Understanding Computer Science Fundamentals Without a Degree
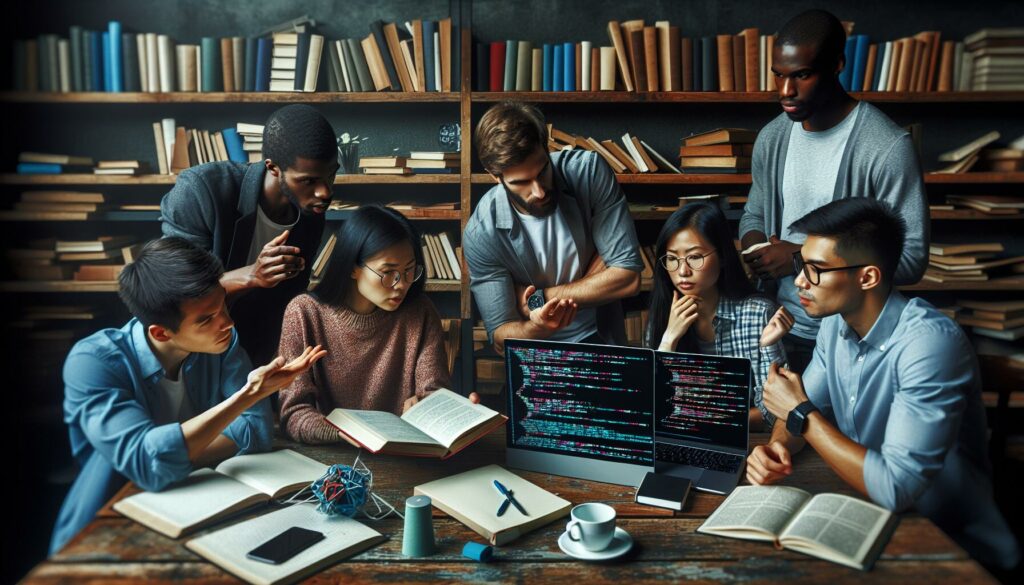
In today’s digital age, computer science has become an increasingly important field, driving innovation and shaping the way we live and work. While many pursue formal education in computer science through university degrees, it’s entirely possible to gain a solid understanding of computer science fundamentals without enrolling in a traditional program. This comprehensive guide will walk you through the essential concepts and provide resources for self-study, helping you build a strong foundation in computer science.
Table of Contents
- Introduction to Computer Science
- Programming Fundamentals
- Data Structures and Algorithms
- Computer Architecture and Organization
- Operating Systems
- Databases
- Computer Networking
- Software Engineering
- Artificial Intelligence and Machine Learning
- Cybersecurity
- Resources for Self-Study
- Conclusion
1. Introduction to Computer Science
Computer science is the study of computation, information processing, and the design of computer systems. It encompasses both theoretical and practical aspects of computing, from abstract mathematical concepts to hands-on programming and system design. To begin your journey in understanding computer science fundamentals, it’s essential to grasp the following core concepts:
- Binary and Boolean logic: Understanding how computers represent and process information at the most basic level.
- Computational thinking: Developing problem-solving skills by breaking down complex problems into smaller, manageable parts.
- Algorithms: Learning about step-by-step procedures for solving problems efficiently.
- Data representation: Exploring how different types of data are stored and manipulated in computer systems.
These foundational concepts will serve as building blocks for more advanced topics in computer science. As you progress, you’ll see how these ideas are applied across various domains within the field.
2. Programming Fundamentals
Programming is a core skill in computer science, allowing you to translate ideas and algorithms into executable code. While there are many programming languages to choose from, it’s crucial to start with the basics that are common across most languages:
- Variables and data types: Understanding how to store and manipulate different kinds of data.
- Control structures: Learning about conditional statements (if-else) and loops (for, while) to control program flow.
- Functions and modules: Organizing code into reusable blocks and understanding scope and modularity.
- Object-oriented programming (OOP): Grasping concepts like classes, objects, inheritance, and polymorphism.
