55 Powerful Techniques for Visualizing Problems to Find Solutions in Programming
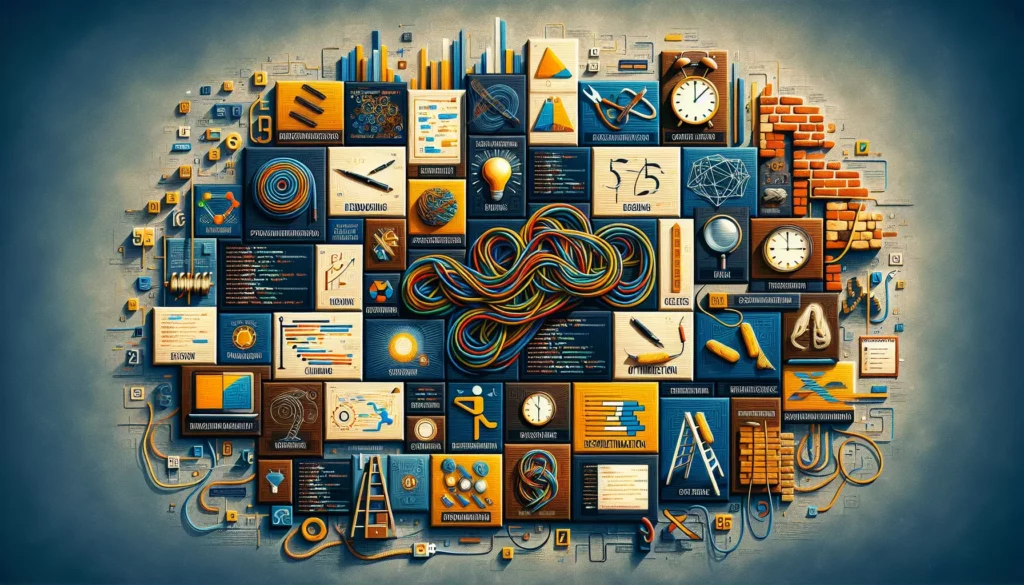
In the world of coding and software development, the ability to visualize problems is often the key to unlocking innovative solutions. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at top tech companies, mastering the art of problem visualization can significantly enhance your problem-solving skills. In this comprehensive guide, we’ll explore 55 powerful techniques that can help you better visualize coding problems and discover effective solutions.
1. Mind Mapping
Mind mapping is a classic technique that can be incredibly useful for visualizing complex problems. Start with the central issue in the middle of a blank page and branch out with related concepts, subtasks, and potential solutions. This technique helps you see the big picture and identify connections between different aspects of the problem.
How to create a mind map for coding problems:
- Write the main problem or algorithm in the center of the page.
- Draw branches for major components or steps of the solution.
- Add sub-branches for more detailed aspects or alternative approaches.
- Use colors and symbols to highlight important elements or connections.
2. Flowcharts
Flowcharts are excellent for visualizing the logical flow of an algorithm or process. They use standardized symbols to represent different types of actions and decisions, making it easier to understand and communicate complex procedures.
Key elements of a flowchart:
- Start/End: Oval shapes
- Process: Rectangle shapes
- Decision: Diamond shapes
- Input/Output: Parallelogram shapes
- Arrows: Connecting the shapes to show flow
3. Pseudocode
Pseudocode is a informal way of describing an algorithm using a combination of natural language and simple programming constructs. It’s an excellent tool for visualizing the logic of your solution before diving into actual code.
Example of pseudocode for a simple sorting algorithm:
FOR each unsorted element X
FOR each sorted element Y
IF X < Y
Insert X before Y
ENDIF
ENDFOR
ENDFOR
4. Data Structure Diagrams
When working with complex data structures like trees, graphs, or linked lists, visual representations can be invaluable. Sketching out these structures helps you understand relationships between elements and visualize operations.
Common data structure diagrams:
- Binary Trees
- Linked Lists
- Graphs (directed and undirected)
- Hash Tables
- Stacks and Queues
5. State Diagrams
State diagrams are particularly useful for visualizing problems involving finite state machines or complex system behaviors. They show different states of a system and the transitions between them.
Components of a state diagram:
- States: Represented by circles or rounded rectangles
- Transitions: Arrows showing movement between states
- Events: Labels on transitions indicating what triggers the state change
- Actions: Operations performed during transitions or within states
6. UML Diagrams
Unified Modeling Language (UML) diagrams are a standardized set of visualization tools used in software engineering. They can help you visualize various aspects of a system, from class relationships to use cases.
