Embark on Your Coding Journey: Essential Guide For Beginners
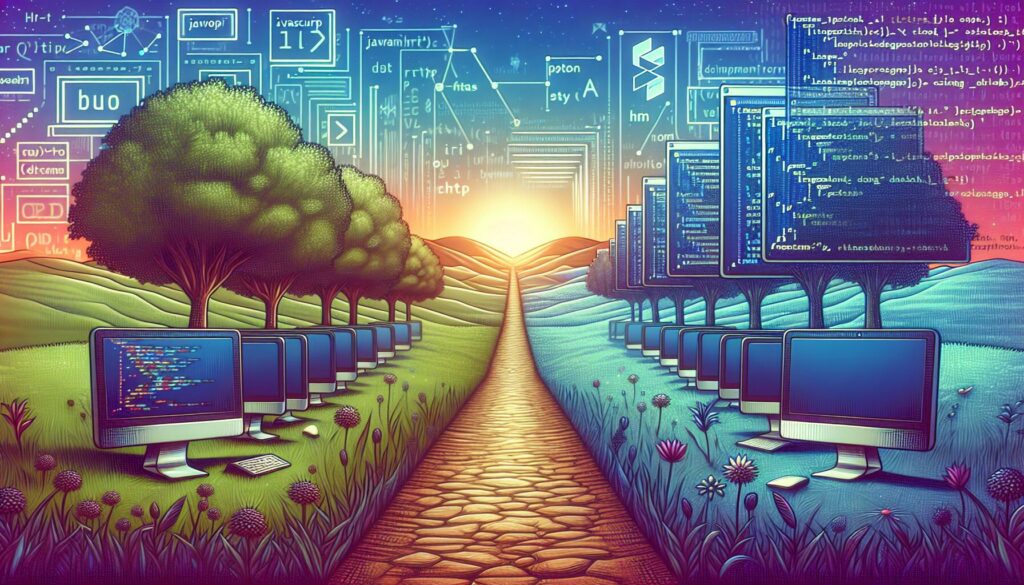
Are you ready to dive into the exciting world of coding? Whether you’re looking to switch careers, enhance your current skill set, or simply explore a new hobby, learning to code can open up a world of opportunities. This comprehensive guide will walk you through the essential steps to start your coding journey, from understanding the basics to preparing for technical interviews at top tech companies.
Why Learn to Code?
Before we delve into the ‘how’, let’s briefly touch on the ‘why’. In today’s digital age, coding skills are more valuable than ever. Here are a few reasons why learning to code is a great investment:
- Career Opportunities: The tech industry is booming, with high demand for skilled programmers across various sectors.
- Problem-Solving Skills: Coding teaches you to think logically and approach problems systematically.
- Creativity: Programming allows you to bring your ideas to life, whether it’s building websites, apps, or software.
- Understanding Technology: Coding gives you a deeper understanding of the technology that surrounds us daily.
- Flexibility: Many coding jobs offer remote work options and flexible schedules.
Getting Started: The Basics of Coding
Starting your coding journey can seem daunting, but remember, every expert was once a beginner. Here are the fundamental concepts you need to grasp:
1. Understanding Programming Languages
Programming languages are the tools we use to communicate with computers. Some popular languages for beginners include:
- Python: Known for its simplicity and readability, Python is an excellent language for beginners.
- JavaScript: Essential for web development, JavaScript is versatile and widely used.
- Java: A popular choice for building large-scale applications, particularly in enterprise environments.
- C++: While more complex, C++ is powerful and often used in game development and system programming.
2. Basic Programming Concepts
Regardless of the language you choose, there are some universal concepts you’ll need to understand:
- Variables: Containers for storing data values.
- Data Types: Categories for different kinds of data (e.g., integers, strings, booleans).
- Operators: Symbols that tell the compiler to perform specific mathematical or logical manipulations.
- Control Structures: Mechanisms that control the flow of execution in a program (e.g., if-else statements, loops).
- Functions: Reusable blocks of code that perform specific tasks.
3. Writing Your First Program
Let’s start with the classic “Hello, World!” program in Python:
print("Hello, World!")
This simple line of code instructs the computer to display the text “Hello, World!” on the screen. It’s a small step, but it’s the beginning of your coding journey!
Building a Strong Foundation
As you progress, you’ll want to focus on building a strong foundation in programming concepts and practices:
1. Data Structures and Algorithms
Understanding data structures (like arrays, linked lists, and trees) and algorithms (like sorting and searching) is crucial for efficient problem-solving in programming. These concepts form the backbone of computer science and are often the focus of technical interviews at major tech companies.
2. Object-Oriented Programming (OOP)
OOP is a programming paradigm based on the concept of “objects,” which can contain data and code. It’s a fundamental concept in many programming languages and is essential for building complex, maintainable software systems.
3. Version Control
Learning to use version control systems like Git is crucial for collaborating with other developers and managing your code. It allows you to track changes, revert to previous versions, and work on different features simultaneously.
4. Debugging Skills
Debugging is the process of finding and fixing errors in your code. It’s an essential skill for any programmer. Learn to use debugging tools and techniques to efficiently identify and resolve issues in your programs.
