Cracking the Coding Interview: A Comprehensive Guide to Acing Your Tech Job Interview
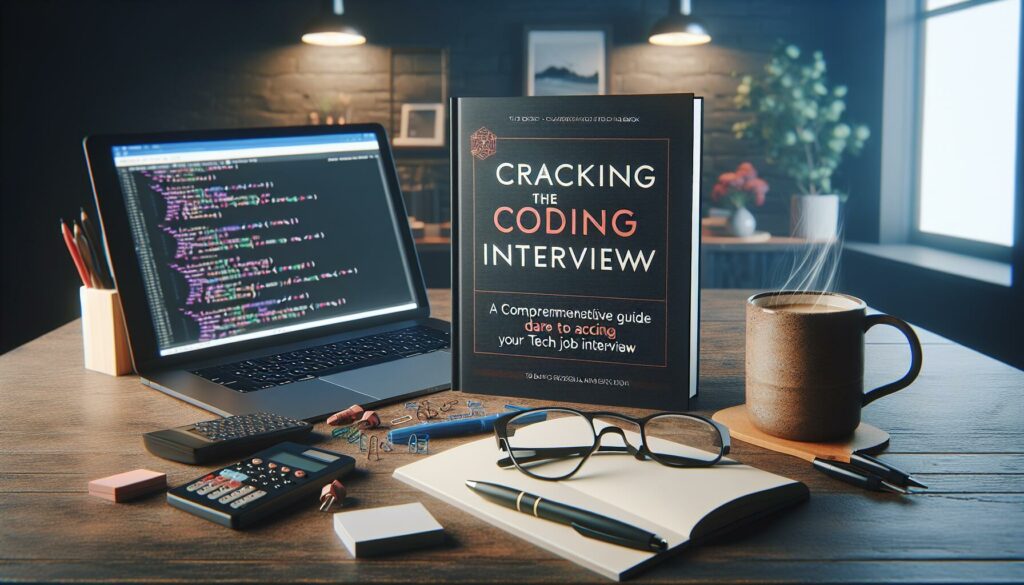
In today’s competitive tech industry, landing a job at a top company like Google, Amazon, or Facebook (often collectively referred to as FAANG – Facebook, Amazon, Apple, Netflix, and Google) requires more than just a strong resume. The coding interview has become a crucial hurdle that candidates must overcome to secure their dream positions. This comprehensive guide will walk you through the essential steps and strategies to help you crack the coding interview and launch your career in the tech world.
Table of Contents
- Understanding the Coding Interview
- Preparing for Success
- Mastering Data Structures and Algorithms
- Problem-Solving Strategies
- Mock Interviews and Practice
- Common Interview Questions
- Coding Best Practices
- Behavioral Interviews
- Company-Specific Preparation
- Interview Day Tips
- Post-Interview Follow-Up
- Continuous Learning and Improvement
1. Understanding the Coding Interview
Before diving into preparation strategies, it’s crucial to understand what a coding interview entails. Typically, a coding interview is a technical assessment where candidates are asked to solve programming problems in real-time. These interviews aim to evaluate your:
- Problem-solving skills
- Coding proficiency
- Algorithmic thinking
- Communication abilities
- Ability to work under pressure
Coding interviews can take various formats, including:
- Whiteboard coding: Solving problems on a whiteboard without access to a computer
- Online coding platforms: Using web-based IDEs to write and test code
- Take-home assignments: Completing a project or set of problems within a given timeframe
- Pair programming: Collaborating with an interviewer to solve a problem
Understanding these formats will help you tailor your preparation and feel more confident during the actual interview.
2. Preparing for Success
Preparation is key to succeeding in coding interviews. Here’s a structured approach to get you started:
2.1. Assess Your Current Skills
Begin by honestly evaluating your current coding skills. Identify your strengths and weaknesses in various programming concepts, data structures, and algorithms. This self-assessment will help you create a focused study plan.