Types of UML diagrams:
- Class Diagrams
- Sequence Diagrams
- Use Case Diagrams
- Activity Diagrams
- Component Diagrams
7. Whiteboarding
Whiteboarding is a technique often used in technical interviews but is also valuable for personal problem-solving. It involves sketching out your thoughts and solutions on a whiteboard (or digital equivalent), allowing for quick iterations and visual brainstorming.
Tips for effective whiteboarding:
- Start with a clear problem statement
- Use different colors for different components
- Leave space for modifications and additions
- Practice explaining your thought process as you draw
8. Venn Diagrams
Venn diagrams can be useful for visualizing set relationships, overlapping conditions, or comparing different algorithms or approaches.
Use cases for Venn diagrams in programming:
- Comparing algorithm complexities (time vs. space)
- Visualizing database joins
- Illustrating the intersection of user requirements and technical constraints
9. Decision Trees
Decision trees are excellent for visualizing problems that involve multiple decision points or conditional logic. They can help you map out different paths through your algorithm based on various conditions.
Creating a decision tree:
- Start with the initial problem or input at the root
- Branch out based on different conditions or decisions
- Continue branching until you reach final outcomes or solutions
- Label each branch with the condition or decision criteria
10. Gantt Charts
While more commonly used in project management, Gantt charts can be adapted to visualize the timeline and dependencies of different components in a complex algorithm or system.
Adapting Gantt charts for algorithm visualization:
- Use tasks to represent different stages or functions of your algorithm
- Show dependencies between different parts of your solution
- Visualize parallel processes or potential areas for optimization
11. Storyboarding
Borrowed from the film industry, storyboarding can be an effective way to visualize user interactions or the step-by-step execution of an algorithm. Create a series of frames showing the progression of your solution.
Steps for storyboarding a coding problem:
- Break down the problem into key steps or stages
- Sketch a frame for each step, showing the state of variables or data structures
- Add annotations explaining what’s happening in each frame
- Review the storyboard to identify potential issues or optimizations
12. Concept Maps
Concept maps are similar to mind maps but focus more on showing relationships between different concepts. They can be useful for visualizing how different components of a system or algorithm interact.
Creating a concept map:
- Identify key concepts related to your problem
- Arrange concepts spatially based on their relationships
- Connect related concepts with labeled lines or arrows
- Use hierarchies or groupings to show higher-level relationships
13. Treemaps
Treemaps are useful for visualizing hierarchical data structures or for comparing the relative sizes of different components in a system. They use nested rectangles to represent different levels of hierarchy.
Applications of treemaps in programming:
- Visualizing file system structures
- Comparing algorithm performance across different inputs
- Representing memory usage in complex systems
14. Force-Directed Graphs
Force-directed graphs are particularly useful for visualizing network structures or complex relationships between entities. They use simulated physical forces to arrange nodes in a visually appealing and often meaningful way.
Use cases for force-directed graphs:
- Visualizing social networks
- Mapping dependencies in large codebases
- Representing complex data structures like graphs
15. Sankey Diagrams
Sankey diagrams are flow diagrams where the width of the arrows is proportional to the flow quantity. They can be adapted to visualize data flow in algorithms or resource allocation in systems.
Adapting Sankey diagrams for coding problems:
- Visualize data transformation pipelines
- Represent resource consumption across different parts of an algorithm
- Show the flow of control in complex systems
16. Ishikawa (Fishbone) Diagrams
Also known as cause-and-effect diagrams, Ishikawa diagrams can help visualize the various factors contributing to a problem or affecting the performance of an algorithm.
Creating an Ishikawa diagram:
- Write the main problem or effect at the “head” of the fish
- Draw major categories of causes as “bones” branching off the spine
- Add specific causes as smaller bones off the main categories
- Analyze the diagram to identify key areas for improvement or optimization
17. Heat Maps
Heat maps use color-coding to represent different values or intensities. In programming, they can be adapted to visualize algorithm performance, code complexity, or usage patterns.
Applications of heat maps in coding:
- Visualizing code coverage in test suites
- Representing the time complexity of nested loops
- Showing the frequency of function calls in a program
18. Radar Charts
Radar charts, also known as spider charts, can be useful for comparing multiple quantitative variables. In programming, they can help visualize trade-offs between different algorithms or approaches.
