The Art of Breaking Down Complex Problems: A Step-by-Step Approach to Coding Challenges
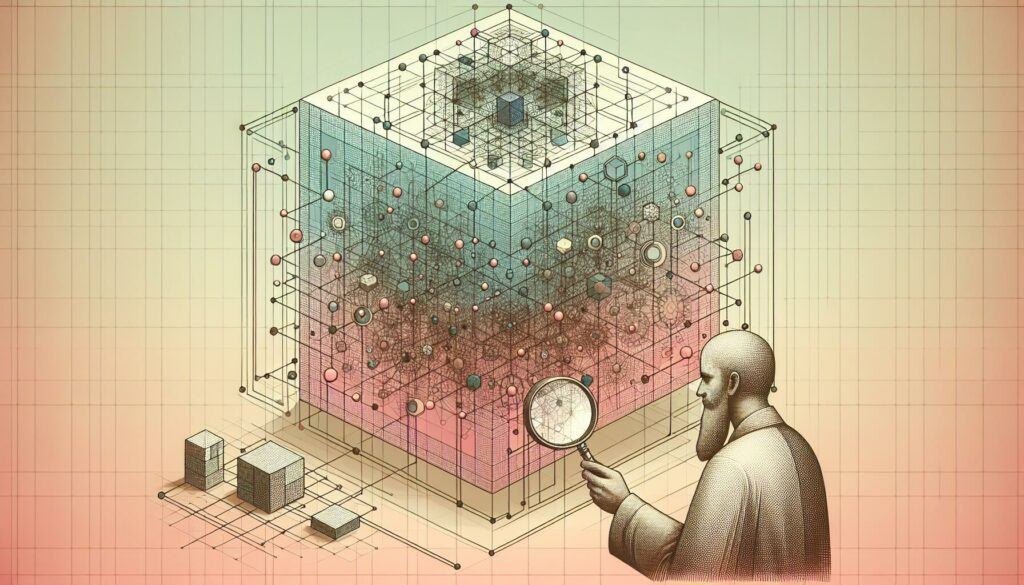
In the world of programming, tackling complex problems is an everyday occurrence. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at major tech companies, the ability to break down intricate problems into manageable parts is an invaluable skill. This approach not only leads to faster solutions but also enhances your overall problem-solving abilities. In this comprehensive guide, we’ll explore the art of deconstructing complex coding challenges and provide a framework for dividing problems into sub-problems, ultimately improving your coding prowess.
The Importance of Problem Decomposition in Coding
Before we dive into the specifics of breaking down complex problems, let’s understand why this skill is crucial for programmers:
- Clarity and Focus: Decomposing a problem helps you gain a clearer understanding of the challenge at hand, allowing you to focus on one aspect at a time.
- Manageable Complexity: Large, complex problems can be overwhelming. Breaking them down makes them more approachable and less daunting.
- Efficient Problem-Solving: By tackling smaller sub-problems, you can often find solutions more quickly and efficiently.
- Improved Code Organization: Decomposition naturally leads to better-structured code, with distinct functions or modules for each sub-problem.
- Enhanced Debugging: When issues arise, it’s easier to isolate and fix problems in smaller, well-defined components.
- Collaboration: Broken-down problems are easier to distribute among team members, facilitating better collaboration.
A Framework for Dividing Coding Problems into Sub-Problems
Now that we understand the importance of problem decomposition, let’s explore a step-by-step framework for breaking down complex coding challenges:
1. Understand the Problem
Before you can effectively break down a problem, you need to fully grasp what it’s asking. This step involves:
- Reading the problem statement carefully, multiple times if necessary.
- Identifying the inputs and expected outputs.
- Clarifying any ambiguities or assumptions.
- Considering edge cases and potential constraints.
For example, if you’re tasked with creating a function to find the longest palindromic substring in a given string, you’d want to understand:
- What constitutes a palindrome?
- Should the function be case-sensitive?
- How should it handle empty strings or strings with no palindromes?
- Are there any constraints on the input string’s length?
