6 Common Mistakes to Avoid During Coding Interviews: A Comprehensive Guide
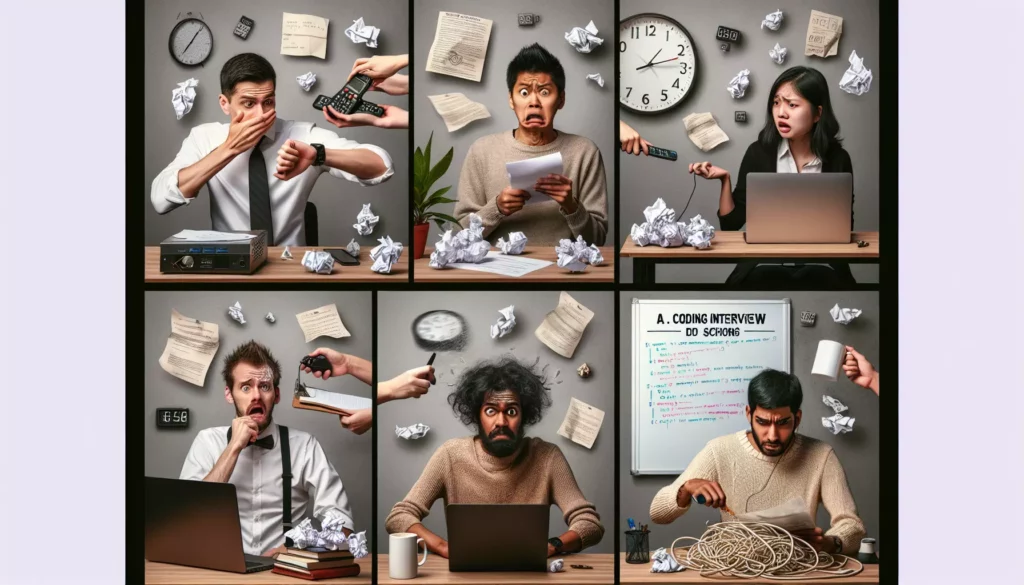
Coding interviews can be a nerve-wracking experience, even for seasoned developers. As you prepare to showcase your skills and land that dream job at a top tech company, it’s crucial to be aware of common pitfalls that could derail your chances of success. In this comprehensive guide, we’ll explore six common mistakes that candidates often make during coding interviews and provide actionable strategies to help you avoid them.
1. Diving into Code Without a Clear Plan
One of the most frequent mistakes candidates make is jumping straight into coding without taking the time to understand the problem and formulate a clear strategy. This approach can lead to confusion, wasted time, and ultimately, a suboptimal solution.
Why It’s a Problem
- Lack of understanding: Without a clear plan, you might miss crucial details or constraints in the problem statement.
- Inefficient use of time: Coding without direction can lead to frequent backtracking and revisions.
- Missed optimization opportunities: A hasty approach might cause you to overlook more efficient solutions.
How to Avoid It
- Read the problem statement carefully, multiple times if necessary.
- Ask clarifying questions to ensure you understand all requirements and constraints.
- Discuss your approach with the interviewer before writing any code.
- Sketch out a high-level algorithm or flowchart on paper or a whiteboard.
- Consider edge cases and potential optimizations before implementation.
By taking the time to plan your approach, you demonstrate thoughtfulness and problem-solving skills, which are highly valued in coding interviews.
2. Poor Communication During Problem-Solving
Many candidates underestimate the importance of clear communication during coding interviews. Remember, the interview is not just about writing correct code; it’s also an opportunity to showcase your ability to articulate your thoughts and collaborate effectively.
Why It’s a Problem
- Missed opportunities for guidance: Interviewers can’t provide hints or feedback if they don’t understand your thought process.
- Difficulty assessing problem-solving skills: Silent coding makes it challenging for interviewers to evaluate your approach and reasoning.
- Lack of teamwork demonstration: Poor communication fails to showcase your ability to work collaboratively in a team environment.
