The Importance of Failure: Learning from Coding Mistakes
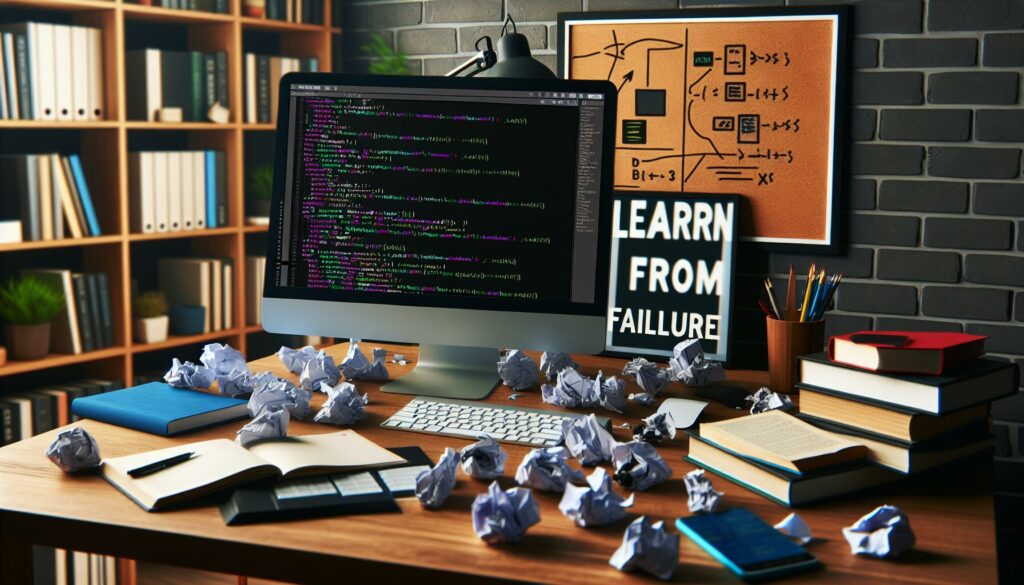
In the world of programming, failure is not just an occasional visitor—it’s a constant companion. Every developer, from novice to expert, encounters errors, bugs, and setbacks on a daily basis. While these moments can be frustrating, they are also invaluable opportunities for growth and learning. In this comprehensive guide, we’ll explore why embracing failure is crucial for becoming a better programmer and how you can turn your coding mistakes into stepping stones for success.
The Inevitability of Failure in Programming
Before we dive into the benefits of failure, it’s important to understand that making mistakes is an inherent part of the coding process. Here’s why:
- Complexity of Code: Modern software systems are incredibly complex, often involving millions of lines of code and numerous interdependencies.
- Rapidly Evolving Technology: Programming languages, frameworks, and best practices are constantly changing, making it challenging to stay up-to-date.
- Human Error: No matter how experienced you are, you’re bound to make mistakes occasionally—it’s part of being human.
- Unique Problem-Solving: Many programming tasks involve solving unique problems, which often leads to trial and error.
Recognizing that failure is normal and expected can help alleviate the stress and self-doubt that often accompany coding mistakes.
The Learning Potential in Coding Mistakes
Now that we’ve established the ubiquity of failure in programming, let’s explore why these setbacks can be powerful learning opportunities:
1. Deepening Understanding of Concepts
When you encounter an error or bug, you’re forced to dig deeper into the underlying concepts and mechanisms of your code. This process often leads to a more profound understanding of programming principles, language syntax, and system architecture.
For example, consider this Python code snippet with a common mistake:
def calculate_average(numbers):
total = 0
for num in numbers:
total += num
return total / len(numbers)
result = calculate_average([1, 2, 3, 4, 5, ""])
print(result)
This code will raise a TypeError because we’re trying to add an empty string to a number. Debugging this error teaches you about type checking, the importance of data validation, and how Python handles different data types in operations.
2. Improving Problem-Solving Skills
Debugging is essentially a form of problem-solving. Each time you encounter and fix an issue, you’re honing your analytical skills and developing strategies for tackling complex problems. This skill set is invaluable not just in programming, but in many aspects of life and work.
3. Enhancing Attention to Detail
Many coding errors stem from small oversights—a misplaced semicolon, a forgotten closing bracket, or an off-by-one error in a loop. As you encounter and fix these mistakes, you’ll naturally become more attentive to details in your code, leading to fewer errors in the future.
4. Fostering Creativity
When faced with a persistent bug or a seemingly unsolvable problem, programmers often need to think outside the box. This necessity can spark creative solutions and innovative approaches to coding challenges.
5. Building Resilience
Programming can be frustrating, especially when you’re stuck on a problem for hours or days. However, each time you persevere and overcome a challenge, you build mental resilience. This grit is crucial not only for your programming career but for personal growth as well.
Strategies for Learning from Coding Mistakes
While failures provide learning opportunities, it’s essential to approach them with the right mindset and strategies. Here are some effective ways to maximize the learning potential of your coding mistakes:
1. Embrace a Growth Mindset
Adopt a perspective that views challenges as opportunities for growth rather than insurmountable obstacles. Remember that every expert was once a beginner, and every successful programmer has a long history of failures behind their achievements.
2. Analyze Your Errors
When you encounter a bug or error, don’t just fix it and move on. Take the time to understand:
- What caused the error?
- Why did your initial approach fail?
- What underlying concept or principle did you misunderstand?
- How can you prevent similar errors in the future?
3. Keep a Coding Journal
Maintain a log of the errors you encounter, the solutions you implement, and the lessons you learn. This journal can serve as a personal knowledge base and a reminder of how far you’ve come in your coding journey.
