15 Exciting C++ Projects for Beginners to Boost Your Programming Skills
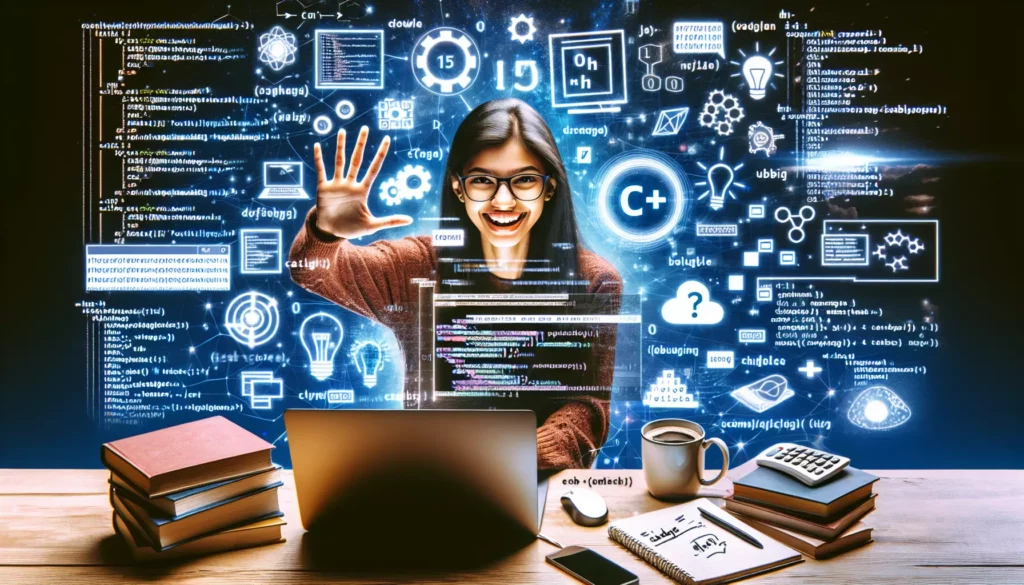
Are you a beginner in C++ programming looking to enhance your skills and build your portfolio? Look no further! In this comprehensive guide, we’ll explore 15 engaging C++ projects that are perfect for beginners. These projects will help you apply your knowledge, learn new concepts, and gain practical experience in C++ programming.
Why C++ Projects are Important for Beginners
Before we dive into the projects, let’s understand why working on C++ projects is crucial for beginners:
- Hands-on experience: Projects allow you to apply theoretical knowledge to real-world problems.
- Problem-solving skills: You’ll learn to break down complex problems into smaller, manageable tasks.
- Code organization: Projects help you understand how to structure your code effectively.
- Debugging practice: You’ll encounter and solve various errors, improving your debugging skills.
- Portfolio building: Completed projects showcase your abilities to potential employers or clients.
15 C++ Projects for Beginners
1. Calculator
A simple calculator is an excellent starting point for beginners. It helps you practice basic arithmetic operations and user input handling.
Features to implement:
- Addition, subtraction, multiplication, and division operations
- User input for numbers and operations
- Error handling for invalid inputs
- Option to perform multiple calculations
Sample code snippet:
#include <iostream>
using namespace std;
int main() {
double num1, num2;
char operation;
cout << "Enter first number: ";
cin >> num1;
cout << "Enter operation (+, -, *, /): ";
cin >> operation;
cout << "Enter second number: ";
cin >> num2;
switch(operation) {
case '+':
cout << "Result: " << num1 + num2 << endl;
break;
case '-':
cout << "Result: " << num1 - num2 << endl;
break;
case '*':
cout << "Result: " << num1 * num2 << endl;
break;
case '/':
if (num2 != 0) {
cout << "Result: " << num1 / num2 << endl;
} else {
cout << "Error: Division by zero!" << endl;
}
break;
default:
cout << "Error: Invalid operation!" << endl;
}
return 0;
}
2. Guess the Number Game
This project introduces random number generation and conditional statements. It’s a fun way to learn about user interaction and game logic.
