10 Exciting C++ Projects to Boost Your Programming Skills
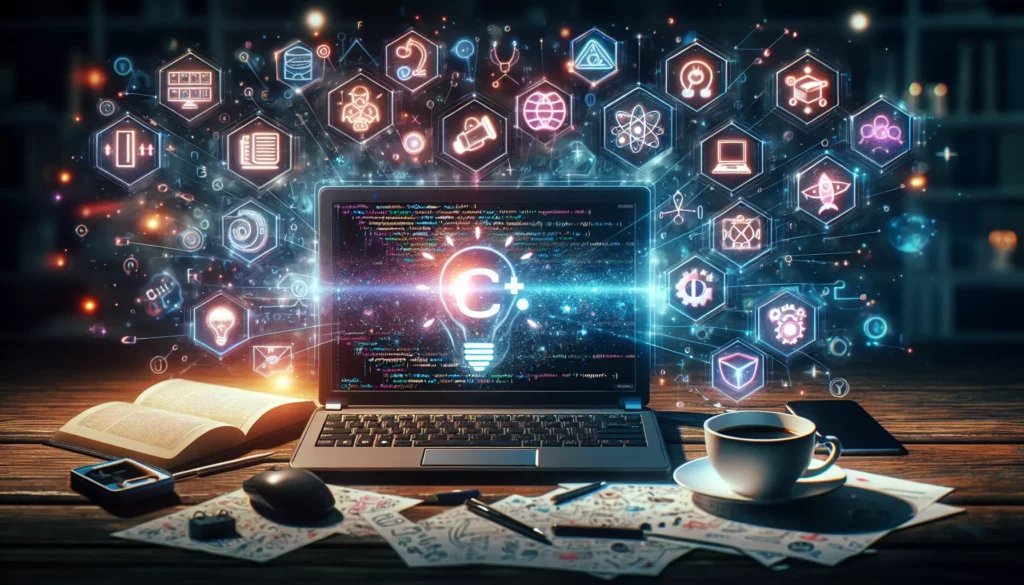
C++ is a powerful and versatile programming language that has been a cornerstone of software development for decades. Whether you’re a beginner looking to improve your skills or an experienced programmer seeking new challenges, working on projects is one of the best ways to enhance your C++ proficiency. In this article, we’ll explore 10 exciting C++ projects that will not only help you sharpen your coding abilities but also create useful applications in the process.
1. Text-Based Adventure Game
Creating a text-based adventure game is an excellent project for beginners to intermediate C++ programmers. This project will help you practice fundamental concepts such as input/output operations, conditional statements, and basic game logic.
Key Features:
- Interactive storytelling
- Multiple story paths and endings
- Inventory system
- Simple combat mechanics
Learning Outcomes:
- String manipulation
- Control flow
- Basic game design principles
Sample Code Snippet:
#include <iostream>
#include <string>
int main() {
std::string playerName;
int choice;
std::cout << "Welcome to the Text Adventure Game!" << std::endl;
std::cout << "Please enter your name: ";
std::getline(std::cin, playerName);
std::cout << "Hello, " << playerName << "! You find yourself at a crossroad." << std::endl;
std::cout << "1. Go left towards the dark forest" << std::endl;
std::cout << "2. Go right towards the misty mountains" << std::endl;
std::cout << "Enter your choice (1 or 2): ";
std::cin >> choice;
if (choice == 1) {
std::cout << "You enter the dark forest..." << std::endl;
// Continue the story
} else if (choice == 2) {
std::cout << "You head towards the misty mountains..." << std::endl;
// Continue the story
} else {
std::cout << "Invalid choice. Game over." << std::endl;
}
return 0;
}
2. Simple File Encryption/Decryption Tool
Building a file encryption and decryption tool is a great way to learn about file I/O operations and basic cryptography concepts. This project will introduce you to working with binary files and implementing simple encryption algorithms.
