Continuous Integration: Streamlining Software Development for Better Efficiency and Quality
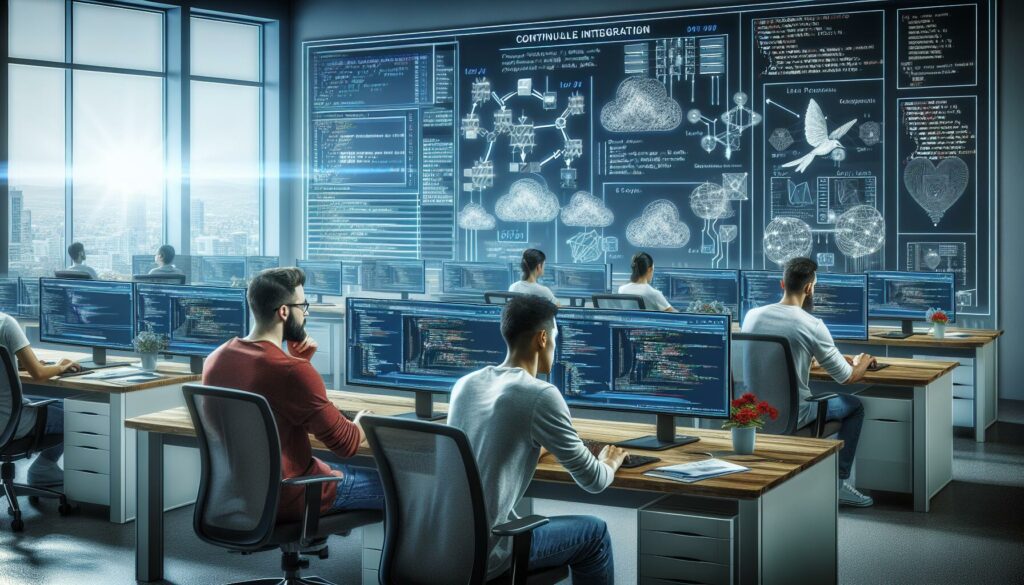
In the fast-paced world of software development, efficiency and quality are paramount. As projects grow in complexity and team sizes increase, it becomes increasingly challenging to maintain a smooth development process. This is where Continuous Integration (CI) comes into play, revolutionizing the way teams collaborate and deliver software. In this comprehensive guide, we’ll explore the concept of Continuous Integration, its benefits, and how it fits into the broader landscape of modern software development practices.
What is Continuous Integration?
Continuous Integration is a software development practice where developers regularly merge their code changes into a central repository, after which automated builds and tests are run. The primary goals of CI are to find and address bugs quicker, improve software quality, and reduce the time it takes to validate and release new software updates.
At its core, CI encourages developers to integrate their work frequently, usually daily, leading to multiple integrations per day. Each integration is verified by an automated build and automated tests to detect integration errors as quickly as possible.
The CI Process
A typical CI process involves the following steps:
- Code Commit: Developers write code and commit their changes to a shared repository.
- Build Triggering: The CI server detects the new commit and triggers a build.
- Code Compilation: The code is compiled, and any compilation errors are reported.
- Unit Testing: Automated unit tests are run to check individual components.
- Integration Testing: Tests are performed to ensure different parts of the application work together.
- Reporting: The CI server generates reports on the build and test results.
- Feedback: Developers receive immediate feedback on their changes.
Benefits of Continuous Integration
Implementing CI in your development workflow offers numerous advantages:
1. Early Bug Detection
By integrating code frequently and running automated tests, bugs are caught early in the development process. This makes them easier and less expensive to fix.
2. Improved Code Quality
Regular integration and testing encourage developers to write better, more modular code. It also helps maintain consistent coding standards across the team.
3. Faster Development Cycles
CI reduces the time spent on integrating code and fixing conflicts, allowing teams to develop and release features more quickly.
4. Increased Visibility
CI provides real-time information about the state of the software, making it easier for team members and stakeholders to stay informed about project progress.
5. Reduced Risk
By catching and fixing issues early, CI reduces the risk of major problems arising late in the development cycle or after release.
Implementing Continuous Integration
To successfully implement CI in your development process, consider the following steps:
1. Choose a CI Server
Select a CI server that fits your needs. Popular options include Jenkins, Travis CI, CircleCI, and GitLab CI/CD. These tools automate the integration process and provide a centralized platform for managing builds and tests.
2. Set Up Version Control
Use a version control system like Git to manage your codebase. This allows multiple developers to work on the same project simultaneously and facilitates easy integration of changes.
3. Automate the Build Process
Create scripts to automate the build process, including compiling code, running tests, and generating reports. This ensures consistency and reduces manual errors.
4. Implement Automated Testing
Develop a comprehensive suite of automated tests, including unit tests, integration tests, and end-to-end tests. These tests should cover as much of your codebase as possible to catch potential issues.
5. Configure Notifications
Set up notifications to alert team members about build statuses, test results, and any issues that arise during the CI process.
