From Zero to MAANG: The Ultimate 6-Month Coding Interview Preparation Plan
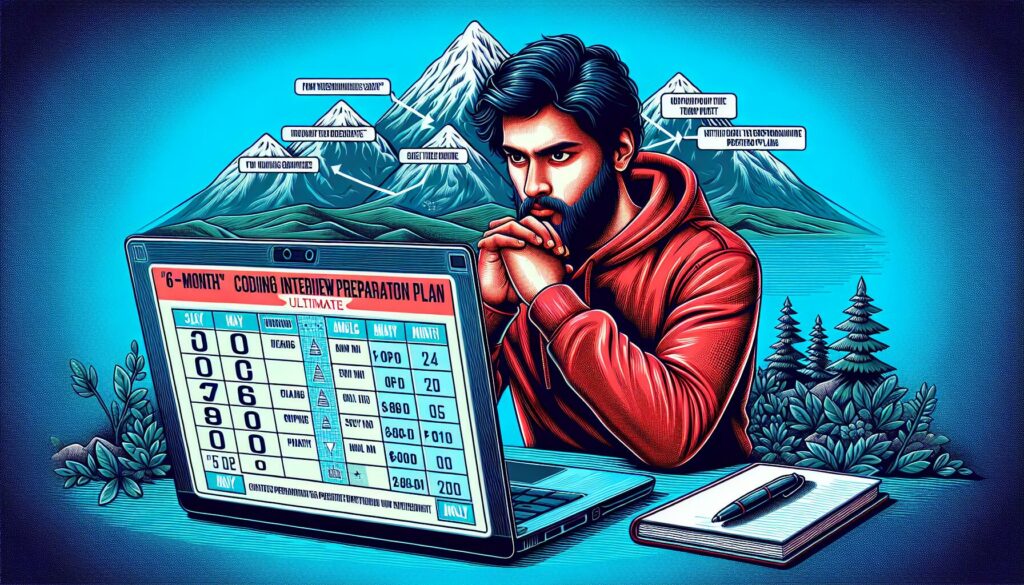
Month 1: Laying the Foundation
Week 1-2: Programming Basics
If you’re starting from scratch, begin with the fundamentals of programming. Choose a language popular in technical interviews, such as Python or Java.
- Learn basic syntax, variables, data types, and control structures
- Practice writing simple programs to solve basic problems
- Familiarize yourself with functions and basic input/output operations
Resources:
- Codecademy’s Python or Java course
- freeCodeCamp’s Programming Fundamentals
- AlgoCademy’s Beginner Python Track
Week 3-4: Data Structures Basics
Understanding data structures is crucial for coding interviews. Start with the basics:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Hash Tables
For each data structure:
- Learn the concept and use cases
- Implement the data structure from scratch
- Solve easy problems using these data structures
Resources:
- GeeksforGeeks Data Structures tutorials
- “Cracking the Coding Interview” by Gayle Laakmann McDowell (Chapters on Data Structures)
- AlgoCademy’s Data Structures course
Month 2: Algorithms and Problem-Solving
Week 5-6: Basic Algorithms
Dive into fundamental algorithms that form the basis of more complex problem-solving:
- Sorting algorithms (Bubble Sort, Selection Sort, Insertion Sort)
- Searching algorithms (Linear Search, Binary Search)
- Basic string manipulation algorithms
- Time and space complexity analysis (Big O notation)
Practice implementing these algorithms and analyzing their efficiency.
Resources:
- MIT OpenCourseWare: Introduction to Algorithms
- AlgoExpert’s Algorithms course
- LeetCode’s Explore Card: Algorithm I
Week 7-8: Problem-Solving Techniques
Learn and practice essential problem-solving paradigms:
- Brute Force approach
- Two-pointer technique
- Sliding Window
- Divide and Conquer
Start solving easy to medium difficulty problems on platforms like LeetCode, HackerRank, or AlgoCademy. Aim to solve at least 2-3 problems daily.
Resources:
- AlgoCademy’s Problem-Solving Patterns course
- “Grokking Algorithms” by Aditya Bhargava
- LeetCode’s curated lists of problems by patterns
Month 3: Advanced Data Structures and Algorithms
Week 9-10: Advanced Data Structures
Build upon your knowledge with more complex data structures:
- Trees (Binary Trees, Binary Search Trees, Balanced Trees)
- Heaps and Priority Queues
- Graphs (representation, traversal algorithms)
- Trie
Implement these data structures and solve related problems. Focus on understanding the trade-offs between different data structures and when to use each.
Resources:
- AlgoCademy’s Advanced Data Structures course
- Coursera: Data Structures and Algorithms Specialization
- “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein (CLRS)
Week 11-12: Advanced Algorithms
Delve into more sophisticated algorithmic techniques:
- Dynamic Programming
- Greedy Algorithms
- Backtracking
- Advanced Graph Algorithms (Dijkstra’s, Bellman-Ford, Floyd-Warshall)
These topics are often considered the most challenging in coding interviews. Spend extra time understanding and practicing these concepts.
