Choosing the Right Programming Language: Start with Simplicity
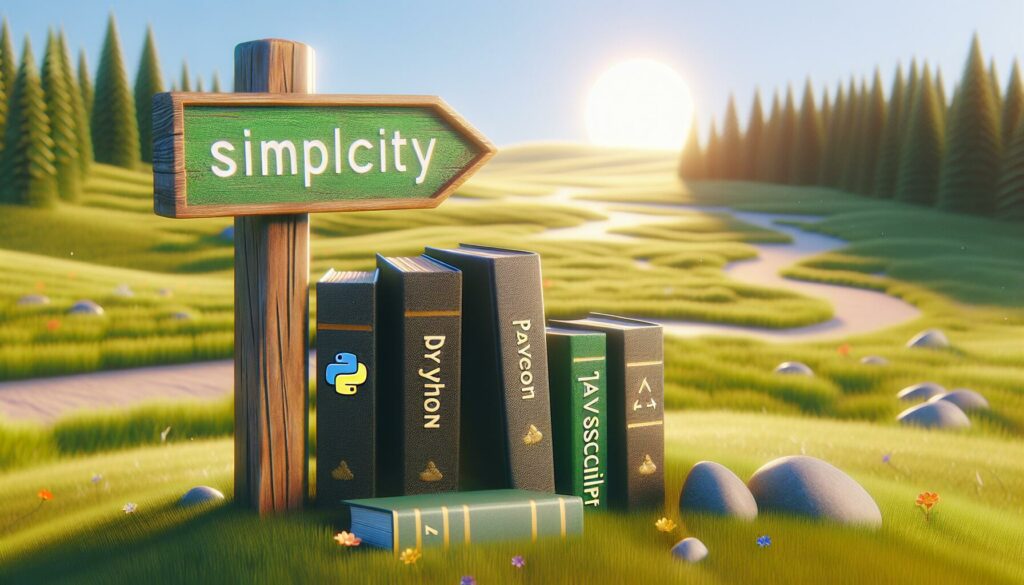
In the vast world of programming, one of the most crucial decisions a beginner can make is choosing the right programming language to start their journey. With countless options available, ranging from traditional languages like C++ and Java to modern alternatives like Python and JavaScript, the choice can be overwhelming. However, for those just starting out, the key to success often lies in embracing simplicity. This article will explore why starting with a simple programming language can set you up for long-term success and help you build a strong foundation in coding.
The Importance of Simplicity for Beginners
When you’re new to programming, the last thing you want is to be bogged down by complex syntax and intricate language features. Starting with a simple language allows you to focus on the fundamental concepts of programming without getting lost in the details. Here’s why simplicity matters:
- Faster learning curve: Simple languages are easier to grasp, allowing you to start writing functional code more quickly.
- Increased motivation: Quick wins and visible progress can boost your confidence and keep you motivated to continue learning.
- Focus on problem-solving: With less time spent on language intricacies, you can dedicate more energy to developing your problem-solving skills.
- Easier debugging: Simpler code is often easier to read and debug, making it less frustrating for beginners to find and fix errors.
Top Simple Programming Languages for Beginners
While there are many programming languages to choose from, some stand out as particularly beginner-friendly due to their simplicity and ease of use. Let’s explore some of the top choices:
1. Python
Python has gained immense popularity in recent years, and for good reason. It’s known for its clean, readable syntax and powerful libraries that make it suitable for a wide range of applications.
Key features:
- Easy-to-read syntax that closely resembles plain English
- Dynamic typing, which means you don’t need to declare variable types
- Extensive standard library and third-party packages
- Versatile applications, including web development, data science, and artificial intelligence
Example Python code:
# A simple Python program to calculate the sum of two numbers
num1 = 10
num2 = 20
sum = num1 + num2
print(f"The sum of {num1} and {num2} is: {sum}")
2. JavaScript
JavaScript is the language of the web and is essential for front-end web development. Its simplicity and ubiquity make it an excellent choice for beginners.
Key features:
- Runs in web browsers, making it easy to see results immediately
- Flexible and forgiving syntax
- Large community and extensive resources for learning
- Can be used for both front-end and back-end development (with Node.js)
Example JavaScript code:
// A simple JavaScript program to greet the user
let name = prompt("What's your name?");
alert(`Hello, ${name}! Welcome to JavaScript programming.`);
3. Ruby
Ruby is known for its elegant syntax and focus on programmer happiness. It’s an excellent language for beginners who want to learn object-oriented programming concepts.
Key features:
- Readable and expressive syntax
- Strong focus on object-oriented programming
- Large standard library and active community
- Popular for web development with the Ruby on Rails framework
Example Ruby code:
# A simple Ruby program to check if a number is even or odd
puts "Enter a number:"
number = gets.chomp.to_i
if number % 2 == 0
puts "#{number} is even."
else
puts "#{number} is odd."
end
The Benefits of Starting with a Simple Language
Choosing a simple programming language as your starting point offers numerous advantages that can significantly impact your learning journey and future career in programming:
1. Faster Concept Mastery
Simple languages allow you to grasp fundamental programming concepts more quickly. These concepts include:
- Variables and data types
- Control structures (if statements, loops)
- Functions and methods
- Basic data structures (arrays, lists, dictionaries)
By learning these concepts in a simpler context, you’ll be better prepared to understand them in more complex languages later on.
