21 Essential Tips for Giving and Receiving Feedback in Code Reviews
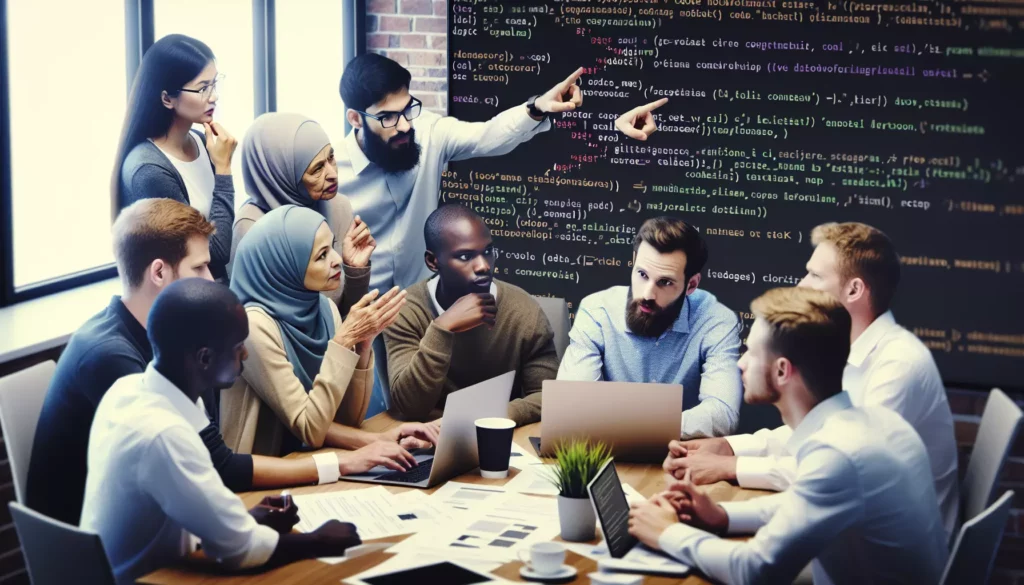
Code reviews are a crucial part of the software development process, fostering collaboration, knowledge sharing, and code quality improvement. Whether you’re a seasoned developer or just starting your coding journey, mastering the art of giving and receiving feedback during code reviews is essential for personal growth and team success. In this comprehensive guide, we’ll explore 21 valuable tips to help you navigate the code review process effectively, ensuring constructive feedback and continuous improvement in your coding practices.
Tips for Giving Feedback in Code Reviews
1. Be Respectful and Constructive
When providing feedback, always maintain a respectful and constructive tone. Remember that the goal is to improve the code and help your colleague grow, not to criticize or belittle their work. Use language that is encouraging and supportive, focusing on the code itself rather than the person who wrote it.
Example of constructive feedback:
Instead of: "This function is poorly written and inefficient."
Try: "I think we could improve the efficiency of this function by considering an alternative approach. Would you be open to exploring some options together?"
2. Start with Positive Feedback
Begin your review by highlighting the positive aspects of the code. This sets a friendly tone for the review and acknowledges the effort put into the work. Recognizing good practices encourages their continued use and helps balance any constructive criticism that follows.
Example:
"Great job on implementing the new feature! I particularly like how you've structured the main components for clarity and reusability."
3. Be Specific and Provide Examples
When suggesting improvements or pointing out issues, be as specific as possible. Provide clear examples or code snippets to illustrate your points. This helps the author understand your feedback more easily and reduces the chances of misinterpretation.
Example:
"In the calculateTotal() function, we could optimize the loop by using a reduce() method. Here's an example of how it could look:
const total = items.reduce((sum, item) => sum + item.price, 0);"
4. Explain the ‘Why’ Behind Your Suggestions
Don’t just point out what should be changed; explain why the change is beneficial. This helps the author understand the reasoning behind your suggestions and promotes learning. It also opens up the possibility for meaningful discussions about best practices.
Example:
"I suggest using a Set instead of an Array for storing unique values. This would improve the time complexity of lookups from O(n) to O(1), which is especially beneficial for larger datasets."
5. Use Questions to Promote Discussion
Frame some of your feedback as questions. This approach encourages dialogue and gives the author an opportunity to explain their reasoning. It also helps to create a collaborative atmosphere rather than a critical one.
Example:
"Have you considered using async/await here instead of promises? It might make the code more readable. What are your thoughts on that?"
6. Prioritize Your Feedback
Organize your feedback by importance. Start with major issues or architectural concerns, then move on to minor style or formatting issues. This helps the author focus on the most critical aspects first and prevents them from getting overwhelmed by a long list of small changes.
Example:
"1. High Priority: Consider refactoring the authentication logic into a separate middleware for better separation of concerns.
2. Medium Priority: The error handling in the API routes could be more consistent.
3. Low Priority: Some variable names could be more descriptive for better readability."
7. Reference Documentation and Best Practices
When suggesting changes based on best practices or coding standards, provide links to relevant documentation or style guides. This adds credibility to your feedback and serves as a learning resource for the author.
