5 Effective Strategies for Dry Running Code with Test Cases
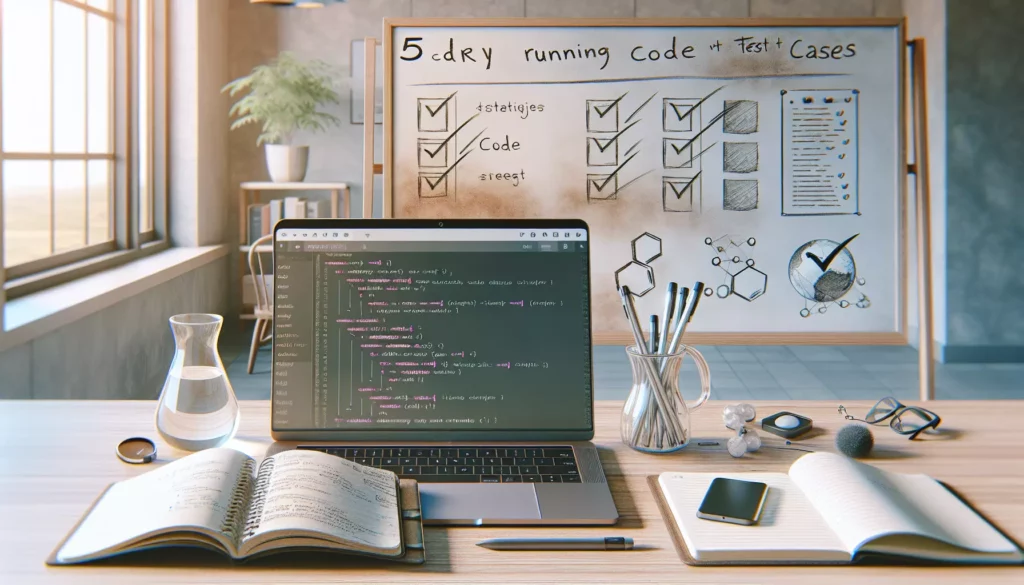
In the world of programming and software development, the ability to effectively dry run code with test cases is an invaluable skill. Whether you’re a beginner learning the ropes or an experienced developer preparing for technical interviews at top tech companies, mastering this technique can significantly improve your coding proficiency and problem-solving abilities. In this comprehensive guide, we’ll explore five powerful strategies for dry running code with test cases, helping you enhance your algorithmic thinking and coding skills.
1. Start with Simple, Edge Cases
When dry running your code, it’s crucial to begin with simple and edge cases. These cases help you quickly identify potential issues and ensure your code handles extreme scenarios correctly.
Why Start Simple?
Starting with simple cases allows you to:
- Verify basic functionality
- Catch obvious errors early
- Build confidence in your solution
Identifying Edge Cases
Edge cases are scenarios that test the boundaries of your code. Common edge cases include:
- Empty inputs
- Minimum and maximum values
- Single-element inputs
- Negative numbers (when applicable)
Example: Binary Search
Let’s consider a binary search algorithm. Here are some simple and edge cases to start with:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
# Test cases
print(binary_search([1, 3, 5, 7, 9], 5)) # Simple case
print(binary_search([1], 1)) # Single-element array
print(binary_search([], 5)) # Empty array
print(binary_search([1, 2, 3, 4, 5], 6)) # Target not in array
print(binary_search([1, 2, 3, 4, 5], 1)) # Target at the beginning
print(binary_search([1, 2, 3, 4, 5], 5)) # Target at the end
By running these test cases, you can quickly verify if your binary search implementation handles various scenarios correctly.
2. Visualize the Process
Visualization is a powerful tool for understanding how your code works and identifying potential issues. By creating visual representations of your algorithm’s steps, you can better grasp the logic and spot errors more easily.
Techniques for Visualization
- Flowcharts: Create a flowchart of your algorithm to map out the decision-making process.
- State diagrams: For algorithms that involve state changes, use state diagrams to illustrate transitions.
- Variable tracking: Keep track of variable changes at each step of the algorithm.
- Array/matrix visualization: For algorithms involving arrays or matrices, draw out the data structure and show how it changes.
Example: Bubble Sort Visualization
Let’s visualize a bubble sort algorithm:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
# Visualization
arr = [64, 34, 25, 12, 22, 11, 90]
print("Original array:", arr)
for i in range(len(arr)):
for j in range(0, len(arr)-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
print(f"Step {i+1}.{j+1}: {arr}")
print("Sorted array:", arr)
This visualization helps you see how the array changes after each swap, making it easier to understand the sorting process and identify any issues.
3. Use Hand-Tracing Techniques
Hand-tracing is a valuable technique for dry running code, especially when preparing for technical interviews. It involves manually stepping through your code line by line, tracking variable changes and control flow.
Steps for Effective Hand-Tracing
- Write out your code on paper or a whiteboard.
- Create a table to track variable values.
- Step through each line of code, updating variable values and noting control flow changes.
- Pay special attention to loops and conditional statements.
- Write out the output at each step.
