15 Fun and Engaging Python Projects for Beginners
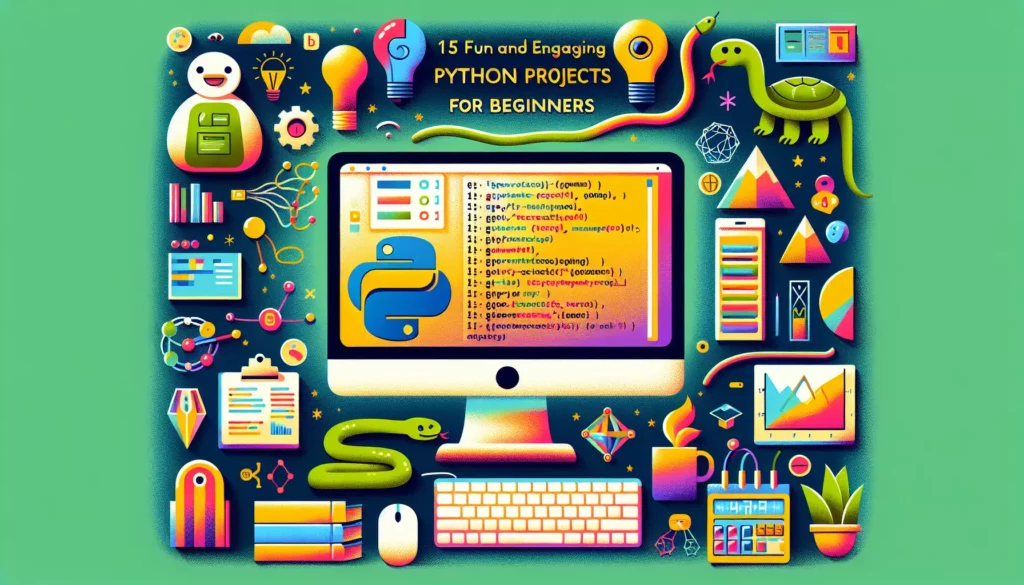
Python is an incredibly versatile and beginner-friendly programming language that has gained immense popularity in recent years. Whether you’re just starting your coding journey or looking to expand your skills, working on projects is one of the best ways to learn and apply your knowledge. In this article, we’ll explore 15 exciting Python projects that are perfect for beginners. These projects will help you build a strong foundation in Python programming while creating practical and fun applications.
1. Number Guessing Game
A number guessing game is an excellent project to start with as it introduces you to basic programming concepts such as loops, conditionals, and user input. In this game, the computer generates a random number, and the player tries to guess it within a certain number of attempts.
Here’s a simple implementation of the number guessing game:
import random
def number_guessing_game():
number = random.randint(1, 100)
attempts = 0
max_attempts = 10
print("Welcome to the Number Guessing Game!")
print(f"I'm thinking of a number between 1 and 100. You have {max_attempts} attempts to guess it.")
while attempts < max_attempts:
guess = int(input("Enter your guess: "))
attempts += 1
if guess < number:
print("Too low! Try again.")
elif guess > number:
print("Too high! Try again.")
else:
print(f"Congratulations! You guessed the number in {attempts} attempts!")
return
print(f"Sorry, you've run out of attempts. The number was {number}.")
number_guessing_game()
This project will help you practice working with random number generation, loops, conditionals, and user input.
2. Rock, Paper, Scissors Game
The classic Rock, Paper, Scissors game is another fun project for beginners. It involves creating a simple game where the user plays against the computer. This project will help you practice working with user input, random choices, and conditional statements.
Here’s a basic implementation of the Rock, Paper, Scissors game:
import random
def rock_paper_scissors():
choices = ["rock", "paper", "scissors"]
print("Welcome to Rock, Paper, Scissors!")
while True:
user_choice = input("Enter your choice (rock/paper/scissors) or 'q' to quit: ").lower()
if user_choice == 'q':
print("Thanks for playing!")
break
if user_choice not in choices:
print("Invalid choice. Please try again.")
continue
computer_choice = random.choice(choices)
print(f"You chose {user_choice}, computer chose {computer_choice}.")
if user_choice == computer_choice:
print("It's a tie!")
elif (user_choice == "rock" and computer_choice == "scissors") or \
(user_choice == "paper" and computer_choice == "rock") or \
(user_choice == "scissors" and computer_choice == "paper"):
print("You win!")
else:
print("Computer wins!")
rock_paper_scissors()
This project will help you understand how to use lists, random selection, and game logic implementation.
