Ultimate Guide to Performance Optimization Techniques: Boost Your System’s Speed and Efficiency
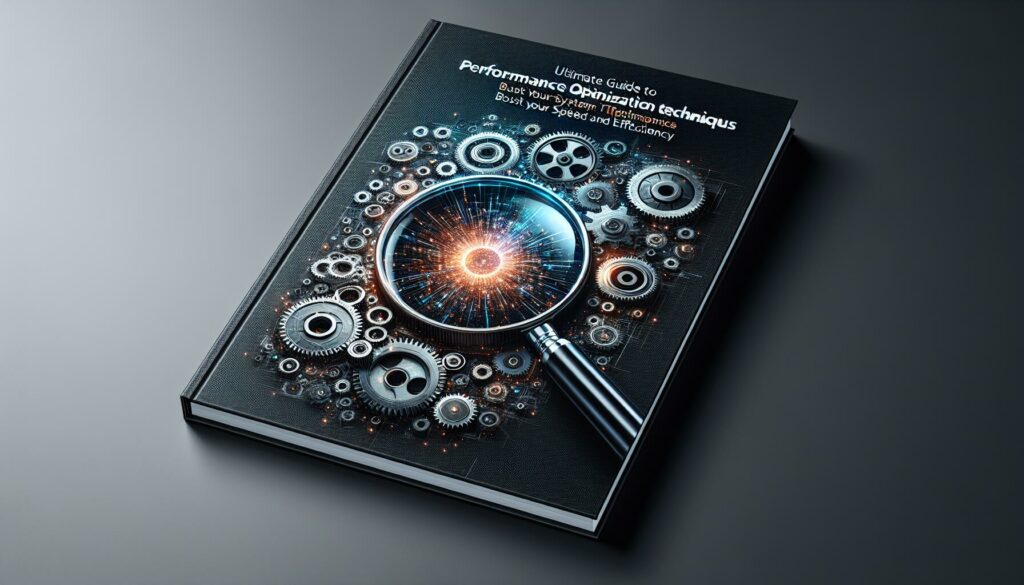
In today’s fast-paced digital world, performance optimization has become a critical aspect of software development and system management. Whether you’re a developer, system administrator, or simply a tech enthusiast, understanding and implementing performance optimization techniques can significantly enhance the speed, efficiency, and overall user experience of your applications and systems. This comprehensive guide will delve into various performance optimization strategies, covering everything from code-level improvements to system-wide enhancements.
1. Code-Level Optimization
The foundation of any high-performing application lies in its code. Here are some essential code-level optimization techniques:
1.1. Algorithmic Efficiency
Choosing the right algorithm for a given task can make a substantial difference in performance. Consider the following points:
- Time Complexity: Analyze the Big O notation of your algorithms and opt for those with lower time complexity when possible.
- Space Complexity: Balance between time and space efficiency, especially for memory-constrained environments.
- Data Structures: Use appropriate data structures that offer optimal performance for your specific use case (e.g., HashMaps for fast lookups).
1.2. Efficient Coding Practices
Adopting efficient coding practices can lead to significant performance improvements:
- Avoid Redundant Computations: Cache results of expensive operations for reuse.
- Minimize Loop Operations: Optimize loops by moving invariant computations outside the loop body.
- Use Appropriate Data Types: Choose the right data types to avoid unnecessary type conversions and memory usage.
- Implement Lazy Loading: Load resources only when needed to reduce initial load times.
1.3. Memory Management
Proper memory management is crucial for maintaining optimal performance:
- Garbage Collection: Understand and optimize garbage collection processes in managed languages.
- Memory Leaks: Regularly profile your application to identify and fix memory leaks.
- Object Pooling: Implement object pooling for frequently created and destroyed objects.
- Buffer Management: Use efficient buffer management techniques to minimize memory allocation and deallocation.
2. Database Optimization
For applications that rely heavily on databases, optimizing database operations is essential:
2.1. Query Optimization
- Indexing: Create appropriate indexes on frequently queried columns.
- Query Execution Plans: Analyze and optimize query execution plans.