Resources:
- AlgoCademy’s Dynamic Programming course
- Stanford’s Algorithms Specialization on Coursera
- “Algorithms” by Robert Sedgewick and Kevin Wayne
Month 4: Intensive Problem Solving
Week 13-14: Medium Difficulty Problems
Now that you have a strong foundation, it’s time to ramp up your problem-solving skills:
- Solve at least 5 medium-difficulty problems daily
- Focus on a variety of problem types to broaden your skills
- Time yourself to improve speed and efficiency
- Review and optimize your solutions
Resources:
- LeetCode’s Top Interview Questions (Medium Collection)
- AlgoCademy’s Curated Problem Sets
- HackerRank Interview Preparation Kit
Week 15-16: Hard Problems and Optimization
Challenge yourself with more difficult problems:
- Attempt 2-3 hard problems daily
- Focus on optimizing your solutions for time and space complexity
- Practice explaining your thought process and approach
- Learn to identify and apply multiple approaches to a single problem
Resources:
- LeetCode’s Top Interview Questions (Hard Collection)
- AlgoCademy’s Advanced Problem-Solving Techniques
- InterviewBit’s Programming track
Month 5: System Design and Object-Oriented Design
Week 17-18: Introduction to System Design
System design is a crucial component of many tech interviews, especially for more senior positions:
- Learn basic system design principles
- Study scalability concepts (vertical vs. horizontal scaling, load balancing)
- Understand database design (SQL vs. NoSQL, sharding, replication)
- Explore caching strategies and content delivery networks (CDNs)
Practice designing simple systems and explaining your design choices.
Resources:
- “System Design Interview” by Alex Xu
- Grokking the System Design Interview on Educative.io
- YouTube channel: Tech Dummies Narendra L
Week 19-20: Advanced System Design and Object-Oriented Design
Deepen your understanding of system design and learn object-oriented design principles:
- Study real-world system architectures (e.g., Netflix, Uber, Twitter)
- Learn about microservices architecture and API design
- Understand SOLID principles and design patterns
- Practice designing object-oriented solutions to common problems
Resources:
- “Designing Data-Intensive Applications” by Martin Kleppmann
- AlgoCademy’s Object-Oriented Design course
- “Head First Design Patterns” by Eric Freeman and Elisabeth Robson
Month 6: Mock Interviews and Final Preparation
Week 21-22: Mock Interviews and Behavioral Preparation
Start simulating real interview experiences:
- Participate in mock interviews (use platforms like Pramp or find a study buddy)
- Practice thinking out loud and explaining your problem-solving process
- Work on your communication skills and ability to ask clarifying questions
- Prepare for behavioral questions using the STAR method
Resources:
- Pramp for peer mock interviews
- AlgoCademy’s Interview Simulation feature
- “Cracking the Coding Interview” (Behavioral Questions section)
Week 23-24: Final Review and Polishing
Use these last two weeks to reinforce your knowledge and fill any gaps:
- Review all major topics, focusing on areas where you feel less confident
- Solve a mix of problems daily, covering various difficulty levels and topics
- Practice system design and object-oriented design questions
- Refine your resume and prepare a strong self-introduction
Resources:
- AlgoCademy’s Comprehensive Review Track
- GeeksforGeeks Last Minute Notes
- Glassdoor interview experiences for your target companies
Additional Tips for Success
Consistency is Key
Maintain a consistent study schedule throughout the 6 months. Even on busy days, try to solve at least one problem or review a concept. Consistency beats intensity in the long run.
Use Spaced Repetition
Implement a spaced repetition system to review concepts and problems you’ve learned. This helps reinforce your memory and prevents forgetting crucial information.
Join Coding Communities
Engage with coding communities on platforms like Reddit (/r/cscareerquestions, /r/leetcode) or Discord. Discussing problems and sharing experiences can provide valuable insights and motivation.
Focus on Understanding, Not Just Memorization
While it’s important to know common patterns and solutions, focus on truly understanding the underlying principles. This will help you adapt to new problems more effectively.
Take Care of Your Health
Don’t neglect your physical and mental health. Regular exercise, proper sleep, and stress management are crucial for maintaining focus and retaining information during this intensive preparation period.
Conclusion
Preparing for coding interviews at top tech companies is a challenging but rewarding journey. This 6-month plan provides a structured approach to build your skills from the ground up, covering all essential aspects of coding interviews. Remember, the key to success is consistent practice, a growth mindset, and the ability to apply your knowledge to solve new problems.
As you progress through this plan, you’ll not only prepare for interviews but also become a more skilled and confident programmer. The habits and problem-solving skills you develop will serve you well throughout your career, regardless of where you end up working.
Stay motivated, track your progress, and don’t hesitate to adjust the plan to fit your personal learning style and pace. With dedication and smart preparation, you’ll be well-equipped to tackle even the most challenging coding interviews at MAANG companies and beyond. Good luck on your journey from zero to MAANG!
Code Example: Two Sum Problem
To illustrate the kind of problem-solving you’ll encounter, let’s look at a classic coding interview question: the Two Sum problem. This problem is often asked in interviews and is an excellent example of how to optimize a solution.
Problem Statement: Given an array of integers nums and an integer target, return indices of the two numbers such that they add up to target. You may assume that each input would have exactly one solution, and you may not use the same element twice.
Here’s a Python implementation with two approaches:
Approach 1: Brute Force (O(n^2) time complexity)
def two_sum_brute_force(nums, target):
for i in range(len(nums)):
for j in range(i + 1, len(nums)):
if nums[i] + nums[j] == target:
return [i, j]
return [] # No solution found
# Example usage
nums = [2, 7, 11, 15]
target = 9
print(two_sum_brute_force(nums, target)) # Output: [0, 1]
Approach 2: Hash Table (O(n) time complexity)
def two_sum_hash(nums, target):
num_map = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_map:
return [num_map[complement], i]
num_map[num] = i
return [] # No solution found
# Example usage
nums = [2, 7, 11, 15]
target = 9
print(two_sum_hash(nums, target)) # Output: [0, 1]
This example demonstrates how to optimize a solution from a brute force approach to a more efficient one using a hash table. Understanding such optimizations and being able to explain them is crucial for success in coding interviews.
As you work through this 6-month plan, you’ll encounter many such problems and learn various techniques to solve them efficiently. Remember to not just memorize solutions, but understand the underlying principles and patterns. This will enable you to tackle new, unseen problems during your interviews and throughout your programming career.