Algorithmic Thinking in Daily Life: How Everyday Decisions Mirror Coding Logic
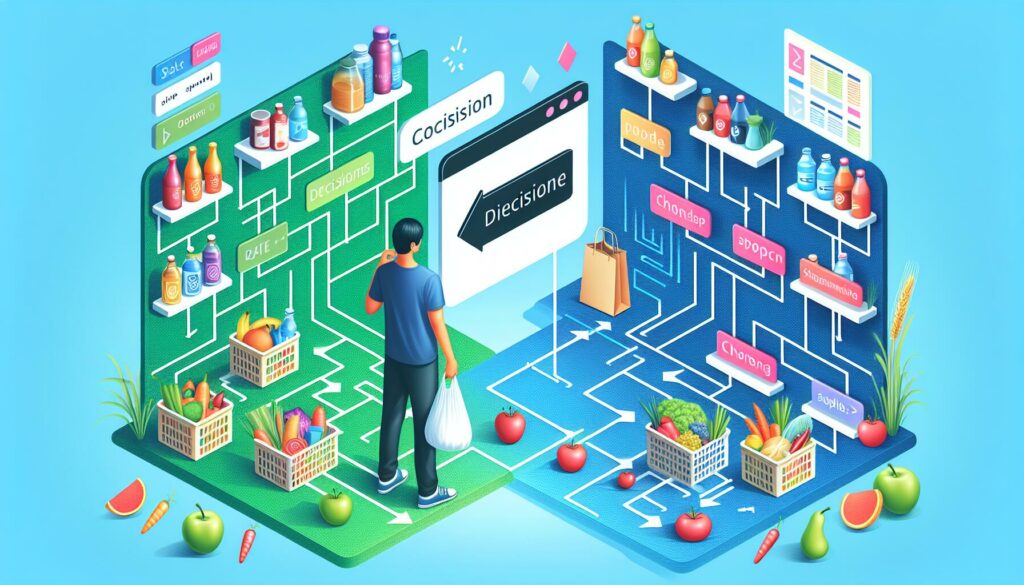
In our increasingly digital world, the principles of computer programming have seeped into various aspects of our lives, often without us even realizing it. Algorithmic thinking, a cornerstone of coding logic, is not just confined to the realm of software development. It’s a powerful problem-solving approach that can be applied to everyday situations, helping us make more efficient and effective decisions. This article explores how the logic we use in our daily lives often mirrors the principles used in coding, and how we can harness this understanding to improve our decision-making processes.
Understanding Algorithmic Thinking
Before we dive into the parallels between coding logic and everyday decision-making, let’s first understand what algorithmic thinking entails.
Algorithmic thinking is a way of approaching problems systematically, breaking them down into smaller, manageable steps to arrive at a solution. It involves:
- Defining the problem clearly
- Breaking the problem into smaller sub-problems
- Identifying the steps needed to solve each sub-problem
- Organizing these steps in a logical sequence
- Executing the steps and evaluating the outcome
This approach is fundamental in computer programming, where complex tasks are broken down into a series of instructions that a computer can understand and execute. However, this same logic can be incredibly useful in our day-to-day lives.
Decision Trees in Daily Life
One of the most common programming concepts that we unconsciously use in our daily lives is the decision tree. In programming, a decision tree is a flowchart-like structure where each internal node represents a test on an attribute, each branch represents the outcome of the test, and each leaf node represents a decision.
Consider a mundane task like deciding what to wear in the morning. Your mental process might look something like this:
if (weather is cold) {
if (it's raining) {
wear warm waterproof jacket
} else {
wear warm coat
}
} else if (weather is warm) {
if (it's sunny) {
wear light shirt
} else {
wear light jacket
}
} else {
wear regular clothes
}
This decision-making process mirrors the if-else statements commonly used in programming. By consciously recognizing this structure in our thinking, we can make our decision-making more efficient and systematic.
Loops in Routine Tasks
Loops are another fundamental concept in programming that we often use in our daily routines without realizing it. In coding, loops are used to repeat a set of instructions until a certain condition is met. This concept is mirrored in many of our daily activities.