- Avoid SELECT *: Only retrieve the columns you need to reduce data transfer and processing time.
- Use Prepared Statements: Leverage prepared statements to reduce query parsing overhead.
2.2. Database Design
- Normalization: Properly normalize your database schema to reduce redundancy and improve data integrity.
- Denormalization: Selectively denormalize when necessary to improve read performance.
- Partitioning: Implement table partitioning for large datasets to improve query performance.
2.3. Caching Strategies
- Result Caching: Cache frequently accessed query results to reduce database load.
- In-Memory Caching: Utilize in-memory caching solutions like Redis or Memcached for frequently accessed data.
- Query Caching: Enable and optimize database query caching mechanisms.
3. Front-End Optimization
Enhancing front-end performance is crucial for providing a smooth user experience:
3.1. Asset Optimization
- Minification: Minify CSS, JavaScript, and HTML files to reduce file sizes.
- Compression: Enable GZIP or Brotli compression for faster file transfers.
- Image Optimization: Compress and resize images, and use appropriate formats (e.g., WebP).
- Lazy Loading: Implement lazy loading for images and other non-critical resources.
3.2. Rendering Optimization
- Critical Rendering Path: Optimize the critical rendering path to improve initial load times.
- Asynchronous Loading: Use async and defer attributes for non-critical scripts.
- CSS Optimization: Minimize render-blocking CSS and inline critical styles.
- Virtual DOM: Utilize virtual DOM techniques (e.g., React) for efficient UI updates.
3.3. Network Optimization
- Content Delivery Networks (CDNs): Use CDNs to serve static assets from geographically distributed servers.
- HTTP/2 and HTTP/3: Leverage modern HTTP protocols for improved performance.
- Browser Caching: Implement effective browser caching strategies.
- Resource Hints: Use resource hints like preload, prefetch, and preconnect to optimize resource loading.
4. Back-End Optimization
Optimizing the back-end is crucial for handling high loads and ensuring scalability:
4.1. Caching Strategies
- Application-Level Caching: Implement caching at various levels of your application.
- Distributed Caching: Use distributed caching systems for improved scalability.
- Cache Invalidation: Implement efficient cache invalidation strategies to ensure data consistency.
4.2. Concurrency and Parallelism
- Asynchronous Programming: Utilize asynchronous programming models to improve I/O-bound operations.
- Multi-threading: Implement multi-threading for CPU-bound tasks.
- Task Queues: Use task queues for background processing and job scheduling.
4.3. Microservices Architecture
- Service Decomposition: Break down monolithic applications into microservices for better scalability.
- Load Balancing: Implement effective load balancing strategies across microservices.
- Service Mesh: Utilize service mesh technologies for improved communication between microservices.
5. Network Optimization
Optimizing network performance is crucial for distributed systems and web applications:
5.1. Protocol Optimization
- TCP Optimization: Fine-tune TCP parameters for optimal performance.
- UDP for Real-Time Applications: Consider UDP for real-time applications where some packet loss is acceptable.
- WebSocket for Real-Time Communication: Utilize WebSocket for efficient bidirectional communication.
5.2. Content Delivery
- Content Delivery Networks (CDNs): Leverage CDNs to reduce latency and improve content delivery speeds.
- Edge Computing: Utilize edge computing to process data closer to the source.
- Adaptive Bitrate Streaming: Implement adaptive bitrate streaming for video content.
5.3. Security Optimization
- SSL/TLS Optimization: Optimize SSL/TLS configurations for improved security and performance.
- DDoS Protection: Implement DDoS protection mechanisms to ensure availability.
- Web Application Firewalls (WAF): Utilize WAFs to protect against common web vulnerabilities.
6. Infrastructure Optimization
Optimizing the underlying infrastructure is essential for overall system performance:
6.1. Hardware Optimization
- CPU Optimization: Choose appropriate CPU architectures and optimize CPU usage.
- Memory Optimization: Implement efficient memory management and sizing.
- Storage Optimization: Utilize SSDs and optimize storage configurations.
- Network Hardware: Invest in high-performance network hardware.
6.2. Virtualization and Containerization
- Virtual Machines: Optimize virtual machine configurations for better resource utilization.
