5 Proven Techniques to Approach Any Coding Interview Problem
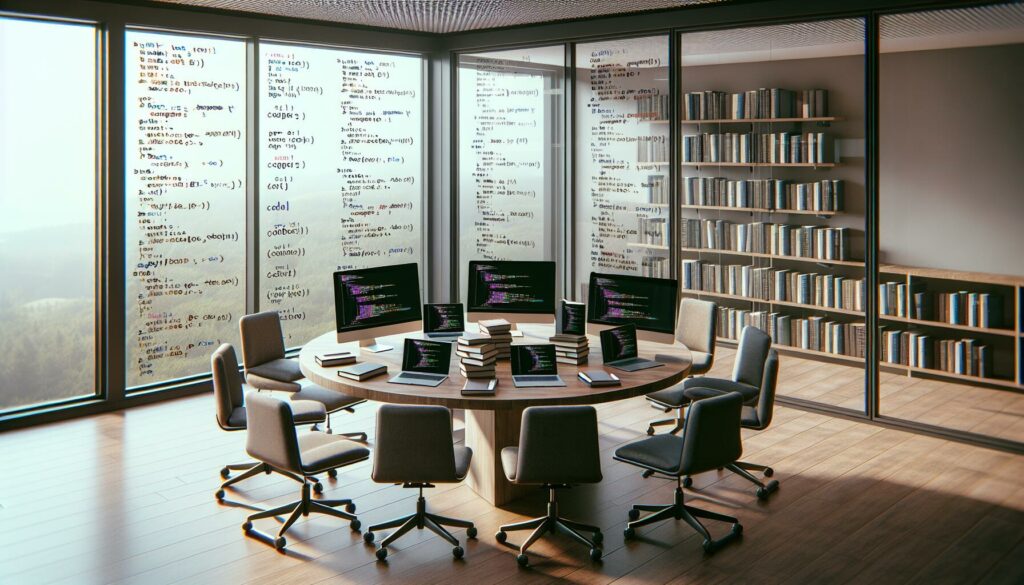
Coding interviews can be daunting, especially when you’re faced with complex algorithmic challenges under time pressure. However, with the right approach and a solid understanding of fundamental problem-solving techniques, you can tackle even the most intimidating coding problems with confidence. In this comprehensive guide, we’ll explore five proven techniques that will help you approach any coding interview problem effectively.
Whether you’re a beginner preparing for your first technical interview or an experienced developer looking to refine your skills, these techniques will serve as valuable tools in your problem-solving arsenal. Let’s dive in and discover how you can leverage these strategies to excel in your next coding interview.
1. Brute Force Approach: The Foundation of Problem-Solving
The brute force approach is often considered the most straightforward method to solve a coding problem. It involves systematically enumerating all possible candidates for the solution and checking whether each candidate satisfies the problem statement. While it may not always be the most efficient solution, it serves as an excellent starting point for understanding the problem and developing more optimized approaches.
When to Use Brute Force:
- When the problem size is small
- As a first step to understand the problem better
- When time complexity is not a primary concern
- To verify the correctness of more optimized solutions
Example: Finding the Maximum Subarray Sum
Let’s consider a classic problem: finding the contiguous subarray with the largest sum within an array of integers. Here’s how we can approach this using the brute force method:
def max_subarray_sum_brute_force(arr):
n = len(arr)
max_sum = float('-inf')
for i in range(n):
for j in range(i, n):
current_sum = sum(arr[i:j+1])
max_sum = max(max_sum, current_sum)
return max_sum
# Example usage
arr = [-2, 1, -3, 4, -1, 2, 1, -5, 4]
result = max_subarray_sum_brute_force(arr)
print(f"Maximum subarray sum: {result}")
This brute force solution has a time complexity of O(n³), which is not efficient for large arrays. However, it clearly demonstrates the problem-solving process and can be used as a stepping stone to develop more optimized solutions.
Pros and Cons of Brute Force:
Pros:
- Simple to implement and understand
- Guaranteed to find the correct solution (if one exists)
- Useful for small input sizes or as a baseline for comparison
Cons:
- Often has high time complexity
- May be impractical for large input sizes
- Not suitable for real-time or performance-critical applications
While brute force may not always be the most efficient solution, it’s an essential technique to master. It helps you understand the problem deeply and can lead to insights for developing more optimized approaches.
