Functional Reactive Programming: A Comprehensive Guide for Modern Developers
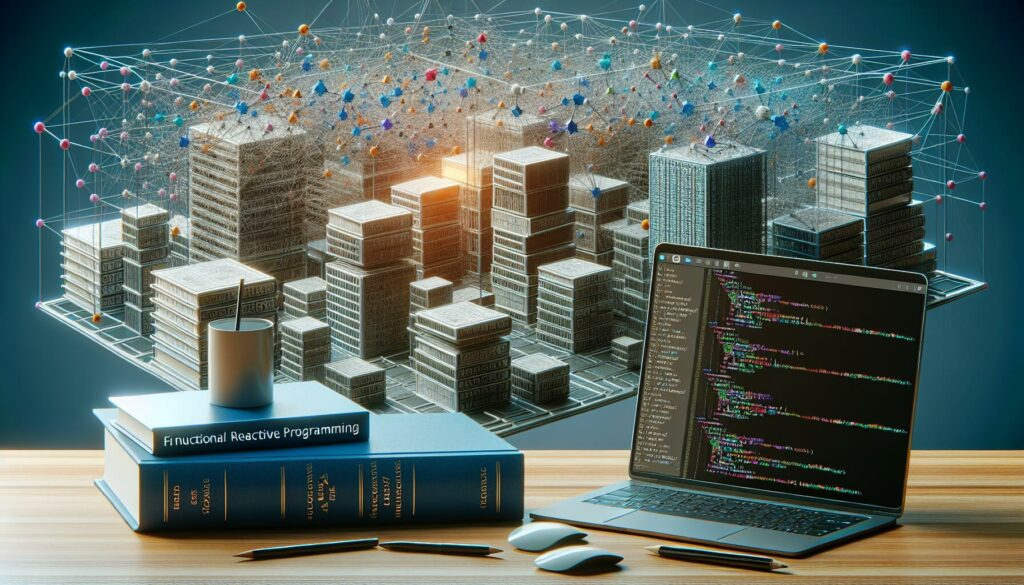
In the ever-evolving landscape of software development, new paradigms and techniques constantly emerge to address the growing complexity of applications. One such paradigm that has gained significant traction in recent years is Functional Reactive Programming (FRP). This powerful approach combines the principles of functional programming with reactive programming, offering developers a robust framework for managing asynchronous data streams and building responsive, scalable applications. In this comprehensive guide, we’ll dive deep into the world of Functional Reactive Programming, exploring its core concepts, benefits, and practical applications.
Table of Contents
- What is Functional Reactive Programming?
- Core Concepts of FRP
- Benefits of Functional Reactive Programming
- FRP vs. Traditional Programming Paradigms
- Popular FRP Libraries and Frameworks
- Practical Examples of FRP in Action
- Best Practices and Design Patterns in FRP
- Challenges and Considerations
- The Future of Functional Reactive Programming
- Conclusion
1. What is Functional Reactive Programming?
Functional Reactive Programming (FRP) is a programming paradigm that combines the principles of functional programming with reactive programming. It provides a declarative approach to handling asynchronous data streams and building reactive systems. At its core, FRP allows developers to express static and dynamic data flows using functional programming constructs, making it easier to manage complex, event-driven applications.
To understand FRP, let’s break it down into its two main components:
Functional Programming
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. Key principles of functional programming include:
- Immutability: Data cannot be changed once created
- Pure functions: Functions that always produce the same output for a given input and have no side effects
- Higher-order functions: Functions that can take other functions as arguments or return them as results
- Declarative programming: Expressing the logic of a computation without describing its control flow
Reactive Programming
Reactive programming is a programming paradigm oriented around data flows and the propagation of change. It focuses on:
- Asynchronous data streams: Sequences of events delivered over time
- Event-driven architecture: Systems that respond to events as they occur
- Data flow: The idea that changes in one part of the system automatically flow through to other parts
- Declarative event composition: Combining and manipulating event streams in a declarative manner
By combining these two paradigms, FRP provides a powerful toolset for dealing with complex, asynchronous systems in a more manageable and predictable way.
