Ultimate Guide to Coding Interview Preparation: Ace Your Next Tech Interview
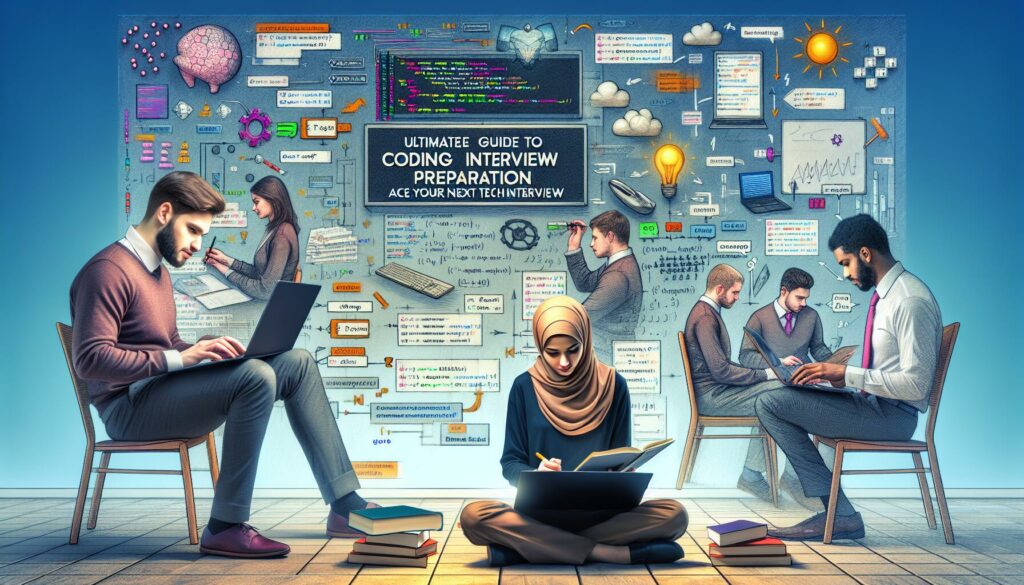
Are you gearing up for a coding interview with a top tech company? Whether you’re aiming for a position at a FAANG (Facebook, Amazon, Apple, Netflix, Google) company or any other tech giant, proper preparation is key to success. In this comprehensive guide, we’ll walk you through the essential steps and strategies to help you ace your next coding interview.
Table of Contents
- Understanding Coding Interviews
- Essential Topics to Master
- Problem-Solving Strategies
- Practice Resources and Platforms
- The Importance of Mock Interviews
- Soft Skills and Communication
- Interview Day Tips
- Continuous Improvement and Learning
1. Understanding Coding Interviews
Before diving into preparation strategies, it’s crucial to understand what coding interviews entail. Tech companies use coding interviews to assess a candidate’s problem-solving skills, coding ability, and technical knowledge. These interviews typically involve:
- Algorithmic problem-solving
- Data structure manipulation
- Code optimization
- System design (for more senior positions)
- Behavioral questions
The format can vary, including whiteboard coding, online coding platforms, or take-home assignments. Understanding the specific format for your target company can help you tailor your preparation accordingly.
2. Essential Topics to Master
To excel in coding interviews, you need a strong foundation in computer science fundamentals. Here are the key areas to focus on:
Data Structures
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
Algorithms
- Sorting (e.g., Quick Sort, Merge Sort)
- Searching (e.g., Binary Search)
- Recursion and Dynamic Programming
- Graph Algorithms (e.g., BFS, DFS)
- Greedy Algorithms
Big O Notation and Time/Space Complexity
Understanding how to analyze and optimize the efficiency of your code is crucial. Learn to express time and space complexity using Big O notation and be prepared to discuss trade-offs between different approaches.
Object-Oriented Programming (OOP) Concepts
Familiarize yourself with OOP principles such as encapsulation, inheritance, polymorphism, and abstraction. Be ready to apply these concepts in your solutions when appropriate.
System Design Basics
For more senior positions, you may be asked about system design. Study concepts like scalability, load balancing, caching, and database design.
3. Problem-Solving Strategies
Developing a structured approach to problem-solving is essential for coding interviews. Here’s a recommended strategy:
- Understand the problem: Carefully read the question and ask clarifying questions if needed.
- Identify constraints: Consider input sizes, time/space complexity requirements, and any special conditions.
- Brainstorm solutions: Think of multiple approaches before settling on one.
- Plan your approach: Outline your solution before coding.
- Implement the solution: Write clean, readable code.
- Test and debug: Use example inputs and edge cases to verify your solution.
- Optimize: Consider ways to improve the time or space complexity of your solution.
Practice this approach with various problems to make it second nature during interviews.
4. Practice Resources and Platforms
Consistent practice is key to improving your coding skills. Here are some excellent resources to help you prepare:
Online Platforms
- LeetCode: Offers a vast collection of coding problems, including many that are frequently asked in tech interviews.
- HackerRank: Provides coding challenges and competitions to hone your skills.
- CodeSignal: Offers a mix of practice problems and real company coding assessments.
- AlgoExpert: Curated list of coding interview questions with video explanations.
