28 Essential Tips for Cracking Coding Interviews in C++
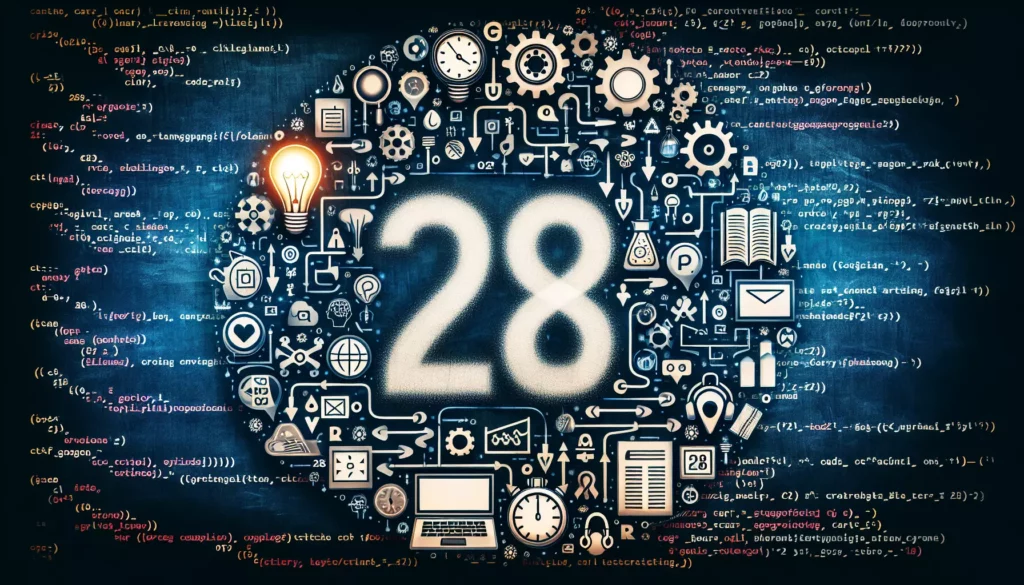
Are you gearing up for a coding interview that focuses on C++? Whether you’re aiming for a position at a FAANG company or any tech firm that values strong programming skills, being well-prepared is crucial. In this comprehensive guide, we’ll explore 28 essential tips to help you ace your C++ coding interviews. From mastering core concepts to tackling common interview questions, we’ve got you covered.
1. Master the Fundamentals of C++
Before diving into complex algorithms and data structures, ensure you have a solid grasp of C++ basics. This includes understanding:
- Variables and data types
- Control structures (if-else, loops)
- Functions and their overloading
- Object-oriented programming concepts
- Memory management
Familiarize yourself with the syntax and peculiarities of C++. For instance, know the difference between:
int x = 5; // C-style initialization
int y{5}; // C++11 uniform initialization
2. Understand C++ Standard Template Library (STL)
The STL is a powerful set of C++ template classes to provide general-purpose classes and functions. Be comfortable with:
- Containers (vector, list, map, set)
- Algorithms (sort, find, binary_search)
- Iterators
- Function objects
For example, sorting a vector in C++ is as simple as:
#include <algorithm>
#include <vector>
std::vector<int> v = {5, 2, 8, 1, 9};
std::sort(v.begin(), v.end());
3. Practice Common Data Structures
Implement and understand the following data structures in C++:
- Arrays and Dynamic Arrays (std::vector)
- Linked Lists
- Stacks and Queues
- Trees (Binary Trees, Binary Search Trees)
- Graphs
- Hash Tables (std::unordered_map)
Here’s a simple implementation of a singly linked list node in C++:
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(nullptr) {}
};
4. Learn Essential Algorithms
Be proficient in implementing and explaining these algorithms:
- Sorting (Quick Sort, Merge Sort)
- Searching (Binary Search)
- Graph traversals (BFS, DFS)
- Dynamic Programming
- Greedy Algorithms
5. Understand Time and Space Complexity
Know how to analyze the time and space complexity of your solutions. Be familiar with Big O notation and be able to optimize your code for better performance.
6. Practice Problem-Solving
Solve problems on platforms like LeetCode, HackerRank, or CodeForces. Focus on medium to hard difficulty problems, as these are more likely to appear in interviews.