3. Simple Calculator
Creating a simple calculator is an excellent way to practice working with functions, user input, and basic arithmetic operations. This project will help you understand how to structure your code and handle different types of input.
Here’s a basic implementation of a simple calculator:
def add(x, y):
return x + y
def subtract(x, y):
return x - y
def multiply(x, y):
return x * y
def divide(x, y):
if y == 0:
return "Error: Division by zero"
return x / y
def calculator():
print("Simple Calculator")
print("Operations: +, -, *, /")
while True:
try:
num1 = float(input("Enter first number: "))
operator = input("Enter operator: ")
num2 = float(input("Enter second number: "))
if operator == '+':
result = add(num1, num2)
elif operator == '-':
result = subtract(num1, num2)
elif operator == '*':
result = multiply(num1, num2)
elif operator == '/':
result = divide(num1, num2)
else:
print("Invalid operator")
continue
print(f"Result: {result}")
again = input("Calculate again? (y/n): ").lower()
if again != 'y':
break
except ValueError:
print("Invalid input. Please enter numbers only.")
except Exception as e:
print(f"An error occurred: {e}")
calculator()
This project introduces you to function definitions, error handling, and basic arithmetic operations in Python.
4. To-Do List Application
A to-do list application is a practical project that helps you learn about data structures, file handling, and basic CRUD (Create, Read, Update, Delete) operations. This project will introduce you to working with lists and dictionaries, as well as reading from and writing to files.
Here’s a simple implementation of a to-do list application:
import json
def load_tasks():
try:
with open("tasks.json", "r") as file:
return json.load(file)
except FileNotFoundError:
return []
def save_tasks(tasks):
with open("tasks.json", "w") as file:
json.dump(tasks, file)
def show_tasks(tasks):
if not tasks:
print("No tasks found.")
else:
for index, task in enumerate(tasks, 1):
status = "✓" if task["completed"] else " "
print(f"{index}. [{status}] {task['description']}")
def add_task(tasks, description):
tasks.append({"description": description, "completed": False})
save_tasks(tasks)
print("Task added successfully.")
def complete_task(tasks, index):
if 1 <= index <= len(tasks):
tasks[index - 1]["completed"] = True
save_tasks(tasks)
print("Task marked as completed.")
else:
print("Invalid task index.")
def remove_task(tasks, index):
if 1 <= index <= len(tasks):
del tasks[index - 1]
save_tasks(tasks)
print("Task removed successfully.")
else:
print("Invalid task index.")
def todo_list():
tasks = load_tasks()
while True:
print("\n===== To-Do List =====")
print("1. Show tasks")
print("2. Add task")
print("3. Complete task")
print("4. Remove task")
print("5. Quit")
choice = input("Enter your choice (1-5): ")
if choice == "1":
show_tasks(tasks)
elif choice == "2":
description = input("Enter task description: ")
add_task(tasks, description)
elif choice == "3":
index = int(input("Enter task index to mark as completed: "))
complete_task(tasks, index)
elif choice == "4":
index = int(input("Enter task index to remove: "))
remove_task(tasks, index)
elif choice == "5":
print("Goodbye!")
break
else:
print("Invalid choice. Please try again.")
todo_list()
This project introduces you to working with JSON for data persistence, as well as implementing CRUD operations on a list of dictionaries.
5. Password Generator
A password generator is a useful tool that creates strong, random passwords. This project will help you practice working with strings, random number generation, and user input validation.