Features to implement:
- Generate a random number within a specified range
- Allow the user to input guesses
- Provide feedback on whether the guess is too high or too low
- Keep track of the number of attempts
- Implement a scoring system based on the number of attempts
Sample code snippet:
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
int main() {
srand(time(0));
int secretNumber = rand() % 100 + 1;
int guess;
int attempts = 0;
cout << "Welcome to Guess the Number!" << endl;
cout << "I'm thinking of a number between 1 and 100." << endl;
do {
cout << "Enter your guess: ";
cin >> guess;
attempts++;
if (guess > secretNumber) {
cout << "Too high! Try again." << endl;
} else if (guess < secretNumber) {
cout << "Too low! Try again." << endl;
} else {
cout << "Congratulations! You guessed the number in " << attempts << " attempts." << endl;
}
} while (guess != secretNumber);
return 0;
}
3. To-Do List Manager
A to-do list manager is an excellent project for practicing file I/O operations and working with data structures like vectors or linked lists.
Features to implement:
- Add new tasks
- Mark tasks as completed
- Remove tasks
- Display all tasks
- Save tasks to a file
- Load tasks from a file
Sample code snippet:
#include <iostream>
#include <vector>
#include <string>
#include <fstream>
using namespace std;
struct Task {
string description;
bool completed;
};
vector<Task> tasks;
void addTask() {
string description;
cout << "Enter task description: ";
cin.ignore();
getline(cin, description);
tasks.push_back({description, false});
cout << "Task added successfully." << endl;
}
void displayTasks() {
if (tasks.empty()) {
cout << "No tasks in the list." << endl;
return;
}
for (int i = 0; i < tasks.size(); i++) {
cout << i + 1 << ". " << (tasks[i].completed ? "[X] " : "[ ] ") << tasks[i].description << endl;
}
}
int main() {
int choice;
do {
cout << "\n1. Add Task\n2. Display Tasks\n3. Exit\nEnter your choice: ";
cin >> choice;
switch (choice) {
case 1:
addTask();
break;
case 2:
displayTasks();
break;
case 3:
cout << "Exiting..." << endl;
break;
default:
cout << "Invalid choice. Please try again." << endl;
}
} while (choice != 3);
return 0;
}
4. Simple Text-Based Game
Creating a text-based game helps you practice control structures, functions, and basic game logic. It’s an engaging way to learn about user interaction and storytelling in programming.
Features to implement:
- Create a story with multiple paths and endings
- Implement player choices using if-else statements or switch cases
- Add inventory system for items collected during the game
- Implement simple combat or puzzle-solving mechanics
- Use functions to organize different game sections
Sample code snippet:
#include <iostream>
#include <string>
using namespace std;
void introduction() {
cout << "Welcome to the Text Adventure Game!" << endl;
cout << "You find yourself in a dark forest. There are two paths ahead." << endl;
}
void pathOne() {
cout << "You take the left path and encounter a giant spider!" << endl;
cout << "Do you want to fight (1) or run (2)?" << endl;
int choice;
cin >> choice;
if (choice == 1) {
cout << "You defeat the spider and find a treasure chest!" << endl;
} else {
cout << "You run away and get lost in the forest." << endl;
}
}
void pathTwo() {
cout << "You take the right path and come across a mysterious old man." << endl;
cout << "He offers you a magic potion. Do you drink it (1) or refuse (2)?" << endl;
int choice;
cin >> choice;
if (choice == 1) {
cout << "The potion gives you superpowers! You fly out of the forest." << endl;
} else {
cout << "The old man disappears, leaving you alone in the forest." << endl;
}
}
int main() {
introduction();
cout << "Which path do you choose? Left (1) or Right (2)?" << endl;
int choice;
cin >> choice;
if (choice == 1) {
pathOne();
} else {
pathTwo();
}
return 0;
}
5. Address Book
An address book project helps you practice working with classes, objects, and file handling. It’s a practical application that introduces you to basic data management concepts.
