Empower Your Coding Arsenal: Dynamic Programming Examples at Your Fingertips
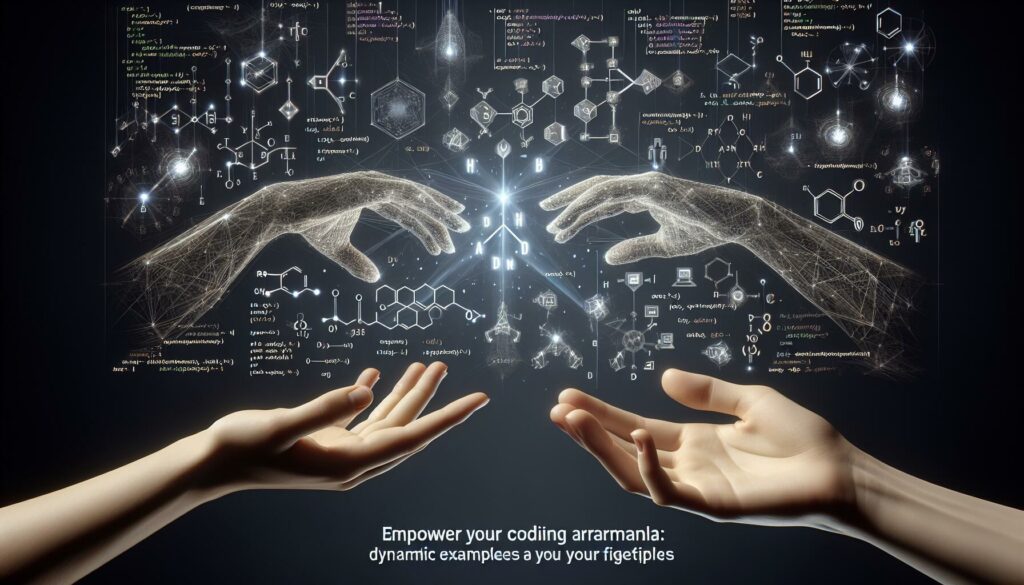
In the ever-evolving world of software development, mastering advanced algorithmic techniques is crucial for solving complex problems efficiently. Among these techniques, dynamic programming (DP) stands out as a powerful tool that can significantly optimize your code’s performance. Whether you’re a coding enthusiast looking to level up your skills or a seasoned developer preparing for technical interviews at top tech companies, understanding and applying dynamic programming can be a game-changer.
In this comprehensive guide, we’ll dive deep into the world of dynamic programming, exploring its core concepts and demonstrating its practical applications through a variety of examples. By the end of this article, you’ll have a solid grasp of DP and be equipped with the knowledge to tackle challenging coding problems with confidence.
What is Dynamic Programming?
Dynamic programming is an algorithmic paradigm that solves complex problems by breaking them down into simpler subproblems. It is particularly useful for optimization problems where you need to find the best solution among many possible options. The key idea behind DP is to store the results of subproblems to avoid redundant computations, thus improving the overall efficiency of the algorithm.
There are two main approaches to implementing dynamic programming:
- Top-down approach (Memoization): This method starts with the main problem and recursively breaks it down into smaller subproblems. It uses a cache (usually a hash table) to store the results of subproblems, preventing redundant calculations.
- Bottom-up approach (Tabulation): This method starts by solving the smallest subproblems first and gradually builds up to the main problem. It typically uses an array or table to store intermediate results.
Now, let’s explore some classic dynamic programming problems and their solutions to better understand how to apply these concepts in practice.
1. Fibonacci Sequence
The Fibonacci sequence is a classic example used to introduce dynamic programming. Each number in the sequence is the sum of the two preceding ones, starting from 0 and 1.
Recursive Solution (without DP)
Here’s a simple recursive implementation without dynamic programming:
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
print(fibonacci(10)) # Output: 55
While this solution works, it has a time complexity of O(2^n), making it inefficient for large values of n due to redundant calculations.