Here’s a simple implementation of a password generator:
import random
import string
def generate_password(length, use_letters=True, use_numbers=True, use_symbols=True):
characters = ''
if use_letters:
characters += string.ascii_letters
if use_numbers:
characters += string.digits
if use_symbols:
characters += string.punctuation
if not characters:
return "Error: At least one character type must be selected."
password = ''.join(random.choice(characters) for _ in range(length))
return password
def password_generator():
print("Welcome to the Password Generator!")
while True:
try:
length = int(input("Enter the desired password length: "))
if length < 1:
print("Password length must be at least 1 character.")
continue
use_letters = input("Include letters? (y/n): ").lower() == 'y'
use_numbers = input("Include numbers? (y/n): ").lower() == 'y'
use_symbols = input("Include symbols? (y/n): ").lower() == 'y'
password = generate_password(length, use_letters, use_numbers, use_symbols)
print(f"Generated password: {password}")
again = input("Generate another password? (y/n): ").lower()
if again != 'y':
break
except ValueError:
print("Invalid input. Please enter a valid number for the password length.")
password_generator()
This project introduces you to working with the random
and string
modules, as well as handling user input and generating random strings based on specific criteria.
6. Hangman Game
The Hangman game is a classic word-guessing game that’s perfect for practicing string manipulation, loops, and conditional statements. This project will help you improve your skills in working with lists and handling user input.
Here’s a simple implementation of the Hangman game:
import random
def hangman():
words = ["python", "programming", "computer", "science", "algorithm", "database", "network"]
word = random.choice(words)
word_letters = set(word)
alphabet = set(string.ascii_lowercase)
used_letters = set()
lives = 6
while len(word_letters) > 0 and lives > 0:
print("You have", lives, "lives left and you have used these letters: ", ' '.join(used_letters))
word_list = [letter if letter in used_letters else "_" for letter in word]
print("Current word: ", ' '.join(word_list))
user_letter = input("Guess a letter: ").lower()
if user_letter in alphabet - used_letters:
used_letters.add(user_letter)
if user_letter in word_letters:
word_letters.remove(user_letter)
else:
lives = lives - 1
print("Letter is not in the word.")
elif user_letter in used_letters:
print("You have already used that letter. Please try again.")
else:
print("Invalid character. Please try again.")
if lives == 0:
print("Sorry, you died. The word was", word)
else:
print("Congratulations! You guessed the word", word, "!!")
hangman()
This project helps you practice working with sets, list comprehensions, and game logic implementation.
7. Tic-Tac-Toe Game
Tic-Tac-Toe is a simple two-player game that’s excellent for practicing 2D list manipulation, game logic, and basic AI implementation. This project will help you understand how to work with multi-dimensional data structures and implement game rules.
Here’s a basic implementation of the Tic-Tac-Toe game:
def print_board(board):
for row in board:
print(" | ".join(row))
print("---------")
def check_winner(board, player):
# Check rows, columns, and diagonals
for i in range(3):
if all(board[i][j] == player for j in range(3)) or \
all(board[j][i] == player for j in range(3)):
return True
if all(board[i][i] == player for i in range(3)) or \
all(board[i][2-i] == player for i in range(3)):
return True
return False
def is_full(board):
return all(cell != " " for row in board for cell in row)
def tic_tac_toe():
board = [[" " for _ in range(3)] for _ in range(3)]
current_player = "X"
while True:
print_board(board)
row = int(input(f"Player {current_player}, enter row (0-2): "))
col = int(input(f"Player {current_player}, enter column (0-2): "))
if 0 <= row < 3 and 0 <= col < 3 and board[row][col] == " ":
board[row][col] = current_player
if check_winner(board, current_player):
print_board(board)
print(f"Player {current_player} wins!")
break
elif is_full(board):
print_board(board)
print("It's a tie!")
break
current_player = "O" if current_player == "X" else "X"
else:
print("Invalid move. Try again.")
tic_tac_toe()
This project introduces you to working with 2D lists, implementing game rules, and handling player turns.
8. Currency Converter
A currency converter is a practical application that helps users convert between different currencies. This project will introduce you to working with APIs, handling JSON data, and performing calculations based on real-time exchange rates.