4. Pair Programming and Code Reviews
Collaborate with other developers through pair programming sessions or code reviews. These practices expose you to different perspectives and problem-solving approaches, helping you learn from others’ experiences and mistakes as well as your own.
5. Practice Deliberate Error-Making
Intentionally introduce errors into your code and then debug them. This controlled practice can help you become more familiar with common error types and hone your debugging skills in a low-stakes environment.
6. Utilize Debugging Tools
Learn to use debugging tools effectively. Most modern IDEs come with powerful debuggers that can help you understand the flow of your program and pinpoint issues more efficiently.
7. Seek Feedback
Don’t hesitate to ask for help or feedback from more experienced programmers. Many developers are happy to share their knowledge, and explaining your problem to others can often lead to new insights.
Real-World Examples of Learning from Coding Failures
Let’s look at some real-world scenarios where coding failures led to significant learning and improvement:
1. The Mars Climate Orbiter Mishap
In 1999, NASA lost the $125 million Mars Climate Orbiter due to a simple unit conversion error. One team used metric units, while another used imperial units, causing the spacecraft to enter Mars’ atmosphere at the wrong angle and disintegrate.
Lesson Learned: This costly mistake highlighted the critical importance of clear communication, standardization, and thorough testing in software development, especially in high-stakes projects.
2. Amazon’s 2018 Prime Day Glitch
In 2018, Amazon’s website experienced significant downtime during its Prime Day sale due to a misconfigured data storage system. The issue cost the company an estimated $72 million in lost sales.
Lesson Learned: This incident emphasized the need for robust testing of systems under extreme load conditions and the importance of having well-planned failover and recovery mechanisms.
3. The Y2K Bug
While not a failure per se, the Y2K bug—where many computer systems were expected to malfunction due to the year change from 1999 to 2000—taught valuable lessons about long-term thinking in software design.
Lesson Learned: This potential crisis highlighted the importance of considering future scalability and potential edge cases when designing software systems.
Overcoming the Fear of Failure
Despite the learning potential in failures, many programmers still fear making mistakes. This fear can be paralyzing and hinder growth. Here are some strategies to overcome this fear:
1. Reframe Your Perspective
Instead of viewing mistakes as failures, see them as experiments. Each error is simply a data point that tells you what doesn’t work, bringing you one step closer to finding what does work.
2. Set Realistic Expectations
Understand that perfection is unattainable, especially in programming. Set realistic goals and celebrate small victories along the way.
3. Create a Supportive Environment
Surround yourself with supportive peers and mentors who understand that mistakes are part of the learning process. A positive environment can make it easier to take risks and learn from failures.
4. Practice Self-Compassion
Be kind to yourself when you make mistakes. Remember that even the most experienced programmers encounter errors and setbacks regularly.
5. Focus on the Process, Not Just the Outcome
Emphasize the learning and problem-solving process rather than solely focusing on the end result. This shift in focus can make failures feel less daunting and more valuable.
Incorporating Failure into Your Learning Process
To truly benefit from your coding mistakes, it’s crucial to integrate failure into your learning process systematically. Here are some ways to do this:
1. Set Learning Goals, Not Just Performance Goals
Instead of focusing solely on completing projects or features, set specific learning goals for each coding session. For example, “Today, I want to understand how to handle exceptions in Python better.”
2. Conduct Regular Retrospectives
After completing a project or a significant coding session, take time to reflect on what went well, what didn’t, and what you learned. This practice can help you identify patterns in your mistakes and areas for improvement.
3. Create a Personal Error Catalog
Maintain a list of common errors you encounter, along with their solutions and the lessons learned. This catalog can serve as a quick reference and a reminder of your progress.
4. Engage in Code Katas
Practice coding katas—small, focused programming exercises—regularly. These exercises provide a safe space to make mistakes and learn from them without the pressure of a real-world project.
5. Participate in Coding Challenges
Join online coding challenges or hackathons. These events often push you out of your comfort zone, leading to valuable learning experiences through trial and error.
The Role of Failure in Professional Growth
As you progress in your programming career, the ability to learn from failures becomes increasingly important. Here’s how embracing failure can contribute to your professional growth:
1. Developing a Problem-Solving Reputation
Employers and colleagues value developers who can tackle challenging problems head-on. By learning from your failures, you develop a reputation as someone who can handle complex issues effectively.
2. Becoming a Better Team Player
Understanding the value of failure makes you more empathetic towards your teammates’ struggles. This empathy fosters better collaboration and a more positive team dynamic.
3. Driving Innovation
A willingness to experiment and learn from failures is crucial for innovation. Many groundbreaking technologies and solutions emerge from repeated failures and iterations.
4. Enhancing Leadership Skills
As you advance in your career, the ability to guide others through failures becomes a valuable leadership skill. Your experiences can help mentor junior developers and create a culture of continuous learning within your team.
Conclusion: Embracing Failure as a Path to Success
In the world of programming, failure is not the opposite of success—it’s an integral part of the journey towards it. By embracing your coding mistakes and viewing them as valuable learning opportunities, you open yourself up to deeper understanding, enhanced problem-solving skills, and continuous growth as a developer.
Remember that every error message, every bug, and every failed attempt is a chance to learn something new. As you navigate your programming journey, maintain a curious and resilient mindset, always asking, “What can I learn from this?” With this approach, you’ll not only become a better coder but also a more adaptable and innovative problem-solver in all aspects of your life and career.
So the next time you encounter a perplexing bug or a seemingly insurmountable coding challenge, take a deep breath and remind yourself: This is not a setback—it’s an opportunity to grow. Embrace the failure, learn from it, and watch as it propels you towards greater heights in your programming journey.