15 Exciting Java Projects for Beginners to Boost Your Programming Skills
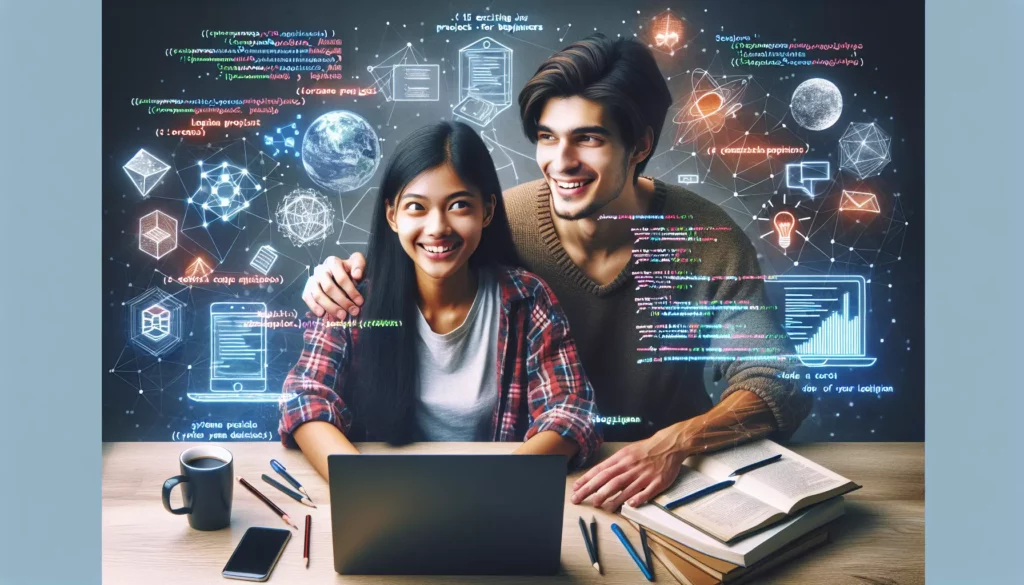
Are you a beginner programmer looking to enhance your Java skills? Look no further! In this comprehensive guide, we’ll explore 15 exciting Java projects that are perfect for beginners. These projects will not only help you practice your coding skills but also give you hands-on experience in building real-world applications. Let’s dive in and start coding!
1. Simple Calculator
Creating a calculator is an excellent way to start your Java programming journey. This project will help you understand basic arithmetic operations, user input handling, and simple GUI development.
Key Concepts:
- Basic arithmetic operations
- User input handling
- Simple GUI development (optional)
Project Steps:
- Create a class for the calculator
- Implement methods for addition, subtraction, multiplication, and division
- Design a simple console-based or GUI interface for user interaction
- Handle user input and display results
Sample Code:
import java.util.Scanner;
public class SimpleCalculator {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter first number: ");
double num1 = scanner.nextDouble();
System.out.print("Enter operator (+, -, *, /): ");
char operator = scanner.next().charAt(0);
System.out.print("Enter second number: ");
double num2 = scanner.nextDouble();
double result = 0;
switch (operator) {
case '+':
result = num1 + num2;
break;
case '-':
result = num1 - num2;
break;
case '*':
result = num1 * num2;
break;
case '/':
if (num2 != 0) {
result = num1 / num2;
} else {
System.out.println("Error: Division by zero");
return;
}
break;
default:
System.out.println("Error: Invalid operator");
return;
}
System.out.println("Result: " + result);
scanner.close();
}
}
2. Guess the Number Game
This fun project will help you practice working with random number generation, user input validation, and control flow statements.
Key Concepts:
- Random number generation
- User input validation
- Control flow statements (if-else, loops)
Project Steps:
- Generate a random number between 1 and 100
- Prompt the user to guess the number
- Provide feedback on whether the guess is too high or too low
- Keep track of the number of attempts
- End the game when the user guesses correctly
Sample Code:
import java.util.Random;
import java.util.Scanner;
public class GuessTheNumber {
public static void main(String[] args) {
Random random = new Random();
int numberToGuess = random.nextInt(100) + 1;
int attempts = 0;
boolean hasGuessedCorrectly = false;
Scanner scanner = new Scanner(System.in);
System.out.println("Welcome to Guess the Number!");
System.out.println("I'm thinking of a number between 1 and 100.");
while (!hasGuessedCorrectly) {
System.out.print("Enter your guess: ");
int guess = scanner.nextInt();
attempts++;
if (guess < numberToGuess) {
System.out.println("Too low! Try again.");
} else if (guess > numberToGuess) {
System.out.println("Too high! Try again.");
} else {
hasGuessedCorrectly = true;
System.out.println("Congratulations! You guessed the number in " + attempts + " attempts.");
}
}
scanner.close();
}
}
3. To-Do List Application
Building a to-do list application is an excellent way to practice working with data structures, file I/O, and basic CRUD (Create, Read, Update, Delete) operations.
