Breaking Down Complex Problems: A Programmer’s Approach
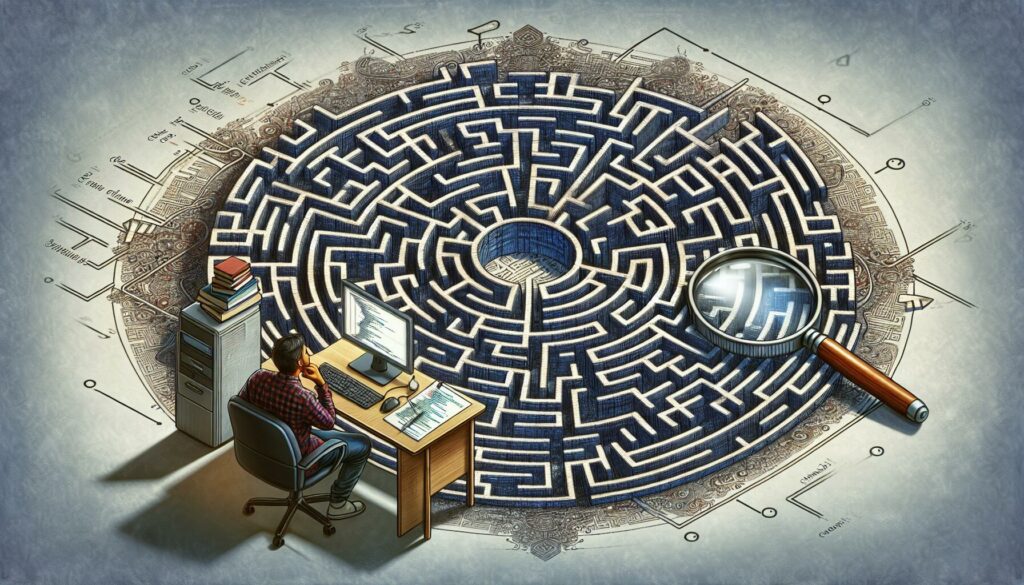
1. Understanding the Problem
Before you can start breaking down a complex problem, it’s crucial to fully understand what you’re trying to solve. This initial step involves:
- Clearly defining the problem statement
- Identifying the inputs and expected outputs
- Recognizing any constraints or limitations
- Determining the scope of the problem
Take the time to ask questions, gather information, and ensure you have a comprehensive understanding of the problem at hand. This foundational step will guide your approach to breaking down the problem and developing a solution.
Example: Building a Social Media Analytics Tool
Let’s say you’ve been tasked with building a social media analytics tool. Your initial problem statement might look like this:
“Create a tool that analyzes social media data to provide insights on user engagement, content performance, and audience demographics for multiple platforms.”
By breaking this down further, you can identify key components:
- Inputs: Raw social media data from multiple platforms
- Outputs: User engagement metrics, content performance analytics, audience demographic information
- Constraints: API rate limits, data privacy regulations, scalability requirements
- Scope: Multiple social media platforms (e.g., Twitter, Facebook, Instagram)
2. Decomposition: Breaking the Problem into Smaller Parts
Once you have a clear understanding of the problem, the next step is to break it down into smaller, more manageable components. This process is called decomposition, and it’s a fundamental technique in problem-solving and software development.
Techniques for Decomposition
- Functional Decomposition: Break the problem down based on different functions or features of the system.
- Object-Oriented Decomposition: Identify the main objects or entities in the problem and their relationships.
- Data Flow Decomposition: Analyze the flow of data through the system and break it down accordingly.
- Event-Driven Decomposition: Identify the main events or triggers in the system and how they relate to different components.
Example: Decomposing the Social Media Analytics Tool
Let’s apply functional decomposition to our social media analytics tool:
- Data Collection
- API Integration for each platform
- Data storage and management
- Data Processing
- Data cleaning and normalization
- Metric calculation (engagement rates, reach, etc.)
- Analysis
- Content performance analysis
- Audience demographics analysis
- Trend identification
- Visualization
- Dashboard creation
- Chart and graph generation
- User Interface
- Front-end design
- User authentication and management
By breaking down the problem into these smaller components, you can now focus on solving each part individually, making the overall task much more manageable.
