15 Fun JavaScript Projects for Beginners to Boost Your Coding Skills
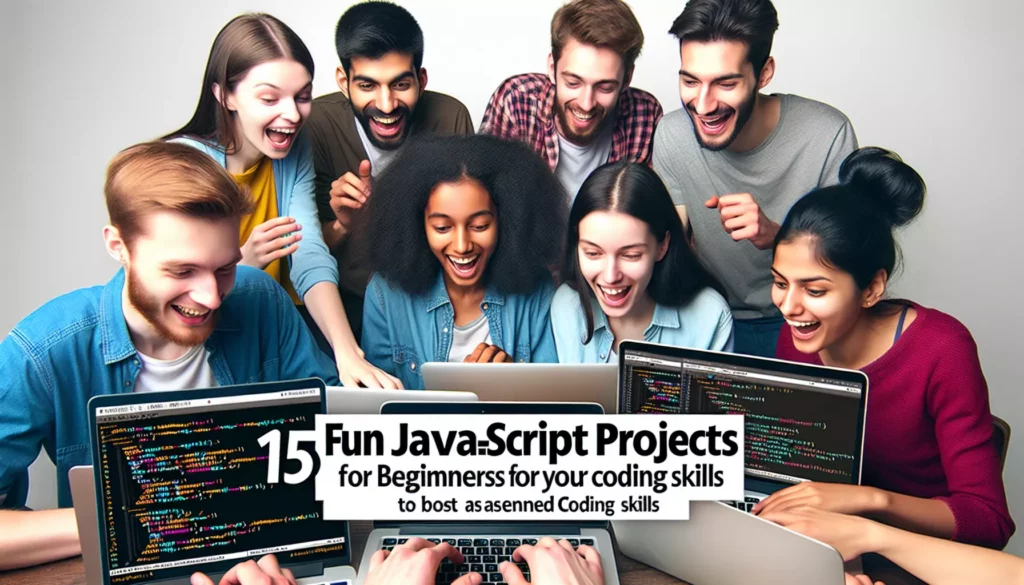
Are you new to JavaScript and looking for some exciting projects to hone your skills? You’ve come to the right place! In this comprehensive guide, we’ll explore 15 engaging JavaScript projects perfect for beginners. These projects will not only help you practice your coding skills but also allow you to create useful and interactive web applications. Let’s dive in!
1. Interactive To-Do List
Creating a to-do list is a classic beginner project that helps you understand the basics of DOM manipulation and event handling. With this project, you’ll learn how to:
- Add and remove items from a list
- Mark items as complete
- Store data in the browser’s local storage
Here’s a simple example of how you might start your to-do list project:
<!-- HTML -->
<input type="text" id="taskInput" placeholder="Enter a new task">
<button onclick="addTask()">Add Task</button>
<ul id="taskList"></ul>
<!-- JavaScript -->
<script>
function addTask() {
var input = document.getElementById("taskInput");
var task = input.value;
if (task !== "") {
var li = document.createElement("li");
li.textContent = task;
document.getElementById("taskList").appendChild(li);
input.value = "";
}
}
</script>
2. Simple Calculator
Building a calculator is an excellent way to practice working with user input, arithmetic operations, and displaying results. You can start with basic operations like addition, subtraction, multiplication, and division, then gradually add more complex features like:
- Percentage calculations
- Square root and exponents
- Memory functions
Here’s a basic structure to get you started:
<!-- HTML -->
<input type="text" id="display" readonly>
<br>
<button onclick="appendToDisplay('7')">7</button>
<button onclick="appendToDisplay('8')">8</button>
<button onclick="appendToDisplay('9')">9</button>
<button onclick="appendToDisplay('+')">+</button>
<!-- Add more buttons for other numbers and operations -->
<button onclick="calculate()">=</button>
<!-- JavaScript -->
<script>
function appendToDisplay(value) {
document.getElementById("display").value += value;
}
function calculate() {
var display = document.getElementById("display");
try {
display.value = eval(display.value);
} catch (error) {
display.value = "Error";
}
}
</script>
3. Random Quote Generator
A random quote generator is a fun project that introduces you to working with arrays and random number generation. You’ll learn how to:
- Store and access data in arrays
- Generate random numbers
- Update the DOM with new content
Here’s a simple implementation:
<!-- HTML -->
<div id="quoteDisplay"></div>
<button onclick="newQuote()">New Quote</button>
<!-- JavaScript -->
<script>
var quotes = [
"Be the change you wish to see in the world. - Mahatma Gandhi",
"Stay hungry, stay foolish. - Steve Jobs",
"The only way to do great work is to love what you do. - Steve Jobs"
];
function newQuote() {
var randomNumber = Math.floor(Math.random() * quotes.length);
document.getElementById("quoteDisplay").innerHTML = quotes[randomNumber];
}
</script>
4. Countdown Timer
Creating a countdown timer helps you understand how to work with dates and times in JavaScript. This project will teach you about:
- The Date object
- setInterval() and clearInterval() functions
- Formatting time display
Here’s a basic countdown timer:
<!-- HTML -->
<input type="datetime-local" id="endTime">
<button onclick="startTimer()">Start Countdown</button>
<div id="timer"></div>
<!-- JavaScript -->
<script>
var countdownTimer;
function startTimer() {
var endTime = new Date(document.getElementById("endTime").value).getTime();
