50 Common Coding Mistakes to Avoid in Interviews
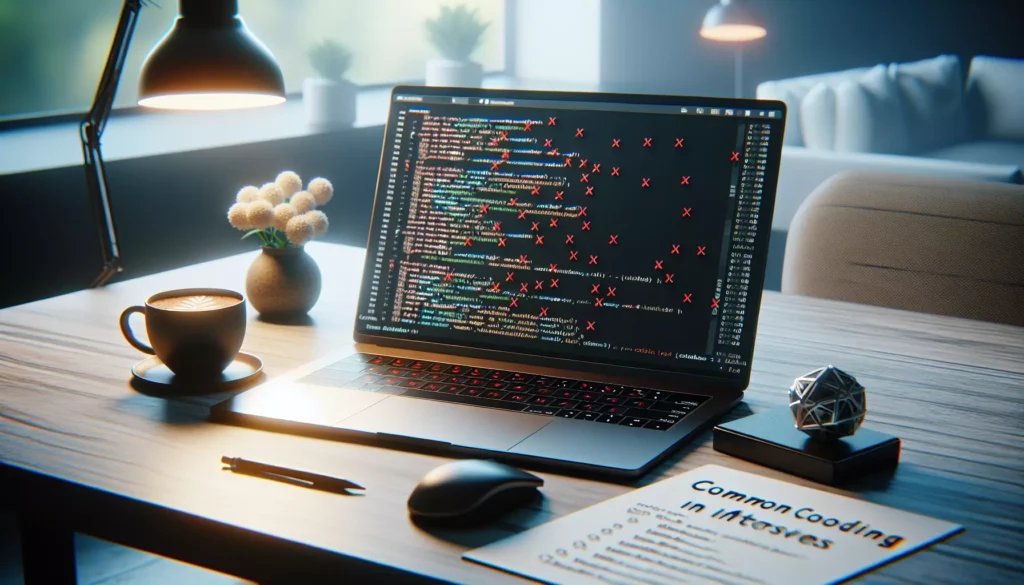
When preparing for coding interviews, especially for major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), it’s crucial to not only focus on what to do but also what to avoid. In this comprehensive guide, we’ll explore 50 common coding mistakes that candidates often make during interviews. By being aware of these pitfalls, you can improve your performance and increase your chances of success.
Algorithmic and Problem-Solving Mistakes
1. Failing to Clarify the Problem
One of the most critical mistakes is jumping into coding without fully understanding the problem. Always take time to ask clarifying questions and ensure you have a clear grasp of the requirements.
2. Neglecting Edge Cases
Overlooking edge cases is a common pitfall. Consider scenarios like empty inputs, null values, or extreme cases that might break your solution.
3. Ignoring Time and Space Complexity
Interviewers often assess your ability to optimize solutions. Don’t forget to analyze and discuss the time and space complexity of your algorithm.
4. Using Brute Force Without Consideration
While brute force solutions can be a starting point, relying solely on them without attempting to optimize can be seen as a lack of problem-solving skills.
5. Overlooking Simpler Solutions
Sometimes, candidates overcomplicate their approach. Always consider if there’s a simpler, more elegant solution to the problem.
6. Not Considering Multiple Approaches
Fixating on a single approach without exploring alternatives can limit your problem-solving abilities. Consider multiple strategies before settling on one.
7. Misunderstanding the Problem Constraints
Pay close attention to the constraints mentioned in the problem statement. Ignoring these can lead to solutions that don’t meet the requirements.
8. Failing to Plan Before Coding
Diving into coding without a clear plan can lead to confusion and wasted time. Spend a few minutes outlining your approach before implementation.
9. Neglecting Input Validation
Assuming inputs will always be valid is a rookie mistake. Always include input validation in your solution to handle unexpected data.
10. Ignoring Scalability
While your solution might work for small inputs, consider how it would perform with large datasets. Scalability is often a key consideration in real-world applications.
Coding and Implementation Mistakes
11. Poor Variable Naming
Using unclear or overly abbreviated variable names can make your code hard to read. Opt for descriptive, meaningful names.
12. Inconsistent Coding Style
Switching between different coding styles (e.g., camelCase and snake_case) within the same solution can be distracting. Maintain consistency throughout your code.
13. Neglecting Code Comments
While clean, self-explanatory code is ideal, strategic comments can help clarify complex logic or explain the reasoning behind certain decisions.
14. Overusing Global Variables
Relying heavily on global variables can lead to code that’s hard to maintain and debug. Use function parameters and return values when possible.
15. Ignoring Code Modularity
Writing monolithic functions instead of breaking down the problem into smaller, manageable functions can make your code less readable and harder to maintain.
16. Misusing Language-Specific Features
Not leveraging built-in language features or using them incorrectly can lead to inefficient code. Familiarize yourself with the language’s standard library and idiomatic practices.
17. Hardcoding Values
Avoid hardcoding values that might change. Use constants or configuration variables for better maintainability.
18. Neglecting Error Handling
Failing to handle potential errors or exceptions can lead to fragile code. Implement proper error handling mechanisms.
19. Overcomplicating Simple Operations
Sometimes, candidates use complex constructs for simple operations. Opt for straightforward, readable code when possible.
20. Inefficient Use of Data Structures
Choosing the wrong data structure for the task at hand can lead to suboptimal performance. Be familiar with various data structures and their use cases.