Top-down DP Solution (Memoization)
Let’s improve the recursive solution using memoization:
def fibonacci_memo(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci_memo(n-1, memo) + fibonacci_memo(n-2, memo)
return memo[n]
print(fibonacci_memo(100)) # Output: 354224848179261915075
This solution has a time complexity of O(n) and can handle much larger inputs efficiently.
Bottom-up DP Solution (Tabulation)
Here’s the bottom-up approach using tabulation:
def fibonacci_tab(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
print(fibonacci_tab(100)) # Output: 354224848179261915075
This solution also has a time complexity of O(n) and uses O(n) space to store the DP table.
2. Longest Common Subsequence (LCS)
The Longest Common Subsequence problem aims to find the longest subsequence common to two sequences. This problem has applications in diff utilities, DNA sequence alignment, and more.
Bottom-up DP Solution
def lcs(X, Y):
m, n = len(X), len(Y)
L = [[0] * (n + 1) for _ in range(m + 1)]
for i in range(1, m + 1):
for j in range(1, n + 1):
if X[i-1] == Y[j-1]:
L[i][j] = L[i-1][j-1] + 1
else:
L[i][j] = max(L[i-1][j], L[i][j-1])
return L[m][n]
X = "ABCDGH"
Y = "AEDFHR"
print(f"Length of LCS is {lcs(X, Y)}") # Output: Length of LCS is 3
This solution has a time complexity of O(mn) and space complexity of O(mn), where m and n are the lengths of the input sequences.
3. Knapsack Problem
The 0/1 Knapsack problem is a classic optimization problem where you need to select items with given weights and values to maximize the total value while staying within a weight limit.
Top-down DP Solution (Memoization)
def knapsack(W, wt, val, n, memo={}):
if n == 0 or W == 0:
return 0
if (W, n) in memo:
return memo[(W, n)]
if wt[n-1] > W:
memo[(W, n)] = knapsack(W, wt, val, n-1, memo)
else:
memo[(W, n)] = max(val[n-1] + knapsack(W-wt[n-1], wt, val, n-1, memo),
knapsack(W, wt, val, n-1, memo))
return memo[(W, n)]
val = [60, 100, 120]
wt = [10, 20, 30]
W = 50
n = len(val)
print(knapsack(W, wt, val, n)) # Output: 220
This solution has a time complexity of O(nW) and uses memoization to avoid redundant calculations.
4. Coin Change Problem
The Coin Change problem asks for the minimum number of coins needed to make a given amount of money, given a set of coin denominations.
Bottom-up DP Solution
def coin_change(coins, amount):
dp = [float('inf')] * (amount + 1)
dp[0] = 0
for i in range(1, amount + 1):
for coin in coins:
if coin <= i:
dp[i] = min(dp[i], dp[i - coin] + 1)
return dp[amount] if dp[amount] != float('inf') else -1
coins = [1, 2, 5]
amount = 11
print(f"Minimum coins needed: {coin_change(coins, amount)}") # Output: Minimum coins needed: 3
This solution has a time complexity of O(amount * len(coins)) and a space complexity of O(amount).
5. Longest Increasing Subsequence (LIS)
The Longest Increasing Subsequence problem aims to find the length of the longest subsequence of a given sequence such that all elements of the subsequence are sorted in ascending order.
Bottom-up DP Solution
def lis(arr):
n = len(arr)
dp = [1] * n
for i in range(1, n):
for j in range(i):
if arr[i] > arr[j]:
dp[i] = max(dp[i], dp[j] + 1)
return max(dp)
arr = [10, 22, 9, 33, 21, 50, 41, 60, 80]
print(f"Length of LIS is {lis(arr)}") # Output: Length of LIS is 6
This solution has a time complexity of O(n^2) and a space complexity of O(n).
6. Matrix Chain Multiplication
The Matrix Chain Multiplication problem determines the most efficient way to multiply a given sequence of matrices.