Example:
"For naming conventions in React components, we follow the Airbnb React/JSX Style Guide. You can find more details here: [link to style guide]"
8. Offer Solutions, Not Just Criticism
When pointing out issues, try to offer potential solutions or alternatives. This shows that you’re not just finding faults but actively trying to help improve the code. It also stimulates problem-solving discussions within the team.
Example:
"The current implementation might lead to performance issues with large datasets. We could consider implementing pagination or virtual scrolling to address this. I'd be happy to pair program with you to explore these options if you're interested."
9. Be Mindful of Tone in Written Communication
Remember that written feedback can sometimes be misinterpreted. Be extra careful with your word choice and consider how your comments might be perceived. Use emojis or friendly language when appropriate to convey a positive tone.
Example:
"Great effort on this complex feature! 👠I have a few suggestions that might help simplify the logic a bit. Let's discuss when you have a moment."
10. Focus on Code, Not Coding Style Preferences
Unless your team has agreed-upon coding style guidelines, avoid giving feedback on personal style preferences. Focus on code functionality, performance, and maintainability rather than debating spaces vs. tabs or where to put curly braces.
Example:
"Instead of discussing indentation preferences, let's focus on how we can improve the error handling in this function to make it more robust."
11. Acknowledge Alternative Approaches
Recognize that there can be multiple valid ways to solve a problem. If the author’s approach is different from what you would have done but still valid, acknowledge this. This promotes a culture of openness to different ideas and approaches.
Example:
"Your approach using a Map for caching is interesting and valid. I might have used a simple object, but I can see how your solution could be more efficient for larger datasets."
Tips for Receiving Feedback in Code Reviews
12. Approach Feedback with an Open Mind
When receiving feedback, maintain an open and receptive attitude. Remember that the goal of a code review is to improve the overall quality of the code and to learn from others. Try to see feedback as an opportunity for growth rather than criticism.
Example mindset:
"I'm looking forward to hearing different perspectives on my code. This is a chance to learn and improve my skills."
13. Ask Questions for Clarification
If you don’t fully understand a piece of feedback, don’t hesitate to ask for clarification. This shows that you’re engaged in the process and eager to learn. It also helps prevent misunderstandings and ensures that you can effectively implement the suggested changes.
Example:
"Could you elaborate on why you suggest using a factory pattern here? I'd like to understand the benefits in this specific context."
14. Explain Your Reasoning
If you disagree with a suggestion or have a specific reason for your implementation, explain your thought process. This can lead to productive discussions and might reveal considerations that the reviewer hadn’t thought of.
Example:
"I chose to use a switch statement here instead of if-else blocks because it improves readability with the multiple conditions we're handling. What are your thoughts on this approach?"
15. Be Grateful for the Feedback
Express appreciation for the time and effort your colleagues put into reviewing your code. This fosters a positive review culture and encourages thorough and thoughtful feedback in the future.
Example:
"Thank you for your detailed review. I really appreciate the time you've taken to help improve this code."
16. Don’t Take Feedback Personally
Remember that code reviews are about the code, not about you as a person or your abilities as a developer. Try to separate yourself emotionally from the code and view feedback objectively.
Example mindset:
"This feedback is helping me create better code. It's not a reflection of my worth as a developer."
17. Follow Up on Implemented Changes
After making changes based on feedback, follow up with the reviewer. This shows that you value their input and allows them to see how their suggestions were implemented.
Example:
"I've implemented the changes you suggested regarding error handling. Could you take another look when you have a moment?"
18. Use Code Reviews as a Learning Opportunity
Treat each code review as a chance to learn something new. Pay attention to recurring themes in the feedback you receive and use this information to improve your coding skills over time.
Example approach:
"I've noticed that I often get feedback about my variable naming. I'll focus on improving this aspect in my future code."
19. Be Open to Pair Programming
If a reviewer suggests pair programming to work through a complex issue, be open to the idea. This can be an excellent opportunity for hands-on learning and collaborative problem-solving.
Example response:
"That's a great suggestion to pair program on this tricky authentication logic. When would be a good time for you to collaborate on this?"
20. Recognize That It’s Okay to Disagree
While it’s important to be open to feedback, it’s also okay to respectfully disagree if you have strong reasons for your implementation. Engage in a constructive dialogue to reach the best solution.
Example:
"I understand your suggestion to use a different library, but I have some concerns about its long-term maintenance. Could we discuss the pros and cons of both approaches?"
21. Reflect on Feedback Patterns
Over time, look for patterns in the feedback you receive. This can help you identify areas for improvement in your coding practices and guide your professional development efforts.
Example reflection:
"I've noticed that I often receive feedback about optimizing database queries. I'll dedicate some time to studying advanced database optimization techniques."
Conclusion
Mastering the art of giving and receiving feedback in code reviews is a valuable skill that can significantly enhance your development process and team collaboration. By following these 21 tips, you’ll be well-equipped to participate in constructive, respectful, and productive code reviews.
Remember that the ultimate goal of code reviews is to improve code quality, share knowledge, and grow as developers. Approach each review with a positive mindset, whether you’re giving or receiving feedback, and you’ll find that code reviews become an invaluable part of your development workflow.
As you continue to hone your skills in this area, you’ll not only improve your own code but also contribute to a culture of continuous learning and improvement within your development team. Happy coding and reviewing!