Key Concepts:
- ArrayList and other data structures
- File I/O operations
- CRUD operations
Project Steps:
- Create a Task class to represent individual tasks
- Implement methods to add, remove, and update tasks
- Design a simple console-based interface for user interaction
- Add functionality to save and load tasks from a file
Sample Code:
import java.util.ArrayList;
import java.util.Scanner;
class Task {
private String description;
private boolean isCompleted;
public Task(String description) {
this.description = description;
this.isCompleted = false;
}
// Getters and setters
}
public class ToDoList {
private ArrayList<Task> tasks;
private Scanner scanner;
public ToDoList() {
tasks = new ArrayList<>();
scanner = new Scanner(System.in);
}
public void addTask() {
System.out.print("Enter task description: ");
String description = scanner.nextLine();
tasks.add(new Task(description));
System.out.println("Task added successfully.");
}
public void displayTasks() {
if (tasks.isEmpty()) {
System.out.println("No tasks in the list.");
} else {
for (int i = 0; i < tasks.size(); i++) {
Task task = tasks.get(i);
System.out.println((i + 1) + ". " + task.getDescription() +
(task.isCompleted() ? " (Completed)" : ""));
}
}
}
// Implement other methods like removeTask(), markTaskAsCompleted(), etc.
public static void main(String[] args) {
ToDoList todoList = new ToDoList();
// Implement menu-driven interface
}
}
4. Simple Chat Application
Creating a basic chat application will introduce you to socket programming and multi-threading in Java, which are essential concepts for network programming.
Key Concepts:
- Socket programming
- Multi-threading
- Client-server architecture
Project Steps:
- Create a server application that can handle multiple client connections
- Implement a client application that can connect to the server
- Add functionality for sending and receiving messages
- Implement a simple GUI for the client (optional)
Sample Code (Server):
import java.io.*;
import java.net.*;
import java.util.*;
public class ChatServer {
private static final int PORT = 5000;
private static HashSet<PrintWriter> writers = new HashSet<>();
public static void main(String[] args) throws Exception {
System.out.println("Chat Server is running...");
ServerSocket listener = new ServerSocket(PORT);
try {
while (true) {
new ClientHandler(listener.accept()).start();
}
} finally {
listener.close();
}
}
private static class ClientHandler extends Thread {
private Socket socket;
private PrintWriter out;
private BufferedReader in;
public ClientHandler(Socket socket) {
this.socket = socket;
}
public void run() {
try {
in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
out = new PrintWriter(socket.getOutputStream(), true);
writers.add(out);
String message;
while ((message = in.readLine()) != null) {
for (PrintWriter writer : writers) {
writer.println(message);
}
}
} catch (IOException e) {
System.out.println(e);
} finally {
if (out != null) {
writers.remove(out);
}
try {
socket.close();
} catch (IOException e) {
}
}
}
}
}
5. Tic-Tac-Toe Game
Developing a Tic-Tac-Toe game is an excellent way to practice working with 2D arrays, game logic, and basic AI concepts.