- Error handling and debugging: Developing skills to identify and fix issues in your code.
To practice these concepts, choose a beginner-friendly language like Python or JavaScript. Here’s a simple example of a Python function that demonstrates some of these concepts:
def calculate_average(numbers):
if not numbers:
return 0
total = sum(numbers)
average = total / len(numbers)
return average
# Example usage
scores = [85, 90, 78, 92, 88]
avg_score = calculate_average(scores)
print(f"The average score is: {avg_score}")
As you become comfortable with these fundamentals, you can explore more advanced programming concepts and additional languages that align with your interests and goals.
3. Data Structures and Algorithms
Data structures and algorithms form the backbone of efficient problem-solving in computer science. Understanding these concepts is crucial for writing optimized code and solving complex computational problems. Key areas to focus on include:
- Basic data structures: Arrays, linked lists, stacks, queues, and hash tables.
- Advanced data structures: Trees, graphs, heaps, and tries.
- Algorithm design techniques: Divide and conquer, dynamic programming, greedy algorithms.
- Sorting and searching algorithms: Bubble sort, quicksort, merge sort, binary search.
- Big O notation: Analyzing and comparing algorithm efficiency.
Here’s an example of a simple binary search algorithm implemented in Python:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
# Example usage
sorted_numbers = [1, 3, 5, 7, 9, 11, 13, 15]
result = binary_search(sorted_numbers, 7)
print(f"Index of 7: {result}")
Practicing implementing various data structures and algorithms will help solidify your understanding and improve your problem-solving skills.
4. Computer Architecture and Organization
Understanding how computers work at a hardware level is essential for any aspiring computer scientist. This topic covers the design and organization of computer systems, including:
- Digital logic and circuits: Understanding the building blocks of computer hardware.
- CPU architecture: Exploring how processors execute instructions and manage data.
- Memory hierarchy: Learning about different types of memory (RAM, cache, storage) and their roles.
- Input/Output systems: Understanding how computers interact with external devices.
- Instruction set architecture: Studying the interface between hardware and software.
While this topic can be quite technical, there are many resources available for self-study, including online courses and simulators that can help you visualize and experiment with computer architecture concepts.
5. Operating Systems
Operating systems (OS) are the foundation of modern computing, managing hardware resources and providing services to applications. Key concepts to understand include:
- Process management: How the OS handles multiple running programs.
- Memory management: Techniques for allocating and managing system memory.
- File systems: How data is organized and stored on disk.
- I/O management: Handling input and output operations efficiently.
- Virtualization: Creating virtual instances of computer resources.
- Security and protection: Safeguarding system resources and user data.
To gain practical experience with operating systems, consider experimenting with different OS environments, such as Linux distributions, and learning basic command-line operations. Here’s an example of a simple bash script that demonstrates some OS interactions:
#!/bin/bash
# List files in the current directory
echo "Files in the current directory:"
ls -l
# Create a new directory
mkdir new_folder
echo "Created a new folder named 'new_folder'"
# Move into the new directory
cd new_folder
# Create a text file with some content
echo "Hello, World!" > greeting.txt
echo "Created a new file 'greeting.txt' with content"
# Display the content of the file
cat greeting.txt
# Move back to the parent directory
cd ..
# Remove the newly created directory and its contents
rm -r new_folder
echo "Removed 'new_folder' and its contents"
This script demonstrates basic file and directory operations, which are fundamental to understanding how operating systems manage resources.
6. Databases
Databases are crucial for storing, retrieving, and managing large amounts of data efficiently. Understanding database concepts is essential for developing robust applications. Key areas to focus on include:
- Relational database management systems (RDBMS): Understanding tables, relationships, and SQL.
- Database design: Learning about normalization, entity-relationship diagrams, and schema design.
- ACID properties: Ensuring data integrity through Atomicity, Consistency, Isolation, and Durability.
- Indexing and query optimization: Improving database performance.
- NoSQL databases: Exploring alternative database models for specific use cases.