Key Features:
- File reading and writing
- Basic encryption algorithm (e.g., XOR cipher)
- Command-line interface
Learning Outcomes:
- File handling in C++
- Bitwise operations
- Basic cryptography concepts
Sample Code Snippet:
#include <iostream>
#include <fstream>
#include <vector>
void encryptFile(const std::string& inputFile, const std::string& outputFile, char key) {
std::ifstream inFile(inputFile, std::ios::binary);
std::ofstream outFile(outputFile, std::ios::binary);
if (!inFile || !outFile) {
std::cerr << "Error opening files!" << std::endl;
return;
}
char ch;
while (inFile.get(ch)) {
ch ^= key; // XOR encryption
outFile.put(ch);
}
inFile.close();
outFile.close();
}
int main() {
std::string inputFile = "input.txt";
std::string outputFile = "encrypted.txt";
char key = 'K'; // Encryption key
encryptFile(inputFile, outputFile, key);
std::cout << "File encrypted successfully!" << std::endl;
return 0;
}
3. Personal Finance Manager
Developing a personal finance manager is an excellent project for practicing object-oriented programming (OOP) concepts and working with data structures. This application can help users track their income, expenses, and savings goals.
Key Features:
- Add and categorize transactions
- Generate financial reports
- Set and track savings goals
- Data persistence (file or database storage)
Learning Outcomes:
- Object-oriented design
- Data structures (e.g., vectors, maps)
- File I/O or database integration
Sample Code Snippet:
#include <iostream>
#include <vector>
#include <string>
class Transaction {
public:
Transaction(double amount, const std::string& category, const std::string& description)
: m_amount(amount), m_category(category), m_description(description) {}
double getAmount() const { return m_amount; }
std::string getCategory() const { return m_category; }
std::string getDescription() const { return m_description; }
private:
double m_amount;
std::string m_category;
std::string m_description;
};
class FinanceManager {
public:
void addTransaction(const Transaction& transaction) {
m_transactions.push_back(transaction);
}
double getTotalBalance() const {
double total = 0.0;
for (const auto& transaction : m_transactions) {
total += transaction.getAmount();
}
return total;
}
private:
std::vector<Transaction> m_transactions;
};
int main() {
FinanceManager manager;
manager.addTransaction(Transaction(1000.0, "Income", "Salary"));
manager.addTransaction(Transaction(-50.0, "Food", "Groceries"));
manager.addTransaction(Transaction(-20.0, "Transportation", "Bus fare"));
std::cout << "Total balance: $" << manager.getTotalBalance() << std::endl;
return 0;
}
4. Simple Web Server
Building a simple web server is an excellent way to learn about network programming and HTTP protocols. This project will introduce you to socket programming and handling concurrent connections.
Key Features:
- Handle basic HTTP GET requests
- Serve static HTML files
- Multi-threaded connection handling
- Basic request logging
Learning Outcomes:
- Socket programming
- Multithreading
- HTTP protocol basics
Sample Code Snippet:
#include <iostream>
#include <string>
#include <cstring>
#include <sys/socket.h>
#include <netinet/in.h>
#include <unistd.h>
const int PORT = 8080;
void handleClient(int clientSocket) {
char buffer[1024] = {0};
read(clientSocket, buffer, 1024);
std::string response = "HTTP/1.1 200 OK\r\nContent-Type: text/html\r\n\r\n"
"<html><body><h1>Hello, World!</h1></body></html>";
write(clientSocket, response.c_str(), response.length());
close(clientSocket);
}
int main() {
int serverSocket = socket(AF_INET, SOCK_STREAM, 0);
if (serverSocket == -1) {
std::cerr << "Failed to create socket" << std::endl;
return 1;
}
sockaddr_in serverAddress;
serverAddress.sin_family = AF_INET;
serverAddress.sin_addr.s_addr = INADDR_ANY;
serverAddress.sin_port = htons(PORT);
if (bind(serverSocket, (struct sockaddr*)&serverAddress, sizeof(serverAddress)) < 0) {
std::cerr << "Failed to bind socket" << std::endl;
return 1;
}
if (listen(serverSocket, 3) < 0) {
std::cerr << "Failed to listen" << std::endl;
return 1;
}
std::cout << "Server listening on port " << PORT << std::endl;
while (true) {
int clientSocket = accept(serverSocket, nullptr, nullptr);
if (clientSocket < 0) {
std::cerr << "Failed to accept connection" << std::endl;
continue;
}
handleClient(clientSocket);
}
return 0;
}
5. Simple Database Management System
Creating a simple database management system (DBMS) is an excellent project for learning about data structures, file I/O, and query processing. This project will help you understand the basics of how databases work under the hood.