3. Identifying Patterns and Similarities
As you break down complex problems, you’ll often notice patterns or similarities between different components. Recognizing these patterns can help you develop more efficient solutions and potentially reuse code or algorithms across different parts of your project.
Common Patterns in Programming
- Design Patterns: Reusable solutions to common problems in software design (e.g., Singleton, Factory, Observer)
- Algorithmic Patterns: Common approaches to solving specific types of problems (e.g., divide and conquer, dynamic programming, greedy algorithms)
- Architectural Patterns: High-level structures for organizing code and systems (e.g., MVC, microservices, layered architecture)
Example: Identifying Patterns in the Social Media Analytics Tool
In our social media analytics tool, we might identify the following patterns:
- API Integration: The process of integrating with different social media APIs will likely follow a similar pattern for each platform. We could create a generic API integration module that can be customized for each specific platform.
- Data Processing: The steps for cleaning and normalizing data might be similar across different data sources. We could create a reusable data processing pipeline that can handle various input formats.
- Visualization: Many of the charts and graphs used to display analytics will share common elements. We could create a set of reusable visualization components that can be easily customized for different metrics.
4. Prioritizing and Ordering Tasks
Once you’ve broken down the problem into smaller components and identified patterns, it’s important to prioritize and order the tasks. This helps you focus on the most critical parts of the problem first and ensures that you’re making steady progress towards your goal.
Prioritization Techniques
- MoSCoW Method: Categorize tasks as Must have, Should have, Could have, or Won’t have
- Eisenhower Matrix: Prioritize tasks based on urgency and importance
- Value vs. Effort: Assess tasks based on the value they provide relative to the effort required
- Dependencies: Identify which tasks depend on others and order them accordingly
Example: Prioritizing Tasks for the Social Media Analytics Tool
Let’s prioritize the main components of our social media analytics tool using the MoSCoW method:
- Must have:
- Data Collection (API Integration for at least one platform)
- Basic Data Processing (cleaning and normalization)
- Simple Analysis (engagement metrics)
- Basic Visualization (simple charts and graphs)
- Minimal User Interface
- Should have:
- API Integration for additional platforms
- Advanced Data Processing (more complex metrics)
- Content Performance Analysis
- More Advanced Visualizations
- Could have:
- Audience Demographics Analysis
- Trend Identification
- Customizable Dashboard
- Won’t have (for the initial version):
- AI-powered predictive analytics
- Integration with non-social media platforms
By prioritizing tasks in this way, you can focus on delivering a functional minimum viable product (MVP) before moving on to more advanced features.
5. Developing a Plan of Action
With your problem broken down into manageable components and priorities set, it’s time to develop a concrete plan of action. This plan will guide your development process and help you stay on track as you work through the problem.
Elements of an Effective Action Plan
- Milestones: Set clear, achievable milestones that represent significant progress in your project.
- Tasks: Break down each milestone into specific tasks that need to be completed.
- Timeline: Estimate how long each task will take and create a realistic timeline for completion.
- Resources: Identify the resources (tools, libraries, APIs) you’ll need for each task.
- Dependencies: Note any dependencies between tasks to ensure proper sequencing.
- Testing and Validation: Include steps for testing and validating your work at each stage.