Key Concepts:
- 2D arrays
- Game logic implementation
- Basic AI (for computer player)
Project Steps:
- Create a class to represent the game board
- Implement methods to make moves and check for wins or draws
- Design a simple console-based or GUI interface for the game
- Add a computer player with basic AI (optional)
Sample Code:
import java.util.Scanner;
public class TicTacToe {
private char[][] board;
private char currentPlayer;
public TicTacToe() {
board = new char[3][3];
currentPlayer = 'X';
initializeBoard();
}
private void initializeBoard() {
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
board[i][j] = '-';
}
}
}
public boolean makeMove(int row, int col) {
if (row < 0 || row >= 3 || col < 0 || col >= 3 || board[row][col] != '-') {
return false;
}
board[row][col] = currentPlayer;
return true;
}
public boolean checkWin() {
// Check rows, columns, and diagonals
// Return true if there's a win, false otherwise
}
public boolean isBoardFull() {
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
if (board[i][j] == '-') {
return false;
}
}
}
return true;
}
public void printBoard() {
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
System.out.print(board[i][j] + " ");
}
System.out.println();
}
}
public void switchPlayer() {
currentPlayer = (currentPlayer == 'X') ? 'O' : 'X';
}
public static void main(String[] args) {
TicTacToe game = new TicTacToe();
Scanner scanner = new Scanner(System.in);
while (true) {
game.printBoard();
System.out.println("Player " + game.currentPlayer + "'s turn.");
System.out.print("Enter row (0-2) and column (0-2): ");
int row = scanner.nextInt();
int col = scanner.nextInt();
if (game.makeMove(row, col)) {
if (game.checkWin()) {
game.printBoard();
System.out.println("Player " + game.currentPlayer + " wins!");
break;
} else if (game.isBoardFull()) {
game.printBoard();
System.out.println("It's a draw!");
break;
}
game.switchPlayer();
} else {
System.out.println("Invalid move. Try again.");
}
}
scanner.close();
}
}
6. Address Book
Building an address book application will help you practice working with object-oriented programming concepts, file I/O, and data persistence.
Key Concepts:
- Object-oriented programming
- File I/O for data persistence
- Data structures (e.g., ArrayList, HashMap)
Project Steps:
- Create a Contact class to represent individual contacts
- Implement methods to add, remove, and update contacts
- Design a simple console-based or GUI interface for user interaction
- Add functionality to save and load contacts from a file
Sample Code:
import java.util.ArrayList;
import java.util.Scanner;
class Contact {
private String name;
private String phoneNumber;
private String email;
public Contact(String name, String phoneNumber, String email) {
this.name = name;
this.phoneNumber = phoneNumber;
this.email = email;
}
// Getters and setters
}
public class AddressBook {
private ArrayList<Contact> contacts;
private Scanner scanner;
public AddressBook() {
contacts = new ArrayList<>();
scanner = new Scanner(System.in);
}
public void addContact() {
System.out.print("Enter name: ");
String name = scanner.nextLine();
System.out.print("Enter phone number: ");
String phoneNumber = scanner.nextLine();
System.out.print("Enter email: ");
String email = scanner.nextLine();
contacts.add(new Contact(name, phoneNumber, email));
System.out.println("Contact added successfully.");
}
public void displayContacts() {
if (contacts.isEmpty()) {
System.out.println("No contacts in the address book.");
} else {
for (int i = 0; i < contacts.size(); i++) {
Contact contact = contacts.get(i);
System.out.println((i + 1) + ". " + contact.getName() + " - " +
contact.getPhoneNumber() + " - " + contact.getEmail());
}
}
}
// Implement other methods like removeContact(), updateContact(), searchContact(), etc.
public static void main(String[] args) {
AddressBook addressBook = new AddressBook();
// Implement menu-driven interface
}
}
7. Simple Text Editor
Creating a basic text editor will introduce you to file handling, GUI development, and text processing in Java.
