Mastering Problem-Solving Skills for Coding Interviews: A Comprehensive Guide
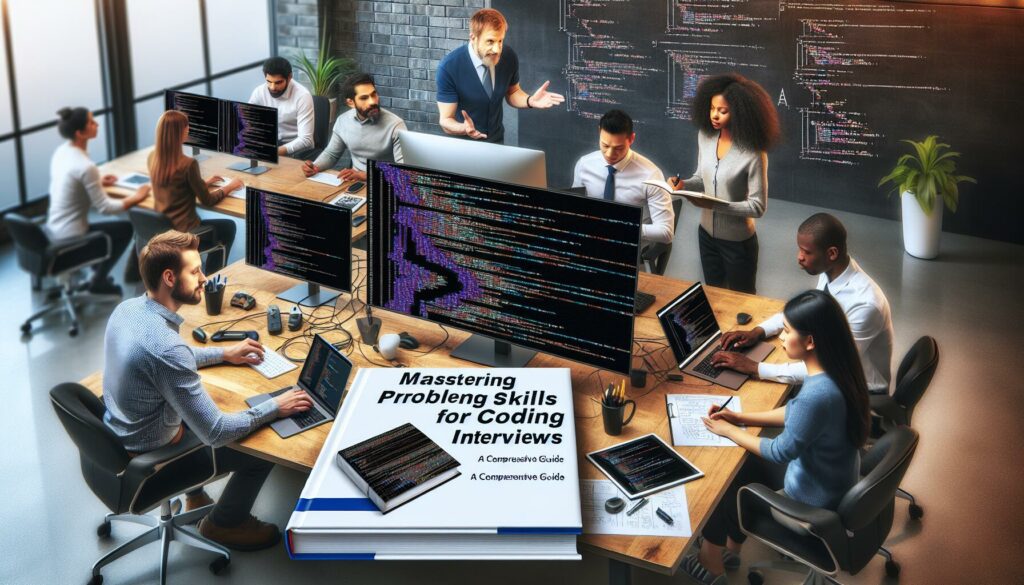
Coding interviews can be daunting, especially when faced with complex problems that require quick thinking and efficient solutions. However, with the right approach and consistent practice, you can significantly improve your problem-solving skills and increase your chances of success. In this comprehensive guide, we’ll explore various strategies and techniques to help you excel in coding interviews and become a more proficient problem solver.
1. The Importance of Regular Practice
One of the most crucial aspects of improving your problem-solving skills is consistent practice. Just like any other skill, the more you practice, the better you become. Here are some ways to incorporate regular practice into your routine:
1.1. Utilize Online Coding Platforms
Take advantage of popular coding platforms that offer a wide range of problems to solve. Some of the best options include:
- LeetCode: Known for its extensive collection of algorithm and data structure problems, LeetCode is a favorite among many developers preparing for technical interviews.
- HackerRank: Offers a variety of coding challenges across different domains, including algorithms, data structures, and specific programming languages.
- CodeSignal: Provides a mix of coding challenges and real-world programming tasks, helping you develop practical problem-solving skills.
Aim to solve at least one problem daily on these platforms. This consistent practice will help you build a strong foundation and expose you to various problem-solving techniques.
1.2. Create a Study Schedule
Develop a structured study plan that allocates specific time slots for problem-solving practice. For example:
- Dedicate 1-2 hours each day to solving coding problems
- Alternate between easy, medium, and hard difficulty levels
- Focus on specific topics or algorithms each week
By following a schedule, you’ll ensure that you’re consistently working on improving your skills and covering a wide range of problem types.
2. Master Common Algorithms and Data Structures
A solid understanding of fundamental algorithms and data structures is essential for tackling coding interview problems effectively. Familiarize yourself with the following concepts:
2.1. Arrays and Strings
Arrays and strings are the building blocks of many coding problems. Make sure you’re comfortable with:
- Array manipulation techniques (e.g., two-pointer approach, sliding window)
- String operations (e.g., substring search, pattern matching)
- Common array algorithms (e.g., kadane’s algorithm for maximum subarray sum)
2.2. Linked Lists
Understand the fundamentals of linked lists and practice common operations such as:
- Traversal and reversal
- Detecting cycles
- Merging and sorting linked lists
2.3. Trees and Graphs
Tree and graph problems are frequently encountered in coding interviews. Focus on:
- Tree traversal algorithms (e.g., inorder, preorder, postorder)
- Binary search trees and their properties
- Graph representation and traversal (e.g., DFS, BFS)
- Shortest path algorithms (e.g., Dijkstra’s, Bellman-Ford)
2.4. Sorting and Searching Algorithms
Be familiar with various sorting and searching techniques, including:
- Comparison-based sorting algorithms (e.g., quicksort, mergesort)
- Linear-time sorting algorithms (e.g., counting sort, radix sort)
- Binary search and its variations
2.5. Dynamic Programming
Dynamic programming is a powerful technique for solving optimization problems. Study common DP patterns and practice problems like:
- Fibonacci sequence
- Longest common subsequence
- Knapsack problem
3. Develop a Problem-Solving Framework
Having a structured approach to problem-solving can greatly improve your efficiency and effectiveness during coding interviews. Here’s a framework you can follow:
3.1. Understand the Problem Thoroughly
Before diving into coding, make sure you have a clear understanding of the problem statement. Ask clarifying questions if needed, and repeat the problem back to the interviewer to confirm your understanding.