Key Features:
- Create, read, update, and delete (CRUD) operations
- Simple query language
- Indexing for faster lookups
- Data persistence using file storage
Learning Outcomes:
- Advanced data structures (e.g., B-trees)
- File I/O and data serialization
- Query parsing and execution
Sample Code Snippet:
#include <iostream>
#include <string>
#include <vector>
#include <map>
#include <fstream>
class Database {
public:
void createTable(const std::string& tableName, const std::vector<std::string>& columns) {
m_tables[tableName] = std::vector<std::map<std::string, std::string>>();
m_tableColumns[tableName] = columns;
}
void insert(const std::string& tableName, const std::map<std::string, std::string>& data) {
if (m_tables.find(tableName) != m_tables.end()) {
m_tables[tableName].push_back(data);
}
}
std::vector<std::map<std::string, std::string>> select(const std::string& tableName) {
if (m_tables.find(tableName) != m_tables.end()) {
return m_tables[tableName];
}
return std::vector<std::map<std::string, std::string>>();
}
void saveToFile(const std::string& filename) {
std::ofstream file(filename);
for (const auto& table : m_tables) {
file << table.first << std::endl;
for (const auto& column : m_tableColumns[table.first]) {
file << column << ",";
}
file << std::endl;
for (const auto& row : table.second) {
for (const auto& column : m_tableColumns[table.first]) {
file << row.at(column) << ",";
}
file << std::endl;
}
file << std::endl;
}
file.close();
}
private:
std::map<std::string, std::vector<std::map<std::string, std::string>>> m_tables;
std::map<std::string, std::vector<std::string>> m_tableColumns;
};
int main() {
Database db;
db.createTable("users", {"id", "name", "email"});
db.insert("users", {{"id", "1"}, {"name", "John Doe"}, {"email", "john@example.com"}});
db.insert("users", {{"id", "2"}, {"name", "Jane Smith"}, {"email", "jane@example.com"}});
auto results = db.select("users");
for (const auto& row : results) {
std::cout << "ID: " << row.at("id") << ", Name: " << row.at("name") << ", Email: " << row.at("email") << std::endl;
}
db.saveToFile("database.txt");
return 0;
}
6. Basic Compiler or Interpreter
Developing a simple compiler or interpreter for a custom programming language is an advanced project that will deepen your understanding of how programming languages work. This project involves lexical analysis, parsing, and code generation or interpretation.
Key Features:
- Lexical analyzer (tokenizer)
- Parser
- Abstract Syntax Tree (AST) generation
- Code generation or interpretation
Learning Outcomes:
- Compiler/interpreter design principles
- Parsing techniques (e.g., recursive descent parsing)
- Abstract Syntax Tree (AST) manipulation
Sample Code Snippet:
#include <iostream>
#include <string>
#include <vector>
#include <cctype>
enum class TokenType {
NUMBER,
PLUS,
MINUS,
MULTIPLY,
DIVIDE,
LPAREN,
RPAREN,
END
};
struct Token {
TokenType type;
std::string value;
};
class Lexer {
public:
Lexer(const std::string& input) : m_input(input), m_position(0) {}
Token getNextToken() {
while (m_position < m_input.length() && std::isspace(m_input[m_position])) {
m_position++;
}
if (m_position >= m_input.length()) {
return {TokenType::END, ""};
}
char currentChar = m_input[m_position];
if (std::isdigit(currentChar)) {
std::string number;
while (m_position < m_input.length() && std::isdigit(m_input[m_position])) {
number += m_input[m_position++];
}
return {TokenType::NUMBER, number};
}
m_position++;
switch (currentChar) {
case '+': return {TokenType::PLUS, "+"};
case '-': return {TokenType::MINUS, "-"};
case '*': return {TokenType::MULTIPLY, "*"};
case '/': return {TokenType::DIVIDE, "/"};
case '(': return {TokenType::LPAREN, "("};
case ')': return {TokenType::RPAREN, ")"};
default: throw std::runtime_error("Invalid character");
}
}
private:
std::string m_input;
size_t m_position;
};
int main() {
std::string input = "3 + 4 * (2 - 1)";
Lexer lexer(input);
Token token;
do {
token = lexer.getNextToken();
std::cout << "Token: " << static_cast<int>(token.type) << ", Value: " << token.value << std::endl;
} while (token.type != TokenType::END);
return 0;
}
7. Image Processing Application
Creating an image processing application is a great way to learn about working with binary data, algorithms, and computer graphics. This project will introduce you to concepts like pixel manipulation, filters, and image format handling.