2. Greedy Algorithms: Making Locally Optimal Choices
Greedy algorithms are a powerful problem-solving technique that makes the locally optimal choice at each step with the hope of finding a global optimum. This approach is particularly useful when dealing with optimization problems where a series of decisions need to be made.
When to Use Greedy Algorithms:
- Optimization problems with local choice property
- When the locally optimal choice leads to a globally optimal solution
- Problems involving scheduling or resource allocation
- When you need to maximize or minimize a certain quantity
Example: Activity Selection Problem
Let’s consider the Activity Selection Problem: Given a set of activities with start and finish times, select the maximum number of non-overlapping activities that can be performed by a single person.
def activity_selection(activities):
# Sort activities based on finish time
activities.sort(key=lambda x: x[1])
selected = [activities[0]]
last_finish = activities[0][1]
for activity in activities[1:]:
if activity[0] >= last_finish:
selected.append(activity)
last_finish = activity[1]
return selected
# Example usage
activities = [(1, 4), (3, 5), (0, 6), (5, 7), (3, 8), (5, 9), (6, 10), (8, 11), (8, 12), (2, 13), (12, 14)]
result = activity_selection(activities)
print(f"Selected activities: {result}")
print(f"Number of activities: {len(result)}")
In this greedy approach, we sort the activities by their finish time and then select activities that don’t overlap with the previously selected activity. This algorithm has a time complexity of O(n log n) due to the sorting step.
Pros and Cons of Greedy Algorithms:
Pros:
- Often simple to implement and understand
- Generally efficient in terms of time complexity
- Can provide optimal solutions for certain problem types
Cons:
- May not always lead to the globally optimal solution
- Requires careful proof of correctness
- Not suitable for all types of problems
Greedy algorithms can be powerful tools when applied to the right problems. However, it’s crucial to verify that the greedy choice property holds for the specific problem you’re solving.
3. Dynamic Programming: Breaking Down Complex Problems
Dynamic Programming (DP) is a method for solving complex problems by breaking them down into simpler subproblems. It is particularly useful when the problem has overlapping subproblems and optimal substructure properties. DP solutions typically involve storing the results of subproblems to avoid redundant computations.
When to Use Dynamic Programming:
- Problems with overlapping subproblems
- Optimization problems with optimal substructure
- When recursive solutions have exponential time complexity
- Problems involving sequences or grids
Example: Fibonacci Sequence
Let’s implement the Fibonacci sequence using dynamic programming. This example demonstrates how DP can dramatically improve the efficiency of a recursive solution:
def fibonacci_dp(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
# Example usage
n = 100
result = fibonacci_dp(n)
print(f"The {n}th Fibonacci number is: {result}")
This DP solution has a time complexity of O(n), which is a significant improvement over the exponential time complexity of a naive recursive approach.
Pros and Cons of Dynamic Programming:
Pros:
- Can solve complex problems efficiently
- Avoids redundant computations
- Often leads to polynomial-time solutions for problems that would be exponential with naive approaches
Cons:
- Can be challenging to identify the optimal substructure
- May require additional space to store subproblem results
- Implementation can be more complex than other approaches
Dynamic Programming is a powerful technique that can significantly optimize solutions for certain types of problems. Mastering DP can give you a significant advantage in coding interviews, especially for complex algorithmic challenges.
4. Divide and Conquer: Breaking Problems into Manageable Pieces
The Divide and Conquer technique involves breaking a problem into smaller, more manageable subproblems, solving these subproblems, and then combining their solutions to solve the original problem. This approach is particularly useful for problems that can be naturally divided into similar, smaller instances of the same problem.
When to Use Divide and Conquer:
- Problems that can be divided into similar subproblems
- When the solution to the original problem can be constructed from solutions to subproblems
- For problems that benefit from parallel processing
- When looking for more efficient alternatives to brute force approaches
Example: Merge Sort
Merge Sort is a classic example of the Divide and Conquer approach. It divides the array into two halves, recursively sorts them, and then merges the sorted halves:
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] <= right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
# Example usage
arr = [38, 27, 43, 3, 9, 82, 10]
sorted_arr = merge_sort(arr)
print(f"Sorted array: {sorted_arr}")
Merge Sort has a time complexity of O(n log n), which is more efficient than simple sorting algorithms like Bubble Sort or Insertion Sort for large datasets.