countdownTimer = setInterval(function() {
var now = new Date().getTime();
var distance = endTime - now;
var days = Math.floor(distance / (1000 * 60 * 60 * 24));
var hours = Math.floor((distance % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60));
var minutes = Math.floor((distance % (1000 * 60 * 60)) / (1000 * 60));
var seconds = Math.floor((distance % (1000 * 60)) / 1000);
document.getElementById("timer").innerHTML = days + "d " + hours + "h " + minutes + "m " + seconds + "s ";
if (distance < 0) {
clearInterval(countdownTimer);
document.getElementById("timer").innerHTML = "EXPIRED";
}
}, 1000);
}
</script>
5. Weather App
Building a weather app introduces you to working with APIs and asynchronous JavaScript. You’ll learn about:
- Making HTTP requests using fetch()
- Parsing JSON data
- Updating the UI based on API responses
Here’s a simple weather app using the OpenWeatherMap API:
<!-- HTML -->
<input type="text" id="cityInput" placeholder="Enter city name">
<button onclick="getWeather()">Get Weather</button>
<div id="weatherInfo"></div>
<!-- JavaScript -->
<script>
const apiKey = "YOUR_API_KEY"; // Replace with your OpenWeatherMap API key
async function getWeather() {
const city = document.getElementById("cityInput").value;
const apiUrl = `https://api.openweathermap.org/data/2.5/weather?q=${city}&appid=${apiKey}&units=metric`;
try {
const response = await fetch(apiUrl);
const data = await response.json();
const weatherInfo = `
<h2>Weather in ${data.name}</h2>
<p>Temperature: ${data.main.temp}°C</p>
<p>Description: ${data.weather[0].description}</p>
<p>Humidity: ${data.main.humidity}%</p>
`;
document.getElementById("weatherInfo").innerHTML = weatherInfo;
} catch (error) {
console.error("Error fetching weather data:", error);
document.getElementById("weatherInfo").innerHTML = "Error fetching weather data";
}
}
</script>
6. Tic-Tac-Toe Game
Creating a Tic-Tac-Toe game is an excellent way to practice working with 2D arrays, game logic, and turn-based systems. This project will help you learn about:
- Manipulating 2D arrays
- Implementing game rules and win conditions
- Managing player turns
Here’s a basic implementation of Tic-Tac-Toe:
<!-- HTML -->
<div id="board"></div>
<p id="status"></p>
<!-- JavaScript -->
<script>
let currentPlayer = "X";
let board = ["", "", "", "", "", "", "", "", ""];
function createBoard() {
const boardElement = document.getElementById("board");
for (let i = 0; i < 9; i++) {
const cell = document.createElement("div");
cell.classList.add("cell");
cell.addEventListener("click", () => makeMove(i));
boardElement.appendChild(cell);
}
}
function makeMove(index) {
if (board[index] === "") {
board[index] = currentPlayer;
updateBoard();
if (checkWin()) {
document.getElementById("status").textContent = `${currentPlayer} wins!`;
} else if (board.every(cell => cell !== "")) {
document.getElementById("status").textContent = "It's a draw!";
} else {
currentPlayer = currentPlayer === "X" ? "O" : "X";
document.getElementById("status").textContent = `${currentPlayer}'s turn`;
}
}
}
function updateBoard() {
const cells = document.getElementsByClassName("cell");
for (let i = 0; i < 9; i++) {
cells[i].textContent = board[i];
}
}
function checkWin() {
const winConditions = [
[0, 1, 2], [3, 4, 5], [6, 7, 8], // Rows
[0, 3, 6], [1, 4, 7], [2, 5, 8], // Columns
[0, 4, 8], [2, 4, 6] // Diagonals
];
return winConditions.some(condition => {
return condition.every(index => board[index] === currentPlayer);
});
}
createBoard();
</script>
7. Memory Card Game
A memory card game is a fun project that helps you practice working with arrays, randomization, and game state management. You’ll learn about:
- Shuffling arrays
