Mastering For Loops: From Novice to Expert
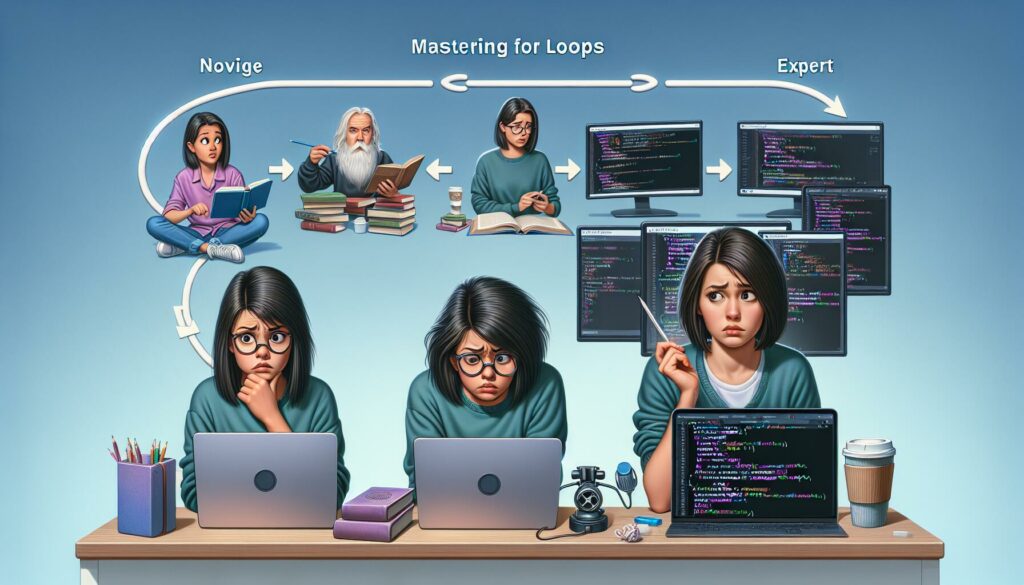
Introduction
For loops are a fundamental construct in programming, serving as a cornerstone for iterative processes across various languages. While they might seem simple at first glance, mastering for loops can significantly enhance your coding skills and problem-solving abilities. This comprehensive guide will take you on a journey from the basics of for loops to advanced techniques, common pitfalls, and best practices.
The Anatomy of a For Loop
Let’s start by breaking down the structure of a for loop:
for (initialization; condition; iteration) {
// Code to be executed
}
- Initialization: This part is executed once at the beginning of the loop. It’s typically used to declare and initialize a counter variable.
- Condition: Evaluated before each iteration of the loop. If true, the loop continues; if false, it terminates.
- Iteration: Executed at the end of each loop iteration, usually to update the counter variable.
From While to For: Understanding the Transformation
To truly grasp the concept of for loops, it’s helpful to understand their relationship to while loops. Consider this while loop:
let i = 0;
while (i < 5) {
console.log(i);
i++;
}
This can be transformed into an equivalent for loop:
for (let i = 0; i < 5; i++) {
console.log(i);
}
The transformation consolidates the loop’s control elements (initialization, condition, and iteration) into a single line, making the code more concise and often more readable.
Basic For Loop Applications
Iterating Through Arrays
The most common use of for loops among beginners is array iteration:
const fruits = ['apple', 'banana', 'cherry'];
for (let i = 0; i < fruits.length; i++) {
console.log(fruits[i]);
}
This loop iterates through each element of the fruits
array, printing each fruit to the console.
Generating Sequences
For loops are excellent for generating numerical sequences:
for (let i = 1; i <= 10; i++) {
console.log(i);
}
This loop prints numbers from 1 to 10.
Advanced For Loop Techniques
While basic array iteration is useful, for loops are capable of much more. Let’s explore some advanced techniques.
Nested Loops
Nested loops are crucial for working with multi-dimensional data or solving complex problems. Here’s an example that generates a multiplication table:
for (let i = 1; i <= 10; i++) {
for (let j = 1; j <= 10; j++) {
console.log(`${i} x ${j} = ${i * j}`);
}
console.log('---');
}
This nested structure creates a 10×10 multiplication table. The outer loop (i
) represents rows, while the inner loop (j
) represents columns.
Iterating Over Object Properties
While not a traditional for loop, the for…in loop is used to iterate over object properties:
const person = { name: 'Alice', age: 30, city: 'New York' };
for (let key in person) {
console.log(`${key}: ${person[key]}`);
}
This loop iterates over each property of the person
object, printing both the key and its corresponding value.
Custom Iteration Steps
For loops aren’t limited to incrementing by 1. You can customize the step size:
// Count by 2s
for (let i = 0; i <= 20; i += 2) {
console.log(i);
}
// Count down by 3s
for (let i = 100; i > 0; i -= 3) {
console.log(i);
}
These examples demonstrate counting up by 2s and counting down by 3s, showcasing the flexibility of for loops in generating various sequences.
Multiple Counters
You can use multiple counters within a single for loop:
for (let i = 0, j = 10; i < 10; i++, j--) {
console.log(i, j);
}
This loop simultaneously increments i
and decrements j
, demonstrating how multiple variables can be managed within a single loop structure.
Infinite Loops (Use with Caution)
While generally avoided, infinite loops can be useful in specific scenarios, such as game loops or continuous monitoring systems:
for (;;) {
// Code to be executed indefinitely
// Make sure to include a break condition!
if (someCondition) break;
}
Be cautious with infinite loops and ensure there’s a way to terminate them to avoid program hangs.
Common Pitfalls and How to Avoid Them
As developers progress from beginners to more experienced coders, they often encounter several common issues with for loops. Understanding these pitfalls is crucial for writing efficient and bug-free code.