Here’s a simple implementation of a currency converter using the ExchangeRate-API:
import requests
API_KEY = "YOUR_API_KEY_HERE" # Replace with your actual API key
BASE_URL = f"https://v6.exchangerate-api.com/v6/{API_KEY}/latest/USD"
def get_exchange_rates():
response = requests.get(BASE_URL)
data = response.json()
if data["result"] == "success":
return data["conversion_rates"]
else:
print("Error fetching exchange rates.")
return None
def convert_currency(amount, from_currency, to_currency, rates):
if from_currency not in rates or to_currency not in rates:
return "Invalid currency code"
# Convert to USD first (base currency)
usd_amount = amount / rates[from_currency]
# Convert from USD to target currency
converted_amount = usd_amount * rates[to_currency]
return round(converted_amount, 2)
def currency_converter():
rates = get_exchange_rates()
if not rates:
return
print("Welcome to the Currency Converter")
print("Available currencies:", ", ".join(rates.keys()))
while True:
try:
amount = float(input("Enter the amount to convert: "))
from_currency = input("Enter the currency to convert from: ").upper()
to_currency = input("Enter the currency to convert to: ").upper()
result = convert_currency(amount, from_currency, to_currency, rates)
print(f"{amount} {from_currency} = {result} {to_currency}")
again = input("Convert another amount? (y/n): ").lower()
if again != 'y':
break
except ValueError:
print("Invalid input. Please enter a valid number for the amount.")
currency_converter()
This project introduces you to working with external APIs, handling JSON data, and performing currency conversions based on real-time exchange rates.
9. URL Shortener
A URL shortener is a web service that creates short aliases for long URLs. This project will help you practice working with web frameworks, databases, and URL handling. For this example, we’ll use Flask as the web framework and SQLite as the database.
Here’s a basic implementation of a URL shortener:
from flask import Flask, request, redirect, render_template
import sqlite3
import string
import random
app = Flask(__name__)
def get_db():
db = sqlite3.connect('urls.db')
db.row_factory = sqlite3.Row
return db
def init_db():
db = get_db()
db.execute('CREATE TABLE IF NOT EXISTS urls (id INTEGER PRIMARY KEY AUTOINCREMENT, original_url TEXT, short_url TEXT)')
db.close()
def generate_short_url():
characters = string.ascii_letters + string.digits
return ''.join(random.choice(characters) for _ in range(6))
@app.route('/', methods=['GET', 'POST'])
def index():
if request.method == 'POST':
original_url = request.form['url']
short_url = generate_short_url()
db = get_db()
db.execute('INSERT INTO urls (original_url, short_url) VALUES (?, ?)', (original_url, short_url))
db.commit()
db.close()
return render_template('index.html', short_url=request.host_url + short_url)
return render_template('index.html')
@app.route('/<short_url>')
def redirect_to_url(short_url):
db = get_db()
result = db.execute('SELECT original_url FROM urls WHERE short_url = ?', (short_url,)).fetchone()
db.close()
if result:
return redirect(result['original_url'])
else:
return "URL not found", 404
if __name__ == '__main__':
init_db()
app.run(debug=True)
This project introduces you to web development with Flask, working with databases using SQLite, and handling URL routing and redirects.
10. Weather App
A weather app is a practical project that allows users to check the current weather conditions for a specific location. This project will help you practice working with APIs, parsing JSON data, and creating a user-friendly interface.
Here’s a simple implementation of a weather app using the OpenWeatherMap API:
import requests
API_KEY = "YOUR_API_KEY_HERE" # Replace with your actual API key
BASE_URL = "http://api.openweathermap.org/data/2.5/weather"
def get_weather(city):
params = {
"q": city,
"appid": API_KEY,
"units": "metric"
}
response = requests.get(BASE_URL, params=params)
data = response.json()
if data["cod"] == 200:
weather = {
"description": data["weather"][0]["description"],
"temperature": data["main"]["temp"],
"humidity": data["main"]["humidity"],
"wind_speed": data["wind"]["speed"]
}
return weather
else:
return None
def weather_app():
print("Welcome to the Weather App!")
while True:
city = input("Enter a city name (or 'q' to quit): ")
if city.lower() == 'q':
break
weather = get_weather(city)
if weather:
print(f"\nWeather in {city}:")
print(f"Description: {weather['description']}")
print(f"Temperature: {weather['temperature']}°C")
print(f"Humidity: {weather['humidity']}%")
print(f"Wind Speed: {weather['wind_speed']} m/s")
else:
print("City not found or there was an error fetching the weather data.")
print()
weather_app()
This project introduces you to working with weather APIs, parsing JSON data, and presenting weather information to users.
11. File Organizer
A file organizer is a useful tool that helps users sort and organize files in a directory based on their file types or other criteria. This project will help you practice working with file systems, handling different file types, and automating file organization tasks.
