79 Essential Tips for Succeeding in Collaborative Coding Projects
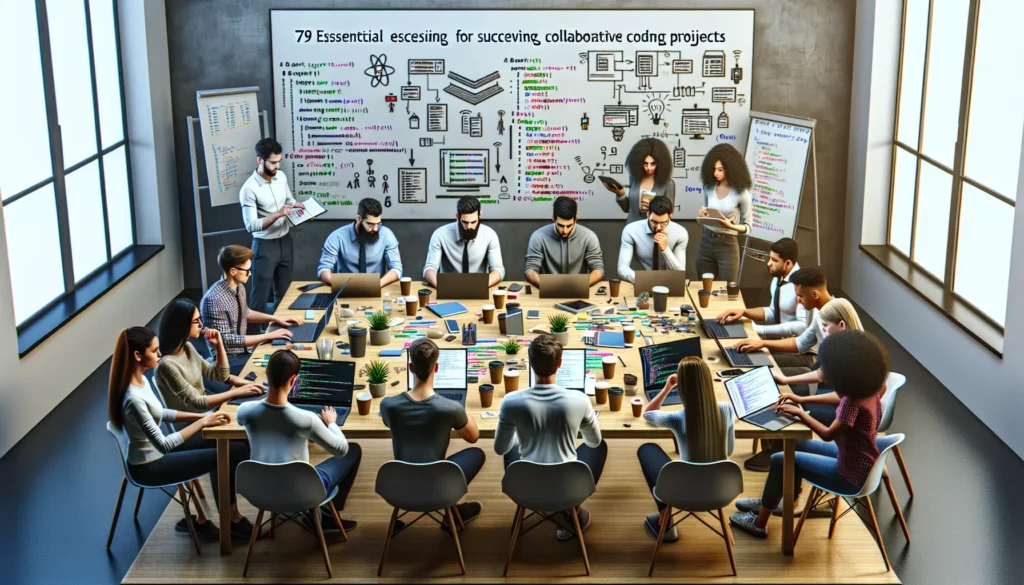
Collaborative coding projects are the backbone of modern software development. Whether you’re working on an open-source initiative, a team project at work, or a group assignment in your coding bootcamp, the ability to effectively collaborate with others is crucial for success. In this comprehensive guide, we’ll explore 79 essential tips to help you excel in collaborative coding environments, enhance your teamwork skills, and deliver high-quality software projects.
1. Communication is Key
Effective communication is the foundation of any successful collaborative project. Here are some tips to improve your communication skills:
- Use clear and concise language when discussing code or project details.
- Practice active listening to fully understand your teammates’ ideas and concerns.
- Be open to feedback and constructive criticism.
- Regularly update your team on your progress and any roadblocks you encounter.
- Use collaboration tools like Slack, Microsoft Teams, or Discord for real-time communication.
2. Version Control Best Practices
Version control systems like Git are essential for collaborative coding. Follow these tips to make the most of your version control workflow:
- Commit frequently with descriptive commit messages.
- Use branching strategies like Git Flow or GitHub Flow to organize your work.
- Keep your branches up-to-date with the main branch to avoid merge conflicts.
- Use pull requests for code reviews and discussions.
- Learn to resolve merge conflicts efficiently.
3. Code Review Etiquette
Code reviews are an important part of the collaborative coding process. Here are some tips for both reviewers and reviewees:
- Be respectful and constructive in your feedback.
- Focus on the code, not the person.
- Explain the reasoning behind your suggestions.
- Be open to alternative solutions and approaches.
- Use code review tools like GitHub’s Pull Request feature or Gerrit for a streamlined process.
4. Documentation and Comments
Good documentation and well-commented code are crucial for collaboration. Consider these tips:
- Write clear and concise comments that explain the “why” behind complex logic.
- Use inline documentation for functions and classes.
- Maintain a comprehensive README file for your project.
- Document your API endpoints and their expected inputs/outputs.
- Keep your documentation up-to-date as the project evolves.
5. Project Management and Organization
Effective project management is essential for successful collaboration. Here are some tips to keep your project organized:
- Use project management tools like Jira, Trello, or Asana to track tasks and progress.
- Break down large tasks into smaller, manageable subtasks.
- Set clear deadlines and milestones for your project.
- Regularly review and update your project roadmap.
- Assign roles and responsibilities clearly within your team.
6. Coding Standards and Style Guides
Consistent coding standards help maintain code quality and readability. Follow these tips:
- Agree on a coding style guide for your project (e.g., PEP 8 for Python).