Example: Hand-Tracing a Recursive Function
Let’s hand-trace a recursive function to calculate the nth Fibonacci number:
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
# Hand-trace for fibonacci(4)
# Step 1: fibonacci(4)
# - n = 4, not <= 1, so we recurse
# - Return fibonacci(3) + fibonacci(2)
# Step 2: fibonacci(3)
# - n = 3, not <= 1, so we recurse
# - Return fibonacci(2) + fibonacci(1)
# Step 3: fibonacci(2)
# - n = 2, not <= 1, so we recurse
# - Return fibonacci(1) + fibonacci(0)
# Step 4: fibonacci(1)
# - n = 1, <= 1, so we return 1
# Step 5: fibonacci(0)
# - n = 0, <= 1, so we return 0
# Now we can start resolving the recursive calls:
# fibonacci(2) = fibonacci(1) + fibonacci(0) = 1 + 0 = 1
# fibonacci(3) = fibonacci(2) + fibonacci(1) = 1 + 1 = 2
# fibonacci(4) = fibonacci(3) + fibonacci(2) = 2 + 1 = 3
print(fibonacci(4)) # Output: 3
By hand-tracing this recursive function, you can better understand how the recursion works and verify that your implementation is correct.
4. Implement Systematic Test Case Generation
Developing a systematic approach to generating test cases is crucial for thorough code testing. This strategy helps ensure you cover a wide range of scenarios and edge cases.
Techniques for Test Case Generation
- Equivalence partitioning: Divide input data into groups that should be processed similarly.
- Boundary value analysis: Test values at the edges of input ranges.
- Decision table testing: Create a table of all possible input combinations and expected outputs.
- State transition testing: For algorithms with different states, test all possible state transitions.
Example: Test Case Generation for a String Reversal Function
def reverse_string(s):
return s[::-1]
# Test case generation
test_cases = [
# Equivalence partitioning
("hello", "olleh"), # Normal case
("", ""), # Empty string
("a", "a"), # Single character
# Boundary value analysis
("ab", "ba"), # Two characters
("abc", "cba"), # Three characters
# Special cases
("12345", "54321"), # Numeric string
("a b c", "c b a"), # String with spaces
("!@#$%", "%$#@!"), # Special characters
# Unicode characters
("ã“ã‚“ã«ã¡ã¯", "ã¯ã¡ã«ã‚“ã“"), # Non-ASCII characters
]
# Run test cases
for input_str, expected_output in test_cases:
result = reverse_string(input_str)
print(f"Input: {input_str}")
print(f"Expected: {expected_output}")
print(f"Result: {result}")
print(f"Pass: {result == expected_output}\n")
By systematically generating test cases, you can ensure your function handles various input types and edge cases correctly.
5. Leverage Test-Driven Development (TDD) Principles
Test-Driven Development is a software development approach where you write tests before implementing the actual code. While full TDD might not always be practical for coding interviews or quick problem-solving, adopting some of its principles can greatly improve your dry running and testing process.
Key TDD Principles for Dry Running
- Write test cases first: Before implementing your solution, write down expected inputs and outputs.
- Start with failing tests: Ensure your test cases fail before you start coding.
- Implement minimal code: Write just enough code to pass the current test case.
- Refactor: Once all tests pass, refactor your code for better efficiency and readability.
- Repeat: Add more test cases and continue the cycle.
Example: TDD Approach for a Palindrome Checker
# Step 1: Write test cases
test_cases = [
("racecar", True),
("hello", False),
("A man a plan a canal Panama", True),
("", True),
("a", True),
("ab", False),
]
# Step 2: Implement a minimal function that fails all tests
def is_palindrome(s):
return False
# Step 3: Run tests and implement code incrementally
def test_is_palindrome():
for input_str, expected_output in test_cases:
result = is_palindrome(input_str)
print(f"Input: {input_str}")
print(f"Expected: {expected_output}")
print(f"Result: {result}")
print(f"Pass: {result == expected_output}\n")
# Initial test run (all tests should fail)
print("Initial tests:")
test_is_palindrome()
# Step 4: Implement the function to pass all tests
def is_palindrome(s):
# Remove non-alphanumeric characters and convert to lowercase
s = ''.join(c.lower() for c in s if c.isalnum())
# Check if the string is equal to its reverse
return s == s[::-1]
# Final test run
print("Final tests:")
test_is_palindrome()
# Step 5: Refactor if necessary (in this case, our implementation is already efficient)
By following TDD principles, you ensure that your code meets all requirements and handles various scenarios correctly.
Conclusion
Mastering the art of dry running code with test cases is essential for becoming a proficient programmer and excelling in technical interviews. By implementing these five strategies – starting with simple and edge cases, visualizing the process, using hand-tracing techniques, implementing systematic test case generation, and leveraging TDD principles – you’ll significantly improve your ability to write robust, error-free code.
Remember, practice is key to perfecting these techniques. As you work through coding challenges and prepare for interviews, make dry running and testing an integral part of your problem-solving process. With time and consistent application, these strategies will become second nature, enhancing your coding skills and boosting your confidence in tackling complex programming problems.
Happy coding, and may your algorithms always run smoothly!