3.2. Identify Inputs and Expected Outputs
Clearly define the input parameters and the expected output format. This will help you structure your solution and avoid misunderstandings.
3.3. Break Down the Problem
Divide the problem into smaller, manageable steps. This approach makes complex problems less overwhelming and helps you identify potential subproblems that can be solved independently.
3.4. Consider Edge Cases
Think about potential edge cases and how your solution would handle them. This demonstrates attention to detail and helps prevent bugs in your code.
3.5. Analyze Time and Space Complexity
Consider the efficiency of your solution in terms of both time and space complexity. Be prepared to discuss trade-offs between different approaches.
4. Start with Brute Force
When faced with a challenging problem, it’s often helpful to start with a simple, brute force solution. This approach has several benefits:
- It helps you understand the problem better by working through a concrete example
- It provides a baseline solution that you can optimize later
- It demonstrates to the interviewer that you can quickly come up with a working solution
After implementing the brute force approach, you can then focus on optimizing and improving your solution.
5. Develop Pattern Recognition Skills
As you solve more problems, you’ll start to notice common patterns and techniques that can be applied to similar problems. Some common patterns to look out for include:
- Two-pointer technique
- Sliding window
- Divide and conquer
- Greedy algorithms
- Backtracking
Keep a notebook or digital document where you can record these patterns and the types of problems they’re useful for. This will help you quickly identify appropriate techniques when faced with new problems.
6. Practice Thinking Aloud
During coding interviews, it’s crucial to communicate your thought process clearly. Practice explaining your approach out loud as you solve problems. This skill has several benefits:
- It helps interviewers understand your reasoning and problem-solving approach
- It allows interviewers to provide hints or guidance if you’re stuck
- It clarifies your own thinking and can help you catch mistakes early
To improve your ability to think aloud:
- Practice solving problems while explaining your thought process to a friend or rubber duck
- Record yourself solving problems and review the recordings to identify areas for improvement
- Participate in mock interviews where you can get feedback on your communication skills
7. Simulate Interview Conditions
To better prepare for the pressure of real coding interviews, it’s important to simulate interview conditions during your practice sessions. Here are some ways to do this:
7.1. Time Yourself
Set time limits for solving problems, similar to what you might encounter in an actual interview. This will help you improve your speed and learn to manage your time effectively.
7.2. Use a Whiteboard or Simple Text Editor
Many coding interviews are conducted on a whiteboard or in a simple text editor without auto-completion or syntax highlighting. Practice solving problems in these environments to get comfortable working without the assistance of an IDE.
7.3. Conduct Mock Interviews
Ask friends or use online platforms that offer mock interview services to simulate real interview experiences. This will help you get comfortable with the interview format and receive valuable feedback.
8. Review and Learn from Solutions
After solving a problem, take the time to review your solution and learn from other approaches. This process can significantly enhance your problem-solving skills:
8.1. Analyze Your Own Solution
Reflect on your problem-solving process and identify areas for improvement. Ask yourself:
- Could I have approached the problem more efficiently?
- Did I consider all edge cases?
- Is there a way to optimize the time or space complexity?
8.2. Study Other Solutions
Look at solutions provided by others, especially those that are more efficient or elegant than your own. Try to understand the reasoning behind these solutions and how you can apply similar techniques in the future.
8.3. Implement Alternative Solutions
After studying other approaches, try implementing them yourself. This hands-on practice will help you internalize new techniques and improve your coding skills.
9. Implement Solutions from Scratch
While it’s tempting to rely on built-in functions or libraries, it’s crucial to be able to implement common algorithms and data structures from scratch. This demonstrates a deeper understanding of the underlying concepts and can be particularly impressive in coding interviews.
Practice implementing the following from scratch:
- Sorting algorithms (e.g., quicksort, mergesort)
- Data structures (e.g., linked list, binary search tree, hash table)
- String manipulation functions (e.g., string reversal, substring search)
10. Develop a Growth Mindset
Lastly, it’s important to approach problem-solving with a growth mindset. Remember that improving your skills takes time and effort. Here are some tips to maintain a positive attitude:
- Embrace challenges as opportunities to learn and grow
- View mistakes as valuable learning experiences
- Celebrate small victories and progress along the way
- Stay persistent and don’t get discouraged by difficult problems
Conclusion
Mastering problem-solving skills for coding interviews is a journey that requires dedication, practice, and a systematic approach. By following the strategies outlined in this guide – from consistent practice and mastering fundamental concepts to developing a structured problem-solving framework and simulating interview conditions – you’ll be well-equipped to tackle even the most challenging coding interview questions.
Remember that improvement comes with time and effort. Stay motivated, keep practicing, and approach each problem as an opportunity to learn and grow. With persistence and the right mindset, you’ll be well on your way to becoming a skilled problem solver and acing your coding interviews.
Good luck with your preparation, and may your future coding interviews be successful!