Example: Action Plan for the Social Media Analytics Tool
Here’s a simplified action plan for the initial development of our social media analytics tool:
- Milestone 1: Data Collection and Storage (2 weeks)
- Task 1.1: Set up development environment (1 day)
- Task 1.2: Implement Twitter API integration (3 days)
- Task 1.3: Design and implement database schema (2 days)
- Task 1.4: Develop data storage module (3 days)
- Task 1.5: Test and validate data collection and storage (2 days)
- Milestone 2: Basic Data Processing and Analysis (2 weeks)
- Task 2.1: Implement data cleaning and normalization (3 days)
- Task 2.2: Develop modules for calculating basic engagement metrics (4 days)
- Task 2.3: Create simple content performance analysis (3 days)
- Task 2.4: Test and validate processing and analysis modules (2 days)
- Milestone 3: Basic Visualization and User Interface (2 weeks)
- Task 3.1: Design and implement basic dashboard layout (3 days)
- Task 3.2: Develop reusable chart components (4 days)
- Task 3.3: Integrate data analysis results with visualization (3 days)
- Task 3.4: Implement basic user authentication (2 days)
- Task 3.5: Test and validate UI and visualizations (2 days)
- Milestone 4: Integration and Testing (1 week)
- Task 4.1: Integrate all components (2 days)
- Task 4.2: Perform end-to-end testing (2 days)
- Task 4.3: Bug fixing and optimization (3 days)
This action plan provides a clear roadmap for developing the initial version of the social media analytics tool, with specific tasks and timelines for each milestone.
6. Implementing Solutions Incrementally
With your plan in place, it’s time to start implementing solutions. The key to successfully tackling complex problems is to work incrementally, focusing on one component at a time and gradually building up to the complete solution.
Benefits of Incremental Implementation
- Allows for early detection and correction of issues
- Provides opportunities for regular testing and validation
- Enables faster feedback and iterative improvements
- Helps maintain motivation by showing consistent progress
Tips for Incremental Implementation
- Start with a Minimal Viable Product (MVP): Focus on implementing the core functionality first, then add features incrementally.
- Use Version Control: Utilize tools like Git to track changes and manage different versions of your code.
- Implement Continuous Integration/Continuous Deployment (CI/CD): Automate testing and deployment processes to catch issues early and streamline development.
- Practice Test-Driven Development (TDD): Write tests before implementing features to ensure code quality and functionality.
- Refactor Regularly: Continuously improve your code structure and efficiency as you add new features.
Example: Incremental Implementation of the Social Media Analytics Tool
Let’s look at how we might incrementally implement the data collection component of our social media analytics tool:
- Implement basic Twitter API connection and fetch a single tweet
import tweepy def fetch_single_tweet(tweet_id): auth = tweepy.OAuthHandler("consumer_key", "consumer_secret") auth.set_access_token("access_token", "access_token_secret") api = tweepy.API(auth) try: tweet = api.get_status(tweet_id) return tweet._json except tweepy.TweepError as e: print(f"Error: {e}") return None # Test the function tweet_data = fetch_single_tweet("1234567890") print(tweet_data)
- Expand to fetch multiple tweets and handle rate limiting
import tweepy import time def fetch_multiple_tweets(tweet_ids, max_retries=3): auth = tweepy.OAuthHandler("consumer_key", "consumer_secret") auth.set_access_token("access_token", "access_token_secret") api = tweepy.API(auth, wait_on_rate_limit=True, wait_on_rate_limit_notify=True) tweets = [] for tweet_id in tweet_ids: for attempt in range(max_retries): try: tweet = api.get_status(tweet_id) tweets.append(tweet._json) break except tweepy.RateLimitError: print("Rate limit reached. Waiting...") time.sleep(60) except tweepy.TweepError as e: print(f"Error fetching tweet {tweet_id}: {e}") break return tweets # Test the function tweet_ids = ["1234567890", "2345678901", "3456789012"] tweets_data = fetch_multiple_tweets(tweet_ids) print(f"Fetched {len(tweets_data)} tweets")
- Implement data storage in a database
import tweepy import time import psycopg2 def store_tweets(tweets): conn = psycopg2.connect("dbname=social_media_analytics user=postgres password=password") cur = conn.cursor() for tweet in tweets: cur.execute( "INSERT INTO tweets (id, text, user_id, created_at) VALUES (%s, %s, %s, %s)", (tweet['id'], tweet['text'], tweet['user']['id'], tweet['created_at']) ) conn.commit() cur.close() conn.close() def fetch_and_store_tweets(tweet_ids): tweets = fetch_multiple_tweets(tweet_ids) store_tweets(tweets) return len(tweets) # Test the function tweet_ids = ["1234567890", "2345678901", "3456789012"] stored_count = fetch_and_store_tweets(tweet_ids) print(f"Stored {stored_count} tweets in the database")
By implementing the solution incrementally, we can test and validate each step, ensuring that our data collection component is working correctly before moving on to more complex features.
