Sieve of Eratosthenes: A Powerful Algorithm for Finding Prime Numbers
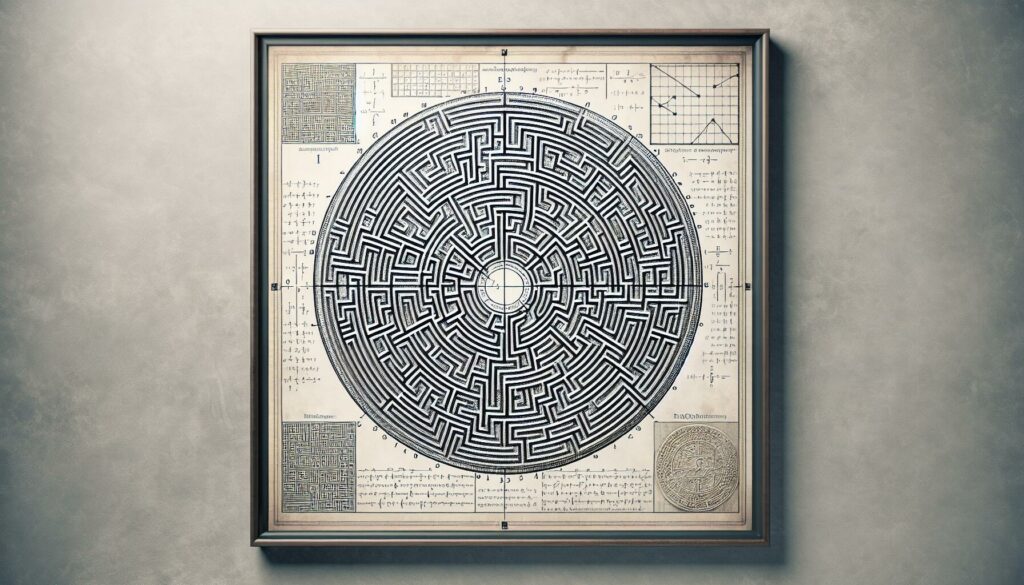
In the vast world of algorithms and mathematical computations, the Sieve of Eratosthenes stands out as an elegant and efficient method for finding prime numbers. This ancient algorithm, named after the Greek mathematician Eratosthenes of Cyrene, has been a cornerstone in number theory and computer science for over two millennia. In this comprehensive guide, we’ll dive deep into the Sieve of Eratosthenes, exploring its history, implementation, optimization techniques, and practical applications in modern programming.
1. Understanding Prime Numbers
Before we delve into the Sieve of Eratosthenes, it’s crucial to have a solid understanding of prime numbers. Prime numbers are the building blocks of mathematics and play a significant role in various fields, including cryptography, computer science, and number theory.
1.1 Definition of Prime Numbers
A prime number is a natural number greater than 1 that is only divisible by 1 and itself. In other words, it has exactly two factors: 1 and the number itself. For example, 2, 3, 5, 7, 11, and 13 are prime numbers.
1.2 Importance of Prime Numbers
Prime numbers are essential in various areas of mathematics and computer science:
- Cryptography: Many encryption algorithms rely on the properties of prime numbers.
- Number Theory: Prime numbers are fundamental in understanding the structure of integers.
- Computer Science: Prime numbers are used in hash functions, pseudorandom number generators, and other algorithms.
- Data Compression: Some compression algorithms utilize properties of prime numbers.
2. The Sieve of Eratosthenes: An Overview
The Sieve of Eratosthenes is an ancient algorithm for finding all prime numbers up to a given limit. It was developed by Eratosthenes, a Greek mathematician who lived in the 3rd century BCE. The algorithm is remarkably simple yet powerful, making it an excellent introduction to algorithmic thinking for beginners in computer science.
2.1 How the Sieve Works
The basic idea behind the Sieve of Eratosthenes is to iteratively mark the multiples of each prime number as composite (not prime), starting from the smallest prime number, 2. The algorithm follows these steps:
- Create a list of consecutive integers from 2 to n (the upper limit).
- Start with the smallest prime number, p = 2.
- Mark all multiples of p (excluding p itself) as composite.
- Find the next unmarked number after p and set it as the new p.
- Repeat steps 3 and 4 until p^2 is greater than n.
- All remaining unmarked numbers are prime.
