13 Essential Time Management Tips for Coding Interviews
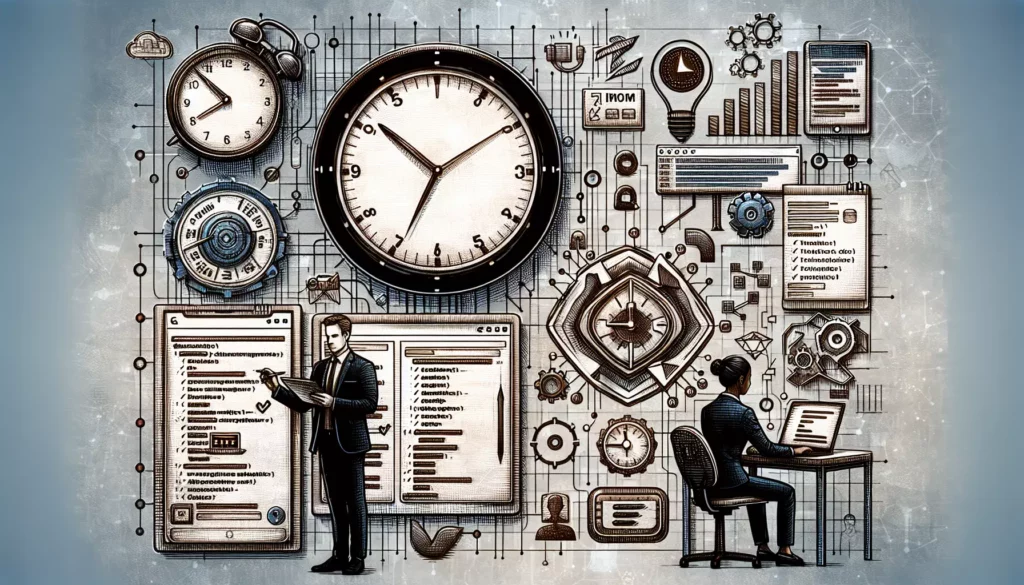
Coding interviews can be intense and time-sensitive experiences. Whether you’re aiming for a position at a FAANG company (Facebook, Amazon, Apple, Netflix, Google) or any tech firm, managing your time effectively during these interviews is crucial. At AlgoCademy, we understand the importance of time management in coding interviews and have compiled 13 essential tips to help you navigate these challenges successfully.
1. Understand the Problem Thoroughly
Before diving into coding, make sure you fully grasp the problem at hand. This initial investment of time can save you from wasting precious minutes later.
- Read the problem statement carefully, multiple times if necessary.
- Ask clarifying questions to understand constraints and edge cases.
- Confirm your understanding with the interviewer before proceeding.
Remember, a clear understanding of the problem is half the battle won. It’s better to spend an extra minute or two ensuring you’re on the right track than to waste time coding an incorrect solution.
2. Plan Your Approach
Once you understand the problem, take a moment to plan your approach. This step is often overlooked by candidates eager to start coding, but it’s crucial for time management.
- Outline your algorithm on paper or a whiteboard.
- Consider different approaches and their time/space complexities.
- Discuss your plan with the interviewer to get early feedback.
A well-thought-out plan can significantly reduce the time spent on actual coding and debugging.
3. Start with a Brute Force Solution
If you’re struggling to find an optimal solution immediately, start with a brute force approach. This serves two purposes:
- It demonstrates that you can solve the problem, even if not optimally.
- It provides a starting point for optimization.
Explain to the interviewer that you’re starting with a brute force solution and that you plan to optimize it if time permits. This shows your problem-solving process and time awareness.
4. Use Pseudocode
Before writing actual code, consider using pseudocode to outline your solution. This can help you:
- Organize your thoughts quickly.
- Spot potential issues in your logic early.
- Communicate your approach clearly to the interviewer.
Pseudocode can be particularly useful when dealing with complex algorithms or data structures.
5. Write Clean, Modular Code
While speed is important, writing clean and modular code can actually save you time in the long run. It makes your code:
- Easier to debug
- More readable for the interviewer
- Simpler to modify if needed
Use meaningful variable names, proper indentation, and break your code into functions where appropriate. This approach not only saves time but also demonstrates your coding best practices.
6. Test Your Code as You Go
Don’t wait until you’ve written the entire solution to start testing. Test your code incrementally:
- Write small sections of code and test them immediately.
- Use print statements or debuggers to verify your logic.
- Check edge cases as you implement each part of your solution.
This approach helps catch errors early, saving time on debugging later.
7. Use Built-in Functions and Data Structures Wisely
Most programming languages come with a rich set of built-in functions and data structures. Leveraging these can significantly speed up your coding process:
- Familiarize yourself with common library functions before the interview.
- Use appropriate data structures (e.g., hash maps for quick lookups).
- Don’t reinvent the wheel if a built-in solution exists.
However, make sure you understand the time and space complexities of the functions and data structures you’re using.
8. Communicate Constantly
Keep the interviewer in the loop throughout the process. This not only demonstrates your thought process but can also save time by:
- Allowing the interviewer to provide hints if you’re stuck.
- Confirming that you’re on the right track.