For example, consider the process of doing laundry:
while (dirty clothes basket is not empty) {
load washing machine
add detergent
set appropriate cycle
start machine
wait for cycle to complete
transfer clothes to dryer
start dryer
wait for cycle to complete
fold and put away clothes
}
This process continues until all the dirty clothes are washed, dried, and put away. By thinking of routine tasks in terms of loops, we can often find ways to optimize our processes and make them more efficient.
Functions and Modular Thinking
In programming, functions are used to group a set of related instructions that perform a specific task. This concept of modular thinking can be incredibly useful in organizing our daily lives and tackling complex tasks.
Let’s take the example of preparing for a dinner party:
function planMenu() {
// Plan appetizers, main course, dessert
}
function shopForIngredients() {
// Make list and purchase items
}
function prepareFood() {
// Cook dishes according to recipes
}
function setTable() {
// Arrange plates, cutlery, decorations
}
function hostDinnerParty() {
planMenu()
shopForIngredients()
prepareFood()
setTable()
// Welcome guests and enjoy the evening
}
By breaking down the complex task of hosting a dinner party into smaller, manageable functions, we can approach the task more systematically and ensure we don’t overlook any important steps.
Variables and State Management
In programming, variables are used to store and manage data. Similarly, in our daily lives, we constantly keep track of various “variables” that affect our decisions and actions.
For instance, managing our personal finances involves keeping track of several variables:
let bankBalance = 5000
let monthlyIncome = 3000
let monthlyExpenses = 2500
function updateFinances() {
bankBalance = bankBalance + monthlyIncome - monthlyExpenses
if (bankBalance < 1000) {
alert("Low balance! Time to cut expenses.")
}
}
By thinking of our finances in terms of variables and regular updates (like a program that runs monthly), we can better manage our money and make informed financial decisions.
Debugging in Real Life
Debugging is an essential part of programming, where developers identify and fix errors in their code. This concept is equally applicable to problem-solving in daily life.
When something goes wrong in our day-to-day activities, we often unknowingly follow a debugging process:
- Identify the problem (What’s not working as expected?)
- Gather information (What were the circumstances when the problem occurred?)
- Form a hypothesis (What might be causing the issue?)
- Test the hypothesis (Try a potential solution)
- Analyze the results (Did it solve the problem?)
- If not solved, repeat steps 3-5
This systematic approach to problem-solving can be applied to a wide range of issues, from figuring out why your car won’t start to resolving conflicts in personal relationships.
Optimization in Daily Routines
In programming, optimization involves improving the efficiency of code to make it run faster or use fewer resources. This concept can be applied to our daily routines to save time and energy.
For example, optimizing your morning routine:
function morningRoutine() {
wakeUp()
while (coffee is brewing) {
takeShower()
}
drinkCoffee()
eatBreakfast()
getDressed()
}
By paralleling tasks (like showering while the coffee brews), we can optimize our routine to save time. This kind of thinking can be applied to various aspects of our lives to increase efficiency.
Error Handling in Decision Making
In programming, error handling is crucial for creating robust software that can gracefully manage unexpected situations. This concept is equally important in our daily decision-making processes.
Consider planning an outdoor event:
function planOutdoorEvent() {
checkWeatherForecast()
if (forecast === "rain") {
try {
bookIndoorVenue()
} catch (error) {
if (error === "no venues available") {
rescheduleEvent()
}
}
} else {
proceedWithOutdoorPlans()
}
}
By anticipating potential problems and having backup plans, we can handle unexpected situations more effectively in our daily lives.
Recursion in Problem Solving
Recursion is a programming concept where a function calls itself to solve a problem. While not as common in everyday thinking, understanding recursion can be useful for tackling complex, nested problems.
For instance, consider the task of organizing a cluttered closet:
function organizeSpace(space) {
if (space is small enough to organize easily) {
organizeItems(space)
} else {
divideIntoSmallerSpaces(space)
for each smallerSpace in space {
organizeSpace(smallerSpace) // Recursive call
}
}
}
This recursive approach allows us to break down complex tasks into manageable pieces, tackling each smaller part with the same strategy we use for the whole.
Data Structures in Information Management
Programmers use various data structures to efficiently store and manage information. Similarly, we can apply these concepts to organize information in our daily lives.
