76. Practical Uses of Bloom Filters: Enhancing Efficiency in Modern Computing
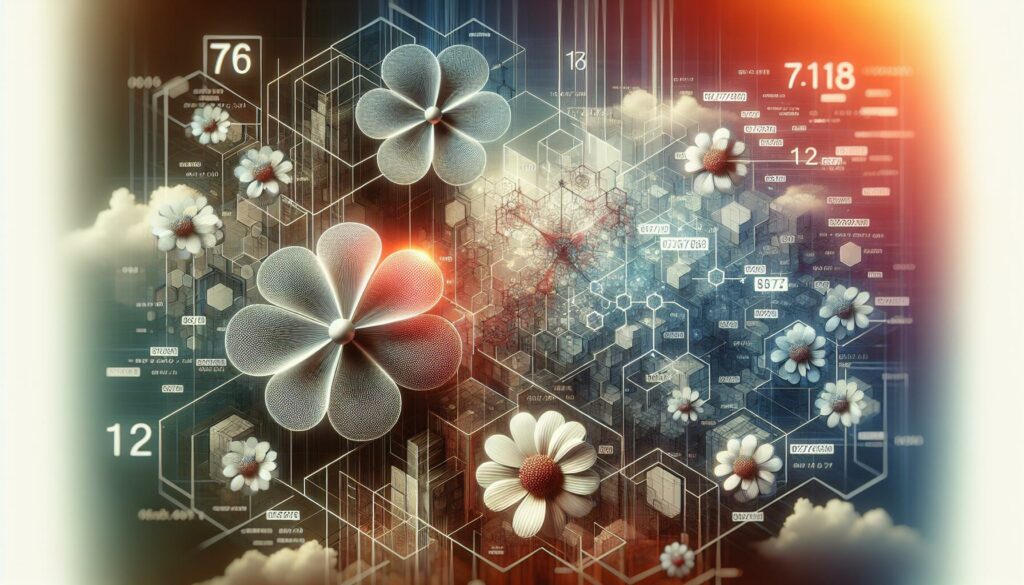
In the ever-evolving landscape of computer science and software engineering, efficiency is paramount. As datasets grow larger and systems become more complex, developers and engineers are constantly seeking innovative ways to optimize performance and resource utilization. One such powerful tool in the algorithmic toolkit is the Bloom filter. In this comprehensive guide, we’ll explore the practical applications of Bloom filters, understand their inner workings, and discover how they can significantly enhance various aspects of modern computing.
What is a Bloom Filter?
Before diving into the practical uses, let’s first understand what a Bloom filter is and how it works. A Bloom filter is a space-efficient probabilistic data structure designed to test whether an element is a member of a set. It was invented by Burton Howard Bloom in 1970 and has since found numerous applications in computer science and beyond.
The key characteristics of a Bloom filter are:
- Space efficiency: It uses a fixed amount of memory regardless of the number of elements in the set.
- Constant-time operations: Both adding elements and querying for membership take O(1) time.
- Probabilistic nature: It can have false positives but never false negatives.
How Does a Bloom Filter Work?
At its core, a Bloom filter consists of a bit array of m bits, initially all set to 0, and k different hash functions. Here’s a step-by-step explanation of its operation:
- To add an element, feed it to each of the k hash functions to get k array positions.
- Set the bits at all these positions to 1.
- To query for an element, feed it to each of the k hash functions to get k array positions.
- If any of the bits at these positions is 0, the element is definitely not in the set.
- If all are 1, then either the element is in the set, or we have a false positive.
Now that we understand the basics, let’s explore some practical applications of Bloom filters in various domains of computing.
1. Web Browsers: Malicious URL Checking
One of the most widely known applications of Bloom filters is in web browsers for quick malicious URL checking. Modern browsers like Google Chrome use Bloom filters to provide a first line of defense against phishing and malware sites.
How it works:
- The browser maintains a local Bloom filter containing hashes of known malicious URLs.
- When a user attempts to visit a website, the URL is checked against this filter.
- If the filter indicates a potential match, the browser then performs a full check against a comprehensive database.
This approach significantly reduces the number of full database lookups, improving browsing speed while maintaining security. The trade-off of potential false positives is acceptable here, as it only leads to an extra security check rather than blocking legitimate sites.
Implementation Example:
Here’s a simple Python implementation demonstrating how a Bloom filter might be used for URL checking:
import mmh3
import math
class BloomFilter:
def __init__(self, size, hash_count):
self.size = size
self.hash_count = hash_count
self.bit_array = [0] * size
def add(self, url):
for seed in range(self.hash_count):
result = mmh3.hash(url, seed) % self.size
self.bit_array[result] = 1
def check(self, url):
for seed in range(self.hash_count):
result = mmh3.hash(url, seed) % self.size
if self.bit_array[result] == 0:
return False
return True
# Usage
bf = BloomFilter(1000000, 5) # 1 million bits, 5 hash functions
# Add some malicious URLs
bf.add("http://malicious-site.com")
bf.add("http://phishing-attempt.net")
# Check URLs
print(bf.check("http://malicious-site.com")) # True
print(bf.check("http://safe-site.org")) # False (probably)
This example uses the MurmurHash3 algorithm (via the mmh3
library) for hashing, which is known for its speed and good distribution properties.