- Showing your problem-solving skills, even if you don’t complete the solution.
Remember, the interview is not just about the final code, but also about how you approach problems.
9. Manage Your Time Actively
Be aware of the time you have left and allocate it wisely:
- Ask the interviewer how much time you have at the start.
- Set mental checkpoints (e.g., “I should have a working solution in 20 minutes”).
- If you’re spending too much time on one part, consider moving on and coming back if time allows.
Active time management shows the interviewer that you can work efficiently under pressure.
10. Don’t Get Stuck on Syntax
In a coding interview, perfect syntax is less important than demonstrating your problem-solving skills and overall coding ability. If you can’t remember exact syntax:
- Use pseudocode or explain your intention.
- Ask the interviewer if you can assume a certain function exists.
- Focus on the logic and algorithm rather than perfect syntax.
Most interviewers are more interested in your approach than in whether you can remember every detail of the language’s syntax.
11. Optimize Your Solution
If you have time after implementing a working solution, try to optimize it:
- Look for redundant operations or unnecessary loops.
- Consider using more efficient data structures.
- Analyze the time and space complexity of your solution and discuss potential improvements.
Even if you don’t have time to implement optimizations, discussing potential improvements shows your analytical skills and understanding of efficiency.
12. Practice Time Management
Like any skill, effective time management in coding interviews improves with practice:
- Use platforms like AlgoCademy to practice coding problems under time constraints.
- Simulate interview conditions by setting a timer when you practice.
- Review your performance and identify areas where you can save time.
Regular practice not only improves your coding skills but also helps you develop a sense of how long different types of problems typically take you to solve.
13. Stay Calm and Focused
Finally, remember that staying calm and focused is crucial for effective time management:
- Take deep breaths if you feel stressed.
- If you get stuck, take a moment to step back and reassess the problem.
- Remember that it’s okay to ask for hints or clarification if you need them.
A clear, calm mind will help you manage your time more effectively and perform at your best.
Putting It All Together
Effective time management in coding interviews is a skill that combines technical knowledge, problem-solving ability, and self-awareness. By following these tips and practicing regularly, you can improve your performance and increase your chances of success in technical interviews.
Remember, the key to successful time management in coding interviews is not just about speed, but about making intelligent decisions about how to allocate your time and effort. It’s about finding the right balance between thoroughness and efficiency, between explaining your thought process and writing code, and between solving the problem at hand and demonstrating your overall coding abilities.
At AlgoCademy, we provide a platform where you can practice these skills in a realistic, time-constrained environment. Our AI-powered assistance and step-by-step guidance can help you develop not just your coding skills, but also your ability to manage time effectively in high-pressure situations.
Example: Applying Time Management in a Coding Interview
Let’s walk through a hypothetical coding interview scenario to see how these time management tips might be applied in practice.
Problem: Given an array of integers, find two numbers such that they add up to a specific target number.
Time allocated: 30 minutes
Here’s how you might approach this using our time management tips:
- Understand the problem (2 minutes): Read the problem carefully and ask clarifying questions. “Can we assume the array is sorted? Is there always a solution? Can we use additional space?”
- Plan your approach (3 minutes): Consider possible solutions. A brute force approach would be to check every pair of numbers. A more efficient solution could use a hash map.
- Communicate your plan (2 minutes): Explain your thought process to the interviewer. “I’m thinking of using a hash map to solve this in O(n) time. Would you like me to start with that, or should I explain the brute force approach first?”
- Write pseudocode (3 minutes):
function findTwoSum(nums, target): create empty hash map for each num in nums: complement = target - num if complement in hash map: return [complement, num] add num to hash map return []
- Implement the solution (10 minutes):
def find_two_sum(nums, target): num_map = {} for i, num in enumerate(nums): complement = target - num if complement in num_map: return [num_map[complement], i] num_map[num] = i return []
- Test your code (5 minutes): Run through a few test cases, including edge cases like an empty array or no solution.
- Optimize and analyze (5 minutes): Discuss the time and space complexity of your solution. Mention that while this solution is O(n) in time and space, there’s a two-pointer solution that uses O(1) space if the array is sorted.
By following this approach, you’ve demonstrated your problem-solving skills, coding ability, and time management within the 30-minute constraint. You’ve also left room for any additional questions or discussion the interviewer might want to have.
Conclusion
Mastering time management in coding interviews is a critical skill that can set you apart in the competitive tech job market. By understanding the problem thoroughly, planning your approach, communicating effectively, and practicing regularly, you can optimize your performance and showcase your true potential.
Remember, the goal is not just to solve the problem, but to demonstrate your thought process, coding skills, and ability to work efficiently under pressure. With these time management tips and consistent practice, you’ll be well-prepared to tackle any coding interview, whether it’s for a FAANG company or any other tech position you’re aiming for.
At AlgoCademy, we’re committed to helping you develop these crucial skills. Our platform offers a variety of resources, from interactive coding tutorials to AI-powered assistance, all designed to help you become a more efficient and effective coder. Keep practicing, stay focused, and approach each coding interview as an opportunity to showcase your skills and problem-solving abilities. With the right preparation and mindset, you’ll be well on your way to acing your next coding interview.