2.2 Visual Representation
To better understand how the Sieve of Eratosthenes works, let’s visualize the process for finding prime numbers up to 30:
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
Step 1: Mark multiples of 2 (in bold)
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
Step 2: Mark multiples of 3 (in bold)
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
Step 3: Mark multiples of 5 (in bold)
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
Step 4: Mark multiples of 7 (in bold)
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
Final result: Prime numbers up to 30
2 3 5 7 11 13 17 19 23 29
3. Implementing the Sieve of Eratosthenes
Now that we understand how the Sieve of Eratosthenes works, let’s implement it in Python. This implementation will help us grasp the algorithm’s logic and serve as a foundation for further optimizations.
3.1 Basic Implementation in Python
def sieve_of_eratosthenes(n):
# Create a boolean array "is_prime[0..n]" and initialize
# all entries it as true. A value in is_prime[i] will
# finally be false if i is Not a prime, else true.
is_prime = [True] * (n + 1)
is_prime[0] = is_prime[1] = False
for i in range(2, int(n**0.5) + 1):
if is_prime[i]:
# Update all multiples of i
for j in range(i*i, n+1, i):
is_prime[j] = False
# Collect all prime numbers
primes = [i for i in range(2, n+1) if is_prime[i]]
return primes
# Example usage
limit = 30
result = sieve_of_eratosthenes(limit)
print(f"Prime numbers up to {limit}: {result}")
This implementation creates a boolean array is_prime
to keep track of prime numbers. It then iterates through the numbers, marking multiples of each prime as composite. Finally, it collects all the remaining prime numbers into a list.
3.2 Time and Space Complexity
The time complexity of the Sieve of Eratosthenes is O(n log log n), where n is the upper limit. This makes it one of the most efficient algorithms for finding all prime numbers up to a given limit. The space complexity is O(n) due to the boolean array used to mark prime numbers.
4. Optimizing the Sieve of Eratosthenes
While the basic implementation of the Sieve of Eratosthenes is already efficient, there are several optimizations we can apply to make it even faster and more memory-efficient.
4.1 Optimization Techniques
- Odd Numbers Only: We can skip even numbers (except 2) since they are not prime.
- Bitset Implementation: Using a bitset instead of a boolean array can significantly reduce memory usage.
- Wheel Factorization: This technique allows us to skip more composite numbers.
- Segmented Sieve: Useful for finding primes in a specific range or when dealing with large numbers.
4.2 Implementing the Odd Numbers Optimization
Let’s implement the odd numbers optimization in Python:
def optimized_sieve(n):
if n < 2:
return []
# Initialize with 2 and odd numbers
is_prime = [True] * ((n // 2) + 1)
is_prime[0] = False # 1 is not prime
for i in range(3, int(n**0.5) + 1, 2):
if is_prime[i // 2]:
# Mark odd multiples of i as composite
for j in range(i * i, n + 1, 2 * i):
is_prime[j // 2] = False
# Collect prime numbers
primes = [2] + [2*i + 1 for i in range(1, (n // 2) + 1) if is_prime[i]]
return primes
# Example usage
limit = 30
result = optimized_sieve(limit)
print(f"Prime numbers up to {limit}: {result}")
This optimized version reduces memory usage by roughly half and performs fewer iterations, making it more efficient for larger numbers.
5. Applications of the Sieve of Eratosthenes
The Sieve of Eratosthenes has numerous practical applications in computer science and mathematics. Let’s explore some of these applications and see how they relate to real-world problems.
5.1 Cryptography
Prime numbers are fundamental to many cryptographic algorithms, especially in public-key cryptography. The Sieve of Eratosthenes can be used to generate prime numbers for:
- RSA algorithm: Requires large prime numbers for key generation.
- Diffie-Hellman key exchange: Uses prime numbers in its mathematical operations.
- Primality testing: As a first step in more advanced primality tests.
5.2 Number Theory Research
Mathematicians and researchers use the Sieve of Eratosthenes to study the distribution of prime numbers and test various conjectures in number theory, such as:
- Goldbach’s Conjecture: Every even integer greater than 2 can be expressed as the sum of two primes.
- Twin Prime Conjecture: There are infinitely many pairs of primes that differ by 2.
5.3 Computer Science Education
The Sieve of Eratosthenes is an excellent algorithm for teaching fundamental concepts in computer science:
- Algorithm design and analysis
- Time and space complexity
- Optimization techniques
- Data structures (arrays, bitsets)
5.4 Project Euler and Competitive Programming
Many programming challenges and competitions involve problems related to prime numbers. The Sieve of Eratosthenes is often a crucial tool for solving these problems efficiently.
