The Power of Pen and Paper in Coding Interviews: A Comprehensive Guide
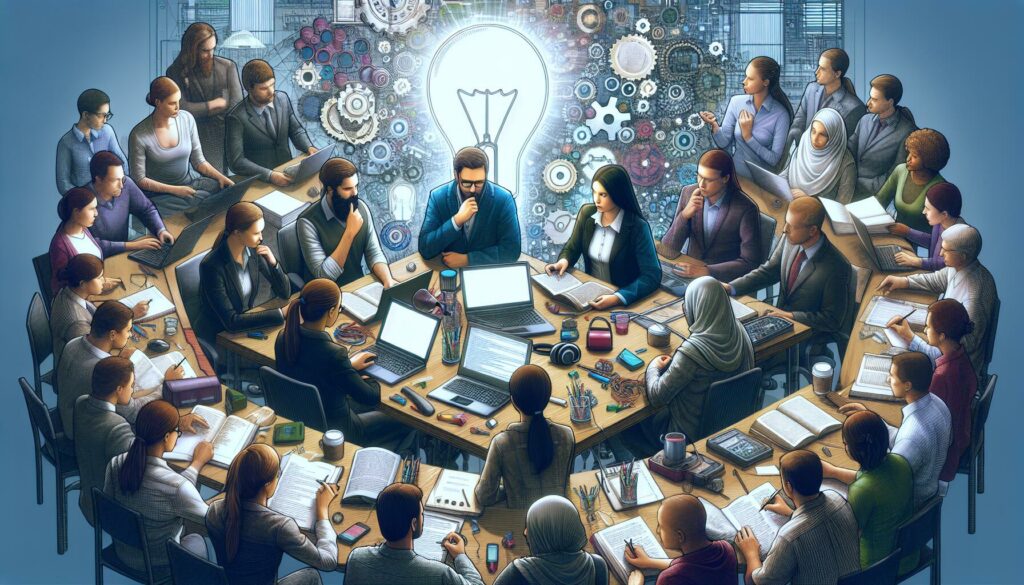
In the digital age, where coding is predominantly done on computers, the idea of using pen and paper or a whiteboard during a coding interview might seem outdated. However, this traditional approach remains a crucial skill for aspiring software developers. This comprehensive guide will explore the benefits of using pen and paper (or whiteboard) in coding interviews, techniques to master this skill, and how it can give you an edge in your next technical interview.
1. Why Use Pen and Paper in Coding Interviews?
Despite the prevalence of digital tools, many companies still incorporate pen-and-paper or whiteboard coding exercises in their interview process. Here’s why:
1.1. Focus on Core Concepts
Using pen and paper forces you to focus on the fundamental concepts of programming rather than relying on IDE features or autocomplete. This approach tests your understanding of algorithms, data structures, and problem-solving skills at a deeper level.
1.2. Communication Skills
Writing code by hand allows interviewers to observe your thought process in real-time. It provides an opportunity to demonstrate your ability to explain your ideas clearly and concisely, a crucial skill in collaborative development environments.
1.3. Adaptability
The ability to code without a computer showcases your adaptability and versatility as a programmer. It demonstrates that you can think on your feet and aren’t dependent on specific tools or environments to solve problems.
1.4. Simulates Real-world Scenarios
In many development roles, you’ll need to sketch out ideas or explain concepts to colleagues using whiteboards or paper. The interview process mimics these real-world scenarios.
2. Techniques for Effective Pen-and-Paper Coding
To excel in pen-and-paper coding interviews, consider the following techniques:
2.1. Practice Writing Code by Hand
Regular practice is key. Set aside time to solve coding problems using only pen and paper. This will help you become comfortable with the process and improve your handwriting speed and clarity.
2.2. Use Proper Indentation and Spacing
Even when writing by hand, maintain good coding practices. Use consistent indentation and leave adequate space between lines to make your code readable and allow for additions or corrections.
2.3. Develop Shorthand for Common Structures
Create abbreviations or symbols for frequently used programming structures to save time. For example:
- “->” for arrow functions in JavaScript
- “fn” for function
- “cls” for class
- “arr” for array
2.4. Use Pseudo-code
Start with pseudo-code to outline your approach before diving into the actual implementation. This helps organize your thoughts and provides a roadmap for your solution.
2.5. Draw Diagrams
Utilize diagrams to visualize complex data structures or algorithms. This can be particularly helpful for problems involving trees, graphs, or linked lists.
3. Common Challenges and How to Overcome Them
While coding on paper offers many benefits, it also presents unique challenges. Here’s how to address them:
3.1. Syntax Errors
Without a compiler to catch syntax errors, it’s easy to make mistakes. Combat this by:
- Reviewing your code carefully after writing
- Practicing writing syntactically correct code by hand
- Focusing on logical correctness rather than perfect syntax during the interview
3.2. Limited Space
Paper or whiteboards have finite space. To manage this:
- Write small but legibly
- Use abbreviations where appropriate
- Plan your space usage before starting
3.3. Time Management
Writing by hand is slower than typing. Improve your time management by:
- Practicing timed coding exercises
- Using pseudo-code to plan before writing detailed implementation
- Communicating your thought process as you write to show progress
4. Best Practices for Whiteboard Coding
If your interview involves whiteboard coding, keep these tips in mind:
4.1. Use the Entire Board
Don’t confine yourself to a small area. Use the entire whiteboard to your advantage, organizing your code and thoughts spatially.
