simplest solution that comes to mind, even if it’s not the most efficient. You can optimize later.
4. Optimize Incrementally
Once you have a working solution, look for ways to improve its time and space complexity.
5. Test Your Code
Always test your code with various inputs, including edge cases, to ensure it works correctly in all scenarios.
6. Practice Regularly
Consistency is key. Set aside time each day or week to practice coding challenges.
Leveraging AlgoCademy for Coding Challenge Success
AlgoCademy offers a comprehensive platform for mastering coding challenges, with features designed to enhance your learning experience:
1. Interactive Tutorials
Step-by-step guides that break down complex concepts into manageable chunks, allowing you to learn at your own pace.
2. AI-Powered Assistance
Get personalized hints and feedback as you work through challenges, helping you overcome obstacles and learn from your mistakes.
3. Diverse Challenge Library
Access a wide range of coding challenges, from beginner to advanced levels, covering various topics and difficulty levels.
4. Real-Time Code Execution
Test your solutions instantly in the browser, with support for multiple programming languages.
5. Progress Tracking
Monitor your improvement over time with detailed statistics and performance metrics.
6. Community Support
Engage with other learners, share solutions, and participate in discussions to enhance your understanding.
Common Pitfalls to Avoid in Coding Challenges
As you practice coding challenges, be aware of these common mistakes:
1. Overlooking Edge Cases
Always consider extreme or unusual inputs that might break your code.
2. Premature Optimization
Focus on getting a working solution first before trying to optimize it.
3. Neglecting Code Readability
Write clean, well-commented code. In real interviews, readability is as important as functionality.
4. Failing to Communicate
In interview settings, explain your thought process as you work. This gives interviewers insight into your problem-solving approach.
5. Ignoring Time and Space Complexity
Always consider the efficiency of your solution, especially for larger inputs.
Advanced Techniques for Coding Challenges
As you progress, consider incorporating these advanced techniques into your problem-solving toolkit:
1. Dynamic Programming
Learn to recognize problems that can be solved more efficiently using dynamic programming techniques.
2. Divide and Conquer
Master the art of breaking complex problems into smaller, more manageable sub-problems.
3. Greedy Algorithms
Understand when and how to apply greedy strategies to optimization problems.
4. Backtracking
Practice using backtracking to solve combinatorial problems efficiently.
5. Advanced Data Structures
Familiarize yourself with complex data structures like segment trees, Fenwick trees, and disjoint set unions.
Preparing for FAANG-Level Coding Challenges
If your goal is to crack interviews at top tech companies, consider these additional tips:
1. Focus on Core CS Fundamentals
Ensure you have a solid grasp of data structures, algorithms, and system design principles.
2. Practice Company-Specific Questions
Research and practice questions commonly asked by your target companies.
3. Mock Interviews
Conduct mock interviews with peers or use platforms that offer simulated interview experiences.
4. Time Management
Practice solving problems within time constraints to prepare for timed coding assessments.
5. Behavioral Preparation
Don’t neglect the non-technical aspects of interviews. Prepare for behavioral questions and company-specific cultural fit assessments.
Implementing a Coding Challenge: The Two Sum Problem
Let’s walk through a popular coding challenge to illustrate the problem-solving process:
Problem Statement: Two Sum
Given an array of integers nums
and an integer target
, return indices of the two numbers such that they add up to target
. You may assume that each input would have exactly one solution, and you may not use the same element twice.
Example:
Input: nums = [2,7,11,15], target = 9
Output: [0,1]
Explanation: Because nums[0] + nums[1] == 9, we return [0, 1].
Solution Approach:
We can solve this efficiently using a hash map to store the complement of each number as we iterate through the array.
Python Implementation:
def two_sum(nums, target):
num_map = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_map:
return [num_map[complement], i]
num_map[num] = i
return [] # No solution found
# Test the function
nums = [2, 7, 11, 15]
target = 9
result = two_sum(nums, target)
print(f"Input: nums = {nums}, target = {target}")
print(f"Output: {result}")
Explanation:
- We use a dictionary (hash map) to store each number and its index as we iterate through the array.