- Implementing game logic
- Managing timeouts for card flipping
Here’s a simple implementation of a memory card game:
<!-- HTML -->
<div id="game-board"></div>
<!-- JavaScript -->
<script>
const cards = ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H'];
let gameBoard = [...cards, ...cards];
let flippedCards = [];
let matchedPairs = 0;
function shuffleArray(array) {
for (let i = array.length - 1; i > 0; i--) {
const j = Math.floor(Math.random() * (i + 1));
[array[i], array[j]] = [array[j], array[i]];
}
}
function createBoard() {
shuffleArray(gameBoard);
const gameBoardElement = document.getElementById("game-board");
gameBoard.forEach((card, index) => {
const cardElement = document.createElement("div");
cardElement.classList.add("card");
cardElement.dataset.cardValue = card;
cardElement.dataset.index = index;
cardElement.addEventListener("click", flipCard);
gameBoardElement.appendChild(cardElement);
});
}
function flipCard() {
if (flippedCards.length < 2 && !this.classList.contains("flipped")) {
this.classList.add("flipped");
this.textContent = this.dataset.cardValue;
flippedCards.push(this);
if (flippedCards.length === 2) {
setTimeout(checkMatch, 1000);
}
}
}
function checkMatch() {
const [card1, card2] = flippedCards;
if (card1.dataset.cardValue === card2.dataset.cardValue) {
card1.removeEventListener("click", flipCard);
card2.removeEventListener("click", flipCard);
matchedPairs++;
if (matchedPairs === cards.length) {
alert("Congratulations! You won!");
}
} else {
card1.classList.remove("flipped");
card2.classList.remove("flipped");
card1.textContent = "";
card2.textContent = "";
}
flippedCards = [];
}
createBoard();
</script>
8. Hangman Game
Creating a Hangman game helps you practice working with strings, arrays, and conditional logic. This project will teach you about:
- String manipulation
- Array methods
- Game state management
Here’s a basic implementation of a Hangman game:
<!-- HTML -->
<div id="word-display"></div>
<div id="guesses"></div>
<input type="text" id="guess-input" maxlength="1">
<button onclick="makeGuess()">Guess</button>
<p id="message"></p>
<!-- JavaScript -->
<script>
const words = ["javascript", "html", "css", "python", "react", "nodejs"];
let selectedWord = words[Math.floor(Math.random() * words.length)];
let guessedLetters = [];
let remainingGuesses = 6;
function updateWordDisplay() {
const wordDisplay = document.getElementById("word-display");
wordDisplay.textContent = selectedWord
.split("")
.map(letter => guessedLetters.includes(letter) ? letter : "_")
.join(" ");
}
function updateGuesses() {
const guessesElement = document.getElementById("guesses");
guessesElement.textContent = `Guessed letters: ${guessedLetters.join(", ")}`;
}
function makeGuess() {
const guessInput = document.getElementById("guess-input");
const guess = guessInput.value.toLowerCase();
guessInput.value = "";
if (guessedLetters.includes(guess)) {
document.getElementById("message").textContent = "You already guessed that letter!";
return;
}
guessedLetters.push(guess);
if (selectedWord.includes(guess)) {
updateWordDisplay();
if (!document.getElementById("word-display").textContent.includes("_")) {
document.getElementById("message").textContent = "Congratulations! You won!";
}
} else {
remainingGuesses--;
if (remainingGuesses === 0) {
document.getElementById("message").textContent = `Game over! The word was ${selectedWord}.`;
} else {
document.getElementById("message").textContent = `Wrong guess! ${remainingGuesses} guesses left.`;
}
}
updateGuesses();
}
updateWordDisplay();
updateGuesses();
</script>
9. Pomodoro Timer
A Pomodoro timer is a productivity tool that helps users manage their work and break times. Building this project will help you learn about:
- Working with time intervals
- State management
- Audio playback in the browser
Here’s a simple implementation of a Pomodoro timer:
<!-- HTML -->
<div id="timer">25:00</div>
<button onclick="startTimer()">Start</button>
<button onclick="pauseTimer()">Pause</button>
<button onclick="resetTimer()">Reset</button>
<p id="status">Work Time</p>
<!-- JavaScript -->
<script>
let timer;
let timeLeft = 25 * 60; // 25 minutes in seconds
let isWorking = true;
let isPaused = true;
function updateDisplay() {
const minutes = Math.floor(timeLeft / 60);
const seconds = timeLeft % 60;
document.getElementById("timer").textContent =
`${minutes.toString().padStart(2, "0")}:${seconds.toString().padStart(2, "0")}`;
}
function startTimer() {
if (isPaused) {
isPaused = false;
timer = setInterval(() => {
timeLeft--;
updateDisplay();
if (timeLeft === 0) {
clearInterval(timer);
if (isWorking) {
timeLeft = 5 * 60; // 5 minute break
isWorking = false;
document.getElementById("status").textContent = "Break Time";
} else {
timeLeft = 25 * 60; // 25 minute work session
isWorking = true;
document.getElementById("status").textContent = "Work Time";
}
updateDisplay();
new Audio("https://actions.google.com/sounds/v1/alarms/beep_short.ogg").play();
}
}, 1000);
}
}
function pauseTimer() {
clearInterval(timer);
isPaused = true;
}
function resetTimer() {
clearInterval(timer);
isPaused = true;
isWorking = true;
timeLeft = 25 * 60;
updateDisplay();
document.getElementById("status").textContent = "Work Time";
}
updateDisplay();
</script>
10. Image Slider
An image slider is a common web component that allows users to browse through a collection of images. This project will help you learn about:
- DOM manipulation
- Working with arrays of objects
- Implementing smooth transitions
Here’s a basic implementation of an image slider:
<!-- HTML -->
<div id="slider-container">
<img id="slider-image" src="" alt="Slider Image">
<button onclick="prevImage()">Previous</button>
<button onclick="nextImage()">Next</button>
</div>
<!-- JavaScript -->
<script>
const images = [
{ src: "https://example.com/image1.jpg", alt: "Image 1" },
{ src: "https://example.com/image2.jpg", alt: "Image 2" },
{ src: "https://example.com/image3.jpg", alt: "Image 3" },
{ src: "https://example.com/image4.jpg", alt: "Image 4" },
{ src: "https://example.com/image5.jpg", alt: "Image 5" }
];
let currentImageIndex = 0;
function updateImage() {
const sliderImage = document.getElementById("slider-image");
sliderImage.src = images[currentImageIndex].src;
sliderImage.alt = images[currentImageIndex].alt;
}
function nextImage() {
currentImageIndex = (currentImageIndex + 1) % images.length;
updateImage();
}
function prevImage() {
currentImageIndex = (currentImageIndex - 1 + images.length) % images.length;
updateImage();
}
updateImage();
</script>
11. Quiz App
Building a quiz app is an excellent way to practice working with objects, arrays, and user input validation. This project will help you learn about:
- Storing and accessing complex data structures
- Implementing scoring systems
- Managing quiz state and progression
Here’s a simple implementation of a quiz app:
<!-- HTML -->
<div id="quiz-container">
<h2 id="question"></h2>
<div id="choices"></div>
<button onclick="submitAnswer()">Submit</button>
</div>
<p id="score"></p>
<!-- JavaScript -->
<script>
const quizData = [
{
question: "What is the capital of France?",
choices: ["London", "Berlin", "Paris", "Madrid"],
correctAnswer: 2
},
{
question: "Which planet is known as the Red Planet?",
choices: ["Venus", "Mars", "Jupiter", "Saturn"],
correctAnswer: 1
},
{
question: "What is the largest mammal?",