2. Identify the Main Components
Once you have a clear understanding of the problem, start identifying the main components or steps required to solve it. For our palindromic substring example, the main components might be:
- Generating all possible substrings
- Checking if a substring is a palindrome
- Keeping track of the longest palindromic substring found
3. Break Down Each Component
Now, take each main component and break it down further into smaller, more manageable tasks. For instance:
Generating all possible substrings:
- Implement nested loops to iterate through the string
- Extract substrings of various lengths
Checking if a substring is a palindrome:
- Compare characters from the start and end, moving inwards
- Handle even and odd-length palindromes
Keeping track of the longest palindromic substring:
- Initialize a variable to store the longest palindrome
- Update this variable whenever a longer palindrome is found
4. Determine the Order of Execution
Decide on the logical order in which these sub-problems should be solved. In our example, a possible order could be:
- Initialize variables to store the result
- Iterate through the string to generate substrings
- For each substring, check if it’s a palindrome
- If it is, compare its length with the current longest palindrome
- Update the result if a longer palindrome is found
- Return the final result
5. Implement Each Sub-Problem
Now that you have a clear roadmap, start implementing each sub-problem. This is where you’ll write the actual code for each component. Let’s look at a Python implementation for our palindromic substring example:
def longest_palindromic_substring(s):
def is_palindrome(substr):
return substr == substr[::-1]
longest = ""
for i in range(len(s)):
for j in range(i, len(s)):
substr = s[i:j+1]
if is_palindrome(substr) and len(substr) > len(longest):
longest = substr
return longest
# Test the function
print(longest_palindromic_substring("babad")) # Output: "bab" or "aba"
print(longest_palindromic_substring("cbbd")) # Output: "bb"
6. Test and Refine
After implementing your solution, it’s crucial to test it thoroughly:
- Start with the example cases provided in the problem statement.
- Test edge cases (empty string, single character, no palindromes, etc.).
- Use larger inputs to check for performance issues.
If you encounter any issues or if the performance is not satisfactory, go back to your sub-problems and refine them. In our example, we might realize that our current solution has a time complexity of O(n^3), which is not optimal for large inputs. This realization would lead us to explore more efficient algorithms, such as expanding around centers or using dynamic programming.
Advanced Techniques for Problem Decomposition
As you become more proficient in breaking down problems, you can employ more advanced techniques to further enhance your problem-solving skills:
1. Divide and Conquer
This technique involves breaking the problem into smaller, similar sub-problems, solving them independently, and then combining the results. It’s particularly useful for problems that exhibit recursive structures.
Example: Merge Sort algorithm
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
# Test the function
print(merge_sort([38, 27, 43, 3, 9, 82, 10]))
# Output: [3, 9, 10, 27, 38, 43, 82]
2. Dynamic Programming
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems. It is particularly useful when subproblems overlap and have optimal substructure.
Example: Fibonacci sequence using dynamic programming
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
# Test the function
print(fibonacci(10)) # Output: 55
3. Greedy Algorithms
Greedy algorithms make the locally optimal choice at each step, hoping to find a global optimum. They can be useful for breaking down problems where a series of choices needs to be made.
Example: Coin change problem using a greedy approach
def coin_change_greedy(amount, coins):
coins.sort(reverse=True)
result = []
for coin in coins:
while amount >= coin:
result.append(coin)
amount -= coin
return result if amount == 0 else []
# Test the function
print(coin_change_greedy(63, [25, 10, 5, 1]))
# Output: [25, 25, 10, 1, 1, 1]
Common Pitfalls and How to Avoid Them
While breaking down complex problems is a powerful technique, there are some common pitfalls to be aware of:
1. Over-complicating the Solution
Sometimes, in an attempt to break down a problem, we might create unnecessary complexity. Always strive for the simplest solution that solves the problem effectively.
How to avoid: After breaking down the problem, take a step back and see if there’s a simpler approach. Sometimes, a single, well-designed function can be more effective than multiple intricate sub-functions.
2. Losing Sight of the Big Picture
When focusing on individual sub-problems, it’s easy to lose track of the overall goal. This can lead to solutions that solve parts of the problem but fail to address the main objective.
How to avoid: Regularly refer back to the original problem statement. After solving each sub-problem, check if it aligns with and contributes to the main goal.
3. Inefficient Decomposition
Breaking down a problem inefficiently can lead to redundant code or suboptimal solutions. This is particularly problematic when dealing with large datasets or time-sensitive operations.
How to avoid: Analyze your decomposition for any repeated work or unnecessary steps. Look for opportunities to optimize by combining or eliminating sub-problems.
4. Ignoring Edge Cases
When breaking down a problem, it’s easy to focus on the “happy path” and overlook edge cases or exceptional scenarios.
How to avoid: For each sub-problem, explicitly consider and handle edge cases. Create a list of potential edge cases early in the problem-solving process and ensure your solution addresses them.