How to Avoid It
- Think out loud as you analyze the problem and consider different approaches.
- Explain your reasoning behind choosing a particular solution or data structure.
- Verbalize your thought process as you write code, discussing any trade-offs or potential optimizations.
- Ask for feedback or clarification when you’re unsure about something.
- Practice explaining your code to others before the interview.
Effective communication not only helps interviewers understand your problem-solving approach but also demonstrates your ability to work collaboratively in a team setting.
3. Neglecting Time and Space Complexity Analysis
In the rush to solve a problem, many candidates forget to consider and discuss the time and space complexity of their solutions. This oversight can be a significant red flag for interviewers, especially when applying for positions at top tech companies.
Why It’s a Problem
- Incomplete solution: A working solution without efficiency considerations is often considered incomplete.
- Missed optimization opportunities: Failing to analyze complexity can lead to overlooking more efficient algorithms.
- Lack of scalability awareness: Not considering complexity shows a lack of understanding of how solutions perform with larger inputs.
How to Avoid It
- Always analyze and discuss the time and space complexity of your solution before and after implementation.
- Consider multiple approaches and compare their complexities.
- Be prepared to explain trade-offs between time and space complexity.
- Practice identifying and expressing big O notation for common algorithms and data structures.
- Discuss potential optimizations and their impact on complexity.
By demonstrating a strong understanding of algorithmic efficiency, you show that you can create solutions that are not just correct, but also performant and scalable.
4. Ignoring Edge Cases and Input Validation
A common pitfall in coding interviews is focusing solely on the happy path and neglecting to consider edge cases or validate input. This oversight can lead to buggy code and demonstrate a lack of attention to detail.
Why It’s a Problem
- Incomplete solutions: Failing to handle edge cases results in code that works only for a subset of inputs.
- Vulnerability to errors: Lack of input validation can lead to runtime errors or unexpected behavior.
- Missed opportunity to showcase thoroughness: Considering edge cases demonstrates a comprehensive approach to problem-solving.
How to Avoid It
- After implementing the main solution, systematically consider potential edge cases:
- Empty inputs
- Single-element inputs
- Very large inputs
- Negative numbers (if applicable)
- Duplicate values
- Null or undefined values
- Implement input validation at the beginning of your function.
- Discuss edge cases with the interviewer and ask if there are any specific scenarios to consider.
- Write test cases that cover both normal scenarios and edge cases.
- Practice identifying and handling edge cases in your preparation.
Here’s an example of how you might implement input validation and handle edge cases in a simple function:
function findMaxElement(arr) {
// Input validation
if (!Array.isArray(arr) || arr.length === 0) {
throw new Error("Input must be a non-empty array");
}
// Edge case: single-element array
if (arr.length === 1) {
return arr[0];
}
// Main logic
let max = arr[0];
for (let i = 1; i < arr.length; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
// Test cases
console.log(findMaxElement([1, 5, 3, 9, 2])); // Expected: 9
console.log(findMaxElement([42])); // Edge case: single-element array
console.log(findMaxElement([-10, -5, -2, -1])); // Edge case: negative numbers
// console.log(findMaxElement([])); // This would throw an error
// console.log(findMaxElement("not an array")); // This would throw an error
By addressing edge cases and implementing input validation, you demonstrate a thorough and robust approach to problem-solving.
5. Failing to Test and Debug Code
In the pressure of an interview setting, candidates often submit their code without properly testing or debugging it. This can lead to easily avoidable errors and give the impression of carelessness.
Why It’s a Problem
- Submission of buggy code: Untested code may contain errors that could have been easily caught and fixed.
- Missed opportunity to demonstrate debugging skills: Effective debugging is a crucial skill for any developer.
- Lack of attention to detail: Failing to test your code can be perceived as a lack of thoroughness.
How to Avoid It
- Always allocate time for testing and debugging in your problem-solving approach.
- Write test cases covering various scenarios, including edge cases.
- Use print statements or debugger tools to verify the correctness of your code.
- Walk through your code step-by-step with a small example to ensure it behaves as expected.