Books
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “Elements of Programming Interviews” by Adnan Aziz, Tsung-Hsien Lee, and Amit Prakash
- “Introduction to Algorithms” by Thomas H. Cormen, Charles E. Leiserson, Ronald L. Rivest, and Clifford Stein
Interactive Coding Tutorials
Platforms like AlgoCademy offer interactive coding tutorials and AI-powered assistance to help you progress from beginner-level coding to advanced algorithmic problem-solving. These resources can provide structured learning paths and immediate feedback on your code.
5. The Importance of Mock Interviews
Mock interviews are invaluable for preparing for the real thing. They help you:
- Practice explaining your thought process out loud
- Get comfortable with the interview format
- Receive feedback on your performance
- Identify areas for improvement
Consider these options for mock interviews:
- Peer practice: Partner with a friend or colleague for mutual practice.
- Online platforms: Websites like Pramp or interviewing.io offer free peer-to-peer mock interviews.
- Professional services: Some companies offer paid mock interview services with experienced interviewers.
6. Soft Skills and Communication
While technical skills are crucial, soft skills can make or break your interview performance. Focus on improving these areas:
Clear Communication
Practice articulating your thoughts clearly. Explain your approach, reasoning, and any assumptions you’re making as you solve problems.
Active Listening
Pay close attention to the interviewer’s questions and hints. Sometimes, they may provide valuable clues or steer you in the right direction.
Handling Pressure
Learn techniques to stay calm under pressure, such as deep breathing or positive self-talk.
Collaboration
Show that you can work well with others by being receptive to feedback and suggestions during the interview.
7. Interview Day Tips
When the big day arrives, keep these tips in mind:
- Arrive early: Whether it’s an in-person or virtual interview, be ready well before the scheduled time.
- Dress appropriately: Even for virtual interviews, dress professionally to set the right mindset.
- Bring necessary items: Have a notepad, pen, and water handy.
- Test your setup: For virtual interviews, check your internet connection, camera, and microphone in advance.
- Stay positive: Maintain a positive attitude throughout the interview, even if you encounter difficult questions.
During the Coding Challenge
- Read the problem carefully and ask clarifying questions.
- Think out loud as you work through the problem.
- Start with a brute force solution if you can’t immediately see an optimal approach.
- Write clean, well-commented code.
- Test your solution with various inputs, including edge cases.
- If you get stuck, don’t panic. Ask for hints or break the problem down into smaller steps.
8. Continuous Improvement and Learning
Remember that interview preparation is an ongoing process. Even after your interview, continue to:
- Reflect on your performance and identify areas for improvement.
- Stay updated with the latest programming languages, frameworks, and industry trends.
- Contribute to open-source projects to gain real-world coding experience.
- Attend coding workshops, hackathons, or tech meetups to network and learn from others.
Learning from Rejections
If you face rejection, don’t be discouraged. Instead:
- Ask for feedback from the interviewer or recruiter.
- Analyze where you can improve based on the feedback.
- Develop a plan to address your weaknesses.
- Keep practicing and applying to other opportunities.
Conclusion
Preparing for coding interviews requires dedication, consistent practice, and a strategic approach. By mastering the essential topics, honing your problem-solving skills, and practicing regularly, you’ll significantly increase your chances of success. Remember that each interview, regardless of the outcome, is a learning opportunity that brings you one step closer to your dream job in tech.
As you embark on your interview preparation journey, consider leveraging platforms like AlgoCademy that offer structured learning paths, interactive coding tutorials, and AI-powered assistance. These resources can provide valuable guidance and help you track your progress as you work towards your goals.
Stay motivated, keep learning, and approach each challenge as an opportunity to grow. With the right preparation and mindset, you’ll be well-equipped to tackle even the most demanding coding interviews at top tech companies. Good luck on your journey to becoming a successful software engineer!
Sample Coding Interview Question and Solution
To give you a taste of what to expect in a coding interview, let’s look at a common problem and walk through a solution:
Problem: Two Sum
Given an array of integers nums
and an integer target
, return indices of the two numbers in the array such that they add up to the target
. You may assume that each input would have exactly one solution, and you may not use the same element twice.
Example:
Input: nums = [2,7,11,15], target = 9
Output: [0,1]
Explanation: Because nums[0] + nums[1] == 9, we return [0, 1].
Solution:
Here’s an efficient solution using a hash map:
class Solution {
public int[] twoSum(int[] nums, int target) {
Map<Integer, Integer> map = new HashMap<>();
for (int i = 0; i < nums.length; i++) {
int complement = target - nums[i];
if (map.containsKey(complement)) {
return new int[] { map.get(complement), i };
}
map.put(nums[i], i);
}
throw new IllegalArgumentException("No two sum solution");
}
}
Explanation:
- We use a HashMap to store each number and its index as we iterate through the array.
- For each number, we calculate its complement (target – current number).
- If the complement exists in our map, we’ve found our pair and return their indices.
- If not, we add the current number and its index to the map and continue.
- If we complete the loop without finding a solution, we throw an exception (though this shouldn’t happen given the problem constraints).
This solution has a time complexity of O(n) and a space complexity of O(n), where n is the length of the input array. It’s more efficient than the brute force approach of checking every pair, which would be O(n^2).
When presenting this solution in an interview, you would:
- Explain your thought process and why you chose this approach.
- Discuss the time and space complexity.
- Walk through the code, explaining each step.
- Discuss any potential edge cases or improvements.
Remember, the key is not just to solve the problem, but to communicate your reasoning clearly throughout the process. This demonstrates both your technical skills and your ability to explain complex concepts, which are both highly valued in the tech industry.