Deep Dive into Data Structures: Essential Knowledge for Coders
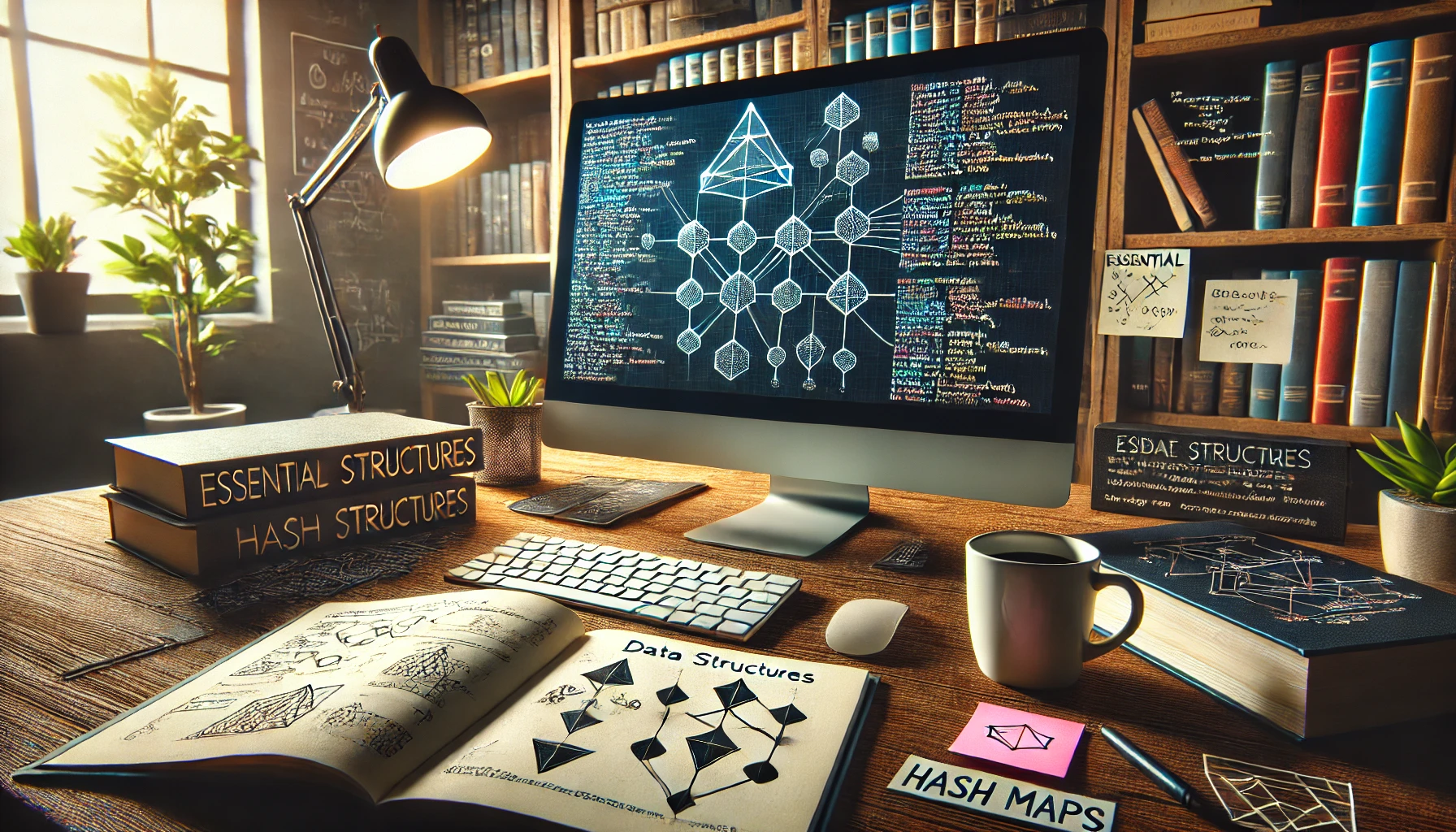
In the world of programming, data structures form the backbone of efficient and organized code. Whether you’re a beginner coder or preparing for technical interviews, a solid understanding of data structures is crucial. This blog post will explore key data structures, their applications, and why they’re essential for programming interviews. We’ll also provide examples and practical usage scenarios to help cement your understanding.
Table of Contents
- Introduction to Data Structures
- Arrays
- Linked Lists
- Stacks
- Queues
- Hash Tables
- Trees
- Graphs
- Heaps
- Why Data Structures Matter in Programming Interviews
- Conclusion
Introduction to Data Structures
Data structures are specialized formats for organizing, processing, retrieving, and storing data. They provide a way to manage large amounts of data efficiently for uses such as large databases and internet indexing services. The choice of the right data structure can significantly impact the performance and efficiency of an algorithm.