2. Increased Confidence
Starting with a simple language can boost your confidence as a programmer. When you can quickly write functional code and see immediate results, it provides a sense of accomplishment that can motivate you to tackle more challenging projects.
3. Improved Problem-Solving Skills
Simple languages allow you to focus more on problem-solving rather than getting bogged down in syntax details. This focus helps you develop critical thinking skills that are essential for programming in any language.
4. Easier Transition to Complex Languages
Once you’ve mastered the basics in a simple language, transitioning to more complex languages becomes easier. You’ll have a solid foundation of programming concepts, making it simpler to adapt to new syntax and features.
Common Misconceptions About Simple Languages
Despite the benefits of starting with a simple language, some misconceptions might discourage beginners from choosing this path. Let’s address some of these concerns:
Misconception 1: Simple Languages Are Less Powerful
While simple languages may have a more straightforward syntax, they are not necessarily less powerful. Many simple languages, like Python, are used in complex applications, including data science, machine learning, and web development.
Misconception 2: You’ll Have to Relearn Everything Later
The fundamental concepts you learn in a simple language are transferable to other languages. While syntax may differ, the underlying principles of programming remain the same across languages.
Misconception 3: Simple Languages Are Not Used in Real-World Applications
Many simple languages are widely used in industry. For example, Python is used by companies like Google, Netflix, and NASA, while JavaScript is essential for web development across countless websites.
How to Choose the Right Simple Language for You
While all simple languages offer benefits for beginners, the best choice for you depends on your goals and interests. Here are some factors to consider:
1. Your Learning Style
Consider how you learn best. Do you prefer visual learning, hands-on practice, or reading documentation? Different languages may have resources that better suit your learning style.
2. Career Goals
Think about what you want to achieve with programming. If you’re interested in web development, JavaScript might be the best choice. For data science or AI, Python could be more suitable.
3. Available Resources
Look at the learning resources available for each language. Consider factors like online courses, tutorials, books, and community support.
4. Project Ideas
Consider the types of projects you’d like to work on. Some languages may be better suited for certain types of applications.
Getting Started with Your Chosen Language
Once you’ve chosen a simple language to start with, here are some steps to begin your learning journey:
1. Set Up Your Development Environment
Install the necessary tools and software for your chosen language. This typically includes:
- A text editor or Integrated Development Environment (IDE)
- The language interpreter or compiler
- Any required libraries or frameworks
2. Start with the Basics
Begin by learning the fundamental concepts of your chosen language. This usually includes:
- Syntax and basic structure
- Variables and data types
- Control structures (if statements, loops)
- Functions and methods
3. Practice Regularly
Consistent practice is key to learning programming. Set aside time each day to write code, even if it’s just for a short period.
4. Work on Small Projects
Start with small, achievable projects to apply what you’ve learned. This could include:
- A simple calculator
- A to-do list application
- A basic game like rock-paper-scissors
5. Join a Community
Engage with other learners and experienced programmers. This can provide support, motivation, and opportunities to learn from others. Look for:
- Online forums and discussion boards
- Local meetup groups
- Coding bootcamps or workshops
Transitioning to More Complex Languages
As you become more comfortable with your first programming language, you may want to explore more complex languages. Here’s how to make that transition smoothly:
1. Identify Your Next Language
Choose a language that aligns with your growing skills and career goals. Some popular choices for a second language include:
- Java for Android development or enterprise applications
- C++ for game development or system programming
- Swift for iOS app development
2. Compare and Contrast
When learning your new language, compare its features and syntax to what you already know. This will help you understand the differences and similarities between languages.
3. Focus on Language-Specific Features
Pay attention to features that are unique to your new language, such as:
- Static typing in languages like Java or C++
- Memory management in C or C++
- Functional programming concepts in languages like Haskell or Scala
4. Apply Your Problem-Solving Skills
Remember that the problem-solving skills you developed with your first language are transferable. Focus on applying these skills to solve problems in your new language.
Conclusion: Embrace Simplicity, Build a Strong Foundation
Choosing the right programming language as a beginner is a crucial step in your coding journey. By starting with a simple language, you set yourself up for success by focusing on fundamental concepts, building confidence, and developing strong problem-solving skills.
Remember that simplicity doesn’t mean lack of power or real-world applicability. Many simple languages are used in complex, professional settings. The key is to choose a language that aligns with your goals and learning style, and to practice consistently.
As you grow in your programming skills, you’ll find that the concepts you learned in your first simple language will serve as a strong foundation for learning more complex languages and tackling more challenging projects. Embrace the simplicity, focus on the fundamentals, and watch your programming skills flourish.
Happy coding!