Mastering Dijkstra’s Algorithm: A Comprehensive Guide for Aspiring Programmers
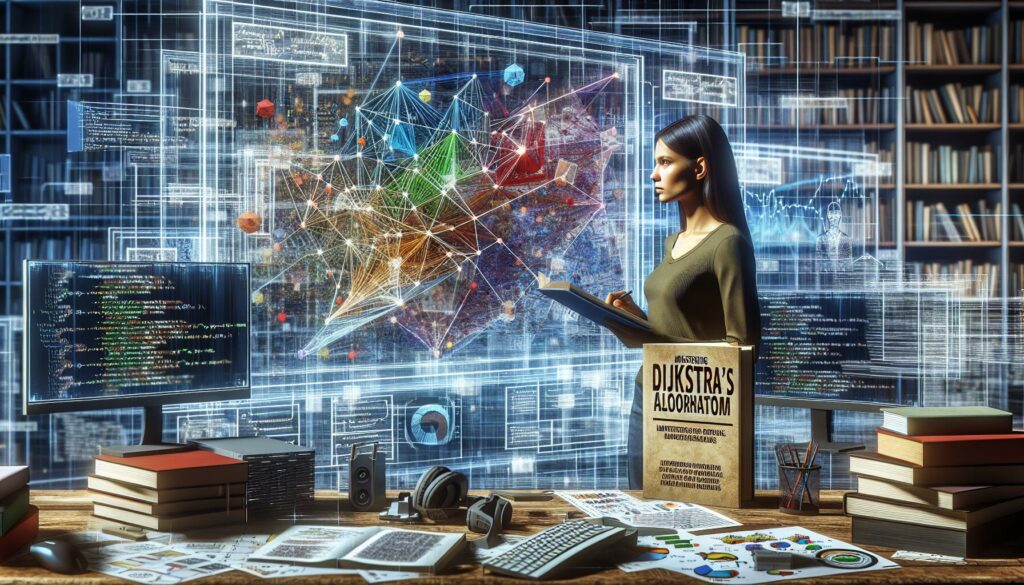
In the world of computer science and algorithmic problem-solving, few algorithms are as fundamental and widely applicable as Dijkstra’s algorithm. Named after its creator, Dutch computer scientist Edsger W. Dijkstra, this powerful algorithm has become a cornerstone in the field of graph theory and is essential knowledge for any aspiring programmer or computer scientist. In this comprehensive guide, we’ll dive deep into Dijkstra’s algorithm, exploring its principles, implementation, and practical applications.
Table of Contents
- Introduction to Dijkstra’s Algorithm
- How Dijkstra’s Algorithm Works
- Implementing Dijkstra’s Algorithm
- Time Complexity Analysis
- Real-world Applications
- Variations and Optimizations
- Comparison with Other Shortest Path Algorithms
- Practice Problems and Exercises
- Conclusion
1. Introduction to Dijkstra’s Algorithm
Dijkstra’s algorithm is a graph traversal algorithm used to find the shortest path between a starting node and all other nodes in a weighted graph. It was conceived by Edsger W. Dijkstra in 1956 and published three years later. The algorithm is particularly useful in routing and navigation systems, network routing protocols, and as a subroutine in other graph algorithms.
At its core, Dijkstra’s algorithm maintains a set of visited vertices and a set of unvisited vertices. It iteratively selects the unvisited vertex with the smallest tentative distance, visits it, and updates the tentative distances to all its unvisited neighbors. This process continues until the destination is reached or all vertices are visited.
2. How Dijkstra’s Algorithm Works
To understand Dijkstra’s algorithm, let’s break it down into steps:
- Initialization:
- Set the distance to the starting node to 0.
- Set the distances to all other nodes to infinity.
- Mark all nodes as unvisited.
- Selection: Select the unvisited node with the smallest known distance.
- Relaxation: For the current node, consider all its unvisited neighbors and calculate their tentative distances through the current node. Compare the newly calculated tentative distance to the current assigned value and assign the smaller one.
- Marking: After considering all neighbors, mark the current node as visited.
- Repeat: If the destination node has been marked visited or if the smallest tentative distance among the unvisited nodes is infinity (indicating that there’s no connection between the initial node and remaining unvisited nodes), then stop. Otherwise, repeat from step 2.
This process ensures that the algorithm always chooses the most promising path to explore next, gradually building up the shortest path to each node from the starting point.
3. Implementing Dijkstra’s Algorithm
Let’s implement Dijkstra’s algorithm in Python. We’ll use a priority queue to efficiently select the node with the smallest tentative distance at each step.
import heapq
def dijkstra(graph, start, end):
# Initialize distances and predecessors
distances = {node: float('infinity') for node in graph}
distances[start] = 0
predecessors = {node: None for node in graph}
# Priority queue to store vertices to visit
pq = [(0, start)]
while pq:
current_distance, current_node = heapq.heappop(pq)
# If we have reached the end node, we can stop
if current_node == end:
path = []
while current_node is not None:
path.append(current_node)
current_node = predecessors[current_node]
return distances[end], path[::-1]
# If we've found a longer path, skip
if current_distance > distances[current_node]:
continue
# Check all neighbors of the current node
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
# If we've found a shorter path, update and add to queue
if distance < distances[neighbor]:
distances[neighbor] = distance
predecessors[neighbor] = current_node
heapq.heappush(pq, (distance, neighbor))
# If we get here, there's no path to the end node
return float('infinity'), []
# Example usage
graph = {
'A': {'B': 4, 'C': 2},
'B': {'D': 3, 'E': 1},
'C': {'B': 1, 'D': 5},
'D': {'E': 2},
'E': {}
}
distance, path = dijkstra(graph, 'A', 'E')
print(f"Shortest distance: {distance}")
print(f"Shortest path: {' -> '.join(path)}")
This implementation uses a dictionary to represent the graph, where each key is a node, and its value is another dictionary of its neighbors and the corresponding edge weights. The algorithm returns both the shortest distance and the path taken.