Logical and Conceptual Mistakes
21. Off-by-One Errors
These errors often occur when dealing with array indices or loop boundaries. Double-check your index calculations and loop conditions.
22. Infinite Loops
Failing to properly update loop variables or using incorrect loop conditions can result in infinite loops. Always ensure your loops have a clear termination condition.
23. Stack Overflow
When using recursion, be mindful of the potential for stack overflow. Consider the maximum depth of recursion and whether an iterative solution might be more appropriate.
24. Misunderstanding Reference vs. Value
Confusion about when objects are passed by reference or by value can lead to unexpected behavior. Understand how your chosen language handles object passing.
25. Ignoring Integer Overflow
When dealing with large numbers or arithmetic operations, be aware of the potential for integer overflow and handle it appropriately.
26. Mishandling Null or Undefined Values
Failing to check for null or undefined values can lead to runtime errors. Implement proper null checks in your code.
27. Incorrect Use of Equality Operators
Mixing up == and === in JavaScript, for example, can lead to unexpected behavior. Understand the nuances of equality comparison in your language.
28. Misunderstanding Operator Precedence
Assuming incorrect operator precedence can lead to logical errors. When in doubt, use parentheses to clarify the order of operations.
29. Ignoring Type Coercion
In languages with dynamic typing, unexpected type coercion can lead to bugs. Be explicit about type conversions when necessary.
30. Misusing Break and Continue Statements
Improper use of break and continue can lead to incorrect loop behavior. Ensure you understand how these statements affect your loop flow.
Testing and Debugging Mistakes
31. Insufficient Testing
Not thoroughly testing your solution with various inputs, including edge cases, can leave bugs undetected. Always test your code before declaring it complete.
32. Ignoring Compiler Warnings
Compiler warnings often indicate potential issues in your code. Don’t ignore them; address each warning to ensure robust code.
33. Ineffective Debugging Techniques
Relying solely on print statements for debugging can be inefficient. Familiarize yourself with debugging tools and techniques for your language and environment.
34. Not Stepping Through Code
When faced with a bug, stepping through your code line by line can reveal issues that might not be apparent at first glance.
35. Assuming Code Works Without Verification
Always verify that your code produces the expected output, even if it compiles without errors. Don’t assume correctness without evidence.
Interview-Specific Mistakes
36. Poor Time Management
Spending too much time on a single aspect of the problem can leave you rushed for the rest. Practice time management during your interview preparation.
37. Lack of Communication
Coding in silence can be detrimental. Explain your thought process and approach as you work through the problem.
38. Ignoring Interviewer Hints
Interviewers often provide subtle hints or guidance. Pay attention to their feedback and be open to changing your approach if needed.
39. Not Asking for Help When Stuck
It’s okay to ask for clarification or a hint if you’re stuck. This shows your ability to collaborate and seek assistance when needed.
40. Rushing to Code Without Planning
Take time to plan your approach before coding. Rushing into implementation without a clear strategy can lead to confusion and mistakes.
Language-Specific Mistakes
41. Python: Mutable Default Arguments
Using mutable objects as default arguments in Python can lead to unexpected behavior. Be cautious when using lists or dictionaries as default arguments.
42. Java: Neglecting to Close Resources
Failing to properly close resources like file handles or database connections can lead to resource leaks. Use try-with-resources or finally blocks to ensure proper cleanup.
43. JavaScript: Misunderstanding ‘this’ Keyword
The behavior of the ‘this’ keyword in JavaScript can be confusing. Understand how it works in different contexts to avoid unexpected results.
44. C++: Memory Management Errors
Improper memory management in C++ can lead to memory leaks or segmentation faults. Be diligent about allocating and freeing memory correctly.
45. Ruby: Forgetting to Use ‘return’ in Methods
In Ruby, the last evaluated expression is implicitly returned. However, explicitly using ‘return’ can make your code clearer and prevent unintended return values.
General Programming Mistakes
46. Overengineering Solutions
Sometimes, candidates try to showcase their skills by implementing overly complex solutions. Remember that simplicity and clarity are often preferred.
47. Ignoring Code Readability
Writing code that’s difficult for others to read and understand is a significant mistake. Prioritize clarity and maintainability in your solutions.
48. Not Considering Performance Implications
While premature optimization is often discouraged, completely ignoring performance considerations can lead to inefficient solutions.
49. Failing to Handle Concurrency Issues
In problems involving multi-threading or concurrent operations, failing to address potential race conditions or deadlocks is a critical mistake.
50. Neglecting to Learn from Mistakes
Perhaps the most crucial mistake is not learning from your errors. Reflect on your mistakes, understand why they occurred, and use that knowledge to improve.
Conclusion
Avoiding these common coding mistakes can significantly improve your performance in technical interviews. Remember, the goal is not just to solve the problem, but to demonstrate your problem-solving skills, coding proficiency, and ability to write clean, efficient, and maintainable code.
As you prepare for your interviews, practice identifying and correcting these mistakes in your own code. Use platforms like AlgoCademy to hone your skills, work through challenging problems, and receive feedback on your solutions. With diligent practice and awareness of these common pitfalls, you’ll be well-equipped to tackle even the most challenging coding interviews at top tech companies.
Remember, becoming a great programmer is an ongoing journey of learning and improvement. Embrace your mistakes as opportunities to grow, and approach each coding challenge with enthusiasm and a willingness to learn. Good luck with your interview preparation!