The Importance of Logic in Learning to Code
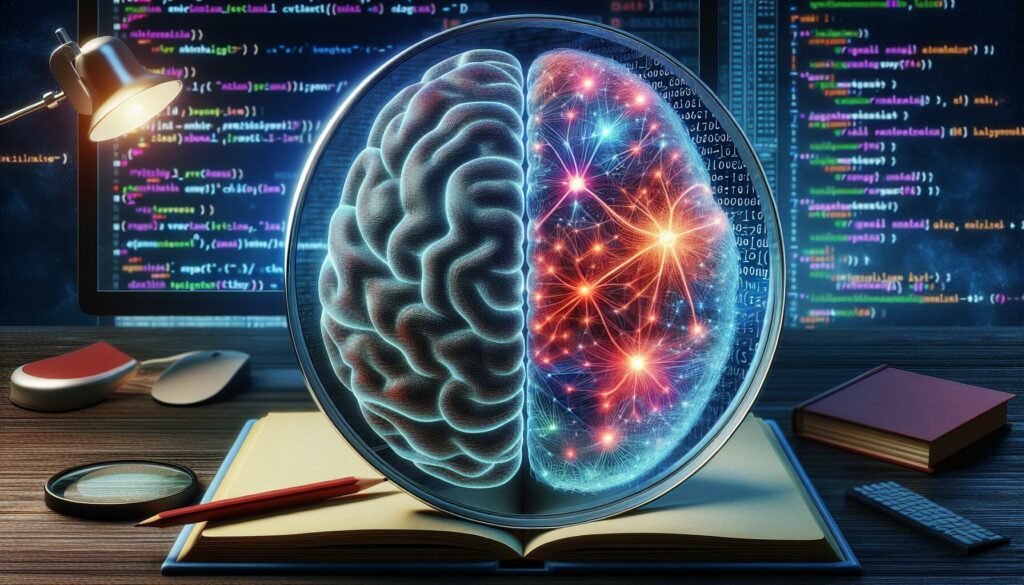
Learning to code is an exciting journey that opens up a world of possibilities in our increasingly digital age. Whether you’re aspiring to become a professional software developer or simply want to enhance your problem-solving skills, understanding the fundamental role of logic in programming is crucial. In this comprehensive guide, we’ll explore why logic is the backbone of coding and how it can significantly impact your learning process and future success as a programmer.
What is Logic in Programming?
Before diving into its importance, let’s first define what we mean by logic in the context of programming. In essence, logic in coding refers to the systematic and rational way of thinking that allows programmers to create efficient, effective, and error-free code. It involves:
- Reasoning about problems and their solutions
- Breaking down complex tasks into smaller, manageable steps
- Creating algorithms and flowcharts
- Making decisions based on given conditions
- Organizing and structuring code in a coherent manner
Logic is the foundation upon which all programming languages are built. Whether you’re working with Python, Java, C++, or any other language, the underlying logical principles remain consistent.
Why is Logic Crucial in Coding?
1. Problem-Solving Skills
At its core, programming is about solving problems. Logic equips you with the mental tools to approach complex issues systematically. By honing your logical thinking skills, you’ll be better prepared to:
- Analyze problems and identify their root causes
- Develop step-by-step solutions
- Anticipate potential issues and edge cases
- Debug and troubleshoot effectively
These problem-solving abilities are not only valuable in coding but also transferable to many other aspects of life and work.
2. Efficient Code Writing
Logic helps you write cleaner, more efficient code. When you understand the logical flow of a program, you can:
- Minimize redundancy and repetition
- Optimize algorithms for better performance
- Reduce the likelihood of bugs and errors
- Create more maintainable and scalable code
This efficiency is crucial, especially when working on large-scale projects or in professional environments where code quality directly impacts the success of a product or service.
3. Understanding Programming Concepts
Many programming concepts are rooted in logic. A strong foundation in logical thinking makes it easier to grasp and apply these concepts, such as:
- Control structures (if-else statements, loops)
- Boolean algebra and logical operators
- Data structures and algorithms
- Object-oriented programming principles
By understanding the logical basis of these concepts, you’ll find it easier to learn new programming languages and paradigms throughout your coding journey.