Key Features:
- Load and save images in common formats (e.g., BMP, PNG)
- Apply basic filters (e.g., grayscale, blur, sharpen)
- Implement image transformations (e.g., resize, rotate)
- Basic GUI for image display and interaction
Learning Outcomes:
- Binary file handling
- Image processing algorithms
- GUI programming (e.g., using Qt or wxWidgets)
Sample Code Snippet:
#include <iostream>
#include <vector>
#include <cmath>
class Image {
public:
Image(int width, int height) : m_width(width), m_height(height) {
m_pixels.resize(width * height * 3);
}
void setPixel(int x, int y, uint8_t r, uint8_t g, uint8_t b) {
int index = (y * m_width + x) * 3;
m_pixels[index] = r;
m_pixels[index + 1] = g;
m_pixels[index + 2] = b;
}
void applyGrayscaleFilter() {
for (int y = 0; y < m_height; ++y) {
for (int x = 0; x < m_width; ++x) {
int index = (y * m_width + x) * 3;
uint8_t r = m_pixels[index];
uint8_t g = m_pixels[index + 1];
uint8_t b = m_pixels[index + 2];
uint8_t gray = static_cast<uint8_t>(0.299 * r + 0.587 * g + 0.114 * b);
m_pixels[index] = gray;
m_pixels[index + 1] = gray;
m_pixels[index + 2] = gray;
}
}
}
private:
int m_width;
int m_height;
std::vector<uint8_t> m_pixels;
};
int main() {
Image img(100, 100);
// Set some pixel values
for (int y = 0; y < 100; ++y) {
for (int x = 0; x < 100; ++x) {
img.setPixel(x, y, x % 256, y % 256, (x + y) % 256);
}
}
img.applyGrayscaleFilter();
std::cout << "Grayscale filter applied to the image." << std::endl;
return 0;
}
8. Neural Network Library
Developing a neural network library is an excellent project for learning about machine learning and artificial intelligence. This project will help you understand the mathematics behind neural networks and implement various optimization algorithms.
Key Features:
- Implement feedforward neural networks
- Backpropagation algorithm for training
- Various activation functions (e.g., sigmoid, ReLU)
- Support for different layer types (e.g., fully connected, convolutional)
Learning Outcomes:
- Neural network architecture
- Optimization algorithms
- Linear algebra and matrix operations
Sample Code Snippet:
#include <iostream>
#include <vector>
#include <cmath>
#include <random>
class NeuralNetwork {
public:
NeuralNetwork(const std::vector<int>& layerSizes) : m_layerSizes(layerSizes) {
initializeWeights();
}
std::vector<double> forward(const std::vector<double>& input) {
std::vector<double> currentLayer = input;
for (size_t i = 0; i < m_weights.size(); ++i) {
std::vector<double> nextLayer(m_layerSizes[i + 1]);
for (int j = 0; j < m_layerSizes[i + 1]; ++j) {
double sum = 0.0;
for (int k = 0; k < m_layerSizes[i]; ++k) {
sum += currentLayer[k] * m_weights[i][j][k];
}
nextLayer[j] = sigmoid(sum);
}
currentLayer = nextLayer;
}
return currentLayer;
}
private:
std::vector<int> m_layerSizes;
std::vector<std::vector<std::vector<double>>> m_weights;
void initializeWeights() {
std::random_device rd;
std::mt19937 gen(rd());
std::uniform_real_distribution<double> dis(-1.0, 1.0);
for (size_t i = 0; i < m_layerSizes.size() - 1; ++i) {
std::vector<std::vector<double>> layerWeights;
for (int j = 0; j < m_layerSizes[i + 1]; ++j) {
std::vector<double> neuronWeights;
for (int k = 0; k < m_layerSizes[i]; ++k) {
neuronWeights.push_back(dis(gen));
}
layerWeights.push_back(neuronWeights);
}
m_weights.push_back(layerWeights);
}
}
double sigmoid(double x) {
return 1.0 / (1.0 + std::exp(-x));
}
};
int main() {
NeuralNetwork nn({2, 3, 1});
std::vector<double> input = {0.5, 0.8};
std::vector<double> output = nn.forward(input);
std::cout << "Output: " << output[0] << std::endl;
return 0;
}
9. Peer-to-Peer File Sharing System
Building a peer-to-peer (P2P) file sharing system is an advanced networking project that will teach you about distributed systems, network protocols, and file transfer mechanisms. This project will help you understand how popular file-sharing applications work.