Using radar charts for algorithm comparison:
- Plot different performance metrics (time complexity, space complexity, ease of implementation) on different axes
- Compare multiple algorithms by plotting them on the same chart
- Identify strengths and weaknesses of different approaches at a glance
19. Entity-Relationship Diagrams (ERDs)
ERDs are crucial for visualizing database structures and relationships between different entities. They can help in designing efficient data models and understanding complex data relationships.
Key components of an ERD:
- Entities: Represented by rectangles
- Attributes: Listed within the entity rectangles
- Relationships: Shown as lines connecting entities
- Cardinality: Indicated on the relationship lines (one-to-one, one-to-many, many-to-many)
20. Petri Nets
Petri nets are a mathematical modeling language for describing distributed systems. They can be particularly useful for visualizing concurrent processes or resource allocation problems.
Elements of a Petri net:
- Places: Represented by circles, indicating possible states
- Transitions: Represented by rectangles, indicating events or actions
- Arcs: Arrows connecting places and transitions
- Tokens: Dots within places, representing current state or resources
21. Sequence Diagrams
Sequence diagrams are a type of UML diagram that shows how processes operate with one another and in what order. They’re particularly useful for visualizing the flow of messages between objects in a system.
Creating a sequence diagram:
- Identify the objects or processes involved
- Arrange them horizontally across the top of the diagram
- Draw vertical lifelines for each object
- Add horizontal arrows between lifelines to represent messages or method calls
- Use dotted arrows for return messages
22. Bubble Charts
Bubble charts are similar to scatter plots but add a third dimension represented by the size of the bubbles. They can be useful for visualizing multiple aspects of algorithms or data structures simultaneously.
Using bubble charts in programming:
- Compare algorithms based on time complexity (x-axis), space complexity (y-axis), and ease of implementation (bubble size)
- Visualize data structures by plotting number of elements (x-axis), access time (y-axis), and memory usage (bubble size)
- Represent function usage in a codebase by plotting frequency of calls (x-axis), execution time (y-axis), and number of parameters (bubble size)
23. Hierarchical Edge Bundling
This visualization technique is particularly useful for showing relationships in hierarchical data structures. It can help in visualizing complex dependencies or call graphs in large codebases.
Applications of hierarchical edge bundling:
- Visualizing module dependencies in large software projects
- Representing call graphs in complex algorithms
- Showing relationships between different classes or functions in object-oriented designs
24. Chord Diagrams
Chord diagrams are circular visualizations that show relationships between entities. In programming, they can be adapted to show inter-dependencies between different components of a system.
Using chord diagrams in software visualization:
- Visualize function calls between different modules
- Show data flow between different parts of an algorithm
- Represent complex many-to-many relationships in data models
25. Sunburst Diagrams
Sunburst diagrams are hierarchical visualizations that use concentric circles to represent different levels of a hierarchy. They can be useful for visualizing nested structures or hierarchical algorithms.
Applications of sunburst diagrams:
- Visualizing file system structures
- Representing the structure of recursive algorithms
- Showing the composition of complex data structures
26. Network Diagrams
Network diagrams are essential for visualizing graph problems and network-related algorithms. They can help in understanding complex relationships and pathfinding problems.
Elements of a network diagram:
- Nodes: Represented by circles or other shapes
- Edges: Lines connecting nodes
- Weights: Numbers on edges (for weighted graphs)
- Directions: Arrows on edges (for directed graphs)
27. Tree Diagrams
Tree diagrams are crucial for visualizing hierarchical data structures and algorithms that work with trees, such as binary search trees or decision trees.
Types of tree diagrams:
- Binary Trees
- N-ary Trees
- Balanced Trees (e.g., AVL, Red-Black)
- Tries
28. Stack and Queue Visualizations
Visual representations of stacks and queues can help in understanding these fundamental data structures and the algorithms that use them.
Visualizing stack operations:
| |
| |
|3| <-- Top
|2|
|1|
---------
Stack
Visualizing queue operations:
Front --> [1][2][3] <-- Rear
Queue
29. Memory Diagrams
Memory diagrams can help visualize how data is stored and accessed in memory, which is particularly useful for understanding pointer operations and memory management.
Elements of a memory diagram:
- Memory addresses
- Variable names
- Data values
- Pointers (represented by arrows)
30. Recursion Trees
Recursion trees are valuable for visualizing the execution of recursive algorithms, showing the branching of recursive calls and helping to analyze time complexity.
