The Socratic Method in Coding Education: Unlocking Deeper Understanding Through Questioning
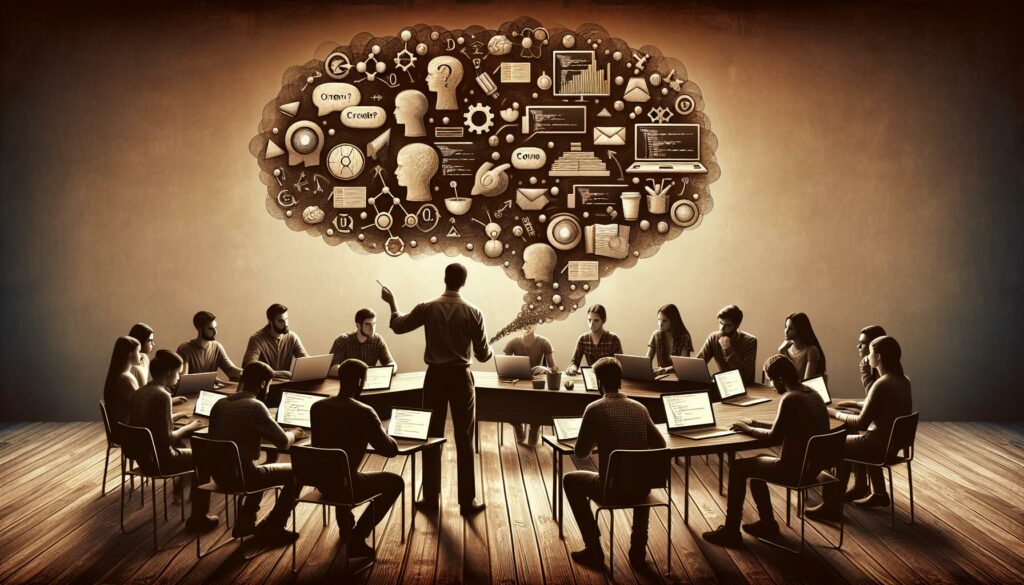
In the ever-evolving world of technology and software development, the ability to think critically and solve complex problems is paramount. As coding education continues to grow in importance, educators and mentors are constantly seeking effective methods to impart not just knowledge, but also the skills of analytical thinking and problem-solving. One such method that has stood the test of time and proven particularly effective in coding education is the Socratic method.
What is the Socratic Method?
Named after the classical Greek philosopher Socrates, the Socratic method is a form of cooperative argumentative dialogue between individuals, based on asking and answering questions to stimulate critical thinking and to draw out ideas and underlying presuppositions. In essence, it’s a way of teaching that involves asking questions to encourage students to think deeply about a topic and arrive at their own conclusions.
In the context of coding education, the Socratic method can be an incredibly powerful tool. It shifts the focus from passive learning to active engagement, encouraging students to think critically about the problems they’re trying to solve and the code they’re writing.
The Power of Asking the Right Questions
At the heart of the Socratic method is the art of asking the right questions. In coding education, these questions can serve multiple purposes:
- They can help students identify gaps in their understanding
- They can guide students towards discovering solutions on their own
- They can encourage students to think about the broader implications of their code
- They can help students develop a deeper understanding of programming concepts
Let’s explore each of these in more detail.
1. Identifying Gaps in Understanding
Often, students may think they understand a concept until they’re asked to explain it or apply it in a different context. By asking probing questions, educators can help students identify areas where their understanding may be incomplete or incorrect.
For example, consider a student who has just learned about for loops in Python. The educator might ask:
- “Can you explain why we use a for loop instead of a while loop in this situation?”
- “What would happen if we changed the range in this for loop?”
- “How would you modify this loop to skip every other number?”
These questions force the student to think more deeply about the concept of loops and how they work, potentially revealing any misunderstandings or gaps in knowledge.
2. Guiding Students Towards Solutions
When a student is stuck on a problem, it can be tempting for an educator to simply provide the solution. However, using the Socratic method, the educator can ask a series of questions that guide the student towards discovering the solution on their own.
For instance, if a student is struggling with a sorting algorithm, the educator might ask:
- “What is the first step you need to take when sorting this list?”
- “How do you decide which elements to compare?”
- “What do you do after you’ve made a comparison?”
- “How do you know when the list is fully sorted?”
By answering these questions, the student is likely to work through the problem step-by-step, arriving at a solution through their own reasoning.
3. Encouraging Broader Thinking
Coding isn’t just about writing syntax-correct statements; it’s about solving problems efficiently and creating maintainable, scalable solutions. The Socratic method can encourage students to think beyond the immediate problem and consider broader implications.
Questions in this vein might include:
- “How would this solution perform with a much larger dataset?”
- “What potential edge cases should we consider?”
- “How might we make this code more reusable?”
- “What are the security implications of this approach?”
These questions push students to think like professional developers, considering factors beyond just getting the code to run.
4. Developing Deeper Understanding
Perhaps the most significant benefit of the Socratic method in coding education is its ability to foster a deeper understanding of programming concepts. By encouraging students to explain their thinking and defend their choices, it helps solidify their understanding and uncover any misconceptions.
For example, when teaching object-oriented programming, an educator might ask:
- “Why did you choose to make this a class method instead of an instance method?”
- “How does encapsulation benefit us in this scenario?”
- “Can you explain the relationship between this parent class and its subclasses?”
These questions require students to articulate their understanding of complex concepts, reinforcing their learning and often leading to “aha!” moments of deeper comprehension.
Implementing the Socratic Method in Coding Education
While the Socratic method can be a powerful teaching tool, it requires skill and practice to implement effectively. Here are some strategies for using the Socratic method in coding education:
1. Start with Open-Ended Questions
Begin discussions or problem-solving sessions with open-ended questions that don’t have a single correct answer. This encourages students to think broadly and creatively. For example:
- “How might we approach this problem?”