Here’s a simple implementation of a file organizer:
import os
import shutil
def organize_files(directory):
# Create directories for different file types
file_types = {
'Images': ['.jpg', '.jpeg', '.png', '.gif'],
'Documents': ['.pdf', '.doc', '.docx', '.txt'],
'Videos': ['.mp4', '.avi', '.mov'],
'Music': ['.mp3', '.wav', '.flac'],
'Archives': ['.zip', '.rar', '.7z'],
'Others': []
}
for folder in file_types:
if not os.path.exists(os.path.join(directory, folder)):
os.mkdir(os.path.join(directory, folder))
# Organize files
for filename in os.listdir(directory):
if os.path.isfile(os.path.join(directory, filename)):
file_ext = os.path.splitext(filename)[1].lower()
moved = False
for folder, extensions in file_types.items():
if file_ext in extensions:
shutil.move(os.path.join(directory, filename), os.path.join(directory, folder, filename))
moved = True
break
if not moved:
shutil.move(os.path.join(directory, filename), os.path.join(directory, 'Others', filename))
def file_organizer():
print("Welcome to the File Organizer!")
directory = input("Enter the directory path to organize: ")
if os.path.isdir(directory):
organize_files(directory)
print("Files organized successfully!")
else:
print("Invalid directory path. Please try again.")
file_organizer()
This project introduces you to working with file systems, handling different file types, and automating file organization tasks using the os
and shutil
modules.
12. Text-based Adventure Game
A text-based adventure game is a fun project that allows you to create an interactive story where players make choices that affect the outcome. This project will help you practice working with dictionaries, control flow, and game logic.
Here’s a simple implementation of a text-based adventure game:
def text_adventure():
def display_intro():
print("Welcome to the Mysterious Forest Adventure!")
print("You find yourself at the edge of a dark, mysterious forest.")
print("Your goal is to find the hidden treasure and make it out alive.")
print("Be careful with your choices, as they will determine your fate.")
print()
def get_choice(options):
while True:
choice = input("Enter your choice: ").lower()
if choice in options:
return choice
print("Invalid choice. Please try again.")
def forest_entrance():
print("You're standing at the entrance of the forest. There are two paths:")
print("1. A narrow, overgrown path to the left")
print("2. A wider, clearer path to the right")
choice = get_choice(['1', '2'])
if choice == '1':
return "dark_cave"
else:
return "river_crossing"
def dark_cave():
print("You follow the narrow path and come across a dark cave.")
print("1. Enter the cave")
print("2. Continue along the path")
choice = get_choice(['1', '2'])
if choice == '1':
print("You enter the cave and find the hidden treasure! Congratulations, you win!")
return "end"
else:
print("You continue along the path and get lost in the forest. Game over.")
return "end"
def river_crossing():
print("You reach a fast-flowing river with a rickety bridge.")
print("1. Try to cross the bridge")
print("2. Look for another way to cross")
choice = get_choice(['1', '2'])
if choice == '1':
print("The bridge collapses, and you fall into the river. Game over.")
return "end"
else:
return "magical_clearing"
def magical_clearing():
print("You find a magical clearing with a mysterious old man.")
print("He offers to teleport you to the treasure for a price.")
print("1. Accept his offer")
print("2. Decline and continue on your own")
choice = get_choice(['1', '2'])
if choice == '1':
print("The old man teleports you to the treasure! Congratulations, you win!")
else:
print("You continue on your own but never find the treasure. Game over.")
return "end"
display_intro()
current_scene = "forest_entrance"
while current_scene != "end":
if current_scene == "forest_entrance":
current_scene = forest_entrance()
elif current_scene == "dark_cave":
current_scene = dark_cave()
elif current_scene == "river_crossing":
current_scene = river_crossing()
elif current_scene == "magical_clearing":
current_scene = magical_clearing()
print("Thanks for playing!")
text_adventure()
This project introduces you to creating interactive narratives, managing game states, and implementing branching storylines based on user choices.
13. Basic Web Scraper
A web scraper is a tool that extracts data from websites. This project will introduce you to web scraping concepts, working with HTML parsing libraries, and data extraction techniques. We’ll use the requests
library to fetch web pages and BeautifulSoup
to parse HTML content.
Here’s a simple