18 Effective Techniques for Solving Backtracking Problems
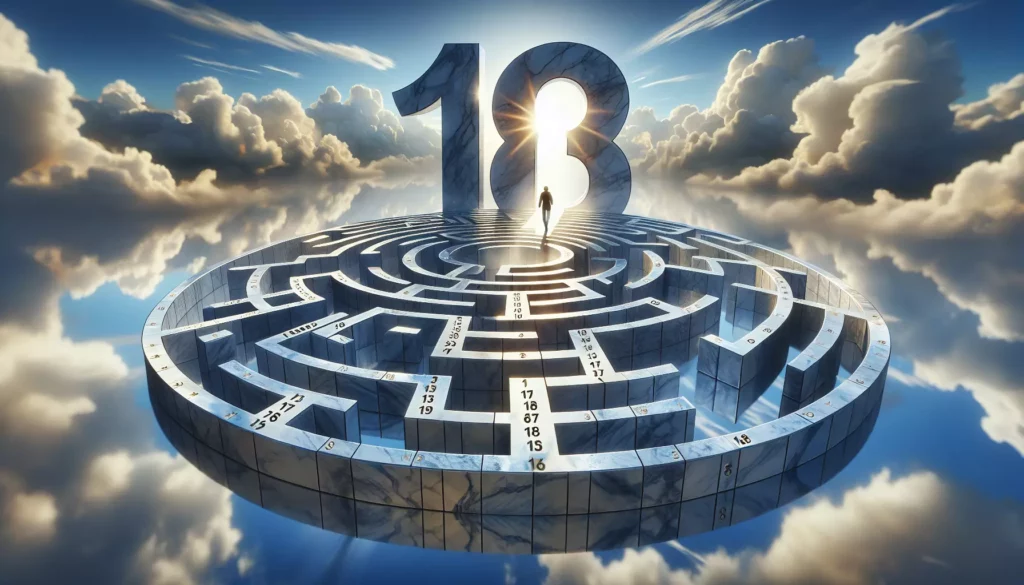
Backtracking is a powerful algorithmic technique used to solve complex problems by incrementally building candidates to the solution and abandoning those that fail to satisfy the problem’s constraints. It’s a crucial skill for any programmer, especially those preparing for technical interviews at top tech companies. In this comprehensive guide, we’ll explore 18 effective techniques for tackling backtracking problems, providing you with the tools you need to excel in your coding journey.
1. Understand the Problem Structure
Before diving into any backtracking problem, it’s essential to thoroughly understand its structure. This involves identifying the following elements:
- The solution space: What are all possible combinations or arrangements you’re exploring?
- Constraints: What conditions must be satisfied for a solution to be valid?
- The objective: What are you trying to optimize or find?
By clearly defining these aspects, you’ll be better equipped to design an effective backtracking algorithm.
2. Visualize the Problem as a Tree
Many backtracking problems can be visualized as a tree structure, where:
- The root represents the initial state
- Each node represents a partial solution
- Branches represent choices or decisions
- Leaf nodes represent complete solutions (valid or invalid)
This visualization can help you understand the problem’s recursive nature and guide your implementation.
3. Implement a Recursive Approach
Backtracking algorithms are typically implemented using recursion. The general structure looks like this:
def backtrack(partial_solution):
if is_solution(partial_solution):
process_solution(partial_solution)
return
for candidate in generate_candidates(partial_solution):
if is_valid(candidate):
add_to_solution(candidate)
backtrack(partial_solution)
remove_from_solution(candidate) # Backtrack
This recursive structure allows you to explore the solution space systematically.
4. Define Clear Base Cases
Establishing clear base cases is crucial for any recursive algorithm, including backtracking. Base cases typically include:
- When a complete solution is found
- When the current partial solution violates a constraint
- When all possibilities have been exhausted
Well-defined base cases prevent infinite recursion and ensure your algorithm terminates correctly.