4. Time Complexity Analysis
The time complexity of Dijkstra’s algorithm depends on how it’s implemented, particularly on how the minimum distance vertex is selected in each iteration.
- Using an array: O(V^2), where V is the number of vertices. This is because we need to search through all vertices to find the minimum distance vertex in each iteration.
- Using a binary heap: O((V + E) log V), where E is the number of edges. This is the case in our implementation above, using Python’s heapq module.
- Using a Fibonacci heap: O(E + V log V), which is the best known theoretical performance for sparse graphs.
The space complexity is O(V), as we need to store the distance and predecessor for each vertex.
5. Real-world Applications
Dijkstra’s algorithm has numerous practical applications across various domains:
- GPS and Navigation Systems: Finding the shortest or fastest route between two locations.
- Network Routing Protocols: Used in routing protocols like OSPF (Open Shortest Path First) to determine the best path for data packets.
- Social Networks: Finding the shortest connection between two users in a social graph.
- Robotics: Path planning for robots to navigate through complex environments.
- Games: Pathfinding for NPCs (Non-Player Characters) in video games.
- Telecommunications: Routing calls through a network with minimal cost or delay.
6. Variations and Optimizations
While the basic Dijkstra’s algorithm is powerful, several variations and optimizations have been developed to address specific scenarios or improve performance:
- Bidirectional Dijkstra: Runs two simultaneous searches, one from the start and one from the goal, potentially finding the shortest path faster.
- A* Algorithm: An extension of Dijkstra’s algorithm that uses heuristics to guide the search towards the goal, often resulting in faster pathfinding.
- Dijkstra’s Algorithm with Fibonacci Heap: Offers better theoretical time complexity for sparse graphs.
- Delta-Stepping: A parallel variant of Dijkstra’s algorithm that can efficiently utilize multiple processors.
7. Comparison with Other Shortest Path Algorithms
While Dijkstra’s algorithm is widely used, it’s important to understand its strengths and limitations compared to other shortest path algorithms:
- Bellman-Ford Algorithm: Can handle negative edge weights, unlike Dijkstra’s, but has a worse time complexity of O(VE).
- Floyd-Warshall Algorithm: Finds shortest paths between all pairs of vertices in O(V^3) time, which is more efficient than running Dijkstra’s algorithm for every pair when the graph is dense.
- A* Algorithm: Often faster than Dijkstra’s for finding a path between two specific nodes, especially in spatial problems, due to its use of heuristics.
8. Practice Problems and Exercises
To truly master Dijkstra’s algorithm, practice is crucial. Here are some exercises and problems to help reinforce your understanding:
- Basic Implementation: Implement Dijkstra’s algorithm from scratch without using any library functions.
- Path Reconstruction: Modify the algorithm to not only find the shortest distance but also reconstruct the actual path taken.
- Multiple Destinations: Adapt the algorithm to find the shortest paths from a single source to multiple destinations efficiently.
- Real-world Graph: Apply Dijkstra’s algorithm to a real-world problem, such as finding the shortest route between two cities using actual road network data.
- Optimization Challenge: Implement and compare different variations of Dijkstra’s algorithm (e.g., with binary heap vs. Fibonacci heap) and analyze their performance on various graph types.
Here’s a sample problem to get you started:
"""
Problem: Cheapest Flights Within K Stops
There are n cities connected by some number of flights. You are given an array flights where
flights[i] = [from_i, to_i, price_i] indicates that there is a flight from city from_i to city to_i with cost price_i.
You are also given three integers src, dst, and k, return the cheapest price from src to dst with at most k stops.
If there is no such route, return -1.
Example:
Input: n = 3, flights = [[0,1,100],[1,2,100],[0,2,500]], src = 0, dst = 2, k = 1
Output: 200
Explanation: The cheapest price from city 0 to city 2 with at most 1 stop costs 200, as marked red in the picture.
Implement a solution using Dijkstra's algorithm.
"""
def findCheapestPrice(n, flights, src, dst, k):
# Your implementation here
pass
# Test the function
n = 3
flights = [[0,1,100],[1,2,100],[0,2,500]]
src = 0
dst = 2
k = 1
print(findCheapestPrice(n, flights, src, dst, k)) # Should output 200
This problem is a variation of the shortest path problem where we have an additional constraint on the number of stops. It’s an excellent exercise to adapt Dijkstra’s algorithm to handle such constraints.
9. Conclusion
Dijkstra’s algorithm is a fundamental tool in the arsenal of any programmer or computer scientist. Its elegance lies in its simplicity and effectiveness in solving the single-source shortest path problem. By mastering this algorithm, you’ll not only enhance your problem-solving skills but also gain insights into graph theory and algorithm design principles that are applicable across various domains in computer science.
As you continue your journey in programming and algorithm design, remember that understanding the underlying principles of algorithms like Dijkstra’s is crucial. It allows you to adapt and apply these concepts to solve complex real-world problems efficiently.
Keep practicing, exploring variations, and applying Dijkstra’s algorithm to different scenarios. With time and experience, you’ll develop an intuition for when and how to use this powerful tool effectively in your programming projects and technical interviews.
Happy coding, and may your paths always be the shortest!