To practice database concepts, you can set up a local database server like MySQL or PostgreSQL and experiment with creating tables, inserting data, and writing queries. Here’s an example of some basic SQL operations:
-- Create a table
CREATE TABLE users (
id INT PRIMARY KEY AUTO_INCREMENT,
username VARCHAR(50) NOT NULL,
email VARCHAR(100) NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
-- Insert data
INSERT INTO users (username, email) VALUES
('john_doe', 'john@example.com'),
('jane_smith', 'jane@example.com');
-- Query data
SELECT * FROM users WHERE username = 'john_doe';
-- Update data
UPDATE users SET email = 'john.doe@example.com' WHERE id = 1;
-- Delete data
DELETE FROM users WHERE id = 2;
As you become more comfortable with basic database operations, you can explore more advanced topics like database transactions, stored procedures, and database administration.
7. Computer Networking
In our interconnected world, understanding computer networking is crucial. This topic covers how computers communicate with each other and the protocols that enable the internet. Key concepts include:
- Network models: Understanding the OSI and TCP/IP models.
- IP addressing and subnetting: Learning how devices are identified and organized on networks.
- Protocols: Studying common protocols like TCP, UDP, HTTP, and DNS.
- Network security: Exploring firewalls, encryption, and secure communication.
- Wireless networking: Understanding Wi-Fi and mobile network technologies.
To gain practical experience, you can set up a home network, experiment with network configuration, and use tools like Wireshark to analyze network traffic. Here’s a simple Python script that demonstrates basic network communication using sockets:
import socket
def start_server():
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind(('localhost', 8000))
server_socket.listen(1)
print("Server listening on localhost:8000")
while True:
client_socket, address = server_socket.accept()
print(f"Connection from {address}")
message = client_socket.recv(1024).decode('utf-8')
print(f"Received: {message}")
client_socket.send("Message received".encode('utf-8'))
client_socket.close()
if __name__ == "__main__":
start_server()
This script creates a simple server that listens for incoming connections and responds to messages. You can create a corresponding client script to test the communication.
8. Software Engineering
Software engineering encompasses the principles and practices used to develop large-scale software systems. It goes beyond just writing code to include the entire software development lifecycle. Key areas to focus on include:
- Software development methodologies: Agile, Scrum, Waterfall, etc.
- Version control: Using tools like Git for managing code changes.
- Software design patterns: Understanding common solutions to recurring design problems.
- Testing and quality assurance: Learning about unit testing, integration testing, and test-driven development.
- Project management: Understanding how to plan, track, and manage software projects.
- DevOps practices: Exploring continuous integration and deployment.
To practice software engineering principles, you can contribute to open-source projects, work on personal projects using version control, and implement design patterns in your code. Here’s an example of a simple unit test in Python using the unittest framework:
import unittest
def add_numbers(a, b):
return a + b
class TestAddNumbers(unittest.TestCase):
def test_add_positive_numbers(self):
self.assertEqual(add_numbers(2, 3), 5)
def test_add_negative_numbers(self):
self.assertEqual(add_numbers(-1, -1), -2)
def test_add_mixed_numbers(self):
self.assertEqual(add_numbers(-1, 1), 0)
if __name__ == '__main__':
unittest.main()
This example demonstrates how to write basic unit tests for a simple function, which is an important practice in software engineering for ensuring code quality and reliability.
9. Artificial Intelligence and Machine Learning
Artificial Intelligence (AI) and Machine Learning (ML) are rapidly growing fields within computer science. While these topics can be quite advanced, understanding the basics is valuable for any aspiring computer scientist. Key concepts include:
- Machine learning algorithms: Supervised, unsupervised, and reinforcement learning.
- Neural networks and deep learning: Understanding the basics of artificial neural networks.
- Natural Language Processing (NLP): How computers process and understand human language.
- Computer vision: Techniques for image and video analysis.
- AI ethics: Considering the ethical implications of AI systems.