2.2. Create a Study Plan
Based on your assessment, create a comprehensive study plan that covers all essential topics. Allocate more time to areas where you need improvement. Your plan should include:
- Daily coding practice
- Review of fundamental concepts
- Learning new algorithms and data structures
- Mock interviews and problem-solving sessions
2.3. Choose the Right Resources
Select high-quality resources to aid your preparation. Some popular options include:
- Books: “Cracking the Coding Interview” by Gayle Laakmann McDowell, “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein
- Online platforms: LeetCode, HackerRank, CodeSignal
- Courses: AlgoCademy, Coursera’s “Algorithms” specialization, MIT OpenCourseWare
- YouTube channels: MIT OpenCourseWare, mycodeschool, Back To Back SWE
2.4. Set Realistic Goals
Set achievable goals for your preparation. This might include solving a certain number of problems per week, mastering specific algorithms, or completing a course. Regularly review and adjust your goals as needed.
3. Mastering Data Structures and Algorithms
A solid understanding of data structures and algorithms is crucial for success in coding interviews. Here’s a list of essential topics to focus on:
3.1. Data Structures
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
3.2. Algorithms
- Sorting (e.g., Quicksort, Mergesort, Heapsort)
- Searching (e.g., Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Greedy Algorithms
- Recursion and Backtracking
- Graph Algorithms (e.g., Dijkstra’s, Kruskal’s)
For each data structure and algorithm, make sure you understand:
- The underlying concept and how it works
- Time and space complexity analysis
- Common use cases and applications
- Implementation details in your preferred programming language
Practice implementing these data structures and algorithms from scratch to deepen your understanding.
4. Problem-Solving Strategies
Developing effective problem-solving strategies is crucial for tackling coding interview questions. Here’s a step-by-step approach to solving problems:
4.1. Understand the Problem
- Read the problem statement carefully
- Identify the input and output requirements
- Ask clarifying questions if needed
- Consider edge cases and constraints
4.2. Plan Your Approach
- Break down the problem into smaller sub-problems
- Consider multiple approaches and their trade-offs
- Choose the most appropriate data structures and algorithms
- Sketch out a high-level solution
4.3. Implement the Solution
- Write clean, modular code
- Use meaningful variable and function names
- Handle edge cases and potential errors
- Optimize your code where possible
4.4. Test and Debug
- Walk through your code with sample inputs
- Test edge cases and boundary conditions
- Identify and fix any bugs or logical errors
- Analyze the time and space complexity of your solution
4.5. Optimize and Improve
- Consider alternative approaches or optimizations
- Discuss trade-offs between different solutions
- Refactor your code for better readability and efficiency
Remember to communicate your thought process clearly throughout the problem-solving stages. This allows the interviewer to understand your approach and provide guidance if needed.
5. Mock Interviews and Practice
Practicing mock interviews is an essential part of your preparation. It helps you get comfortable with the interview format, improve your problem-solving skills, and build confidence. Here are some ways to incorporate mock interviews into your preparation:
5.1. Online Platforms
Utilize online platforms that offer mock interview experiences:
- Pramp: Peer-to-peer mock interviews with other candidates
- InterviewBit: Simulated coding interviews with detailed feedback
- LeetCode’s Mock Interview feature
5.2. Study Groups
Form or join study groups with other aspiring developers. Take turns interviewing each other and providing constructive feedback.
5.3. Professional Mock Interviews
Consider hiring a professional mock interviewer or using services like Interviewing.io for expert feedback and guidance.
5.4. Self-Practice
Conduct self-practice sessions by timing yourself while solving problems and explaining your thought process out loud.
5.5. Record and Review
Record your mock interview sessions and review them to identify areas for improvement in your communication and problem-solving approach.
6. Common Interview Questions
While each interview is unique, certain types of questions frequently appear in coding interviews. Familiarize yourself with these common question categories:
6.1. Array and String Manipulation
Example: Implement a function to reverse a string in-place.
def reverse_string(s):
left, right = 0, len(s) - 1
s = list(s)
while left < right:
s[left], s[right] = s[right], s[left]
left += 1
right -= 1
return ''.join(s)
# Test the function
print(reverse_string("Hello, World!")) # Output: !dlroW ,olleH
6.2. Linked List Operations
Example: Detect a cycle in a linked list.