- If you encounter a bug, demonstrate your debugging process to the interviewer.
Here’s an example of how you might approach testing and debugging during an interview:
function reverseString(str) {
if (typeof str !== 'string') {
throw new Error("Input must be a string");
}
let reversed = '';
for (let i = str.length - 1; i >= 0; i--) {
reversed += str[i];
}
return reversed;
}
// Test cases
console.log(reverseString("hello")); // Expected: "olleh"
console.log(reverseString("")); // Edge case: empty string
console.log(reverseString("a")); // Edge case: single character
console.log(reverseString("12345")); // Testing with numbers
// Debugging example
function debugReverseString(str) {
console.log("Input string:", str);
let reversed = '';
for (let i = str.length - 1; i >= 0; i--) {
console.log(`Adding character: ${str[i]}`);
reversed += str[i];
console.log(`Current reversed string: ${reversed}`);
}
console.log("Final reversed string:", reversed);
return reversed;
}
debugReverseString("debug");
By demonstrating your ability to test and debug code effectively, you show that you’re capable of producing reliable and error-free solutions.
6. Giving Up Too Easily on Challenging Problems
When faced with a difficult problem, some candidates may become discouraged and give up too quickly. This can be a missed opportunity to demonstrate perseverance and problem-solving skills.
Why It’s a Problem
- Incomplete assessment: Giving up prevents interviewers from fully evaluating your problem-solving abilities.
- Missed learning opportunity: Challenging problems are chances to learn and grow, even during an interview.
- Negative impression: Giving up easily may be interpreted as a lack of resilience or determination.
How to Avoid It
- Stay calm and maintain a positive attitude when faced with a difficult problem.
- Break down the problem into smaller, more manageable sub-problems.
- Start with a brute-force solution and then work on optimizing it.
- Communicate your thoughts and partial progress, even if you haven’t solved the entire problem.
- Ask for hints or clarification if you’re stuck, but try to solve the problem independently first.
- Practice solving challenging problems regularly to build confidence and resilience.
Remember, the interview process is not just about getting the correct answer, but also about demonstrating your problem-solving approach and ability to work through challenges.
Conclusion: Mastering the Art of Coding Interviews
Coding interviews can be challenging, but by avoiding these common mistakes, you can significantly improve your chances of success. Let’s recap the key points:
- Always plan before coding
- Communicate clearly throughout the problem-solving process
- Analyze time and space complexity
- Consider edge cases and validate input
- Test and debug your code thoroughly
- Persevere through challenging problems
Remember, practice is key to mastering these skills. Platforms like AlgoCademy offer a wealth of resources to help you prepare for coding interviews, including interactive tutorials, AI-powered assistance, and step-by-step guidance on algorithmic thinking and problem-solving.
As you continue your journey towards landing that dream job at a top tech company, keep these tips in mind and approach each coding challenge as an opportunity to showcase your skills and grow as a developer. With dedication, practice, and the right mindset, you’ll be well-equipped to tackle any coding interview that comes your way.
Additional Resources for Interview Preparation
To further enhance your coding interview skills, consider exploring the following resources:
- Online coding platforms: Practice on websites like LeetCode, HackerRank, or CodeSignal to familiarize yourself with common interview questions.
- Data Structures and Algorithms books: “Cracking the Coding Interview” by Gayle Laakmann McDowell and “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein are excellent resources.
- Mock interviews: Participate in mock interviews with peers or use platforms that offer simulated interview experiences.
- Language-specific resources: Deepen your knowledge of your preferred programming language(s) through official documentation and advanced tutorials.
- System design resources: For senior roles, study system design principles and practice designing scalable systems.
- Soft skills development: Work on your communication and problem-solving skills through public speaking or collaborative coding projects.
Remember, success in coding interviews is not just about technical knowledge, but also about how you approach problems, communicate your thoughts, and collaborate with others. By focusing on these aspects alongside your coding skills, you’ll be well-prepared to excel in your next interview and take a significant step forward in your software development career.