4.2. Write Legibly
Ensure your handwriting is clear and easy to read. This is crucial for both you and your interviewer to follow the code.
4.3. Leave Space for Corrections
Anticipate the need for changes or additions. Leave space between lines and sections of your code.
4.4. Use Colors Wisely
If multiple colors are available, use them strategically to highlight different parts of your code or emphasize important concepts.
5. The Role of Sketches in Problem-Solving
Beyond writing code, sketches play a crucial role in problem-solving during interviews. Here’s how to leverage them effectively:
5.1. Visualize the Problem
Start by drawing a visual representation of the problem. This could be a flowchart, a diagram of data structures, or a simple illustration of the scenario.
5.2. Outline the Solution
Use sketches to create a high-level outline of your solution before diving into the code. This can help you organize your thoughts and spot potential issues early.
5.3. Iterate on Your Ideas
Don’t be afraid to draw multiple versions of your solution. Sketching allows for quick iterations and comparisons between different approaches.
5.4. Communicate Complex Concepts
Some ideas are easier to explain visually than verbally. Use sketches to clarify complex algorithms or data transformations to your interviewer.
6. Preparing for Pen-and-Paper Coding Interviews
To excel in pen-and-paper coding interviews, incorporate these preparation strategies:
6.1. Practice Regularly
Set aside time each week to solve coding problems using only pen and paper. This will help you become comfortable with the process and improve your speed and accuracy.
6.2. Study Common Algorithms and Data Structures
Focus on understanding and implementing fundamental algorithms and data structures without the aid of a computer. This includes:
- Sorting algorithms (e.g., quicksort, mergesort)
- Search algorithms (e.g., binary search)
- Data structures (e.g., linked lists, trees, graphs)
- Dynamic programming problems
6.3. Review Basic Syntax
Ensure you’re comfortable writing basic syntax for your preferred programming language without relying on autocomplete or documentation.
6.4. Practice Explaining Your Thought Process
Get comfortable talking through your problem-solving approach. Practice explaining your code and decisions to a friend or mentor.
7. Leveraging Pen-and-Paper Skills in Digital Interviews
Even in digital or remote interviews, the skills developed through pen-and-paper practice remain valuable:
7.1. Online Whiteboard Tools
Many remote interviews use digital whiteboard tools. The skills you’ve developed with physical whiteboards transfer directly to these platforms.
7.2. Pseudo-code in Code Editors
When using online code editors during interviews, start with pseudo-code comments to outline your approach, mimicking the planning stage of pen-and-paper coding.
7.3. Verbal Communication
The practice of explaining your thought process while writing code by hand enhances your ability to articulate your ideas clearly during any type of interview.
8. Code Examples: From Paper to Digital
Let’s look at how a hand-written solution might translate to digital code. Consider a simple problem: reversing a string.
Hand-written pseudo-code might look like this:
function reverseString(str):
initialize empty result string
for each char in str (from end to start):
add char to result
return result
This could then be implemented in Python as:
def reverse_string(s):
result = ""
for i in range(len(s) - 1, -1, -1):
result += s[i]
return result
# Test the function
print(reverse_string("Hello, World!")) # Output: !dlroW ,olleH
Notice how the hand-written pseudo-code captures the essence of the algorithm, making the transition to actual code straightforward.
9. The Future of Coding Interviews
While the tech industry continues to evolve, the fundamental skills tested by pen-and-paper coding remain relevant:
9.1. Emphasis on Problem-Solving
Regardless of the medium, the ability to break down problems and devise efficient solutions is always valuable.
9.2. Adaptation of Traditional Methods
Many companies are finding ways to incorporate the benefits of hand-coding into modern interview processes, such as using tablet devices or digital whiteboards.
9.3. Balancing Technical Skills with Communication
The trend is moving towards a holistic evaluation of candidates, where communication skills are valued alongside technical proficiency.
10. Conclusion
Mastering the art of pen-and-paper coding is a valuable skill that can significantly boost your performance in technical interviews. It sharpens your problem-solving abilities, improves your communication skills, and demonstrates your adaptability as a programmer.
Remember, the goal is not to write perfect, compilable code on paper, but to showcase your thought process, problem-solving approach, and fundamental understanding of programming concepts. By incorporating the techniques and practices outlined in this guide, you’ll be well-prepared to tackle any coding interview, whether it involves a whiteboard, pen and paper, or a digital platform.
Embrace the challenge of coding without a computer, and you’ll find that it not only prepares you for interviews but also makes you a more well-rounded and adaptable software developer in the long run.