Creating a recursion tree:
- Start with the initial function call at the root
- Branch out for each recursive call
- Continue until you reach base cases
- Annotate each node with the size of the subproblem
31. Voronoi Diagrams
While less common in everyday programming, Voronoi diagrams can be useful for visualizing certain types of spatial problems and algorithms.
Applications of Voronoi diagrams in programming:
- Nearest neighbor problems
- Spatial partitioning in game development
- Visualizing clustering algorithms
32. Pixel Matrix Visualizations
For problems involving image processing or 2D grid manipulations, visualizing the data as a pixel matrix can be incredibly helpful.
Creating a pixel matrix visualization:
- Represent each cell as a colored square
- Use different colors to represent different states or values
- Add grid lines to clearly separate cells
- Include a legend to explain the color coding
33. Animated Visualizations
For complex algorithms or data structures, static visualizations may not be sufficient. Animated visualizations can help in understanding the step-by-step execution of an algorithm.
Tools for creating animated algorithm visualizations:
- JavaScript libraries like D3.js or p5.js
- Python libraries such as matplotlib or Manim
- Specialized algorithm visualization platforms like VisuAlgo
34. Interval Trees
Interval trees are specialized data structures for storing intervals or segments. Visualizing them can help in understanding algorithms dealing with time intervals or ranges.
Visualizing an interval tree:
- Represent nodes as circles containing the interval’s midpoint
- Show the interval range as a horizontal line through the node
- Use different colors for left and right subtrees
- Annotate with max endpoint values for each subtree
35. Fractal Visualizations
While not directly applicable to many programming problems, fractal visualizations can be useful for understanding recursive patterns and self-similar structures.
Examples of fractal visualizations in programming:
- Visualizing recursive backtracking algorithms
- Understanding divide-and-conquer strategies
- Illustrating concepts of self-similarity in data structures
36. System Architecture Diagrams
For complex systems or large-scale applications, system architecture diagrams can help visualize the overall structure and interactions between different components.
Elements of a system architecture diagram:
- Components (e.g., servers, databases, APIs)
- Connections between components
- Data flow indicators
- External interfaces or user touchpoints
37. Compiler Design Visualizations
For problems related to compiler design or language processing, specialized visualizations can be helpful.
Types of compiler design visualizations:
- Abstract Syntax Trees (ASTs)
- Parse trees
- State machines for lexical analysis
- Data flow graphs
38. Karnaugh Maps
While primarily used in digital logic design, Karnaugh maps can be adapted to visualize boolean logic problems in programming.
Using Karnaugh maps in programming:
- Simplifying complex boolean expressions
- Optimizing if-else structures
- Visualizing truth tables for logic problems
39. Spiral Model Visualizations
Borrowed from software development methodologies, spiral model visualizations can be adapted to represent iterative problem-solving approaches.
Adapting spiral models for problem visualization:
- Represent each iteration as a loop in the spiral
- Show refinement of the solution as you move outwards
- Highlight key decision points or milestones
40. Parallel Coordinates
Parallel coordinate plots can be useful for visualizing multi-dimensional data or comparing multiple attributes of different algorithms or solutions.
Using parallel coordinates in algorithm analysis:
- Compare multiple performance metrics across different algorithms
- Visualize trade-offs between different solution approaches
- Represent multi-dimensional data points in algorithm output
41. Alluvial Diagrams
Alluvial diagrams are flow diagrams that can help visualize changes in data structure or composition over multiple states or time periods.
Applications of alluvial diagrams in programming:
- Visualizing data transformations in ETL processes
- Showing the evolution of data structures during algorithm execution
- Representing user flow through different states in an application
42. Circular Packing Diagrams
Circular packing diagrams can be useful for visualizing hierarchical data where the size of elements is significant.
Using circular packing in coding visualizations:
- Representing file sizes in directory structures
- Visualizing memory allocation in heap structures
- Showing the relative importance of different components in a system
43. Flame Graphs
Flame graphs are particularly useful for visualizing performance profiles of software, showing where time is spent in different function calls.
Creating and interpreting flame graphs:
- X-axis represents the population (usually time or memory)
- Y-axis shows the stack depth
- Each rectangle represents a function in the stack
- Width of rectangles indicates the time spent in that function
44. Dependency Graphs
Dependency graphs are crucial for visualizing relationships and dependencies between different components of a software system.