- For each number, we calculate its complement (target – num).
- If the complement exists in our map, we’ve found our pair and return their indices.
- If not, we add the current number and its index to the map and continue.
- If we complete the iteration without finding a solution, we return an empty list.
This solution has a time complexity of O(n) and a space complexity of O(n), where n is the length of the input array.
The Role of Coding Challenges in Building Real-World Skills
While coding challenges are often associated with interview preparation, their benefits extend far beyond landing a job. They play a crucial role in developing skills that are directly applicable to real-world software development:
1. Problem Decomposition
Coding challenges teach you to break down complex problems into smaller, manageable parts—a critical skill in tackling large-scale software projects.
2. Algorithmic Thinking
Regular practice with challenges enhances your ability to think algorithmically, helping you design more efficient solutions in your day-to-day work.
3. Code Optimization
As you solve challenges and compare your solutions with others, you learn various optimization techniques that can be applied to improve the performance of real-world applications.
4. Debugging Skills
Working through coding challenges sharpens your debugging skills, making you more efficient at identifying and fixing issues in production code.
5. Time Management
Timed challenges help you learn to work efficiently under pressure, a valuable skill in meeting project deadlines.
Integrating Coding Challenges into Your Learning Routine
To maximize the benefits of coding challenges, consider integrating them into your daily or weekly routine:
1. Set Realistic Goals
Start with a manageable number of challenges per week and gradually increase as you become more comfortable.
2. Diversify Your Practice
Mix easy, medium, and hard challenges to ensure you’re constantly pushing your boundaries while reinforcing fundamentals.
3. Review and Reflect
After solving a challenge, take time to review your solution and compare it with other approaches. Reflect on what you’ve learned and how you can improve.
4. Join Coding Communities
Participate in online coding communities or local meetups to share experiences, learn from others, and stay motivated.
5. Apply Learnings to Personal Projects
Try to incorporate concepts and techniques learned from coding challenges into your personal or professional projects.
The Future of Coding Challenges and Education
As technology continues to evolve, so does the landscape of coding challenges and programming education. Here are some trends shaping the future:
1. AI-Enhanced Learning
Platforms like AlgoCademy are increasingly incorporating AI to provide more personalized learning experiences, adapting to individual learning styles and paces.
2. Virtual Reality (VR) Coding Environments
VR technology may soon offer immersive coding challenge experiences, allowing learners to visualize complex algorithms and data structures in three-dimensional space.
3. Real-Time Collaboration Features
Future platforms may emphasize collaborative problem-solving, mimicking real-world development environments where teamwork is crucial.
4. Integration with Job Markets
Coding challenge platforms may become more closely integrated with job markets, potentially serving as direct pipelines for tech recruitment.
5. Focus on Emerging Technologies
As fields like artificial intelligence, blockchain, and quantum computing grow, coding challenges will likely evolve to include problems specific to these domains.
Conclusion
Mastering coding challenges is a journey that requires dedication, consistent practice, and a willingness to learn from both successes and failures. Platforms like AlgoCademy provide invaluable resources and tools to support this journey, offering structured learning paths, interactive tutorials, and AI-powered assistance.
Remember, the goal of tackling coding challenges goes beyond just preparing for interviews. It’s about developing a problem-solving mindset, enhancing your algorithmic thinking, and building the confidence to tackle any programming task that comes your way. Whether you’re aiming for a position at a FAANG company or looking to excel in your current role, the skills you develop through coding challenges will serve you well throughout your career in tech.
As you continue your coding education, stay curious, embrace new challenges, and never stop learning. The world of programming is vast and ever-evolving, and with the right approach and resources, you can position yourself at the forefront of this exciting field. Happy coding!