2. Core Concepts of FRP
To fully grasp Functional Reactive Programming, it’s essential to understand its core concepts:
Streams
In FRP, streams (also known as observables or signals) are the fundamental building blocks. A stream represents a sequence of values over time. These values can be anything from user inputs and server responses to sensor data or timer events. Streams can be infinite and asynchronous, allowing developers to work with data that arrives at unpredictable intervals.
Operators
Operators are functions that transform, combine, or filter streams. They allow developers to manipulate data flows declaratively. Common operators include:
- Map: Transform each value in a stream
- Filter: Select only certain values from a stream based on a predicate
- Merge: Combine multiple streams into a single stream
- Scan: Accumulate values over time, similar to a reduce operation
- SwitchMap: Transform a stream and flatten the result, useful for handling nested asynchronous operations
Subscriptions
Subscriptions allow components to react to changes in streams. When a component subscribes to a stream, it receives updates whenever new values are emitted. This creates a reactive relationship between data sources and the parts of the application that depend on them.
Time as a First-Class Citizen
FRP treats time as a core concept, allowing developers to reason about and manipulate time-based operations easily. This is particularly useful for dealing with debouncing, throttling, and other time-dependent behaviors.
Declarative Approach
FRP encourages a declarative style of programming, where developers describe what should happen rather than how it should happen. This leads to more readable and maintainable code, as the underlying system handles the complexities of managing asynchronous events and data flow.
3. Benefits of Functional Reactive Programming
Adopting Functional Reactive Programming can bring numerous benefits to your development process and the quality of your applications:
Improved Code Readability and Maintainability
FRP’s declarative nature and focus on data flows make code easier to read and understand. Complex asynchronous operations can be expressed in a more linear and intuitive manner, reducing the cognitive load on developers.
Better Handling of Asynchronous Operations
FRP provides a unified approach to dealing with various types of asynchronous events, from user interactions to network requests. This consistency simplifies error handling and makes it easier to compose complex asynchronous workflows.
Reduced State Management Complexity
By treating data as immutable streams, FRP reduces the need for complex state management. This can lead to fewer bugs related to unexpected state changes and make it easier to reason about application behavior.
Enhanced Scalability
FRP’s modular and composable nature makes it easier to scale applications. New features can be added by composing existing streams and operators, without significantly increasing the overall complexity of the system.
Better Performance
Many FRP implementations are optimized for efficient handling of asynchronous events and data flows. This can lead to improved performance, especially in applications with complex event-driven logic.
Easier Testing
The use of pure functions and immutable data in FRP makes unit testing simpler and more reliable. Streams can be easily mocked or stubbed, allowing for comprehensive testing of reactive components.
4. FRP vs. Traditional Programming Paradigms
To better understand the advantages of Functional Reactive Programming, let’s compare it to some traditional programming paradigms:
FRP vs. Imperative Programming
Imperative programming focuses on describing how a program operates through a sequence of statements that change program state. In contrast, FRP takes a declarative approach, focusing on what the program should accomplish without specifying the exact sequence of steps.
Example: Handling a button click
Imperative approach:
let count = 0;
const button = document.getElementById('myButton');
button.addEventListener('click', () => {
count++;
console.log(`Button clicked ${count} times`);
});
FRP approach (using RxJS):
import { fromEvent } from 'rxjs';
import { scan } from 'rxjs/operators';
const button = document.getElementById('myButton');
const clicks$ = fromEvent(button, 'click');
clicks$.pipe(
scan((count) => count + 1, 0)
).subscribe(count => console.log(`Button clicked ${count} times`));
In the FRP approach, we define a stream of click events and use operators to transform and react to those events declaratively.
FRP vs. Object-Oriented Programming (OOP)
While OOP focuses on organizing code into objects that encapsulate data and behavior, FRP emphasizes the flow of data through pure functions. FRP can complement OOP by providing a more functional approach to handling events and state changes within an object-oriented architecture.