Elements of a dependency graph:
- Nodes representing modules or components
- Directed edges showing dependencies
- Color coding to indicate different types of dependencies
- Annotations for version numbers or other metadata
45. Timelines
Timelines can be adapted to visualize the execution flow of algorithms, especially those involving time-based operations or scheduling.
Using timelines in algorithm visualization:
- Represent different stages of an algorithm along a horizontal axis
- Show parallel execution of different components
- Highlight critical paths or bottlenecks
46. Sankey Diagrams
Sankey diagrams are flow diagrams where the width of the arrows is proportional to the flow quantity. They can be useful for visualizing data transformations or resource allocation in algorithms.
Applications of Sankey diagrams in programming:
- Visualizing data flow in complex algorithms
- Showing resource allocation in scheduling problems
- Representing user journeys through a system
47. Force-Directed Graphs
Force-directed graph drawing algorithms produce layouts based on physical simulations. They’re particularly useful for visualizing network structures or complex relationships between entities.
Using force-directed graphs in coding problems:
- Visualizing social network structures
- Representing complex data relationships
- Showing module dependencies in large codebases
48. Treemaps
Treemaps display hierarchical data using nested rectangles. They can be useful for visualizing hierarchical structures where size is an important factor.
Applications of treemaps in programming:
- Visualizing file system usage
- Representing memory allocation in programs
- Showing the composition of complex data structures
49. Chord Diagrams
Chord diagrams visualize the inter-relationships between entities. In programming, they can be used to show complex interactions between different parts of a system.
Using chord diagrams in software visualization:
- Showing function call relationships in a codebase
- Visualizing data flow between different modules
- Representing complex database relationships
50. Heatmaps
Heatmaps use color-coding to represent different values in a two-dimensional grid. They can be particularly useful for visualizing performance characteristics or data patterns.
Applications of heatmaps in coding:
- Visualizing algorithm performance across different inputs
- Showing code coverage in test suites
- Representing usage patterns in user interfaces
51. Radar Charts
Radar charts, also known as spider charts, display multivariate data in the form of a two-dimensional chart with three or more quantitative variables.
Using radar charts for algorithm comparison:
- Compare multiple algorithms across various performance metrics
- Visualize trade-offs between different solution approaches
- Represent the strengths and weaknesses of different data structures
52. Voronoi Diagrams
Voronoi diagrams partition a plane into regions based on distance to points in a specific subset of the plane. They can be useful for certain types of spatial problems.
Applications of Voronoi diagrams in programming:
- Solving nearest neighbor problems
- Optimizing spatial databases
- Generating procedural textures in computer graphics
53. Pixel Matrices
Pixel matrices represent data as a grid of colored cells. They can be particularly useful for visualizing image processing algorithms or cellular automata.
Using pixel matrices in algorithm visualization:
- Showing the steps of image processing algorithms
- Visualizing the state of cellular automata
- Representing 2D grid-based problems
54. Fractal Visualizations
Fractal visualizations can help in understanding recursive algorithms and self-similar structures in programming.
Applications of fractal visualizations:
- Illustrating recursive algorithms
- Visualizing divide-and-conquer strategies
- Representing self-similar data structures
55. Interactive Visualizations
Interactive visualizations allow users to explore and manipulate the visual representation of data or algorithms in real-time. They can be particularly powerful for understanding complex systems or algorithms.
Creating interactive visualizations:
- Use web technologies like D3.js or Three.js for browser-based visualizations
- Implement controls for zooming, panning, and filtering data
- Allow users to input different parameters and see real-time updates
- Provide tooltips or additional information on hover or click events
Conclusion
Mastering these 55 visualization techniques can significantly enhance your problem-solving skills in programming. By effectively visualizing problems, you can gain deeper insights, identify patterns more easily, and develop more efficient solutions. Remember that different problems may require different visualization approaches, so it’s valuable to be familiar with a wide range of techniques.
As you practice these visualization methods, you’ll likely find that some work better for you than others. Experiment with different techniques and combine them as needed to create the most effective visualizations for your specific problems. With time and practice, you’ll develop a keen intuition for which visualization methods to apply in different situations, making you a more effective problem solver and coder.
Keep in mind that visualization is just one part of the problem-solving process. It should be used in conjunction with other skills like logical thinking, algorithm design, and coding proficiency. As you prepare for technical interviews or tackle complex coding challenges, remember to leverage these visualization techniques to break down problems, explore solutions, and communicate your ideas effectively.
Happy coding and visualizing!