Pros and Cons of Divide and Conquer:
Pros:
- Can lead to efficient algorithms for complex problems
- Often results in logarithmic time complexity
- Suitable for parallel processing
- Can simplify the problem-solving process for certain types of problems
Cons:
- May require more memory due to recursive calls
- Not all problems can be efficiently divided into subproblems
- The overhead of dividing and combining can sometimes outweigh the benefits for small input sizes
The Divide and Conquer technique is a powerful tool in algorithm design and problem-solving. It’s particularly useful for developing efficient sorting and searching algorithms, as well as for solving problems in computational geometry and matrix multiplication.
5. Two-Pointer Technique: Efficient Array Manipulation
The Two-Pointer technique is a simple and effective way to solve problems involving arrays or linked lists. It involves using two pointers that either move towards each other or in the same direction to solve the problem efficiently.
When to Use the Two-Pointer Technique:
- Problems involving sorted arrays or linked lists
- When searching for pairs in an array
- For problems that require in-place array manipulation
- When looking for subarrays or subsequences with certain properties
Example: Two Sum II – Input Array is Sorted
Let’s solve the “Two Sum II” problem: Given a sorted array of integers, find two numbers such that they add up to a specific target number.
def two_sum_sorted(numbers, target):
left, right = 0, len(numbers) - 1
while left < right:
current_sum = numbers[left] + numbers[right]
if current_sum == target:
return [left + 1, right + 1] # Adding 1 for 1-based indexing
elif current_sum < target:
left += 1
else:
right -= 1
return [] # No solution found
# Example usage
numbers = [2, 7, 11, 15]
target = 9
result = two_sum_sorted(numbers, target)
print(f"Indices of the two numbers: {result}")
This solution has a time complexity of O(n) and uses constant extra space, making it very efficient.
Pros and Cons of the Two-Pointer Technique:
Pros:
- Often results in linear time complexity
- Uses constant extra space
- Effective for in-place array manipulation
- Simple to implement and understand
Cons:
- Limited to specific types of problems, usually involving arrays or linked lists
- May not be applicable to unsorted data structures without preprocessing
- Can be tricky to implement correctly for more complex problems
The Two-Pointer technique is a valuable tool for solving array-based problems efficiently. It’s particularly useful in coding interviews where time and space complexity are important considerations.
Putting It All Together: A Structured Approach to Problem-Solving
Now that we’ve explored these five proven techniques, let’s discuss how to approach a coding interview problem systematically:
- Understand the problem: Carefully read the problem statement and ask clarifying questions if needed.
- Analyze the input and output: Identify the data structures involved and the expected format of the solution.
- Consider constraints: Take note of any time or space complexity requirements.
- Start with a brute force solution: This helps you understand the problem better and provides a baseline for optimization.
- Optimize: Consider if any of the techniques we’ve discussed (greedy, dynamic programming, divide and conquer, or two-pointer) could improve the solution.
- Implement: Write clean, well-commented code for your chosen approach.
- Test: Use example inputs and edge cases to verify your solution’s correctness.
- Analyze complexity: Discuss the time and space complexity of your solution.
- Consider further optimizations: If time permits, think about alternative approaches or potential improvements.
Conclusion: Mastering the Art of Problem-Solving
Coding interviews can be challenging, but with these five proven techniques in your toolkit, you’ll be well-equipped to tackle a wide range of problems. Remember, the key to success is not just knowing these techniques, but understanding when and how to apply them effectively.
As you practice, you’ll develop an intuition for which approach is best suited for different types of problems. Don’t be discouraged if you don’t immediately recognize the optimal solution – even experienced developers often start with a brute force approach and iteratively optimize their solution.
Keep practicing, stay curious, and always be open to learning new problem-solving strategies. With time and dedication, you’ll find yourself approaching coding interview problems with confidence and creativity.
Happy coding, and best of luck in your future interviews!