- “What are some potential solutions we could consider?”
- “What do you think are the key challenges in this task?”
2. Use Follow-Up Questions
When a student provides an answer, don’t stop there. Ask follow-up questions to encourage deeper thinking:
- “Can you elaborate on that?”
- “What led you to that conclusion?”
- “How does that relate to what we learned earlier?”
3. Encourage Peer Discussion
The Socratic method doesn’t have to be limited to teacher-student interactions. Encourage students to ask questions of each other and discuss their ideas. This can be particularly effective in pair programming scenarios.
4. Embrace Mistakes
When a student makes a mistake or gives an incorrect answer, use it as a learning opportunity. Ask questions that help the student identify and correct their own errors:
- “What would happen if we ran this code?”
- “Can you walk me through your logic step by step?”
- “How could we test if this solution works in all cases?”
5. Be Patient
The Socratic method often takes more time than simply providing answers. Be patient and allow students time to think and formulate their responses. The deeper understanding they gain is worth the extra time invested.
Challenges and Considerations
While the Socratic method can be highly effective, it’s not without its challenges. Here are some things to keep in mind:
1. It Can Be Time-Consuming
Guiding students to discover answers on their own often takes longer than simply providing the information. In a curriculum with tight time constraints, this can be challenging.
2. It Requires Skill to Implement Effectively
Knowing how to ask the right questions at the right time is a skill that takes practice to develop. Educators need training and experience to use this method effectively.
3. It Can Be Frustrating for Some Students
Some students may find it frustrating not to receive direct answers to their questions. It’s important to balance the Socratic method with other teaching approaches and to explain its benefits to students.
4. It May Not Be Suitable for All Topics
While the Socratic method is excellent for developing problem-solving skills and deep understanding, it may be less effective for teaching straightforward factual information or syntax.
Real-World Application: A Case Study
Let’s look at how the Socratic method might be applied in a real coding education scenario. Imagine a student is learning about recursion and is trying to implement a function to calculate the nth Fibonacci number.
The student presents this initial solution:
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
Instead of immediately pointing out the inefficiencies in this solution, an educator using the Socratic method might ask:
- “Can you walk me through how this function works for n = 5?”
- “What happens when we call fibonacci(5)?”
- “How many times is fibonacci(2) calculated in this process?”
- “Do you see any potential issues with this approach for large values of n?”
- “Can you think of a way to avoid recalculating the same values multiple times?”
Through this line of questioning, the student is likely to realize on their own that the function is inefficient due to repeated calculations. This realization is much more powerful than simply being told that the solution is inefficient.
The educator might then guide the student towards optimizing the solution:
- “How could we store the results of our calculations to avoid repeating them?”
- “What data structure might be useful for this?”
- “How would you modify your function to use this approach?”
This process not only helps the student arrive at a more efficient solution but also deepens their understanding of recursion, memoization, and algorithm optimization.
The Long-Term Benefits of the Socratic Method in Coding Education
While the immediate goal of using the Socratic method in coding education is to help students understand specific concepts and solve particular problems, its benefits extend far beyond this.
1. Developing Critical Thinking Skills
By constantly engaging with probing questions, students learn to think critically about their code and their problem-solving approaches. This skill is invaluable in the ever-changing landscape of technology, where new languages, frameworks, and paradigms are constantly emerging.
2. Fostering Independence
The Socratic method teaches students how to ask themselves the right questions. Over time, they internalize this process, becoming more independent problem-solvers who can tackle new challenges without constant guidance.
3. Enhancing Communication Skills
Articulating thoughts and explaining reasoning are crucial skills for any developer. The Socratic method provides ample practice in clearly communicating complex ideas.
4. Encouraging Lifelong Learning
By emphasizing the process of questioning and discovery, the Socratic method instills a love for learning. This is crucial in the field of software development, where continuous learning is necessary to stay relevant.
5. Preparing for Real-World Scenarios
In professional settings, developers often need to justify their decisions, consider various approaches, and think through the implications of their code. The Socratic method prepares students for these scenarios.
Integrating the Socratic Method with Modern Coding Education Techniques
While the Socratic method is an ancient teaching technique, it can be effectively integrated with modern coding education approaches:
1. Project-Based Learning
In project-based learning scenarios, the Socratic method can be used to guide students through the planning and implementation stages, encouraging them to think deeply about their design choices and implementation strategies.
2. Pair Programming
The Socratic method naturally complements pair programming. The “navigator” can use Socratic questioning to guide the “driver” through the coding process.
3. Code Reviews
During code reviews, reviewers can use Socratic questioning to help the code author think critically about their implementation and consider alternative approaches.
4. Online Learning Platforms
Even in online or self-paced learning environments, the principles of the Socratic method can be incorporated through carefully crafted prompts and reflection questions.
Conclusion: The Socratic Method as a Cornerstone of Effective Coding Education
The Socratic method, with its emphasis on questioning and critical thinking, is a powerful tool in coding education. By asking the right questions, educators can guide students towards deeper understanding, more effective problem-solving, and a more thorough grasp of programming concepts.
While it requires skill and patience to implement effectively, the long-term benefits of the Socratic method are substantial. It doesn’t just teach students to code; it teaches them to think like programmers, to approach problems analytically, and to continually question and improve their own understanding and solutions.
As we continue to refine and improve coding education techniques, the Socratic method stands out as a timeless approach that aligns perfectly with the critical thinking and problem-solving skills required in the ever-evolving world of software development. By fostering these skills, we prepare students not just for their first programming job, but for a lifetime of learning and growth in the field of technology.