Key Concepts:
- File I/O operations
- GUI development (e.g., using Swing or JavaFX)
- Text processing
Project Steps:
- Create a simple GUI with a text area and menu options
- Implement file operations (open, save, save as)
- Add basic text editing functionality (cut, copy, paste)
- Implement find and replace features (optional)
Sample Code (using Swing):
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.io.*;
public class SimpleTextEditor extends JFrame {
private JTextArea textArea;
private JFileChooser fileChooser;
public SimpleTextEditor() {
setTitle("Simple Text Editor");
setSize(800, 600);
setDefaultCloseOperation(EXIT_ON_CLOSE);
textArea = new JTextArea();
JScrollPane scrollPane = new JScrollPane(textArea);
add(scrollPane, BorderLayout.CENTER);
createMenuBar();
fileChooser = new JFileChooser();
}
private void createMenuBar() {
JMenuBar menuBar = new JMenuBar();
JMenu fileMenu = new JMenu("File");
JMenuItem openItem = new JMenuItem("Open");
JMenuItem saveItem = new JMenuItem("Save");
JMenuItem exitItem = new JMenuItem("Exit");
openItem.addActionListener(e -> openFile());
saveItem.addActionListener(e -> saveFile());
exitItem.addActionListener(e -> System.exit(0));
fileMenu.add(openItem);
fileMenu.add(saveItem);
fileMenu.addSeparator();
fileMenu.add(exitItem);
menuBar.add(fileMenu);
setJMenuBar(menuBar);
}
private void openFile() {
int returnVal = fileChooser.showOpenDialog(this);
if (returnVal == JFileChooser.APPROVE_OPTION) {
File file = fileChooser.getSelectedFile();
try (BufferedReader reader = new BufferedReader(new FileReader(file))) {
textArea.read(reader, null);
} catch (IOException ex) {
JOptionPane.showMessageDialog(this, "Error reading file", "Error", JOptionPane.ERROR_MESSAGE);
}
}
}
private void saveFile() {
int returnVal = fileChooser.showSaveDialog(this);
if (returnVal == JFileChooser.APPROVE_OPTION) {
File file = fileChooser.getSelectedFile();
try (BufferedWriter writer = new BufferedWriter(new FileWriter(file))) {
textArea.write(writer);
} catch (IOException ex) {
JOptionPane.showMessageDialog(this, "Error saving file", "Error", JOptionPane.ERROR_MESSAGE);
}
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
new SimpleTextEditor().setVisible(true);
});
}
}
8. Currency Converter
Developing a currency converter will help you practice working with APIs, JSON parsing, and basic GUI development.
Key Concepts:
- API integration
- JSON parsing
- GUI development
Project Steps:
- Create a simple GUI for user input and display
- Integrate with a currency exchange rate API (e.g., Open Exchange Rates)
- Implement currency conversion logic
- Add error handling and input validation
Sample Code:
import javax.swing.*;
import java.awt.*;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import org.json.JSONObject;
public class CurrencyConverter extends JFrame {
private JTextField amountField;
private JComboBox<String> fromCurrency, toCurrency;
private JButton convertButton;
private JLabel resultLabel;
private static final String API_KEY = "YOUR_API_KEY_HERE";
private static final String API_URL = "https://openexchangerates.org/api/latest.json?app_id=" + API_KEY;
public CurrencyConverter() {
setTitle("Currency Converter");
setSize(400, 200);
setDefaultCloseOperation(EXIT_ON_CLOSE);
setLayout(new GridLayout(4, 2));
add(new JLabel("Amount:"));
amountField = new JTextField();
add(amountField);
add(new JLabel("From:"));
fromCurrency = new JComboBox<>(new String[]{"USD", "EUR", "GBP", "JPY"});
add(fromCurrency);
add(new JLabel("To:"));
toCurrency = new JComboBox<>(new String[]{"USD", "EUR", "GBP", "JPY"});
add(toCurrency);
convertButton = new JButton("Convert");
convertButton.addActionListener(e -> convertCurrency());
add(convertButton);
resultLabel = new JLabel("Result: ");
add(resultLabel);
}
private void convertCurrency() {
try {
double amount = Double.parseDouble(amountField.getText());
String from = (String) fromCurrency.getSelectedItem();
String to = (String) toCurrency.getSelectedItem();
URL url = new URL(API_URL);
HttpURLConnection con = (HttpURLConnection) url.openConnection();
con.setRequestMethod("GET");
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuilder response = new StringBuilder();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
JSONObject jsonResponse = new JSONObject(response.toString());
JSONObject rates = jsonResponse.getJSONObject("rates");
double fromRate = rates.getDouble(from);
double toRate = rates.getDouble(to);
double result = (amount / fromRate) * toRate;
resultLabel.setText(String.format("Result: %.2f %s", result, to));
} catch (Exception ex) {
JOptionPane.showMessageDialog(this, "Error: " + ex.getMessage(), "Error", JOptionPane.ERROR_MESSAGE);
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
new CurrencyConverter().setVisible(true);
});
}
}
9. Simple Web Scraper
Building a basic web scraper will introduce you to HTTP requests, HTML parsing, and working with external libraries in Java.