Arrays
Arrays are one of the most fundamental data structures in computer science. They store elements of the same type in contiguous memory locations.
Key Characteristics:
- Fixed size (in most languages)
- Constant-time access to elements by index
- Efficient for random access
Applications:
- Storing and accessing sequential data
- Temporarily storing objects
- Used as buffers for I/O operations
Example (Python):
# Creating an array
fruits = ["apple", "banana", "cherry", "date"]
# Accessing elements
print(fruits[1]) # Output: banana
# Modifying elements
fruits[2] = "grape"
print(fruits) # Output: ["apple", "banana", "grape", "date"]
Practical Usage:
Arrays are commonly used in image processing, where each pixel’s color values are stored in a 2D array. They’re also fundamental in implementing other data structures like stacks, queues, and hash tables.
Linked Lists
Linked lists consist of nodes, where each node contains a data field and a reference (or link) to the next node in the sequence.
Key Characteristics:
- Dynamic size
- Efficient insertion and deletion
- Non-contiguous memory allocation
Applications:
- Implementing stacks and queues
- Creating symbol tables in compiler design
- Managing memory allocation in operating systems
Example (Python):
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def append(self, data):
new_node = Node(data)
if not self.head:
self.head = new_node
return
last = self.head
while last.next:
last = last.next
last.next = new_node
# Usage
llist = LinkedList()
llist.append(1)
llist.append(2)
llist.append(3)
Practical Usage:
Linked lists are used in implementing file systems, where each block points to the next block. They’re also used in browser’s back and forward navigation, where each page points to the previous and next pages.
Stacks
Stacks follow the Last-In-First-Out (LIFO) principle. The last element added to the stack will be the first one to be removed.
Key Characteristics:
- LIFO data structure
- Push and pop operations
- Constant time complexity for push and pop
Applications:
- Function call management in programming languages
- Undo mechanisms in text editors
- Expression evaluation and syntax parsing
Example (Python):
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
return self.items.pop()
def peek(self):
return self.items[-1]
def is_empty(self):
return len(self.items) == 0
# Usage
stack = Stack()
stack.push(1)
stack.push(2)
stack.push(3)
print(stack.pop()) # Output: 3
Practical Usage:
Stacks are used in backtracking algorithms, such as finding the correct path in a maze or tree traversal algorithms. They’re also used in compilers to keep track of nested function calls.
Queues
Queues follow the First-In-First-Out (FIFO) principle. The first element added to the queue will be the first one to be removed.
Key Characteristics:
- FIFO data structure
- Enqueue and dequeue operations
- Constant time complexity for enqueue and dequeue
Applications:
- Task scheduling in operating systems
- Handling of interrupt requests in real-time systems
- Breadth-First Search algorithm in graphs
Example (Python):
from collections import deque
class Queue:
def __init__(self):
self.items = deque()
def enqueue(self, item):
self.items.append(item)
def dequeue(self):
return self.items.popleft()
def is_empty(self):
return len(self.items) == 0
# Usage
queue = Queue()
queue.enqueue(1)
queue.enqueue(2)
queue.enqueue(3)
print(queue.dequeue()) # Output: 1
Practical Usage:
Queues are used in printer spooling, where print jobs are processed in the order they are received. They’re also used in streaming media applications to manage buffering of data packets.
Hash Tables
Hash tables provide fast insertion, deletion, and lookup of key-value pairs using a hash function to compute an index into an array of buckets or slots.
Key Characteristics:
- Constant-time average case for insert, delete, and lookup
- Uses hash function to map keys to indices
- Handles collisions through techniques like chaining or open addressing
Applications:
- Implementing associative arrays
- Database indexing
- Caching mechanisms
Example (Python):
class HashTable:
def __init__(self, size):
self.size = size
self.table = [[] for _ in range(self.size)]
def _hash(self, key):
return hash(key) % self.size
def insert(self, key, value):
index = self._hash(key)
for item in self.table[index]:
if item[0] == key:
item[1] = value
return
self.table[index].append([key, value])
def get(self, key):
index = self._hash(key)
for item in self.table[index]:
if item[0] == key:
return item[1]
raise KeyError(key)
# Usage
ht = HashTable(10)
ht.insert("apple", 5)
ht.insert("banana", 7)
print(ht.get("apple")) # Output: 5
Practical Usage:
Hash tables are used in database indexing to speed up data retrieval. They’re also used in caching mechanisms, such as memoization in dynamic programming, to store previously computed results.
Trees
Trees are hierarchical data structures consisting of nodes connected by edges. They’re widely used for representing hierarchical relationships.