- Use linters and code formatters to enforce coding standards automatically.
- Implement pre-commit hooks to catch style violations before they’re committed.
- Be consistent with naming conventions for variables, functions, and classes.
- Follow the principle of “Clean Code” by writing self-explanatory and modular code.
7. Testing and Quality Assurance
Robust testing practices ensure the reliability of your collaborative project. Consider these tips:
- Write unit tests for your code to catch bugs early.
- Implement integration tests to ensure different parts of your application work together.
- Use continuous integration (CI) tools like Jenkins or GitHub Actions to automate testing.
- Practice test-driven development (TDD) when appropriate.
- Conduct regular code reviews to catch potential issues and improve code quality.
8. Performance Optimization
Optimizing your code for performance is crucial in collaborative projects. Here are some tips:
- Use profiling tools to identify performance bottlenecks.
- Optimize database queries and indexing for better performance.
- Implement caching mechanisms where appropriate.
- Consider asynchronous programming techniques for I/O-bound operations.
- Regularly benchmark your application to track performance improvements or regressions.
9. Security Considerations
Security should be a top priority in any collaborative coding project. Follow these tips:
- Implement proper authentication and authorization mechanisms.
- Use encryption for sensitive data both in transit and at rest.
- Regularly update your dependencies to patch known vulnerabilities.
- Conduct security audits and penetration testing.
- Follow the principle of least privilege when designing your system architecture.
10. Continuous Learning and Improvement
Staying up-to-date with the latest technologies and best practices is essential. Consider these tips:
- Attend conferences, webinars, and workshops related to your tech stack.
- Read technical blogs and books to expand your knowledge.
- Participate in coding challenges and hackathons to sharpen your skills.
- Share your knowledge with your team through presentations or tech talks.
- Be open to learning new programming languages and frameworks.
11. Effective Code Reuse
Reusing code efficiently can save time and improve consistency. Here are some tips:
- Create reusable components and modules whenever possible.
- Use design patterns to solve common problems in a standardized way.
- Implement a package manager for your project to manage dependencies.
- Document how to use and extend your reusable code.
- Consider creating an internal library for commonly used functions across projects.
12. Debugging and Troubleshooting
Effective debugging skills are crucial in collaborative environments. Follow these tips:
- Use debugging tools and IDEs to step through code and identify issues.
- Write detailed bug reports with steps to reproduce the problem.
- Implement logging throughout your application for easier troubleshooting.
- Use error tracking tools like Sentry or Rollbar to monitor production issues.
- Practice rubber duck debugging by explaining the problem out loud.
13. Code Architecture and Design
A well-designed architecture is crucial for scalable and maintainable projects. Consider these tips:
- Follow SOLID principles in your object-oriented design.
- Use design patterns appropriately to solve common architectural challenges.
- Implement a modular architecture to improve code organization and reusability.
- Consider microservices architecture for large-scale applications.
- Regularly refactor your code to improve its structure and maintainability.
14. Effective Team Collaboration
Building a strong team dynamic is essential for successful collaboration. Here are some tips:
- Foster a culture of open communication and knowledge sharing.
- Conduct regular team retrospectives to identify areas for improvement.
- Pair programming can be an effective way to share knowledge and solve complex problems.
- Celebrate team successes and learn from failures together.
- Respect diverse perspectives and coding styles within your team.
15. Continuous Integration and Deployment (CI/CD)
Implementing CI/CD practices can streamline your development process. Consider these tips:
- Set up automated build and test processes for your project.
- Implement continuous deployment to automatically push changes to production.
- Use feature flags to safely roll out new features to a subset of users.
- Monitor your application’s performance and stability after each deployment.
- Implement a rollback strategy in case of critical issues in production.
16. Cross-functional Collaboration
Collaborating with non-technical team members is often necessary. Here are some tips:
- Learn to communicate technical concepts in non-technical terms.
- Work closely with product managers and designers to understand project requirements.
- Involve QA testers early in the development process to catch issues sooner.
- Collaborate with DevOps teams to ensure smooth deployment and operations.
Conclusion
Succeeding in collaborative coding projects requires a combination of technical skills, communication abilities, and a willingness to learn and adapt. By following these 79 tips, you’ll be well-equipped to tackle the challenges of collaborative development and contribute effectively to your team’s success.
Remember that collaboration is an ongoing process of improvement. Continuously seek feedback, stay open to new ideas, and always strive to enhance your skills. With practice and dedication, you’ll become an invaluable asset to any collaborative coding project.
Happy coding, and may your collaborative projects be successful and rewarding!