Key Concepts:
- HTTP requests
- HTML parsing
- Working with external libraries (e.g., JSoup)
Project Steps:
- Set up the project with the required dependencies (e.g., JSoup)
- Implement a method to fetch HTML content from a given URL
- Parse the HTML content to extract desired information
- Display or save the extracted data
Sample Code:
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
import java.io.IOException;
public class SimpleWebScraper {
public static void main(String[] args) {
String url = "https://example.com"; // Replace with the URL you want to scrape
try {
Document doc = Jsoup.connect(url).get();
// Extract title
String title = doc.title();
System.out.println("Title: " + title);
// Extract all links
Elements links = doc.select("a[href]");
System.out.println("\nLinks:");
for (Element link : links) {
System.out.println(link.attr("href") + " - " + link.text());
}
// Extract all paragraphs
Elements paragraphs = doc.select("p");
System.out.println("\nParagraphs:");
for (Element paragraph : paragraphs) {
System.out.println(paragraph.text());
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
10. Quiz Application
Creating a quiz application will help you practice working with data structures, file I/O, and basic GUI development.
Key Concepts:
- Data structures (e.g., ArrayList, HashMap)
- File I/O for storing quiz questions
- GUI development
Project Steps:
- Create a Question class to represent individual quiz questions
- Implement methods to load questions from a file
- Design a simple GUI for displaying questions and collecting user answers
- Add functionality to score the quiz and display results
Sample Code:
import javax.swing.*;
import java.awt.*;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
class Question {
private String question;
private ArrayList<String> options;
private int correctAnswer;
public Question(String question, ArrayList<String> options, int correctAnswer) {
this.question = question;
this.options = options;
this.correctAnswer = correctAnswer;
}
// Getters
}
public class QuizApplication extends JFrame {
private ArrayList<Question> questions;
private int currentQuestionIndex;
private int score;
private JLabel questionLabel;
private JRadioButton[] optionButtons;
private JButton submitButton;
public QuizApplication() {
questions = new ArrayList<>();
currentQuestionIndex = 0;
score = 0;
setTitle("Quiz Application");
setSize(500, 300);
setDefaultCloseOperation(EXIT_ON_CLOSE);
setLayout(new BorderLayout());
loadQuestionsFromFile("questions.txt");
initializeUI();
displayQuestion();
}
private void loadQuestionsFromFile(String filename) {
try (BufferedReader br = new BufferedReader(new FileReader(filename))) {
String line;
while ((line = br.readLine()) != null) {
String question = line;
ArrayList<String> options = new ArrayList<>();
for (int i = 0; i < 4; i++) {
options.add(br.readLine());
}
int correctAnswer = Integer.parseInt(br.readLine());
questions.add(new Question(question, options, correctAnswer));
}
} catch (IOException e) {
e.printStackTrace();
}
}
private void initializeUI() {
questionLabel = new JLabel();
add(questionLabel, BorderLayout.NORTH);
JPanel optionsPanel = new JPanel(new GridLayout(4, 1));
optionButtons = new JRadioButton[4];
ButtonGroup buttonGroup = new ButtonGroup();
for (int i = 0; i < 4; i++) {
optionButtons[i] = new JRadioButton();
buttonGroup.add(optionButtons[i]);
optionsPanel.add(optionButtons[i]);
}
add(optionsPanel, BorderLayout.CENTER);
submitButton = new JButton("Submit");
submitButton.addActionListener(e -> submitAnswer());
add(submitButton, BorderLayout.SOUTH);
}
private void displayQuestion() {
if (currentQuestionIndex < questions.size()) {
Question currentQuestion = questions.get(currentQuestionIndex);
questionLabel.setText(currentQuestion.getQuestion());
for (int i = 0; i < 4; i++) {
optionButtons[i].setText(currentQuestion.getOptions().get(i));
optionButtons[i].setSelected(false);
}
} else {
showResult();
}
}
private void submitAnswer() {
Question currentQuestion = questions.get(currentQuestionIndex);
for (int i = 0; i < 4; i++) {
if (optionButtons[i].isSelected() && i == currentQuestion.getCorrectAnswer()) {
score++;
break;
}
}
currentQuestionIndex++;
displayQuestion();
}
private void showResult() {
JOptionPane.showMessageDialog(this, "Quiz completed! Your score: " + score + "/" + questions.size());
System.exit(0);
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
new QuizApplication().setVisible(true);
});
}
}
11. File Explorer
Building a simple file explorer will help you practice working with file systems, GUI development, and event handling in Java.
Key Concepts:
- File and directory operations
- GUI development (e.g., using Swing)
- Event handling