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def has_cycle(head):
if not head or not head.next:
return False
slow = head
fast = head.next
while slow != fast:
if not fast or not fast.next:
return False
slow = slow.next
fast = fast.next.next
return True
# Test the function
# Create a linked list with a cycle
node1 = ListNode(1)
node2 = ListNode(2)
node3 = ListNode(3)
node4 = ListNode(4)
node1.next = node2
node2.next = node3
node3.next = node4
node4.next = node2 # Creates a cycle
print(has_cycle(node1)) # Output: True
6.3. Tree Traversal and Manipulation
Example: Implement an in-order traversal of a binary tree.
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def inorder_traversal(root):
result = []
def inorder(node):
if node:
inorder(node.left)
result.append(node.val)
inorder(node.right)
inorder(root)
return result
# Test the function
# Create a binary tree
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.left.left = TreeNode(4)
root.left.right = TreeNode(5)
print(inorder_traversal(root)) # Output: [4, 2, 5, 1, 3]
6.4. Dynamic Programming
Example: Implement a function to find the nth Fibonacci number using dynamic programming.
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
# Test the function
print(fibonacci(10)) # Output: 55
6.5. Graph Algorithms
Example: Implement Depth-First Search (DFS) on a graph.
from collections import defaultdict
class Graph:
def __init__(self):
self.graph = defaultdict(list)
def add_edge(self, u, v):
self.graph[u].append(v)
def dfs_util(self, v, visited):
visited.add(v)
print(v, end=' ')
for neighbor in self.graph[v]:
if neighbor not in visited:
self.dfs_util(neighbor, visited)
def dfs(self, v):
visited = set()
self.dfs_util(v, visited)
# Test the function
g = Graph()
g.add_edge(0, 1)
g.add_edge(0, 2)
g.add_edge(1, 2)
g.add_edge(2, 0)
g.add_edge(2, 3)
g.add_edge(3, 3)
print("DFS starting from vertex 2:")
g.dfs(2) # Output: 2 0 1 3
Practice solving these types of questions and variations of them to build your problem-solving skills and familiarity with common interview topics.
7. Coding Best Practices
During a coding interview, it’s not just about finding the correct solution; how you write your code matters too. Here are some best practices to follow:
7.1. Write Clean and Readable Code
- Use consistent indentation and formatting
- Follow naming conventions for variables, functions, and classes
- Keep functions small and focused on a single task
- Use comments to explain complex logic or assumptions
7.2. Optimize for Efficiency
- Consider time and space complexity of your solutions
- Look for opportunities to optimize your code
- Discuss trade-offs between different approaches
7.3. Handle Edge Cases
- Consider and handle null inputs, empty collections, and boundary conditions
- Implement input validation where appropriate
7.4. Use Appropriate Data Structures
- Choose the most suitable data structures for the problem at hand
- Understand the pros and cons of different data structures
7.5. Write Modular and Reusable Code
- Break down complex problems into smaller, manageable functions
- Avoid code duplication by creating reusable helper functions
7.6. Test Your Code
- Write test cases to verify your solution
- Consider edge cases and potential errors in your tests
8. Behavioral Interviews
In addition to technical skills, many companies also conduct behavioral interviews to assess your soft skills, work style, and cultural fit. Here are some tips for acing behavioral interviews:
8.1. Use the STAR Method
When answering behavioral questions, use the STAR (Situation, Task, Action, Result) method to structure your responses:
- Situation: Describe the context or background
- Task: Explain the challenge or goal you faced
- Action: Describe the steps you took to address the situation
- Result: Share the outcomes and what you learned
8.2. Prepare Stories
Have a set of prepared stories that showcase your skills and experiences. These might include:
- A time you overcame a significant challenge
- An example of your leadership or teamwork skills
- A situation where you had to resolve a conflict
- A project you’re particularly proud of
8.3. Research the Company
Familiarize yourself with the company’s values, culture, and recent news. This will help you tailor your responses and ask informed questions.