5. Implement Efficient State Management
Effective state management is key to writing efficient backtracking algorithms. This involves:
- Choosing appropriate data structures to represent the current state
- Efficiently updating the state as you explore different possibilities
- Properly restoring the state when backtracking
Good state management can significantly improve your algorithm’s performance.
6. Use Pruning Techniques
Pruning is the process of eliminating branches of the search tree that are guaranteed not to lead to a valid solution. This can drastically reduce the search space and improve efficiency. Common pruning techniques include:
- Early termination: Stop exploring a branch as soon as you know it can’t lead to a valid solution
- Constraint propagation: Use problem-specific knowledge to eliminate invalid choices early
- Symmetry breaking: Avoid exploring symmetric solutions that are essentially the same
7. Implement Iterative Deepening
For problems where solutions might exist at various depths, iterative deepening can be an effective technique. It involves repeatedly running the backtracking algorithm with increasing depth limits. This approach combines the space-efficiency of depth-first search with the completeness of breadth-first search.
8. Use Memoization
In some backtracking problems, you might encounter overlapping subproblems. Memoization can help avoid redundant computations by storing the results of expensive function calls and returning the cached result when the same inputs occur again. This technique can significantly improve the time complexity of your algorithm.
9. Implement Forward Checking
Forward checking is a look-ahead technique that checks the constraints of the unassigned variables whenever a variable is assigned. This can help detect failures earlier in the search process, reducing the number of dead ends you explore.
10. Use the Minimum Remaining Values (MRV) Heuristic
The MRV heuristic suggests choosing the variable with the fewest legal values remaining. This can help reduce the branching factor of the search tree, potentially leading to faster solutions.
11. Implement Constraint Propagation
Constraint propagation involves using the problem’s constraints to reduce the domain of variables before making assignments. This can significantly reduce the search space and improve efficiency.
12. Use Bitwise Operations
For problems involving sets or boolean states, using bitwise operations can lead to more efficient implementations. Bitwise operations are typically faster than their non-bitwise counterparts and can help reduce memory usage.
13. Implement Incremental Goal Checking
Instead of checking if a complete solution satisfies all constraints only at the leaf nodes, implement incremental goal checking. This involves checking partial solutions against the problem’s constraints at each step, allowing for earlier detection of invalid paths.
14. Use Problem-Specific Heuristics
Many backtracking problems can benefit from problem-specific heuristics. These are rules or guidelines derived from the problem’s domain knowledge that can help guide the search towards promising solutions more quickly.
15. Implement Parallel Backtracking
For problems with large search spaces, consider implementing parallel backtracking. This involves dividing the search space among multiple processors or threads, potentially leading to significant speedups on multi-core systems.
16. Use Iterative Broadening
Iterative broadening is a technique where you gradually increase the branching factor of your search. This can be useful in situations where you want to find a solution quickly but don’t know the optimal branching factor in advance.
17. Implement Backjumping
Backjumping is an improvement over simple backtracking where, instead of going back to the previous decision point, you jump back to the source of the conflict. This can help avoid exploring unnecessary branches of the search tree.
18. Use Randomization
For some problems, introducing an element of randomness in your candidate selection can help avoid getting stuck in local optima. This technique can be particularly useful when combined with multiple restarts.
Conclusion
Mastering these 18 techniques for solving backtracking problems will significantly enhance your problem-solving skills and prepare you for even the most challenging technical interviews. Remember, the key to becoming proficient with backtracking is practice. Try implementing these techniques in various problems, analyze their impact, and gradually build your intuition for when and how to apply each one.
As you continue your journey in algorithmic problem-solving, keep in mind that backtracking is just one of many powerful techniques in a programmer’s toolkit. Stay curious, keep learning, and don’t hesitate to explore other algorithmic paradigms to broaden your problem-solving capabilities.
Happy coding, and may your backtracking adventures lead you to elegant and efficient solutions!