- Containers: Leverage containerization technologies like Docker for improved portability and resource efficiency.
- Orchestration: Use container orchestration platforms like Kubernetes for efficient management and scaling.
6.3. Cloud Optimization
- Auto-scaling: Implement auto-scaling to dynamically adjust resources based on demand.
- Serverless Computing: Utilize serverless computing for certain workloads to reduce operational overhead.
- Cloud-Native Services: Leverage cloud-native services for improved performance and scalability.
7. Monitoring and Profiling
Continuous monitoring and profiling are essential for identifying and addressing performance issues:
7.1. Application Performance Monitoring (APM)
- Real-Time Monitoring: Implement real-time monitoring of application performance metrics.
- Distributed Tracing: Utilize distributed tracing to identify bottlenecks in microservices architectures.
- Error Tracking: Implement robust error tracking and logging mechanisms.
7.2. Profiling Tools
- Code Profilers: Use code profilers to identify performance hotspots in your application.
- Memory Profilers: Utilize memory profilers to detect memory leaks and optimize memory usage.
- Database Profilers: Employ database profilers to optimize query performance.
7.3. Load Testing
- Stress Testing: Conduct stress tests to identify system breaking points.
- Performance Testing: Regularly perform performance tests to ensure system stability under various loads.
- Capacity Planning: Use load testing results for effective capacity planning.
8. Optimization for Mobile Devices
With the increasing prevalence of mobile devices, optimizing for mobile is crucial:
8.1. Responsive Design
- Fluid Layouts: Implement fluid layouts that adapt to different screen sizes.
- Mobile-First Approach: Adopt a mobile-first approach to ensure optimal performance on smaller devices.
- Touch-Friendly Interfaces: Design interfaces that are touch-friendly and easy to navigate on mobile devices.
8.2. Mobile Network Optimization
- Minimize Payload: Reduce the size of resources sent over mobile networks.
- Offline Capabilities: Implement offline capabilities using technologies like Service Workers.
- Progressive Loading: Implement progressive loading techniques to improve perceived performance.
8.3. Mobile-Specific Optimizations
- Battery Usage Optimization: Optimize applications to minimize battery drain.
- Memory Management: Implement efficient memory management techniques for memory-constrained devices.
- Native vs. Hybrid: Choose between native and hybrid app development based on performance requirements.
9. Continuous Optimization
Performance optimization is an ongoing process that requires continuous attention:
9.1. Performance Budgets
- Set Performance Targets: Establish clear performance targets and budgets.
- Automated Monitoring: Implement automated monitoring to ensure adherence to performance budgets.
- Regular Audits: Conduct regular performance audits to identify areas for improvement.
9.2. A/B Testing
- Performance Experiments: Conduct A/B tests to evaluate the impact of performance optimizations.
- User Feedback: Collect and analyze user feedback to identify performance issues.
- Iterative Improvements: Implement iterative improvements based on test results and feedback.
9.3. Stay Updated
- Technology Trends: Stay informed about the latest performance optimization techniques and technologies.
- Best Practices: Regularly review and update your optimization strategies based on industry best practices.
- Continuous Learning: Invest in continuous learning and skill development for your team.
Conclusion
Performance optimization is a multifaceted discipline that requires a holistic approach. By implementing the techniques discussed in this guide, you can significantly enhance the speed, efficiency, and user experience of your applications and systems. Remember that optimization is an ongoing process, and staying up-to-date with the latest trends and best practices is crucial for maintaining peak performance in an ever-evolving technological landscape.
As you embark on your performance optimization journey, keep in mind that the key to success lies in a balanced approach. Not every optimization technique will be applicable or beneficial in every scenario. It’s essential to analyze your specific use case, measure the impact of each optimization, and focus on the areas that provide the most significant improvements for your users and your business.
By making performance optimization an integral part of your development and operational processes, you’ll be well-equipped to deliver fast, efficient, and scalable solutions that meet the demands of today’s digital world. Remember, in the realm of technology, performance isn’t just about speed—it’s about creating seamless, responsive, and enjoyable experiences for your users.