1. Off-by-One Errors
One of the most common mistakes is the “off-by-one” error, where the loop iterates one too many or one too few times.
// Incorrect: Prints 0 to 10 (11 numbers)
for (let i = 0; i <= 10; i++) {
console.log(i);
}
// Correct: Prints 0 to 9 (10 numbers)
for (let i = 0; i < 10; i++) {
console.log(i);
}
Always double-check your loop conditions, especially when working with array indices.
2. Infinite Loops
Forgetting to increment the loop variable or setting an incorrect condition can lead to infinite loops:
// Infinite loop: i is never incremented
for (let i = 0; i < 10; ) {
console.log(i);
}
Always ensure that your loop has a way to terminate.
3. Modifying Loop Variables Inside the Loop
Changing the loop variable within the loop body can lead to unexpected behavior:
// Unpredictable behavior
for (let i = 0; i < 10; i++) {
console.log(i);
i += 2; // Avoid this!
}
It’s generally best to avoid modifying the loop variable within the loop body unless you have a specific reason to do so.
4. Performance Issues with Nested Loops
Nested loops can quickly become performance bottlenecks, especially with large datasets:
for (let i = 0; i < 1000; i++) {
for (let j = 0; j < 1000; j++) {
// This will execute 1,000,000 times!
}
}
Be mindful of the number of iterations in nested loops and look for ways to optimize when working with large datasets.
Best Practices for Using For Loops
To write clean, efficient, and maintainable code, consider these best practices when working with for loops:
1. Use Meaningful Variable Names
Instead of single-letter variables like i
, j
, k
, use descriptive names when appropriate:
for (let studentIndex = 0; studentIndex < students.length; studentIndex++) {
// ...
}
2. Precalculate the Loop Limit
When iterating over an array, precalculate the length to avoid recalculating it in each iteration:
const len = array.length;
for (let i = 0; i < len; i++) {
// ...
}
3. Consider forEach for Simple Array Iterations
For simple array iterations, the forEach
method can be more readable:
fruits.forEach(fruit => console.log(fruit));
4. Use for…of for Iterating Over Iterable Objects
When you don’t need the index, for...of
provides a clean syntax for iterating over arrays and other iterable objects:
for (const fruit of fruits) {
console.log(fruit);
}
5. Break and Continue
Use break
to exit a loop early and continue
to skip to the next iteration when needed:
for (let i = 0; i < 10; i++) {
if (i === 5) break; // Exit loop when i is 5
if (i % 2 === 0) continue; // Skip even numbers
console.log(i);
}
Advanced For Loop Patterns
As you become more comfortable with for loops, you can leverage them for more complex tasks. Here are some advanced patterns:
1. Generating Combinations
Use nested loops to generate all possible combinations of elements:
const letters = ['A', 'B', 'C'];
const numbers = [1, 2, 3];
for (let i = 0; i < letters.length; i++) {
for (let j = 0; j < numbers.length; j++) {
console.log(letters[i] + numbers[j]);
}
}
This generates combinations like A1, A2, A3, B1, B2, B3, C1, C2, C3.
2. Flattening Nested Arrays
Use a for loop to flatten a nested array structure:
function flattenArray(arr) {
let flattened = [];
for (let i = 0; i < arr.length; i++) {
if (Array.isArray(arr[i])) {
flattened = flattened.concat(flattenArray(arr[i]));
} else {
flattened.push(arr[i]);
}
}
return flattened;
}
const nestedArray = [1, [2, 3, [4, 5]], 6, [7, 8]];
console.log(flattenArray(nestedArray)); // [1, 2, 3, 4, 5, 6, 7, 8]
This recursive function uses a for loop to traverse and flatten a nested array structure.
3. Implementing Sorting Algorithms
Many sorting algorithms rely heavily on for loops. Here’s a simple implementation of the bubble sort algorithm:
function bubbleSort(arr) {
const len = arr.length;
for (let i = 0; i < len; i++) {
for (let j = 0; j < len - 1 - i; j++) {
if (arr[j] > arr[j + 1]) {
// Swap elements
[arr[j], arr[j + 1]] = [arr[j + 1], arr[j]];
}
}
}
return arr;
}
console.log(bubbleSort([64, 34, 25, 12, 22, 11, 90]));
This implementation uses nested for loops to compare and swap adjacent elements until the array is sorted.
Exercises to Improve Your For Loop Skills
To truly master for loops, practice is key. Here are some exercises to help you improve:
- FizzBuzz: Write a program that prints numbers from 1 to 100. For multiples of 3, print “Fizz” instead of the number. For multiples of 5, print “Buzz”. For numbers which are multiples of both 3 and 5, print “FizzBuzz”.
- Pattern Printing: Use nested for loops to print various patterns, such as:
*
**
***
****
*****
- Prime Number Generator: Write a function that uses a for loop to generate all prime numbers up to a given limit.
- Matrix Multiplication: Implement a function that multiplies two matrices using for loops.
- String Manipulation: Write a function that reverses every word in a sentence using for loops.
Conclusion
For loops are a powerful tool in a programmer’s toolkit. From simple iterations to complex algorithmic implementations, mastering for loops can significantly enhance your problem-solving abilities and coding efficiency. Remember, the key to mastery is practice and experimentation. Don’t be afraid to tackle complex problems using for loops, and always look for ways to optimize and improve your code.
As you continue your programming journey, you’ll find that the versatility of for loops makes them indispensable in various scenarios. Whether you’re working on data processing, algorithmic challenges, or building complex applications, a solid understanding of for loops will serve you well.
Keep practicing, stay curious, and happy coding!