7. Testing and Validation
As you implement your solution incrementally, it’s crucial to incorporate testing and validation throughout the process. This ensures that each component works as expected and helps identify and fix issues early in the development cycle.
Types of Testing
- Unit Testing: Test individual functions or methods in isolation
- Integration Testing: Test how different components work together
- Functional Testing: Test entire features or user scenarios
- Performance Testing: Evaluate the system’s performance under various conditions
- User Acceptance Testing (UAT): Verify that the solution meets user requirements
Testing Strategies
- Test-Driven Development (TDD): Write tests before implementing features
- Continuous Integration (CI): Automatically run tests whenever code is pushed to the repository
- Automated Testing: Use testing frameworks and tools to automate repetitive tests
- Manual Testing: Perform exploratory testing to catch edge cases and user experience issues
Example: Testing the Social Media Analytics Tool
Let’s look at some example tests for our social media analytics tool:
- Unit Test for Tweet Fetching
import unittest from unittest.mock import patch from your_module import fetch_single_tweet class TestTweetFetching(unittest.TestCase): @patch('tweepy.API.get_status') def test_fetch_single_tweet(self, mock_get_status): mock_tweet = type('obj', (object,), {'_json': {'id': '1234567890', 'text': 'Test tweet'}}) mock_get_status.return_value = mock_tweet result = fetch_single_tweet('1234567890') self.assertEqual(result, {'id': '1234567890', 'text': 'Test tweet'}) mock_get_status.assert_called_once_with('1234567890') if __name__ == '__main__': unittest.main()
- Integration Test for Data Storage
import unittest from unittest.mock import patch from your_module import fetch_and_store_tweets class TestDataStorage(unittest.TestCase): @patch('your_module.fetch_multiple_tweets') @patch('your_module.store_tweets') def test_fetch_and_store_tweets(self, mock_store_tweets, mock_fetch_multiple_tweets): mock_tweets = [{'id': '1', 'text': 'Tweet 1'}, {'id': '2', 'text': 'Tweet 2'}] mock_fetch_multiple_tweets.return_value = mock_tweets result = fetch_and_store_tweets(['1', '2']) self.assertEqual(result, 2) mock_fetch_multiple_tweets.assert_called_once_with(['1', '2']) mock_store_tweets.assert_called_once_with(mock_tweets) if __name__ == '__main__': unittest.main()
These tests help ensure that our tweet fetching and data storage functions are working correctly, both in isolation and when integrated together.
8. Iterative Refinement and Optimization
As you progress through the implementation of your solution, it’s important to continuously refine and optimize your code and processes. This iterative approach allows you to improve the efficiency, maintainability, and scalability of your solution over time.
Strategies for Refinement and Optimization
- Code Reviews: Regularly review code with peers to identify areas for improvement and share knowledge.
- Profiling and Performance Analysis: Use tools to identify bottlenecks and optimize performance-critical sections of your code.
- Refactoring: Continuously improve code structure and readability without changing its external behavior.
- Design Pattern Application: Apply appropriate design patterns to improve code organization and flexibility.
- Scalability Considerations: Anticipate future growth and design your system to handle increased load and complexity.
