22 Common Mistakes to Avoid in Coding Interviews: A Comprehensive Guide
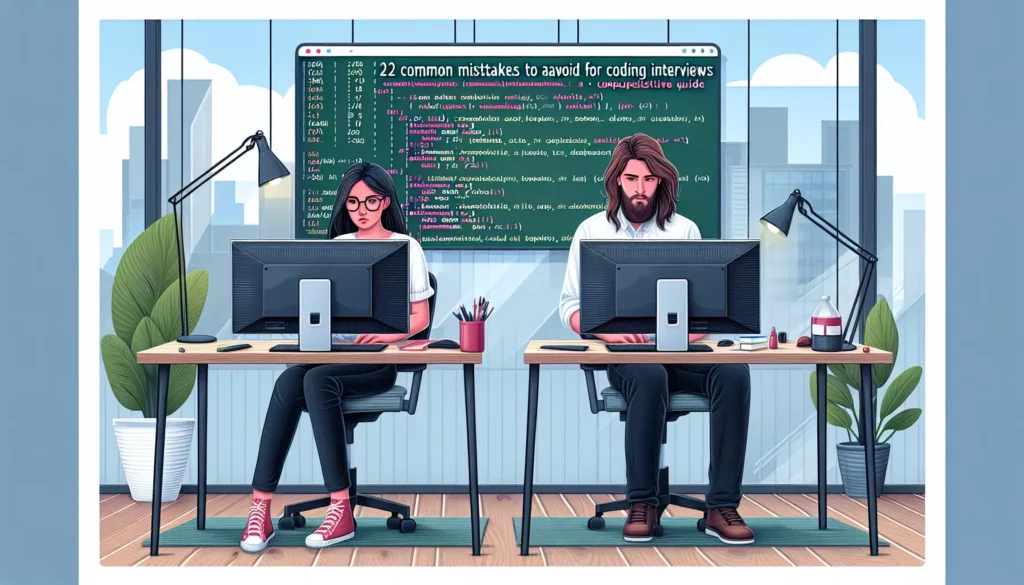
Coding interviews can be nerve-wracking experiences, even for seasoned developers. Whether you’re a fresh graduate or an experienced professional looking to land your dream job at a top tech company, it’s crucial to be well-prepared and avoid common pitfalls. In this comprehensive guide, we’ll explore 22 common mistakes that candidates often make during coding interviews and provide valuable insights on how to avoid them.
1. Failing to Clarify the Problem
One of the most critical mistakes candidates make is jumping straight into coding without fully understanding the problem at hand. Always take the time to clarify the requirements, constraints, and expected outcomes with your interviewer.
How to Avoid:
- Ask questions to ensure you understand the problem completely
- Discuss edge cases and potential assumptions
- Repeat the problem statement back to the interviewer to confirm your understanding
2. Not Planning Before Coding
Diving into code without a clear plan can lead to confusion and wasted time. It’s essential to think through your approach before writing any code.
How to Avoid:
- Spend a few minutes outlining your approach on paper or a whiteboard
- Discuss your high-level strategy with the interviewer
- Break down the problem into smaller, manageable steps
3. Choosing the Wrong Data Structure
Selecting an inappropriate data structure can significantly impact the efficiency of your solution. It’s crucial to choose the right tool for the job.
How to Avoid:
- Review common data structures and their use cases
- Consider the time and space complexity requirements of the problem
- Discuss trade-offs between different data structures with your interviewer
4. Ignoring Time and Space Complexity
Many candidates focus solely on getting a working solution without considering the efficiency of their code. Understanding and optimizing for time and space complexity is crucial in coding interviews.
How to Avoid:
- Analyze the time and space complexity of your solution
- Discuss potential optimizations with your interviewer
- Be prepared to explain the Big O notation of your algorithm
5. Not Testing Your Code
Failing to test your code thoroughly can lead to overlooking bugs and edge cases. Always allocate time for testing and debugging.
How to Avoid:
- Write test cases for various scenarios, including edge cases
- Walk through your code with sample inputs
- Consider using techniques like boundary value analysis
6. Poor Communication
Coding interviews aren’t just about writing code; they’re also about demonstrating your ability to communicate effectively. Poor communication can hinder your chances of success.
How to Avoid:
- Explain your thought process as you code
- Ask for feedback and clarification when needed
- Practice articulating your ideas clearly and concisely
7. Neglecting Code Readability
Writing clean, readable code is just as important as getting the correct solution. Messy or poorly structured code can leave a negative impression on the interviewer.
How to Avoid:
- Use meaningful variable and function names
- Follow consistent indentation and formatting
- Break down complex logic into smaller, well-named functions
8. Overlooking Edge Cases
Failing to consider and handle edge cases can result in incomplete or incorrect solutions. It’s essential to think beyond the happy path.
How to Avoid:
- Identify potential edge cases during the problem-solving phase
- Discuss edge cases with your interviewer
- Write test cases that cover various scenarios, including edge cases
9. Not Managing Time Effectively
Poor time management can lead to incomplete solutions or rushed implementations. It’s crucial to allocate your time wisely during the interview.
How to Avoid:
- Practice solving problems within time constraints
- Set mental checkpoints for different stages of the interview
- Be prepared to discuss partial solutions if time runs short
10. Failing to Ask for Help
Some candidates hesitate to ask for help when stuck, fearing it might reflect poorly on them. However, knowing when to seek assistance is a valuable skill.