Features to implement:
- Create a Contact class with properties like name, phone number, and email
- Add new contacts
- Edit existing contacts
- Delete contacts
- Search for contacts by name or phone number
- Save contacts to a file
- Load contacts from a file
Sample code snippet:
#include <iostream>
#include <vector>
#include <string>
using namespace std;
class Contact {
public:
string name;
string phoneNumber;
string email;
Contact(string n, string p, string e) : name(n), phoneNumber(p), email(e) {}
void display() {
cout << "Name: " << name << ", Phone: " << phoneNumber << ", Email: " << email << endl;
}
};
class AddressBook {
private:
vector<Contact> contacts;
public:
void addContact() {
string name, phone, email;
cout << "Enter name: ";
cin.ignore();
getline(cin, name);
cout << "Enter phone number: ";
getline(cin, phone);
cout << "Enter email: ";
getline(cin, email);
contacts.push_back(Contact(name, phone, email));
cout << "Contact added successfully." << endl;
}
void displayContacts() {
if (contacts.empty()) {
cout << "No contacts in the address book." << endl;
return;
}
for (const auto& contact : contacts) {
contact.display();
}
}
};
int main() {
AddressBook addressBook;
int choice;
do {
cout << "\n1. Add Contact\n2. Display Contacts\n3. Exit\nEnter your choice: ";
cin >> choice;
switch (choice) {
case 1:
addressBook.addContact();
break;
case 2:
addressBook.displayContacts();
break;
case 3:
cout << "Exiting..." << endl;
break;
default:
cout << "Invalid choice. Please try again." << endl;
}
} while (choice != 3);
return 0;
}
6. Simple File Encryption/Decryption Tool
This project introduces you to basic cryptography concepts and file handling. It’s a great way to learn about bitwise operations and character manipulation.
Features to implement:
- Read content from a file
- Implement a simple encryption algorithm (e.g., XOR cipher)
- Encrypt the file content
- Save the encrypted content to a new file
- Implement decryption functionality
- Allow users to choose between encryption and decryption
Sample code snippet:
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
void encryptFile(const string& inputFile, const string& outputFile, char key) {
ifstream in(inputFile, ios::binary);
ofstream out(outputFile, ios::binary);
char ch;
while (in.get(ch)) {
ch ^= key;
out.put(ch);
}
cout << "File encrypted successfully." << endl;
}
void decryptFile(const string& inputFile, const string& outputFile, char key) {
// Decryption is the same as encryption for XOR cipher
encryptFile(inputFile, outputFile, key);
cout << "File decrypted successfully." << endl;
}
int main() {
string inputFile, outputFile;
char key;
int choice;
cout << "Enter input file name: ";
cin >> inputFile;
cout << "Enter output file name: ";
cin >> outputFile;
cout << "Enter encryption key (single character): ";
cin >> key;
cout << "Choose operation:\n1. Encrypt\n2. Decrypt\nEnter choice: ";
cin >> choice;
if (choice == 1) {
encryptFile(inputFile, outputFile, key);
} else if (choice == 2) {
decryptFile(inputFile, outputFile, key);
} else {
cout << "Invalid choice." << endl;
}
return 0;
}
7. Simple Drawing Application
A basic drawing application helps you learn about graphics programming and event handling. It’s an excellent introduction to GUI development in C++.
Features to implement:
- Create a window for drawing
- Implement basic drawing tools (e.g., line, circle, rectangle)
- Add color selection
- Implement brush size selection
- Add undo/redo functionality
- Implement save and load features for drawings
Note: This project typically requires a graphics library like SFML or SDL. Here’s a basic example using SFML:
Sample code snippet:
#include <SFML/Graphics.hpp>
#include <vector>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "Simple Drawing App");
std::vector<sf::Vertex> points;
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
if (event.type == sf::Event::MouseButtonPressed) {
if (event.mouseButton.button == sf::Mouse::Left) {
sf::Vector2i mousePos = sf::Mouse::getPosition(window);
points.push_back(sf::Vertex(sf::Vector2f(mousePos.x, mousePos.y), sf::Color::Black));
}
}
}
window.clear(sf::Color::White);
window.draw(&points[0], points.size(), sf::Points);
window.display();
}
return 0;
}
8. Basic Text Editor
Creating a simple text editor helps you practice file I/O operations, string manipulation, and basic text processing. It’s a practical project that introduces you to working with larger amounts of text data.