Bottom-up DP Solution
def matrix_chain_order(p):
n = len(p) - 1
m = [[0 for _ in range(n)] for _ in range(n)]
for L in range(2, n + 1):
for i in range(n - L + 1):
j = i + L - 1
m[i][j] = float('inf')
for k in range(i, j):
q = m[i][k] + m[k+1][j] + p[i]*p[k+1]*p[j+1]
if q < m[i][j]:
m[i][j] = q
return m[0][n-1]
arr = [40, 20, 30, 10, 30]
print(f"Minimum number of multiplications is {matrix_chain_order(arr)}")
# Output: Minimum number of multiplications is 26000
This solution has a time complexity of O(n^3) and a space complexity of O(n^2).
7. Edit Distance
The Edit Distance problem calculates the minimum number of operations (insert, delete, or replace) required to transform one string into another.
Bottom-up DP Solution
def edit_distance(str1, str2):
m, n = len(str1), len(str2)
dp = [[0 for _ in range(n + 1)] for _ in range(m + 1)]
for i in range(m + 1):
for j in range(n + 1):
if i == 0:
dp[i][j] = j
elif j == 0:
dp[i][j] = i
elif str1[i-1] == str2[j-1]:
dp[i][j] = dp[i-1][j-1]
else:
dp[i][j] = 1 + min(dp[i-1][j], # Delete
dp[i][j-1], # Insert
dp[i-1][j-1]) # Replace
return dp[m][n]
str1 = "kitten"
str2 = "sitting"
print(f"Edit Distance: {edit_distance(str1, str2)}") # Output: Edit Distance: 3
This solution has a time complexity of O(mn) and a space complexity of O(mn), where m and n are the lengths of the input strings.
8. Palindrome Partitioning
The Palindrome Partitioning problem finds the minimum number of cuts needed to partition a string such that every substring is a palindrome.
Bottom-up DP Solution
def palindrome_partitioning(s):
n = len(s)
dp = [[0 for _ in range(n)] for _ in range(n)]
for L in range(2, n + 1):
for i in range(n - L + 1):
j = i + L - 1
if L == 2:
dp[i][j] = 0 if s[i] == s[j] else 1
elif s[i] == s[j]:
dp[i][j] = dp[i+1][j-1]
else:
dp[i][j] = min(dp[i][k] + dp[k+1][j] + 1 for k in range(i, j))
return dp[0][n-1]
s = "ababbbabbababa"
print(f"Minimum cuts needed: {palindrome_partitioning(s)}") # Output: Minimum cuts needed: 3
This solution has a time complexity of O(n^3) and a space complexity of O(n^2).
Conclusion
Dynamic programming is a powerful technique that can significantly improve the efficiency of your algorithms when dealing with optimization problems. By breaking down complex problems into smaller subproblems and storing their results, DP allows you to avoid redundant calculations and find optimal solutions more quickly.
In this article, we’ve explored several classic dynamic programming problems and their solutions, including:
- Fibonacci Sequence
- Longest Common Subsequence
- Knapsack Problem
- Coin Change Problem
- Longest Increasing Subsequence
- Matrix Chain Multiplication
- Edit Distance
- Palindrome Partitioning
By studying these examples and practicing similar problems, you can develop a strong intuition for identifying and solving DP problems. This skill will prove invaluable as you progress in your coding journey, tackle more complex challenges, and prepare for technical interviews at top tech companies.
Remember, mastering dynamic programming takes time and practice. Don’t get discouraged if you find some problems challenging at first. Keep working on different problems, analyze their patterns, and try to develop a systematic approach to solving DP problems. With persistence and dedication, you’ll soon find yourself confidently tackling even the most complex optimization challenges.
As you continue to expand your coding arsenal, consider exploring other advanced algorithmic techniques such as greedy algorithms, divide and conquer, and graph algorithms. Each of these approaches has its own strengths and applications, and a well-rounded understanding of various problem-solving techniques will make you a more versatile and effective programmer.
Happy coding, and may your dynamic programming skills lead you to optimal solutions in both your coding projects and your career!