Best Practices for Continuous Integration
To get the most out of your CI implementation, follow these best practices:
1. Commit Code Frequently
Encourage developers to commit their changes at least once a day. This reduces the likelihood of integration conflicts and makes it easier to identify and fix issues.
2. Maintain a Single Source Repository
Keep all code and assets in a single repository to ensure everyone is working with the latest version and to simplify the integration process.
3. Automate Deployment
Extend your CI pipeline to include automated deployment to staging or production environments. This practice, known as Continuous Deployment (CD), further streamlines the development process.
4. Keep the Build Fast
Optimize your build and test processes to run as quickly as possible. Aim for a build time of less than 10 minutes to maintain developer productivity.
5. Test in a Clone of the Production Environment
Ensure your CI environment closely mirrors your production environment to catch environment-specific issues early.
Continuous Integration Tools and Technologies
There are numerous tools available to support CI practices. Here are some popular options:
1. Jenkins
Jenkins is an open-source automation server that supports building, deploying, and automating any project. It offers a wide range of plugins and integrations, making it highly customizable.
2. Travis CI
Travis CI is a hosted CI service that integrates well with GitHub repositories. It’s known for its ease of use and support for multiple programming languages.
3. CircleCI
CircleCI is a cloud-based CI/CD platform that supports rapid software development and deployment. It offers parallelism to speed up build times and integrates with various cloud platforms.
4. GitLab CI/CD
GitLab CI/CD is part of the GitLab platform, offering a complete DevOps solution. It provides built-in CI/CD capabilities tightly integrated with GitLab’s version control system.
5. GitHub Actions
GitHub Actions is GitHub’s built-in CI/CD solution, allowing developers to automate their software workflows directly from their GitHub repositories.
Continuous Integration in Practice: A Simple Example
Let’s look at a basic example of how CI might work in practice using a simple Python project and GitHub Actions.
Imagine you have a Python project with a simple function to add two numbers:
# math_operations.py
def add(a, b):
return a + b
And a corresponding test file:
# test_math_operations.py
import unittest
from math_operations import add
class TestMathOperations(unittest.TestCase):
def test_add(self):
self.assertEqual(add(2, 3), 5)
self.assertEqual(add(-1, 1), 0)
self.assertEqual(add(0, 0), 0)
if __name__ == '__main__':
unittest.main()
To set up CI for this project using GitHub Actions, you would create a workflow file in your repository at .github/workflows/ci.yml
:
name: Python CI
on: [push]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: '3.x'
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install -r requirements.txt
- name: Run tests
run: python -m unittest discover tests
This workflow does the following:
- Triggers on every push to the repository
- Sets up a Python environment
- Installs any required dependencies
- Runs the unit tests
Now, every time you push changes to your repository, GitHub Actions will automatically run your tests and report the results. If a test fails, you’ll be notified immediately, allowing you to address the issue before it affects other parts of your project.
Continuous Integration and Coding Education
Understanding and implementing Continuous Integration is crucial for aspiring software developers, especially those preparing for technical interviews at major tech companies. Here’s how CI relates to coding education and skill development:
1. Best Practices
CI enforces many software development best practices, such as frequent code commits, automated testing, and maintaining a clean, buildable codebase. Learning these practices early can set students up for success in their future careers.
2. Real-world Scenarios
Many coding education platforms, including AlgoCademy, can incorporate CI concepts into their curriculum to provide students with real-world scenarios. This could involve setting up CI pipelines for projects or troubleshooting integration issues.
3. Collaboration Skills
CI encourages collaboration among team members. Learning to work with CI systems can help students develop the collaborative skills necessary for working in professional software development teams.
4. Problem-solving
Debugging integration issues and interpreting CI feedback requires strong problem-solving skills. These are the same skills that are crucial for succeeding in technical interviews and day-to-day development work.
5. Tool Familiarity
Exposure to CI tools and practices gives students familiarity with technologies they’re likely to encounter in professional settings, making them more attractive to potential employers.
Conclusion
Continuous Integration is more than just a development practice; it’s a fundamental shift in how we approach software development. By automating the integration process, catching bugs early, and promoting collaboration, CI significantly improves the efficiency and quality of software development.
For those on the path to becoming professional developers, understanding and implementing CI is invaluable. It not only enhances your technical skills but also prepares you for the collaborative, fast-paced nature of modern software development. Whether you’re just starting your coding journey or preparing for technical interviews at top tech companies, embracing Continuous Integration will give you a significant advantage in your career.
As you continue to develop your coding skills, consider incorporating CI practices into your projects. Experiment with different CI tools, automate your testing processes, and get comfortable with frequent integration. These skills will not only make you a better developer but also a more attractive candidate in the competitive tech job market.
Remember, Continuous Integration is just one piece of the modern development puzzle. It often goes hand in hand with practices like Continuous Delivery and Deployment, forming a comprehensive DevOps approach to software development. As you grow in your career, exploring these related concepts will further enhance your capabilities as a software developer.
Embrace the power of Continuous Integration, and watch as it transforms your development process, improves your code quality, and accelerates your path to becoming a skilled, professional developer ready to tackle the challenges of the tech industry.