To get started with AI and ML, you can use libraries like scikit-learn or TensorFlow to implement basic machine learning models. Here’s a simple example using scikit-learn to train a linear regression model:
from sklearn.linear_model import LinearRegression
from sklearn.model_selection import train_test_split
import numpy as np
# Generate some sample data
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([2, 4, 5, 4, 5])
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create and train the model
model = LinearRegression()
model.fit(X_train, y_train)
# Make predictions
predictions = model.predict(X_test)
print("Predictions:", predictions)
print("Actual values:", y_test)
This example demonstrates how to train a simple linear regression model and make predictions, which is a fundamental concept in machine learning.
10. Cybersecurity
In an increasingly digital world, cybersecurity has become a critical aspect of computer science. Understanding the basics of cybersecurity is essential for developing secure systems and protecting against threats. Key areas to focus on include:
- Cryptography: Understanding encryption, hashing, and digital signatures.
- Network security: Learning about firewalls, intrusion detection systems, and VPNs.
- Web security: Understanding common vulnerabilities like SQL injection and cross-site scripting (XSS).
- Authentication and access control: Implementing secure user authentication and authorization.
- Security best practices: Learning about secure coding practices and security audits.
To gain practical experience in cybersecurity, you can set up a home lab, practice ethical hacking on platforms like HackTheBox, and implement security measures in your own projects. Here’s an example of how to hash a password using Python’s built-in hashlib library:
import hashlib
import os
def hash_password(password):
# Generate a random salt
salt = os.urandom(32)
# Create the hash
hash = hashlib.pbkdf2_hmac('sha256', password.encode('utf-8'), salt, 100000)
# Combine the salt and hash
return salt + hash
def verify_password(stored_password, provided_password):
salt = stored_password[:32] # The salt is stored at the beginning
stored_hash = stored_password[32:]
hash = hashlib.pbkdf2_hmac('sha256', provided_password.encode('utf-8'), salt, 100000)
return hash == stored_hash
# Example usage
password = "mysecretpassword"
hashed_password = hash_password(password)
print(f"Hashed password: {hashed_password.hex()}")
# Verify the password
is_correct = verify_password(hashed_password, password)
print(f"Password is correct: {is_correct}")
This example demonstrates how to securely hash and verify passwords, which is a fundamental concept in cybersecurity for protecting user credentials.
11. Resources for Self-Study
While learning computer science fundamentals without a degree requires self-discipline and dedication, there are numerous resources available to support your journey:
- Online courses: Platforms like Coursera, edX, and Udacity offer comprehensive computer science courses, often from top universities.
- Coding bootcamps: Intensive programs that focus on practical programming skills.
- Open source textbooks: Many computer science textbooks are available freely online.
- Coding practice platforms: Websites like LeetCode, HackerRank, and CodeWars offer coding challenges to improve your skills.
- YouTube tutorials: Many channels offer in-depth explanations of computer science concepts.
- GitHub repositories: Explore open-source projects to learn from real-world code.
- Tech blogs and podcasts: Stay updated with the latest trends and technologies in the field.
- Local meetups and conferences: Network with other enthusiasts and professionals in the field.
Remember to create a structured learning plan and set achievable goals. Consistency is key when self-studying a complex field like computer science.
12. Conclusion
Understanding computer science fundamentals without a degree is certainly challenging, but it’s an achievable goal with dedication and the right resources. By focusing on the core areas outlined in this guide – from programming and data structures to operating systems and cybersecurity – you can build a solid foundation in computer science.
Remember that computer science is a vast and ever-evolving field. While this guide covers the fundamentals, there’s always more to learn. Stay curious, keep practicing, and don’t be afraid to dive deep into areas that interest you most. Whether you’re aiming for a career in software development, pursuing a passion project, or simply expanding your knowledge, understanding these computer science fundamentals will serve you well in our increasingly digital world.
As you continue your learning journey, remember to apply your knowledge through practical projects, collaborate with others, and stay updated with the latest developments in the field. With persistence and hands-on experience, you can gain a comprehensive understanding of computer science that rivals that of many degree programs. Good luck on your learning adventure!