choices: ["Elephant", "Blue Whale", "Giraffe", "Hippopotamus"],
correctAnswer: 1
}
];
let currentQuestionIndex = 0;
let score = 0;
function loadQuestion() {
const currentQuestion = quizData[currentQuestionIndex];
document.getElementById("question").textContent = currentQuestion.question;
const choicesContainer = document.getElementById("choices");
choicesContainer.innerHTML = "";
currentQuestion.choices.forEach((choice, index) => {
const button = document.createElement("button");
button.textContent = choice;
button.onclick = () => selectChoice(index);
choicesContainer.appendChild(button);
});
}
function selectChoice(choiceIndex) {
const buttons = document.getElementById("choices").getElementsByTagName("button");
for (let button of buttons) {
button.classList.remove("selected");
}
buttons[choiceIndex].classList.add("selected");
}
function submitAnswer() {
const selectedButton = document.querySelector("#choices button.selected");
if (!selectedButton) {
alert("Please select an answer!");
return;
}
const selectedAnswer = Array.from(selectedButton.parentNode.children).indexOf(selectedButton);
if (selectedAnswer === quizData[currentQuestionIndex].correctAnswer) {
score++;
}
currentQuestionIndex++;
if (currentQuestionIndex < quizData.length) {
loadQuestion();
} else {
finishQuiz();
}
}
function finishQuiz() {
document.getElementById("quiz-container").style.display = "none";
document.getElementById("score").textContent = `You scored ${score} out of ${quizData.length}!`;
}
loadQuestion();
</script>
12. Budget Tracker
A budget tracker is a practical project that helps users manage their finances. Building this app will teach you about:
- Working with forms and user input
- Performing calculations
- Data visualization (optional)
Here’s a simple implementation of a budget tracker:
<!-- HTML -->
<h2>Budget Tracker</h2>
<form id="transaction-form">
<input type="text" id="description" placeholder="Description" required>
<input type="number" id="amount" placeholder="Amount" required>
<select id="type">
<option value="income">Income</option>
<option value="expense">Expense</option>
</select>
<button type="submit">Add Transaction</button>
</form>
<div id="balance"></div>
<div id="transaction-list"></div>
<!-- JavaScript -->
<script>
let transactions = [];
document.getElementById("transaction-form").addEventListener("submit", function(e) {
e.preventDefault();
const description = document.getElementById("description").value;
const amount = parseFloat(document.getElementById("amount").value);
const type = document.getElementById("type").value;
transactions.push({ description, amount, type });
updateTransactionList();
updateBalance();
this.reset();
});
function updateTransactionList() {
const transactionList = document.getElementById("transaction-list");
transactionList.innerHTML = "";
transactions.forEach((transaction, index) => {
const li = document.createElement("li");
li.textContent = `${transaction.description}: ${transaction.type === "income" ? "+" : "-"}$${transaction.amount}`;
const deleteButton = document.createElement("button");
deleteButton.textContent = "Delete";
deleteButton.onclick = () => deleteTransaction(index);
li.appendChild(deleteButton);
transactionList.appendChild(li);
});
}
function updateBalance() {
const balance = transactions.reduce((total, transaction) => {
return transaction.type === "income" ? total + transaction.amount : total - transaction.amount;
}, 0);
document.getElementById("balance").textContent = `Balance: $${balance.toFixed(2)}`;
}
function deleteTransaction(index) {
transactions.splice(index, 1);
updateTransactionList();
updateBalance();
}
updateBalance();
</script>
13. Rock Paper Scissors Game
The classic Rock