6. Advanced Variations of the Sieve
As we delve deeper into the world of prime number generation, we encounter more advanced variations of the Sieve of Eratosthenes. These variations are designed to handle specific scenarios or to improve performance for certain ranges of numbers.
6.1 Segmented Sieve
The Segmented Sieve is a variation of the Sieve of Eratosthenes that allows us to find prime numbers in a specific range [L, R] or to handle very large numbers that might not fit in memory. The basic idea is to divide the range into smaller segments and apply the sieve to each segment.
def segmented_sieve(L, R):
# Find primes up to sqrt(R)
limit = int(R**0.5)
primes = optimized_sieve(limit)
# Initialize segment
is_prime = [True] * (R - L + 1)
# Mark composites in the current range
for prime in primes:
start = max(prime * prime, (L + prime - 1) // prime * prime)
for i in range(start, R + 1, prime):
is_prime[i - L] = False
# Collect primes in the range
return [i + L for i in range(R - L + 1) if is_prime[i] and i + L >= 2]
# Example usage
L, R = 100, 150
result = segmented_sieve(L, R)
print(f"Prime numbers between {L} and {R}: {result}")
The Segmented Sieve is particularly useful when dealing with large ranges or when memory is a constraint.
6.2 Wheel Factorization
Wheel Factorization is an optimization technique that extends the idea of skipping even numbers. It creates a “wheel” of numbers to skip, based on the first few prime numbers. This technique can significantly reduce the number of operations required, especially for larger numbers.
Here’s a simple example of wheel factorization using the first prime number (2):
def wheel_sieve(n):
if n < 2:
return []
# Initialize with 2 and odd numbers
is_prime = [True] * ((n - 1) // 2)
for i in range(3, int(n**0.5) + 1, 2):
if is_prime[(i - 1) // 2]:
for j in range(i * i, n + 1, 2 * i):
is_prime[(j - 1) // 2] = False
# Collect prime numbers
primes = [2] + [2*i + 1 for i in range(len(is_prime)) if is_prime[i]]
return primes
# Example usage
limit = 30
result = wheel_sieve(limit)
print(f"Prime numbers up to {limit}: {result}")
This implementation uses a wheel of size 2, skipping all even numbers except 2. More advanced implementations can use larger wheels based on the first 3, 5, or even more prime numbers, further improving efficiency.
7. Practical Considerations and Limitations
While the Sieve of Eratosthenes is an powerful algorithm, it’s important to understand its practical considerations and limitations when applying it to real-world problems.
7.1 Memory Usage
The primary limitation of the Sieve of Eratosthenes is its memory usage. For large values of n, the memory required to store the boolean array can become prohibitive. This is where optimizations like the Segmented Sieve become crucial.
7.2 Upper Limit on Input Size
Most implementations of the Sieve of Eratosthenes have an upper limit on the input size, typically around 10^8 to 10^9, depending on the available memory and the specific implementation. For finding primes beyond this range, more advanced techniques like the quadratic sieve or the general number field sieve are used.
7.3 Time Complexity for Specific Queries
While the Sieve of Eratosthenes is efficient for generating all primes up to a given number, it may not be the best choice for specific queries, such as checking if a single large number is prime or finding the nth prime number. In these cases, other algorithms like the Miller-Rabin primality test or probabilistic prime-counting functions might be more appropriate.
8. Conclusion and Further Learning
The Sieve of Eratosthenes is a fascinating algorithm that has stood the test of time. Its simplicity, efficiency, and the fundamental nature of prime numbers make it an essential topic in computer science education and a valuable tool in various applications.
As you continue your journey in algorithm design and implementation, consider exploring these related topics:
- Advanced primality testing algorithms (Miller-Rabin, AKS)
- Prime factorization techniques
- Applications of prime numbers in cryptography
- Number-theoretic algorithms and their implementations
Remember, mastering algorithms like the Sieve of Eratosthenes not only enhances your problem-solving skills but also provides valuable insights into efficient programming techniques. As you prepare for technical interviews or work on complex software projects, the principles learned from studying and implementing the Sieve of Eratosthenes will serve as a solid foundation for tackling more advanced algorithmic challenges.
Keep practicing, experimenting with different optimizations, and applying these concepts to real-world problems. The world of algorithms is vast and exciting, and the Sieve of Eratosthenes is just the beginning of your journey into the fascinating realm of computational mathematics and computer science.