Practical Learning: Building Projects
Theory is important, but nothing beats hands-on experience. As you learn, start building small projects to apply your knowledge. Here are some ideas:
- A simple calculator
- A to-do list application
- A basic website
- A simple game (like Tic-Tac-Toe)
These projects will help you understand how different components of a program work together and give you practical experience in problem-solving.
Leveraging Online Resources
The internet is a treasure trove of coding resources. Here are some platforms that can aid your learning:
- AlgoCademy: Offers interactive coding tutorials and AI-powered assistance, focusing on algorithmic thinking and problem-solving.
- Codecademy: Provides interactive coding lessons in various programming languages.
- freeCodeCamp: Offers free coding courses and certifications.
- LeetCode: Provides a platform for practicing coding problems, especially useful for interview preparation.
- GitHub: A platform for hosting and sharing code, as well as collaborating with other developers.
Developing Problem-Solving Skills
Coding is essentially problem-solving. To excel in programming, you need to develop strong analytical and logical thinking skills. Here are some ways to improve your problem-solving abilities:
1. Break Down Complex Problems
When faced with a complex problem, break it down into smaller, manageable parts. This approach, known as “divide and conquer,” is a fundamental strategy in programming.
2. Practice Regularly
Consistent practice is key to improving your coding skills. Set aside time each day to work on coding problems or projects.
3. Learn from Others
Study other people’s code. Platforms like GitHub allow you to explore open-source projects and learn from experienced developers.
4. Participate in Coding Challenges
Websites like HackerRank and LeetCode offer coding challenges that can help you improve your problem-solving skills and prepare for technical interviews.
Understanding Algorithmic Thinking
Algorithmic thinking is the ability to define clear steps to solve a problem or complete a task. It’s a crucial skill for programmers. Here’s a simple example of algorithmic thinking applied to a daily task:
Algorithm for Making a Sandwich:
1. Gather ingredients (bread, filling, condiments)
2. Take two slices of bread
3. Apply condiments to one side of each bread slice
4. Place filling on one slice of bread
5. Put the other slice on top of the filling, condiment side down
6. Cut the sandwich diagonally (optional)
7. Serve the sandwich
This same principle of breaking down tasks into clear, logical steps is applied in programming to solve complex problems.
The Importance of Clean Code
As you progress in your coding journey, you’ll learn that writing code that works is only part of the challenge. Writing clean, maintainable code is equally important. Here are some principles of clean code:
- Readability: Your code should be easy for others (and yourself) to read and understand.
- Simplicity: Strive for simple solutions. Complicated code is more prone to bugs and harder to maintain.
- DRY (Don’t Repeat Yourself): Avoid duplicating code. If you find yourself writing the same code in multiple places, it’s time to create a function.
- Meaningful Names: Use clear, descriptive names for variables, functions, and classes.
- Comments: Use comments to explain why you’re doing something, not what you’re doing (the code itself should be clear enough to explain what it’s doing).
Preparing for Technical Interviews
If your goal is to land a job at a major tech company (often referred to as FAANG – Facebook, Amazon, Apple, Netflix, Google), you’ll need to prepare for technical interviews. These interviews often focus on data structures, algorithms, and problem-solving skills. Here’s how you can prepare:
1. Study Core Computer Science Concepts
Make sure you have a solid understanding of data structures (arrays, linked lists, trees, graphs, etc.) and algorithms (sorting, searching, dynamic programming, etc.).
2. Practice Coding Problems
Websites like LeetCode, HackerRank, and AlgoCademy offer a wide range of coding problems similar to those you might encounter in interviews. Practice solving these problems and explaining your thought process.
3. Mock Interviews
Practice with friends or use platforms that offer mock interview services. This will help you get comfortable explaining your thought process while coding under pressure.
4. System Design
For more senior positions, you may be asked about system design. Study concepts like scalability, load balancing, caching, and database design.
5. Behavioral Questions
Don’t forget to prepare for behavioral questions. Be ready to discuss your past projects, challenges you’ve overcome, and how you work in a team.
Continuing Your Learning Journey
Remember, learning to code is a lifelong journey. Technology is constantly evolving, and there’s always something new to learn. Here are some tips for continuing your education:
- Stay Updated: Follow tech blogs, podcasts, and news sites to stay informed about the latest developments in the programming world.
- Attend Meetups and Conferences: These events are great for networking and learning about new technologies and best practices.
- Contribute to Open Source: Contributing to open-source projects is an excellent way to gain real-world experience and give back to the community.
- Never Stop Building: Continue to challenge yourself by building increasingly complex projects.
- Teach Others: Teaching is one of the best ways to solidify your own understanding of a concept.
Conclusion: Your Coding Adventure Begins
Embarking on your coding journey is an exciting step towards a future full of possibilities. Remember, every expert was once a beginner, and with dedication, persistence, and the right resources, you can achieve your coding goals.
Whether you’re aiming to build your own apps, contribute to open-source projects, or land a job at a top tech company, the skills you’ll learn along the way will be invaluable. Coding is not just about writing programs; it’s about problem-solving, creativity, and continuous learning.
So, take that first step. Write your first “Hello, World!” program. Solve your first algorithm problem. Build your first project. Before you know it, you’ll be well on your way to becoming the programmer you aspire to be.
Remember, platforms like AlgoCademy are here to support you every step of the way, from your first lines of code to preparing for technical interviews at major tech companies. Happy coding, and welcome to the wonderful world of programming!