FRP vs. Callback-based Asynchronous Programming
Traditional callback-based approaches to handling asynchronous operations can lead to complex and hard-to-maintain code, often referred to as “callback hell.” FRP provides a more structured and composable way to handle asynchronous operations, making it easier to reason about and maintain complex asynchronous workflows.
5. Popular FRP Libraries and Frameworks
Several libraries and frameworks have emerged to support Functional Reactive Programming across different languages and platforms:
RxJS (Reactive Extensions for JavaScript)
RxJS is one of the most popular FRP libraries for JavaScript and TypeScript. It provides a rich set of operators for working with asynchronous data streams and is widely used in Angular and other front-end frameworks.
Key features of RxJS:
- Comprehensive set of operators for transforming and combining streams
- Support for creating custom operators
- Excellent TypeScript support
- Integration with popular front-end frameworks
React with Redux-Observable
Redux-Observable is a middleware for Redux that allows you to use RxJS to handle side effects in React applications. It enables the use of FRP principles within the Redux ecosystem.
Bacon.js
Bacon.js is another popular FRP library for JavaScript. It focuses on simplicity and ease of use, making it a good choice for developers new to FRP.
Akka Streams (for Scala and Java)
Akka Streams provides a way to do FRP in Scala and Java, with a focus on building reactive, concurrent, and distributed systems.
ReactiveCocoa (for iOS/macOS)
ReactiveCocoa brings FRP to Apple’s platforms, allowing developers to use reactive patterns in Swift and Objective-C.
Reactor (for Java)
Reactor is a fourth-generation reactive library for building non-blocking applications on the JVM, based on the Reactive Streams Specification.
6. Practical Examples of FRP in Action
Let’s explore some practical examples of how Functional Reactive Programming can be applied to common development scenarios:
Example 1: Autocomplete Search
Implementing an autocomplete search feature is a classic use case for FRP. Here’s how you might implement it using RxJS:
import { fromEvent } from 'rxjs';
import { debounceTime, distinctUntilChanged, switchMap } from 'rxjs/operators';
const searchInput = document.getElementById('search-input');
const results = document.getElementById('results');
const search$ = fromEvent(searchInput, 'input').pipe(
debounceTime(300),
distinctUntilChanged(),
switchMap(event => {
const term = event.target.value;
return fetch(`https://api.example.com/search?q=${term}`).then(response => response.json());
})
);
search$.subscribe(data => {
results.innerHTML = data.map(item => `<li>${item.name}</li>`).join('');
});
This example demonstrates how FRP can elegantly handle debouncing user input, avoiding duplicate requests, and managing asynchronous API calls.
Example 2: Real-time Data Dashboard
FRP is excellent for building real-time data dashboards. Here’s a simple example using RxJS to create a dashboard that updates with stock prices:
import { interval } from 'rxjs';
import { switchMap } from 'rxjs/operators';
const stockPrices$ = interval(5000).pipe(
switchMap(() => fetch('https://api.example.com/stocks').then(response => response.json()))
);
stockPrices$.subscribe(stocks => {
stocks.forEach(stock => {
const element = document.getElementById(stock.symbol);
element.textContent = `${stock.symbol}: $${stock.price}`;
});
});
This example shows how FRP can be used to create a continuously updating dashboard with minimal code.
Example 3: Form Validation
FRP can simplify complex form validation logic. Here’s an example of real-time form validation using RxJS:
import { fromEvent, combineLatest } from 'rxjs';
import { map, debounceTime } from 'rxjs/operators';
const emailInput = document.getElementById('email');
const passwordInput = document.getElementById('password');
const submitButton = document.getElementById('submit');
const email$ = fromEvent(emailInput, 'input').pipe(
debounceTime(300),
map(event => event.target.value)
);
const password$ = fromEvent(passwordInput, 'input').pipe(
debounceTime(300),
map(event => event.target.value)
);
const form$ = combineLatest([email$, password$]).pipe(
map(([email, password]) => {
const isEmailValid = /^[^\s@]+@[^\s@]+\.[^\s@]+$/.test(email);
const isPasswordValid = password.length >= 8;
return isEmailValid && isPasswordValid;
})
);
form$.subscribe(isValid => {
submitButton.disabled = !isValid;
});
This example demonstrates how FRP can be used to create reactive form validation, updating the form’s state in real-time as the user types.