Features to implement:
- Open and read text files
- Display file content
- Allow text editing
- Implement save functionality
- Add basic text formatting options (e.g., uppercase, lowercase)
- Implement find and replace functionality
Sample code snippet:
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
using namespace std;
class TextEditor {
private:
vector<string> lines;
string filename;
public:
void openFile(const string& fname) {
filename = fname;
ifstream file(filename);
string line;
while (getline(file, line)) {
lines.push_back(line);
}
file.close();
}
void displayContent() {
for (int i = 0; i < lines.size(); i++) {
cout << i + 1 << ": " << lines[i] << endl;
}
}
void addLine(const string& line) {
lines.push_back(line);
}
void saveFile() {
ofstream file(filename);
for (const auto& line : lines) {
file << line << endl;
}
file.close();
cout << "File saved successfully." << endl;
}
};
int main() {
TextEditor editor;
string filename;
cout << "Enter filename to open: ";
cin >> filename;
editor.openFile(filename);
int choice;
do {
cout << "\n1. Display content\n2. Add line\n3. Save file\n4. Exit\nEnter choice: ";
cin >> choice;
switch (choice) {
case 1:
editor.displayContent();
break;
case 2: {
string line;
cout << "Enter line to add: ";
cin.ignore();
getline(cin, line);
editor.addLine(line);
break;
}
case 3:
editor.saveFile();
break;
case 4:
cout << "Exiting..." << endl;
break;
default:
cout << "Invalid choice. Please try again." << endl;
}
} while (choice != 4);
return 0;
}
9. Basic Banking System
A simple banking system project helps you practice object-oriented programming concepts, file handling, and basic data management. It’s an excellent way to learn about class relationships and data persistence.
Features to implement:
- Create Account class with properties like account number, balance, and owner name
- Implement deposit and withdrawal functions
- Add account creation functionality
- Implement account information display
- Add transaction history tracking
- Implement file-based storage for account information
Sample code snippet:
#include <iostream>
#include <vector>
#include <string>
using namespace std;
class Account {
private:
string accountNumber;
string ownerName;
double balance;
public:
Account(string accNum, string name, double initialBalance)
: accountNumber(accNum), ownerName(name), balance(initialBalance) {}
void deposit(double amount) {
if (amount > 0) {
balance += amount;
cout << "Deposit successful. New balance: " << balance << endl;
} else {
cout << "Invalid deposit amount." << endl;
}
}
void withdraw(double amount) {
if (amount > 0 && amount <= balance) {
balance -= amount;
cout << "Withdrawal successful. New balance: " << balance << endl;
} else {
cout << "Invalid withdrawal amount or insufficient funds." << endl;
}
}
void displayInfo() {
cout << "Account Number: " << accountNumber << endl;
cout << "Owner Name: " << ownerName << endl;
cout << "Balance: " << balance << endl;
}
};
class Bank {
private:
vector<Account> accounts;
public:
void createAccount() {
string accNum, name;
double initialBalance;
cout << "Enter account number: ";
cin >> accNum;
cout << "Enter owner name: ";
cin.ignore();
getline(cin, name);
cout << "Enter initial balance: ";
cin >> initialBalance;
accounts.push_back(Account(accNum, name, initialBalance));
cout << "Account created successfully." << endl;
}
void displayAllAccounts() {
for (const auto& account : accounts) {
account.displayInfo();
cout << "------------------------" << endl;
}
}
};
int main() {
Bank bank;
int choice;
do {
cout << "\n1. Create Account\n2. Display All Accounts\n3. Exit\nEnter choice: ";
cin >> choice;
switch (choice) {
case 1:
bank.createAccount();
break;
case 2:
bank.displayAllAccounts();
break;
case 3:
cout << "Exiting..." << endl;
break;
default:
cout << "Invalid choice. Please try again." << endl;
}
} while (choice != 3);
return 0;
}
10. Basic Chat Application
A simple chat application introduces you to network programming and multi-threading concepts. It’s an excellent project to learn about client-server architecture and socket programming.