How to Avoid:
- Don’t be afraid to ask for hints or clarification
- Communicate your thought process and where you’re struggling
- Show your willingness to learn and collaborate
11. Overengineering the Solution
While it’s important to write efficient code, overcomplicating the solution can be counterproductive. Strive for a balance between simplicity and effectiveness.
How to Avoid:
- Start with a simple, working solution
- Optimize only when necessary and after discussing with the interviewer
- Avoid premature optimization
12. Neglecting to Handle Errors and Exceptions
Robust code should handle potential errors and exceptions gracefully. Failing to do so can lead to unstable or unreliable solutions.
How to Avoid:
- Consider potential error scenarios in your code
- Implement appropriate error handling and exception management
- Discuss your error handling approach with the interviewer
13. Forgetting to Use Built-in Functions and Libraries
Reinventing the wheel by implementing functionality that already exists in standard libraries can waste valuable time and introduce unnecessary complexity.
How to Avoid:
- Familiarize yourself with common built-in functions and libraries
- Use standard library functions when appropriate
- Discuss the trade-offs of using built-in functions vs. custom implementations
14. Not Considering Alternative Solutions
Fixating on a single approach without exploring alternatives can limit your problem-solving capabilities. It’s important to consider multiple solutions and their trade-offs.
How to Avoid:
- Brainstorm different approaches before settling on one
- Discuss alternative solutions with your interviewer
- Be open to changing your approach if a better solution presents itself
15. Failing to Explain Your Reasoning
Simply writing code without explaining your thought process can make it difficult for the interviewer to assess your problem-solving skills.
How to Avoid:
- Verbalize your thoughts as you work through the problem
- Explain the rationale behind your decisions
- Be prepared to defend your choices and discuss alternatives
16. Ignoring Code Reusability and Modularity
Writing monolithic, tightly coupled code can make it difficult to maintain and extend. Demonstrating an understanding of modular design principles is valuable.
How to Avoid:
- Break down your solution into logical, reusable components
- Use functions to encapsulate specific behaviors
- Discuss the benefits of your modular approach with the interviewer
17. Not Practicing with a Timer
Coding under time pressure is a crucial skill in interviews. Failing to practice with time constraints can lead to poor performance during the actual interview.
How to Avoid:
- Set a timer when practicing coding problems
- Simulate interview conditions during your preparation
- Learn to manage your time effectively under pressure
18. Overlooking the Importance of Problem-Solving Techniques
Focusing solely on memorizing solutions to specific problems can leave you unprepared for novel challenges. It’s essential to develop strong problem-solving skills.
How to Avoid:
- Study and practice common problem-solving techniques (e.g., divide and conquer, dynamic programming)
- Focus on understanding the underlying principles rather than memorizing solutions
- Practice applying these techniques to a variety of problems
19. Neglecting to Review Your Code
Submitting your solution without reviewing it can lead to overlooking simple mistakes or opportunities for improvement.
How to Avoid:
- Allocate time at the end of the interview to review your code
- Look for opportunities to refactor or optimize your solution
- Double-check your logic and test cases
20. Failing to Prepare for System Design Questions
Many candidates focus solely on algorithmic problems and neglect system design questions, which are crucial for more senior positions.
How to Avoid:
- Study common system design patterns and architectures
- Practice designing scalable systems
- Be prepared to discuss trade-offs in system design decisions
21. Not Staying Calm Under Pressure
Letting nervousness or stress impact your performance can hinder your ability to showcase your skills effectively.
How to Avoid:
- Practice relaxation techniques before and during the interview
- Remember that it’s okay to take a moment to gather your thoughts
- Focus on the problem at hand rather than the pressure of the situation
22. Neglecting to Follow Up After the Interview
While not directly related to coding, failing to follow up after the interview can be a missed opportunity to leave a positive impression.
How to Avoid:
- Send a thank-you email to your interviewer(s) within 24 hours
- Reflect on the interview experience and any areas for improvement
- Express your continued interest in the position
Conclusion
Avoiding these common mistakes can significantly improve your performance in coding interviews. Remember that preparation is key, and practice makes perfect. Familiarize yourself with common algorithms, data structures, and problem-solving techniques. Utilize resources like AlgoCademy to hone your skills and prepare for technical interviews, especially those targeting major tech companies.
By focusing on clear communication, efficient problem-solving, and clean code implementation, you’ll be well-equipped to tackle even the most challenging coding interviews. Stay calm, think critically, and don’t forget to showcase your passion for programming and continuous learning. With the right preparation and mindset, you’ll be well on your way to acing your next coding interview and landing your dream job in the tech industry.
Additional Resources
To further enhance your coding interview preparation, consider exploring the following resources:
- AlgoCademy’s interactive coding tutorials and AI-powered assistance
- LeetCode and HackerRank for practicing coding problems
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- Online courses on algorithms and data structures
- Mock interview platforms to practice with real interviewers
Remember, success in coding interviews comes from a combination of technical skills, problem-solving abilities, and effective communication. By avoiding these common mistakes and continuously improving your skills, you’ll be well-prepared to tackle any coding interview with confidence.