7. Best Practices and Design Patterns in FRP
To make the most of Functional Reactive Programming, consider the following best practices and design patterns:
Compose Streams for Complex Logic
Instead of creating large, monolithic streams, compose smaller, focused streams to build complex logic. This improves readability and maintainability.
Use Higher-Order Observables Wisely
Higher-order observables (observables of observables) can be powerful but complex. Use operators like switchMap, mergeMap, or concatMap to flatten them appropriately based on your use case.
Handle Errors Gracefully
Always include error handling in your streams. Use operators like catchError to handle and recover from errors without breaking the entire stream.
Unsubscribe to Avoid Memory Leaks
In long-lived applications, always unsubscribe from observables when they’re no longer needed to prevent memory leaks.
Use the AsyncSubject Pattern for Caching
When you need to cache the last value of a completed observable, consider using an AsyncSubject.
Leverage the Power of Schedulers
Use schedulers to control the execution context of your observables, which can be crucial for performance optimization and testing.
Follow the Single Responsibility Principle
Each stream should have a single, well-defined purpose. This makes your code more modular and easier to test and maintain.
8. Challenges and Considerations
While Functional Reactive Programming offers many benefits, it also comes with its own set of challenges:
Learning Curve
FRP introduces new concepts and ways of thinking about program flow, which can be challenging for developers accustomed to more traditional paradigms.
Debugging Complexity
Debugging asynchronous streams can be more complex than debugging synchronous code. Tools and techniques specific to FRP debugging are often necessary.
Potential for Overuse
Not every problem needs to be solved with FRP. Overusing reactive patterns can lead to unnecessary complexity in simple scenarios.
Performance Considerations
While FRP can lead to performance improvements in many cases, improper use of operators or creation of too many streams can impact performance negatively.
Integration with Existing Codebases
Integrating FRP into existing, non-reactive codebases can be challenging and may require significant refactoring.
9. The Future of Functional Reactive Programming
As software systems continue to grow in complexity and the demand for responsive, real-time applications increases, Functional Reactive Programming is poised to play an increasingly important role in software development:
Integration with AI and Machine Learning
FRP’s ability to handle streams of data makes it well-suited for integration with AI and machine learning systems, potentially leading to more reactive and intelligent applications.
Improved Tooling and Debugging Support
As FRP becomes more mainstream, we can expect to see better tooling and debugging support, making it easier for developers to work with reactive streams.
Standardization
While there are currently multiple libraries and approaches to FRP, we may see efforts towards standardization, similar to how the Reactive Streams specification has standardized aspects of reactive programming in Java.
Adoption in New Domains
FRP principles are likely to be adopted in new domains, such as IoT (Internet of Things) and edge computing, where handling streams of data from multiple sources is crucial.
Language-Level Support
Some programming languages may start to incorporate FRP concepts at the language level, similar to how async/await has been integrated into many languages for handling asynchronous operations.
10. Conclusion
Functional Reactive Programming represents a powerful paradigm shift in how we approach building complex, event-driven applications. By combining the principles of functional programming with reactive programming, FRP offers a declarative and composable way to handle asynchronous data flows and build responsive systems.
While it comes with its own set of challenges and considerations, the benefits of FRP – including improved code readability, better handling of asynchronous operations, and enhanced scalability – make it a valuable tool in the modern developer’s toolkit.
As you continue your journey in software development, consider exploring Functional Reactive Programming further. Start by experimenting with FRP libraries in your preferred language, and gradually incorporate reactive patterns into your projects. With practice and experience, you’ll be well-equipped to leverage the power of FRP to build more robust, responsive, and maintainable applications.
Remember, the key to mastering any programming paradigm is practice and application. So, don’t hesitate to dive in and start building with FRP. Happy coding!