7. Mock Interviews
Conduct mock interviews with friends or use platforms like Pramp to simulate real interview conditions. This helps you get comfortable with explaining your thought process while coding.
8. Learn to Write Clean, Readable Code
Follow good coding practices:
- Use meaningful variable and function names
- Keep functions small and focused
- Comment your code when necessary
- Follow consistent indentation and formatting
9. Understand Memory Management in C++
Know the differences between stack and heap memory. Understand concepts like:
- Pointers and references
- Dynamic memory allocation (new and delete)
- Smart pointers (unique_ptr, shared_ptr)
Here’s an example of using a unique_ptr:
#include <memory>
std::unique_ptr<int> ptr = std::make_unique<int>(5);
// No need to manually delete, memory is automatically freed when ptr goes out of scope
10. Be Familiar with C++11 and Later Features
Modern C++ introduced many useful features. Be familiar with:
- Auto keyword
- Lambda expressions
- Range-based for loops
- Move semantics
11. Understand Object-Oriented Programming in C++
Know how to implement and use:
- Classes and objects
- Inheritance
- Polymorphism
- Encapsulation
12. Practice Explaining Your Thought Process
During interviews, it’s crucial to communicate your thinking. Practice explaining your approach, considering edge cases, and discussing trade-offs between different solutions.
13. Learn to Handle Edge Cases
Always consider edge cases in your solutions. This includes:
- Empty inputs
- Single-element inputs
- Very large inputs
- Negative numbers (when applicable)
14. Understand and Use Design Patterns
Familiarize yourself with common design patterns like:
- Singleton
- Factory
- Observer
- Strategy
15. Know How to Debug Effectively
Learn to use debugging tools and techniques. Be able to find and fix bugs in your code quickly.
16. Understand Multithreading Basics
Know the basics of concurrent programming in C++:
- std::thread
- Mutexes and locks
- Condition variables
17. Be Familiar with File I/O
Know how to read from and write to files in C++. Understand the differences between text and binary file operations.
18. Practice Implementing Common Data Structures from Scratch
While STL provides many data structures, be prepared to implement them from scratch. This shows a deeper understanding of their inner workings.
19. Understand the Concept of References vs Pointers
Know when to use references and when to use pointers. Understand the differences in syntax and behavior.
20. Learn About Exception Handling
Understand how to use try-catch blocks and throw exceptions. Know when it’s appropriate to use exception handling.
21. Be Comfortable with Bitwise Operations
Some interview questions may involve bit manipulation. Be familiar with operations like:
- AND (&)
- OR (|)
- XOR (^)
- Left shift (<<)
- Right shift (>>)
22. Understand the Differences Between C and C++
Know what features C++ adds to C, such as classes, function overloading, and templates.
23. Practice Implementing Recursive Solutions
Many problems can be solved elegantly using recursion. Practice implementing both recursive and iterative solutions to problems.
24. Learn About Template Metaprogramming
While not always necessary for interviews, understanding template metaprogramming can demonstrate advanced C++ knowledge.
25. Be Familiar with Standard Input/Output Operations
Know how to use cin, cout, and the <iostream> library effectively.
26. Understand the Concept of Const Correctness
Know how and when to use const in your code to prevent unintended modifications and improve code safety.
27. Practice Coding Without an IDE
Many interviews are conducted on whiteboards or in simple text editors. Practice coding without the help of auto-completion and error highlighting.
28. Stay Updated with C++ Standards
Keep yourself informed about the latest C++ standards (C++17, C++20) and their new features. This shows that you’re passionate about the language and stay current with its evolution.
Conclusion
Mastering these 28 tips will significantly boost your chances of success in C++ coding interviews. Remember, consistent practice and a deep understanding of the language are key. Don’t just memorize solutions; strive to understand the underlying concepts and principles.
At AlgoCademy, we provide interactive coding tutorials and resources to help you master these skills. Our platform offers AI-powered assistance and step-by-step guidance to help you progress from beginner-level coding to acing technical interviews at major tech companies.
Keep practicing, stay curious, and approach each problem as an opportunity to learn. With dedication and the right preparation, you’ll be well-equipped to tackle any C++ coding interview that comes your way. Good luck!