Key Characteristics:
- Hierarchical structure with a root node
- Each node can have multiple children
- Efficient for search, insert, and delete operations (for balanced trees)
Applications:
- File systems in operating systems
- XML/HTML document object models
- Implementing expression parsers and evaluators
Example (Python – Binary Search Tree):
class TreeNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
class BinarySearchTree:
def __init__(self):
self.root = None
def insert(self, value):
if not self.root:
self.root = TreeNode(value)
else:
self._insert_recursive(self.root, value)
def _insert_recursive(self, node, value):
if value < node.value:
if node.left is None:
node.left = TreeNode(value)
else:
self._insert_recursive(node.left, value)
else:
if node.right is None:
node.right = TreeNode(value)
else:
self._insert_recursive(node.right, value)
# Usage
bst = BinarySearchTree()
bst.insert(5)
bst.insert(3)
bst.insert(7)
Practical Usage:
Trees are used in implementing file systems, where directories can contain files and other directories. They’re also used in game development for decision trees in AI and in compilers for abstract syntax trees.
Graphs
Graphs consist of a set of vertices (or nodes) and a set of edges connecting these vertices. They’re used to represent networks and complex relationships between objects.
Key Characteristics:
- Vertices connected by edges
- Can be directed or undirected
- Can be weighted or unweighted
Applications:
- Social networks
- Routing algorithms
- Dependency resolution in build systems
Example (Python – Adjacency List):
class Graph:
def __init__(self):
self.graph = {}
def add_edge(self, u, v):
if u not in self.graph:
self.graph[u] = []
self.graph[u].append(v)
def print_graph(self):
for vertex in self.graph:
print(f"{vertex} -> {' '.join(map(str, self.graph[vertex]))}")
# Usage
g = Graph()
g.add_edge(0, 1)
g.add_edge(0, 2)
g.add_edge(1, 2)
g.add_edge(2, 0)
g.add_edge(2, 3)
g.print_graph()
Practical Usage:
Graphs are used in social network analysis to represent relationships between users. They’re also used in GPS navigation systems to find the shortest path between two locations.
Heaps
Heaps are special tree-based data structures that satisfy the heap property. In a max heap, for any given node I, the value of I is greater than or equal to the values of its children.
Key Characteristics:
- Complete binary tree
- Efficiently maintains the largest (or smallest) element at the root
- Constant time to retrieve the max/min element
- Logarithmic time for insertion and deletion
Applications:
- Priority queues
- Scheduling algorithms
- Heap sort algorithm
Example (Python – using heapq module):
import heapq
# Creating a min heap
min_heap = []
heapq.heappush(min_heap, 4)
heapq.heappush(min_heap, 1)
heapq.heappush(min_heap, 7)
heapq.heappush(min_heap, 3)
print(heapq.heappop(min_heap)) # Output: 1
# Creating a max heap (by negating the values)
max_heap = []
heapq.heappush(max_heap, -4)
heapq.heappush(max_heap, -1)
heapq.heappush(max_heap, -7)
heapq.heappush(max_heap, -3)
print(-heapq.heappop(max_heap)) # Output: 7
Practical Usage:
Heaps are used in operating systems for task scheduling, where processes with higher priority are executed first. They’re also used in Dijkstra’s algorithm for finding the shortest path in a graph.
Why Data Structures Matter in Programming Interviews
Understanding data structures is crucial for programming interviews for several reasons:
- Efficiency: Choosing the right data structure can significantly impact the time and space complexity of your algorithms. Interviewers often look for candidates who can optimize their solutions using appropriate data structures.
- Problem-solving skills: Many interview questions are designed to test your ability to choose and implement the most suitable data structure for a given problem.
- Fundamental knowledge: Data structures form the building blocks of more complex algorithms and systems. A strong grasp of data structures demonstrates a solid foundation in computer science principles.
- Real-world applications: Many real-world software systems rely heavily on efficient data structures. Understanding them helps you design and implement better solutions in your day-to-day work.
- Language agnostic: Data structures are conceptual and can be implemented in any programming language. This knowledge is transferable across different technologies and platforms.
Conclusion
Data structures are fundamental to computer science and software development. They provide efficient ways to organize and manipulate data, which is crucial for solving complex problems and optimizing algorithms. By understanding the characteristics, applications, and implementations of various data structures, you’ll be better equipped to tackle programming challenges and excel in technical interviews.
Remember, the key to mastering data structures is practice. Implement them from scratch, solve problems using them, and analyze their time and space complexities. With time and effort, you’ll develop a intuitive understanding of when and how to use each data structure effectively.
Keep exploring, keep coding, and never stop learning!