2. Database Systems: Query Optimization
In database management systems, Bloom filters can be used to optimize query performance, especially for join operations and distributed databases.
Use cases in databases:
- Join Optimization: Before performing expensive join operations, a Bloom filter can quickly eliminate rows that definitely won’t be in the join result.
- Distributed Databases: In systems like Cassandra or HBase, Bloom filters can reduce unnecessary disk reads and network traffic by quickly determining if a particular row exists in a specific node.
- Cache Management: Bloom filters can be used to determine if an item is likely to be in cache before performing a costly cache lookup.
Example: Join Optimization
Consider a scenario where we need to join two large tables: Orders
and Customers
. Instead of performing a full join, we can use a Bloom filter to optimize the process:
- Create a Bloom filter and add all unique
customer_id
values from theOrders
table. - Before joining, check each
customer_id
in theCustomers
table against the Bloom filter. - Only proceed with the join for customers that pass the Bloom filter check.
This approach can significantly reduce the number of unnecessary comparisons, especially when many customers in the Customers
table don’t have any orders.
3. Network Routers: Packet Filtering
In networking, Bloom filters can be employed for efficient packet filtering and routing. They’re particularly useful in scenarios where routers need to quickly decide whether to forward or drop packets based on certain criteria.
Applications in networking:
- IP Address Filtering: Quickly determine if an IP address is in a blocklist or allowlist.
- Content-Based Routing: Route packets based on their content without deep packet inspection.
- DDoS Protection: Identify and filter out malicious traffic patterns.
Example: IP Address Filtering
Here’s a simplified example of how a router might use a Bloom filter for IP address filtering:
class RouterBloomFilter:
def __init__(self, size, hash_count):
self.bloom_filter = BloomFilter(size, hash_count)
def add_blocked_ip(self, ip):
self.bloom_filter.add(ip)
def should_forward_packet(self, packet):
source_ip = packet.get_source_ip()
if self.bloom_filter.check(source_ip):
# Potentially blocked IP, perform full check
return self.full_ip_check(source_ip)
return True # Definitely not blocked
def full_ip_check(self, ip):
# Perform a full check against the actual blocklist
# This is a more expensive operation
pass
# Usage
router_bf = RouterBloomFilter(1000000, 5)
router_bf.add_blocked_ip("192.168.1.100")
router_bf.add_blocked_ip("10.0.0.1")
# Simulating packet routing
class Packet:
def __init__(self, source_ip):
self.source_ip = source_ip
def get_source_ip(self):
return self.source_ip
packet1 = Packet("192.168.1.100")
packet2 = Packet("172.16.0.1")
print(router_bf.should_forward_packet(packet1)) # False (probably)
print(router_bf.should_forward_packet(packet2)) # True
This example demonstrates how a router can quickly decide whether to forward a packet or perform a more thorough check based on the source IP address.
4. Spell Checkers: Fast Word Lookup
Spell checkers can benefit from Bloom filters to quickly determine if a word might be correctly spelled without needing to search through an entire dictionary.
How it enhances spell checking:
- Reduces memory usage compared to storing a full dictionary.
- Provides fast lookups, improving the responsiveness of spell-checking applications.
- Can be combined with other techniques for more accurate results.
Implementation Example:
class SpellChecker:
def __init__(self, dictionary, size=1000000, hash_count=5):
self.bloom_filter = BloomFilter(size, hash_count)
self.load_dictionary(dictionary)
def load_dictionary(self, dictionary):
for word in dictionary:
self.bloom_filter.add(word.lower())
def check_word(self, word):
return self.bloom_filter.check(word.lower())
# Usage
dictionary = ["apple", "banana", "cherry", "date", "elderberry"]
spell_checker = SpellChecker(dictionary)
print(spell_checker.check_word("apple")) # True
print(spell_checker.check_word("aple")) # False
print(spell_checker.check_word("banana")) # True
print(spell_checker.check_word("grape")) # False
This simple spell checker can quickly determine if a word is potentially correct. In a real-world application, this would be combined with other techniques to handle edge cases and provide suggestions for misspelled words.
5. Blockchain and Cryptocurrencies: Efficient Verification
In the world of blockchain and cryptocurrencies, Bloom filters play a crucial role in lightweight clients and efficient transaction verification.
Applications in blockchain:
- SPV (Simplified Payment Verification) Wallets: Allow light clients to verify transactions without downloading the entire blockchain.
- Transaction Filtering: Quickly check if a transaction is relevant to a particular wallet.
- Peer Discovery: Efficiently share information about which transactions and blocks a node has.