Example: Optimizing the Social Media Analytics Tool
Let’s look at some ways we might optimize our social media analytics tool:
- Implement caching to reduce API calls
import redis import json redis_client = redis.Redis(host='localhost', port=6379, db=0) def fetch_tweet_with_cache(tweet_id, cache_expiry=3600): cached_tweet = redis_client.get(f"tweet:{tweet_id}") if cached_tweet: return json.loads(cached_tweet) tweet = fetch_single_tweet(tweet_id) if tweet: redis_client.setex(f"tweet:{tweet_id}", cache_expiry, json.dumps(tweet)) return tweet
- Optimize database queries
from sqlalchemy import create_engine, text engine = create_engine("postgresql://user:password@localhost/social_media_analytics") def get_user_engagement_stats(user_id): with engine.connect() as conn: result = conn.execute(text(""" SELECT AVG(retweet_count) as avg_retweets, AVG(favorite_count) as avg_favorites, COUNT(*) as total_tweets FROM tweets WHERE user_id = :user_id AND created_at > NOW() - INTERVAL '30 days' """), {"user_id": user_id}) return result.fetchone()
- Implement asynchronous processing for large datasets
import asyncio import aiohttp async def fetch_tweet_async(session, tweet_id): url = f"https://api.twitter.com/1.1/statuses/show.json?id={tweet_id}" async with session.get(url) as response: return await response.json() async def fetch_multiple_tweets_async(tweet_ids): async with aiohttp.ClientSession() as session: tasks = [fetch_tweet_async(session, tweet_id) for tweet_id in tweet_ids] return await asyncio.gather(*tasks) # Usage tweet_ids = ["1234567890", "2345678901", "3456789012"] tweets = asyncio.run(fetch_multiple_tweets_async(tweet_ids))
These optimizations help improve the performance and scalability of our social media analytics tool, allowing it to handle larger datasets and more concurrent users efficiently.
9. Documentation and Knowledge Sharing
As you work through complex problems and develop solutions, it’s crucial to document your process, decisions, and code. Good documentation not only helps you maintain and update your solution in the future but also allows others to understand and build upon your work.
Types of Documentation
- Code Comments: Inline explanations of complex or non-obvious code sections
- Function and Class Documentation: Descriptions of purpose, parameters, and return values
- README Files: Overview of the project, setup instructions, and basic usage guide
- API Documentation: Detailed descriptions of available endpoints, request/response formats
- Architecture Documentation: High-level overview of system design and component interactions
- User Manuals: Guides for end-users on how to use the software
Best Practices for Documentation
- Keep documentation up-to-date as you make changes
- Use clear, concise language
- Include examples and use cases where appropriate
- Use diagrams and visual aids to explain complex concepts
- Follow consistent formatting and style guidelines
Example: Documenting the Social Media Analytics Tool
Here’s an example of how we might document a function in our social media analytics tool:
def calculate_engagement_rate(likes, comments, shares, impressions):
"""
Calculate the engagement rate for a social media post.
The engagement rate is calculated as the total number of engagements
(likes + comments + shares) divided by the number of impressions,
expressed as a percentage.
Args:
likes (int): Number of likes on the post
comments (int): Number of comments on the post
shares (int): Number of times the post was shared
impressions (int): Number of times the post was displayed to users
Returns:
float: The engagement rate as a percentage, rounded to two decimal places
Raises:
ValueError: If impressions is zero or any input is negative
Example:
>>> calculate_engagement_rate(100, 20, 5, 1000)
12.50
"""
if impressions == 0:
raise ValueError("Impressions cannot be zero")
if any(x < 0 for x in (likes, comments, shares, impressions)):
raise ValueError("All inputs must be non-negative")
total_engagements = likes + comments + shares
engagement_rate = (total_engagements / impressions) * 100
return round(engagement_rate, 2)
This documentation provides a clear explanation of what the function does, its parameters, return value, potential errors, and even includes an example of how to use it.
Conclusion
Breaking down complex problems is an essential skill for programmers, enabling you to tackle challenging projects with confidence and efficiency. By following the approach outlined in this guide – understanding the problem, decomposing it into smaller parts, identifying patterns, prioritizing tasks, developing a plan, implementing incrementally, testing thoroughly, refining continuously, and documenting clearly – you’ll be well-equipped to handle even the most complex programming challenges.
Remember that problem-solving is an iterative process, and it’s okay to revisit and adjust your approach as you gain new insights or encounter unexpected challenges. With practice and persistence, you’ll become more adept at breaking down complex problems and developing robust, efficient solutions.
As you apply these techniques to your own projects, you’ll not only improve your problem-solving skills but also become a more valuable asset to your team and organization. Embrace the complexity, enjoy the process of discovery and creation, and never stop learning and refining your approach to tackling complex problems in programming.