Applying Problem Decomposition in Real-World Scenarios
The skills you develop in breaking down complex coding problems have applications far beyond coding challenges or technical interviews. Let’s explore how this approach can be applied in real-world scenarios:
1. Building Large-Scale Applications
When developing complex applications, breaking down the project into smaller, manageable modules is crucial. This could involve:
- Separating the application into front-end and back-end components
- Dividing the back-end into microservices
- Breaking down the front-end into reusable components
Example: E-commerce Platform
// Simplified structure of an e-commerce platform
// User Service
class UserService {
register(user) { /* ... */ }
login(credentials) { /* ... */ }
updateProfile(userId, data) { /* ... */ }
}
// Product Service
class ProductService {
addProduct(product) { /* ... */ }
getProduct(productId) { /* ... */ }
searchProducts(query) { /* ... */ }
}
// Order Service
class OrderService {
createOrder(userId, items) { /* ... */ }
getOrderStatus(orderId) { /* ... */ }
cancelOrder(orderId) { /* ... */ }
}
// Payment Service
class PaymentService {
processPayment(orderId, paymentDetails) { /* ... */ }
refundPayment(orderId) { /* ... */ }
}
// Main Application
class ECommerceApp {
constructor() {
this.userService = new UserService();
this.productService = new ProductService();
this.orderService = new OrderService();
this.paymentService = new PaymentService();
}
// Application logic using the services
}
2. Data Analysis and Processing
When working with large datasets or complex data processing tasks, breaking down the analysis into smaller steps can make the process more manageable and less error-prone.
Example: Analyzing Sales Data
import pandas as pd
import matplotlib.pyplot as plt
def analyze_sales_data(file_path):
# Step 1: Load and clean data
df = load_data(file_path)
df = clean_data(df)
# Step 2: Calculate summary statistics
summary_stats = calculate_summary_stats(df)
# Step 3: Perform time series analysis
time_series_results = analyze_time_series(df)
# Step 4: Segment customers
customer_segments = segment_customers(df)
# Step 5: Visualize results
visualize_results(summary_stats, time_series_results, customer_segments)
def load_data(file_path):
return pd.read_csv(file_path)
def clean_data(df):
# Remove duplicates, handle missing values, etc.
return df
def calculate_summary_stats(df):
# Calculate total sales, average order value, etc.
return {}
def analyze_time_series(df):
# Perform time series decomposition, trend analysis, etc.
return {}
def segment_customers(df):
# Use clustering algorithms to segment customers
return {}
def visualize_results(summary_stats, time_series_results, customer_segments):
# Create various plots and charts
pass
# Run the analysis
analyze_sales_data("sales_data.csv")
3. Algorithmic Trading Strategies
In financial technology, breaking down complex trading strategies into smaller components can help in developing more robust and maintainable systems.
Example: Simple Moving Average Crossover Strategy
import pandas as pd
import numpy as np
class SMAStrategy:
def __init__(self, short_window, long_window):
self.short_window = short_window
self.long_window = long_window
def calculate_signals(self, data):
# Calculate short and long moving averages
data['SMA_Short'] = data['Close'].rolling(window=self.short_window).mean()
data['SMA_Long'] = data['Close'].rolling(window=self.long_window).mean()
# Generate buy/sell signals
data['Signal'] = np.where(data['SMA_Short'] > data['SMA_Long'], 1, 0)
data['Position'] = data['Signal'].diff()
return data
def backtest(self, data):
signals = self.calculate_signals(data)
# Calculate returns
signals['Returns'] = np.log(signals['Close'] / signals['Close'].shift(1))
signals['Strategy_Returns'] = signals['Position'].shift(1) * signals['Returns']
# Calculate performance metrics
total_return = signals['Strategy_Returns'].sum()
sharpe_ratio = signals['Strategy_Returns'].mean() / signals['Strategy_Returns'].std() * np.sqrt(252)
return {'Total Return': total_return, 'Sharpe Ratio': sharpe_ratio}
# Usage
data = pd.read_csv('stock_data.csv', parse_dates=['Date'], index_col='Date')
strategy = SMAStrategy(short_window=50, long_window=200)
results = strategy.backtest(data)
print(results)
Conclusion: Mastering the Art of Problem Decomposition
Breaking down complex problems into smaller, manageable parts is a fundamental skill in programming and problem-solving. By following the framework outlined in this article and practicing regularly, you can significantly improve your ability to tackle complex coding challenges, whether you’re preparing for technical interviews at major tech companies or working on large-scale software projects.
Remember, the key steps in this process are:
- Thoroughly understand the problem
- Identify the main components
- Break down each component into smaller tasks
- Determine the logical order of execution
- Implement each sub-problem
- Test and refine your solution
As you become more proficient, you’ll find that this approach not only leads to faster solutions but also results in more organized, maintainable, and efficient code. Moreover, the skills you develop in problem decomposition will serve you well beyond coding, applying to various aspects of project management and complex problem-solving in your career.
Keep practicing, stay curious, and remember that even the most complex problems can be solved when broken down into manageable pieces. Happy coding!