4. Debugging and Troubleshooting
When your code doesn’t work as expected, logical thinking is your best tool for identifying and fixing the issue. Strong logical skills enable you to:
- Trace the flow of your program step by step
- Identify inconsistencies or errors in your logic
- Form and test hypotheses about what might be causing the problem
- Implement and verify solutions systematically
Effective debugging is a critical skill for any programmer, and it relies heavily on logical reasoning.
5. Adaptability to New Technologies
The tech world is constantly evolving, with new programming languages, frameworks, and tools emerging regularly. A strong foundation in logic makes it easier to adapt to these changes because:
- The underlying logical principles remain consistent across different technologies
- You can quickly understand the rationale behind new concepts and features
- You’re better equipped to evaluate and choose the right tools for specific problems
This adaptability is crucial for long-term success in the ever-changing field of software development.
How to Develop Logical Thinking for Coding
Now that we understand the importance of logic in coding, let’s explore some strategies to develop and strengthen your logical thinking skills:
1. Practice Problem-Solving
Engage in activities that challenge your problem-solving abilities:
- Solve puzzles and brain teasers
- Play strategy games like chess or Sudoku
- Participate in coding challenges on platforms like LeetCode or HackerRank
- Try to solve real-world problems using logical reasoning
The more you practice, the more natural logical thinking will become.
2. Learn Boolean Algebra
Boolean algebra is the mathematical foundation of computer logic. Familiarize yourself with concepts such as:
- AND, OR, NOT operations
- Truth tables
- De Morgan’s laws
- Logical equivalences
Understanding these principles will greatly enhance your ability to work with conditional statements and complex logical expressions in your code.
3. Study Algorithms and Data Structures
Algorithms and data structures are practical applications of logic in programming. By studying them, you’ll improve your ability to:
- Break down problems into logical steps
- Choose the most efficient solutions for specific scenarios
- Understand the trade-offs between different approaches
Start with basic algorithms like sorting and searching, then progress to more complex ones as you build your skills.
4. Practice Pseudocode and Flowcharts
Before writing actual code, get into the habit of planning your solution using pseudocode or flowcharts. This practice helps you:
- Organize your thoughts logically
- Visualize the flow of your program
- Identify potential issues before you start coding
- Communicate your ideas more effectively to others
These planning techniques are valuable skills in both learning and professional environments.
5. Analyze and Refactor Existing Code
Reading and understanding code written by others is an excellent way to improve your logical thinking. Try to:
- Understand the logic behind existing solutions
- Identify areas where the code could be improved or optimized
- Rewrite or refactor code to make it more efficient or readable
- Experiment with different approaches to solve the same problem
This practice will expose you to various logical approaches and help you develop a critical eye for code quality.
6. Teach Others
One of the best ways to solidify your understanding of logical concepts is to explain them to others. Consider:
- Participating in coding forums or discussion groups
- Writing blog posts or creating tutorials about programming concepts
- Mentoring beginners or helping classmates with their code
Teaching forces you to break down complex ideas into simpler, logical components, which in turn deepens your own understanding.
Applying Logic in Different Programming Paradigms
As you progress in your coding journey, you’ll encounter different programming paradigms, each with its own logical approach. Let’s briefly explore how logic applies in some common paradigms:
Procedural Programming
In procedural programming, logic is applied through a sequence of steps to solve a problem. Key logical concepts include:
- Sequential execution of instructions
- Use of variables and data types
- Control structures (if-else, loops)
- Functions and modular programming
Example in Python:
def calculate_area(length, width):
return length * width
def main():
length = 5
width = 3
area = calculate_area(length, width)
print(f"The area is: {area}")
main()
Object-Oriented Programming (OOP)
OOP applies logic through the creation and interaction of objects. Important logical concepts include:
- Encapsulation of data and behavior
- Inheritance and polymorphism
- Abstraction of complex systems
- Logical relationships between classes
Example in Java:
public class Rectangle {
private double length;
private double width;
public Rectangle(double length, double width) {
this.length = length;
this.width = width;
}
public double calculateArea() {
return length * width;
}
public static void main(String[] args) {
Rectangle rect = new Rectangle(5, 3);
System.out.println("The area is: " + rect.calculateArea());
}
}
Functional Programming
Functional programming emphasizes the use of pure functions and immutable data. Logical concepts in this paradigm include:
- Function composition
- Higher-order functions
- Recursion
- Immutability and side-effect free operations
Example in JavaScript:
const calculateArea = (length, width) => length * width;
const printArea = (length, width) => {
const area = calculateArea(length, width);
console.log(`The area is: ${area}`);
};
printArea(5, 3);
Common Logical Pitfalls in Coding
Even with a strong foundation in logic, it’s easy to fall into certain traps when coding. Being aware of these common pitfalls can help you avoid them:
1. Off-by-One Errors
These occur when you miscalculate the boundaries of loops or arrays. For example:
// Incorrect: Will cause an IndexOutOfBoundsException
for (int i = 1; i <= array.length; i++) {
System.out.println(array[i]);
}
// Correct
for (int i = 0; i < array.length; i++) {
System.out.println(array[i]);
}
2. Infinite Loops
These happen when the termination condition for a loop is never met. For instance:
// Infinite loop
while (true) {
// Code that never breaks the loop
}
3. Logical Short-Circuiting
This occurs when using logical AND (&&) and OR (||) operators. The second condition may not be evaluated if the first condition determines the result:
if (x != 0 && 10 / x > 1) {
// This avoids division by zero
}
4. Confusing Assignment (=) with Equality (==)
In many languages, using a single equals sign in a condition performs assignment instead of comparison:
// Incorrect: Always true, assigns 5 to x
if (x = 5) {
// This code will always execute
}
// Correct: Compares x to 5
if (x == 5) {
// This code executes only if x equals 5
}
5. Neglecting Edge Cases
Failing to consider all possible inputs or scenarios can lead to unexpected behavior:
function divide(a, b) {
// Neglects the case where b could be zero
return a / b;
}
// Improved version
function safeDivide(a, b) {
if (b === 0) {
throw new Error("Cannot divide by zero");
}
return a / b;
}
The Role of Logic in Software Design
Beyond writing individual lines of code, logic plays a crucial role in the broader aspects of software design and architecture. Understanding and applying logical principles at this higher level can lead to more robust, scalable, and maintainable software systems.
1. System Architecture
When designing the overall structure of a software system, logical thinking helps in:
- Identifying and separating concerns
- Designing modular and loosely coupled components
- Planning for scalability and future expansion
- Choosing appropriate design patterns and architectural styles
For example, the logical separation of presentation, business logic, and data access layers in a three-tier architecture is based on sound logical principles of organization and responsibility distribution.
2. Data Modeling
Creating effective data models requires strong logical skills to:
- Identify entities and their relationships
- Normalize data to reduce redundancy
- Design efficient database schemas
- Balance between data integrity and performance
The process of database normalization, for instance, is a systematic application of logical rules to organize data effectively.
3. API Design
Designing clear, intuitive, and efficient APIs relies heavily on logical thinking:
- Creating logical groupings of related functionality
- Defining clear and consistent naming conventions
- Planning for versioning and backwards compatibility
- Anticipating various use cases and edge cases
A well-designed API reflects a logical understanding of the problem domain and the needs of the API consumers.
4. Testing Strategies
Effective software testing is grounded in logical thinking:
- Identifying critical paths and edge cases to test
- Designing test cases that provide good coverage
- Creating logical hierarchies of unit, integration, and system tests
- Applying test-driven development (TDD) principles
The ability to anticipate potential issues and systematically verify software behavior is a direct application of logical reasoning.
Conclusion
The importance of logic in learning to code cannot be overstated. It forms the foundation upon which all programming skills are built, from writing individual lines of code to designing complex software systems. By developing strong logical thinking skills, you’ll not only become a more effective programmer but also enhance your problem-solving abilities in all areas of life.
Remember that improving your logical thinking is an ongoing process. Embrace challenges, practice regularly, and don’t be afraid to tackle complex problems. With time and persistence, you’ll find that logical thinking becomes second nature, allowing you to approach coding tasks with confidence and creativity.
As you continue your coding journey, keep in mind that logic is not just about following rules rigidly. It’s about developing a flexible, analytical mindset that can adapt to new situations and find innovative solutions. The logical skills you develop in programming will serve you well in many aspects of your personal and professional life, making the investment in this fundamental skill set truly invaluable.
So, as you dive into your next coding project or tackle a challenging algorithm, take a moment to appreciate the logical principles at work. Embrace the process of logical thinking, and watch as it transforms not just your code, but your entire approach to problem-solving. Happy coding!