Features to implement:
- Create a server that can handle multiple client connections
- Implement client-side application for sending and receiving messages
- Add user authentication
- Implement private messaging between users
- Add basic chat rooms or channels
- Implement file transfer functionality
Note: This project requires knowledge of socket programming and multi-threading. Here’s a basic example of a chat server:
Sample code snippet:
#include <iostream>
#include <string>
#include <vector>
#include <thread>
#include <mutex>
#include <WinSock2.h>
#pragma comment(lib, "ws2_32.lib")
using namespace std;
vector<SOCKET> clients;
mutex clientsMutex;
void broadcastMessage(const string& message, SOCKET sender) {
lock_guard<mutex> lock(clientsMutex);
for (auto client : clients) {
if (client != sender) {
send(client, message.c_str(), message.length(), 0);
}
}
}
void handleClient(SOCKET clientSocket) {
char buffer[4096];
while (true) {
int bytesReceived = recv(clientSocket, buffer, 4096, 0);
if (bytesReceived <= 0) {
cout << "Client disconnected." << endl;
break;
}
string message(buffer, bytesReceived);
broadcastMessage(message, clientSocket);
}
closesocket(clientSocket);
{
lock_guard<mutex> lock(clientsMutex);
clients.erase(remove(clients.begin(), clients.end(), clientSocket), clients.end());
}
}
int main() {
WSADATA wsData;
WORD ver = MAKEWORD(2, 2);
int wsOk = WSAStartup(ver, &wsData);
if (wsOk != 0) {
cerr << "Can't Initialize winsock! Quitting" << endl;
return 1;
}
SOCKET listening = socket(AF_INET, SOCK_STREAM, 0);
if (listening == INVALID_SOCKET) {
cerr << "Can't create a socket! Quitting" << endl;
return 1;
}
sockaddr_in hint;
hint.sin_family = AF_INET;
hint.sin_port = htons(54000);
hint.sin_addr.S_un.S_addr = INADDR_ANY;
bind(listening, (sockaddr*)&hint, sizeof(hint));
listen(listening, SOMAXCONN);
cout << "Server is listening..." << endl;
while (true) {
SOCKET client = accept(listening, nullptr, nullptr);
{
lock_guard<mutex> lock(clientsMutex);
clients.push_back(client);
}
thread clientThread(handleClient, client);
clientThread.detach();
}
closesocket(listening);
WSACleanup();
return 0;
}
11. Basic Image Processing Tool
An image processing tool introduces you to working with binary files and pixel manipulation. It’s an excellent project to learn about file formats and basic image processing algorithms.
Features to implement:
- Read and write image files (start with BMP format for simplicity)
- Implement basic image transformations (e.g., grayscale conversion, image inversion)
- Add simple filters (e.g., blur, sharpen)
- Implement basic drawing functions (e.g., draw line, draw circle)
- Add image resizing functionality
- Implement basic image analysis (e.g., histogram generation)
Sample code snippet (Grayscale conversion for BMP):
#include <iostream>
#include <fstream>
#include <vector>
#include <cstdint>
using namespace std;
#pragma pack(push, 1)
struct BMPHeader {
uint16_t fileType;
uint32_t fileSize;
uint16_t reserved1;
uint16_t reserved2;
uint32_t offsetData;
};
struct BMPInfoHeader {
uint32_t size;
int32_t width;
int32_t height;
uint16_t planes;
uint16_t bitCount;
uint32_t compression;
uint32_t sizeImage;
int32_t xPelsPerMeter;
int32_t yPelsPerMeter;
uint32_t clrUsed;
uint32_t clrImportant;
};
#pragma pack(pop)
void convertToGrayscale(const string& inputFile, const string& outputFile) {
ifstream input(inputFile, ios::binary);
if (!input) {
cerr << "Can't open input file." << endl;
return;
}
BMPHeader header;
BMPInfoHeader infoHeader;
input.read(reinterpret_cast<char*>(&header), sizeof(header));
input.read(reinterpret_cast<char*>(&infoHeader), sizeof(infoHeader));
if (infoHeader.bitCount != 24) {
cerr <<