For example, using a stack (last-in-first-out) for managing tasks:
let taskStack = []
function addTask(task) {
taskStack.push(task)
}
function completeTask() {
return taskStack.pop()
}
// Usage
addTask("Reply to emails")
addTask("Prepare presentation")
addTask("Call client")
while (taskStack.length > 0) {
let currentTask = completeTask()
// Do the task
}
This approach ensures that we tackle our most recent and often most urgent tasks first.
Algorithms in Everyday Problem Solving
Algorithms are step-by-step procedures for solving problems or accomplishing tasks. While we may not think of our daily problem-solving in terms of formal algorithms, we often use algorithmic thinking without realizing it.
For instance, consider the process of finding the fastest route to work:
function findFastestRoute(start, end) {
let possibleRoutes = getAllRoutes(start, end)
let fastestRoute = null
let shortestTime = Infinity
for each route in possibleRoutes {
let time = estimateTravelTime(route)
if (time < shortestTime) {
shortestTime = time
fastestRoute = route
}
}
return fastestRoute
}
This algorithmic approach to finding the best route mirrors how navigation apps work, but it’s also similar to how we might mentally evaluate different routes based on traffic, distance, and other factors.
Version Control in Personal and Professional Growth
In software development, version control systems like Git are used to track changes in code over time. This concept can be applied to personal and professional development.
Imagine tracking your skill development like this:
// Initial state
let skills = {
"programming": 3,
"communication": 4,
"teamwork": 3
}
function learnNewSkill(skill, improvement) {
if (skill in skills) {
skills[skill] += improvement
} else {
skills[skill] = improvement
}
commitSkillUpdate(skill, improvement)
}
function commitSkillUpdate(skill, improvement) {
// Log the improvement with a timestamp
console.log(`${new Date()}: Improved ${skill} by ${improvement}`)
}
// Usage
learnNewSkill("programming", 1)
learnNewSkill("public speaking", 2)
By “committing” our personal growth regularly, we can track our progress over time and identify areas for improvement.
Continuous Integration and Deployment in Habit Formation
In software development, Continuous Integration and Continuous Deployment (CI/CD) involve automatically testing and deploying code changes. This concept can be applied to forming and maintaining habits.
Consider a habit-tracking system:
let habits = {
"exercise": {streak: 0, goal: 30},
"meditation": {streak: 0, goal: 60},
"reading": {streak: 0, goal: 20}
}
function trackHabit(habit) {
habits[habit].streak++
if (habits[habit].streak >= habits[habit].goal) {
console.log(`Congratulations! You've reached your ${habit} goal!`)
}
}
function breakStreak(habit) {
habits[habit].streak = 0
console.log(`Oh no! You broke your ${habit} streak. Time to start again!`)
}
// Daily check-in
function dailyUpdate() {
for (let habit in habits) {
let response = prompt(`Did you ${habit} today? (yes/no)`)
if (response === "yes") {
trackHabit(habit)
} else {
breakStreak(habit)
}
}
}
This system mimics CI/CD by regularly “testing” our habits and providing immediate feedback, helping us to continuously improve and “deploy” better versions of ourselves.
Conclusion: The Power of Algorithmic Thinking
As we’ve explored throughout this article, the principles of programming and algorithmic thinking are not confined to the world of computers. They are powerful tools that can be applied to a wide range of everyday situations, from simple decision-making to complex problem-solving.
By recognizing the parallels between coding logic and our daily thought processes, we can:
- Approach problems more systematically
- Break down complex tasks into manageable steps
- Optimize our routines for greater efficiency
- Handle unexpected situations more gracefully
- Track and improve our personal growth
While we don’t need to think in strict programming terms all the time, being aware of these parallels can provide us with valuable frameworks for tackling life’s challenges. Whether you’re a programmer or not, cultivating algorithmic thinking can enhance your problem-solving skills and decision-making processes in numerous aspects of your life.
So the next time you face a complex problem or decision, try approaching it like a programmer. Break it down, consider your variables, plan for potential errors, and optimize your solution. You might be surprised at how effective this approach can be in navigating the complexities of everyday life.