8.4. Practice Common Questions
Prepare for common behavioral questions such as:
- “Tell me about a time when you faced a difficult technical challenge.”
- “How do you handle disagreements with team members?”
- “Describe a situation where you had to meet a tight deadline.”
8.5. Be Honest and Authentic
While it’s important to present yourself in the best light, always be truthful in your responses. Authenticity is key to building trust with your interviewer.
9. Company-Specific Preparation
Different companies have different interview processes and focus areas. Tailor your preparation to the specific company you’re interviewing with:
9.1. Research the Interview Process
- Understand the number and types of interviews you’ll face
- Learn about any company-specific coding platforms or tools
- Familiarize yourself with the company’s preferred programming languages
9.2. Study Company-Specific Topics
- Review the company’s products and technologies
- Focus on areas relevant to the role you’re applying for
- Understand the company’s engineering challenges and how they solve them
9.3. Practice Company-Specific Questions
- Look for interview experiences shared by other candidates on platforms like Glassdoor or LeetCode
- Practice problems that are frequently asked by the company
9.4. Understand the Company Culture
- Research the company’s values and mission
- Read about the company’s engineering blog or tech talks
- Connect with current or former employees if possible
10. Interview Day Tips
On the day of your interview, follow these tips to perform at your best:
10.1. Prepare Your Environment
- Ensure a quiet, well-lit space for virtual interviews
- Test your audio and video equipment in advance
- Have a pen and paper ready for notes
10.2. Manage Your Time
- Arrive early or log in to virtual interviews ahead of time
- Pace yourself during coding challenges
- Ask for clarification if you’re unsure about time constraints
10.3. Communicate Clearly
- Explain your thought process as you work through problems
- Ask questions to clarify requirements or constraints
- Be open to hints or suggestions from the interviewer
10.4. Stay Calm and Focused
- Take deep breaths if you feel nervous
- If you get stuck, don’t panic – explain your thoughts and ask for guidance
- Stay positive and maintain a problem-solving mindset
10.5. Be Professional
- Dress appropriately, even for virtual interviews
- Be polite and respectful to all interviewers and staff
- Thank the interviewer for their time at the end of the interview
11. Post-Interview Follow-Up
After your interview, take these steps to leave a positive impression:
11.1. Send a Thank-You Note
- Email a personalized thank-you note to your interviewer(s) within 24-48 hours
- Express your appreciation for their time and reaffirm your interest in the position
11.2. Reflect on Your Performance
- Review the questions you were asked and how you answered them
- Identify areas where you could improve for future interviews
11.3. Follow Up on Next Steps
- If you haven’t received information about next steps, politely inquire about the timeline
- Be patient and respect the company’s hiring process
11.4. Continue Practicing
- Regardless of the outcome, continue honing your skills
- Work on areas where you felt less confident during the interview
12. Continuous Learning and Improvement
The tech industry is constantly evolving, and so should your skills. Here are some strategies for continuous learning and improvement:
12.1. Stay Updated with Industry Trends
- Follow tech blogs, podcasts, and news sites
- Attend tech conferences or webinars
- Participate in online coding communities
12.2. Contribute to Open Source Projects
- Gain real-world coding experience
- Collaborate with other developers
- Build a public portfolio of your work
12.3. Take Online Courses
- Enroll in courses on platforms like Coursera, edX, or Udacity
- Learn new programming languages or frameworks
- Deepen your understanding of computer science fundamentals
12.4. Practice Coding Regularly
- Solve coding problems on platforms like LeetCode or HackerRank
- Participate in coding competitions
- Work on personal projects to apply your skills
12.5. Seek Feedback and Mentorship
- Ask for code reviews from more experienced developers
- Find a mentor in your field of interest
- Offer to mentor others to reinforce your own knowledge
By following these strategies and continuously improving your skills, you’ll be well-prepared to crack the coding interview and succeed in your tech career. Remember that the journey to becoming a great developer is ongoing, and each interview experience, whether successful or not, is an opportunity to learn and grow.
Good luck with your coding interviews, and may your hard work and preparation lead you to the tech career of your dreams!