Example: SPV Wallet Transaction Verification
Here’s a simplified example of how an SPV wallet might use a Bloom filter to verify transactions:
class SPVWallet:
def __init__(self, addresses, size=1000000, hash_count=5):
self.bloom_filter = BloomFilter(size, hash_count)
for address in addresses:
self.bloom_filter.add(address)
def is_relevant_transaction(self, transaction):
for output in transaction.outputs:
if self.bloom_filter.check(output.address):
return True
return False
# Usage
wallet = SPVWallet(["1BvBMSEYstWetqTFn5Au4m4GFg7xJaNVN2", "3J98t1WpEZ73CNmQviecrnyiWrnqRhWNLy"])
class TransactionOutput:
def __init__(self, address):
self.address = address
class Transaction:
def __init__(self, outputs):
self.outputs = outputs
tx1 = Transaction([TransactionOutput("1BvBMSEYstWetqTFn5Au4m4GFg7xJaNVN2")])
tx2 = Transaction([TransactionOutput("1CounterpartyXXXXXXXXXXXXXXXUWLpVr")])
print(wallet.is_relevant_transaction(tx1)) # True
print(wallet.is_relevant_transaction(tx2)) # False
This example shows how an SPV wallet can quickly determine if a transaction is potentially relevant without needing to store or process the entire blockchain.
6. Caching Systems: Efficient Cache Management
Bloom filters can significantly enhance the performance of caching systems by reducing unnecessary cache lookups and optimizing memory usage.
Uses in caching:
- Cache Miss Optimization: Quickly determine if an item is definitely not in the cache.
- Cache Eviction Policies: Use Bloom filters to implement efficient least recently used (LRU) or least frequently used (LFU) policies.
- Distributed Caching: In a distributed cache, use Bloom filters to determine which node is likely to contain a particular item.
Example: Cache Miss Optimization
class BloomFilterCache:
def __init__(self, size=1000000, hash_count=5):
self.bloom_filter = BloomFilter(size, hash_count)
self.cache = {}
def add(self, key, value):
self.bloom_filter.add(key)
self.cache[key] = value
def get(self, key):
if not self.bloom_filter.check(key):
return None # Definitely not in cache
return self.cache.get(key) # May or may not be in cache
# Usage
cache = BloomFilterCache()
cache.add("user:1", {"name": "Alice", "age": 30})
cache.add("user:2", {"name": "Bob", "age": 25})
print(cache.get("user:1")) # {'name': 'Alice', 'age': 30}
print(cache.get("user:3")) # None (definitely not in cache)
This example demonstrates how a Bloom filter can be used to quickly determine if a key is definitely not in the cache, avoiding unnecessary lookups in the main cache storage.
7. Search Engines: Query Optimization
Search engines can leverage Bloom filters to optimize various aspects of their operations, from crawling to query processing.
Applications in search engines:
- Crawl Deduplication: Quickly check if a URL has already been crawled.
- Query Optimization: Determine which shards or indices are relevant for a given query.
- Spelling Suggestions: Efficiently store and check against a large dictionary of correctly spelled words.
Example: Crawl Deduplication
class WebCrawler:
def __init__(self, size=10000000, hash_count=7):
self.bloom_filter = BloomFilter(size, hash_count)
def crawl(self, url):
if self.bloom_filter.check(url):
print(f"Skipping {url} - likely already crawled")
return
# Crawl the URL
print(f"Crawling {url}")
self.bloom_filter.add(url)
# ... perform actual crawling ...
# Usage
crawler = WebCrawler()
crawler.crawl("https://example.com")
crawler.crawl("https://example.com/page1")
crawler.crawl("https://example.com") # Will be skipped
This example shows how a web crawler can use a Bloom filter to efficiently avoid recrawling URLs it has likely already visited.
Considerations and Limitations
While Bloom filters are incredibly useful in many scenarios, it’s important to be aware of their limitations and considerations:
- False Positives: Bloom filters can produce false positives, meaning they may indicate an element is in the set when it actually isn’t. The probability of false positives increases as the filter fills up.
- No Deletion: Standard Bloom filters don’t support element deletion. Variants like Counting Bloom Filters address this limitation but with increased complexity and memory usage.
- Size Trade-offs: The size of the bit array and the number of hash functions need to be carefully chosen based on the expected number of elements and the desired false positive rate.
- Not Suitable for Exact Counts: Bloom filters can’t tell you how many times an element was added or provide an exact count of unique elements.
Conclusion
Bloom filters are a powerful and versatile tool in the modern programmer’s toolkit. Their ability to provide space-efficient, probabilistic set membership tests makes them invaluable in numerous applications across various domains of computing. From enhancing web browsing security to optimizing database queries, managing network traffic, and improving search engine performance, Bloom filters offer a unique combination of efficiency and simplicity.
As data continues to grow exponentially and performance optimization remains crucial, understanding and effectively implementing Bloom filters can give developers a significant edge. Whether you’re working on large-scale distributed systems, building high-performance web applications, or tackling complex algorithmic challenges, considering Bloom filters as part of your solution can lead to more efficient and scalable designs.
Remember, like any tool, Bloom filters are not a one-size-fits-all solution. Their probabilistic nature and limitations must be carefully considered in the context of each specific use case. However, when applied appropriately, they can provide substantial benefits in terms of speed, memory usage, and overall system performance.
As you continue your journey in software development and computer science, keep Bloom filters in mind as a valuable technique for solving problems involving large datasets, rapid lookups, and resource constraints. Their elegant simplicity and powerful capabilities make them a fascinating subject for further study and a practical tool for real-world applications.