Key Features:
- Peer discovery and management
- File indexing and searching
- Chunk-based file transfer
- Basic security measures (e.g., file integrity checks)
Learning Outcomes:
- Distributed systems concepts
- Advanced network programming
- Concurrency and multithreading
Sample Code Snippet:
#include <iostream>
#include <string>
#include <vector>
#include <map>
#include <thread>
#include <mutex>
class Peer {
public:
Peer(const std::string& id, const std::string& ip, int port)
: m_id(id), m_ip(ip), m_port(port) {}
std::string getId() const { return m_id; }
std::string getIp() const { return m_ip; }
int getPort() const { return m_port; }
private:
std::string m_id;
std::string m_ip;
int m_port;
};
class P2PNetwork {
public:
void addPeer(const Peer& peer) {
std::lock_guard<std::mutex> lock(m_mutex);
m_peers[peer.getId()] = peer;
}
void removePeer(const std::string& peerId) {
std::lock_guard<std::mutex> lock(m_mutex);
m_peers.erase(peerId);
}
void addFile(const std::string& fileName, const std::string& peerId) {
std::lock_guard<std::mutex> lock(m_mutex);
m_fileIndex[fileName].push_back(peerId);
}
std::vector<std::string> searchFile(const std::string& fileName) {
std::lock_guard<std::mutex> lock(m_mutex);
if (m_fileIndex.find(fileName) != m_fileIndex.end()) {
return m_fileIndex[fileName];
}
return std::vector<std::string>();
}
private:
std::map<std::string, Peer> m_peers;
std::map<std::string, std::vector<std::string>> m_fileIndex;
std::mutex m_mutex;
};
int main() {
P2PNetwork network;
// Simulate adding peers and files
network.addPeer(Peer("peer1", "192.168.1.1", 8080));
network.addPeer(Peer("peer2", "192.168.1.2", 8080));
network.addFile("file1.txt", "peer1");
network.addFile("file2.txt", "peer2");
network.addFile("file1.txt", "peer2");
// Search for a file
std::string searchFileName = "file1.txt";
std::vector<std::string> peerIds = network.searchFile(searchFileName);
std::cout << "Peers with file '" << searchFileName << "':" << std::endl;
for (const auto& peerId : peerIds) {
std::cout << "- " << peerId << std::endl;
}
return 0;
}
10. Real-time Chat Application
Developing a real-time chat application is an excellent project for learning about network programming, concurrency, and user interface design. This project will help you understand how modern messaging applications work and handle multiple simultaneous connections.
Key Features:
- Client-server architecture
- Real-time message broadcasting
- User authentication
- Private messaging
- Basic GUI for the client
Learning Outcomes:
- Socket programming
- Multithreading and concurrency
- GUI development (e.g., using Qt or wxWidgets)
- Basic security concepts (e.g., password hashing)
Sample Code Snippet:
#include <iostream>
#include <string>
#include <vector>
#include <thread>
#include <mutex>
#include <queue>
#include <condition_variable>
class ChatServer {
public:
void start() {
std::thread serverThread(&ChatServer::serverLoop, this);
server