10 Exciting Beginner Coding Projects to Kickstart Your Programming Journey
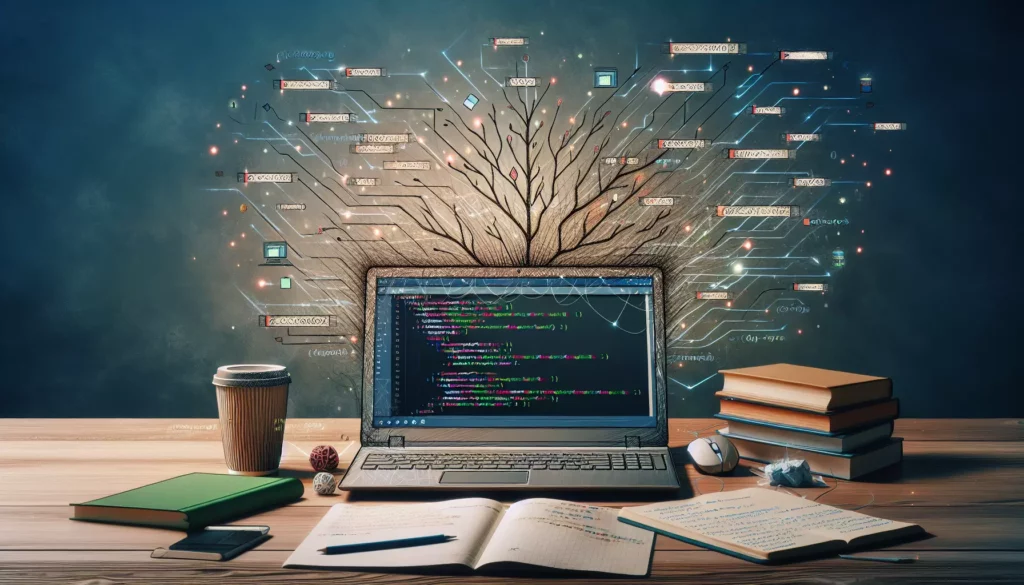
Are you new to coding and looking for some fun, practical projects to hone your skills? You’ve come to the right place! In this comprehensive guide, we’ll explore 10 beginner-friendly coding projects that will not only help you learn the basics but also give you a taste of what’s possible with programming. These projects are designed to be achievable for newcomers while still providing valuable learning experiences.
Table of Contents
- Simple Calculator
- To-Do List Application
- Quiz Game
- Weather App
- Hangman Game
- Password Generator
- Rock Paper Scissors Game
- Countdown Timer
- Currency Converter
- Tic-Tac-Toe Game
1. Simple Calculator
A calculator is an excellent first project for beginners. It introduces you to basic programming concepts like variables, user input, and arithmetic operations. You can start with a simple command-line calculator and gradually add more features as you become comfortable with the basics.
Key Features:
- Basic arithmetic operations (addition, subtraction, multiplication, division)
- User input for numbers and operations
- Display of results
Advanced Features (Optional):
- Graphical user interface (GUI)
- Scientific calculator functions (square root, exponents, etc.)
- Memory functions (store and recall values)
Sample Python Code for a Basic Calculator:
# Simple Calculator in Python
def add(x, y):
return x + y
def subtract(x, y):
return x - y
def multiply(x, y):
return x * y
def divide(x, y):
if y == 0:
return "Error! Division by zero."
return x / y
print("Select operation:")
print("1. Add")
print("2. Subtract")
print("3. Multiply")
print("4. Divide")
while True:
choice = input("Enter choice (1/2/3/4): ")
if choice in ('1', '2', '3', '4'):
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
if choice == '1':
print(num1, "+", num2, "=", add(num1, num2))
elif choice == '2':
print(num1, "-", num2, "=", subtract(num1, num2))
elif choice == '3':
print(num1, "*", num2, "=", multiply(num1, num2))
elif choice == '4':
print(num1, "/", num2, "=", divide(num1, num2))
break
else:
print("Invalid Input")
This project will help you understand basic programming concepts and how to structure a simple program. As you progress, you can add more features and even create a graphical interface using libraries like Tkinter in Python.
2. To-Do List Application
A to-do list application is a practical project that introduces you to working with data structures, file I/O, and basic CRUD (Create, Read, Update, Delete) operations. This project will help you understand how to store and manipulate data in your programs.
Key Features:
- Add new tasks
- View all tasks
- Mark tasks as complete
- Delete tasks
Advanced Features (Optional):
- Save tasks to a file for persistence
- Add due dates to tasks
- Categorize tasks
- Create a GUI for the application
Sample Python Code for a Basic To-Do List:
# Simple To-Do List in Python
tasks = []
def add_task(task):
tasks.append(task)
print(f"Task '{task}' added successfully!")
def view_tasks():
if len(tasks) == 0:
print("No tasks in the list.")
else:
for i, task in enumerate(tasks, 1):
print(f"{i}. {task}")
def complete_task(task_number):
if 1 <= task_number <= len(tasks):
task = tasks.pop(task_number - 1)
print(f"Task '{task}' marked as complete.")
else:
print("Invalid task number.")
while True:
print("\n--- To-Do List Menu ---")
print("1. Add Task")
print("2. View Tasks")
print("3. Complete Task")
print("4. Quit")
choice = input("Enter your choice (1-4): ")
if choice == '1':
task = input("Enter the task: ")
add_task(task)
elif choice == '2':
view_tasks()
elif choice == '3':
view_tasks()
task_number = int(input("Enter the task number to mark as complete: "))
complete_task(task_number)
elif choice == '4':
print("Thank you for using the To-Do List application. Goodbye!")
break
else:
print("Invalid choice. Please try again.")
This project will teach you about list manipulation, user input handling, and basic program flow control. As you expand on this project, you can learn about file handling to save tasks persistently and even create a graphical interface for a more user-friendly experience.
3. Quiz Game
Creating a quiz game is an excellent way to practice working with data structures, conditional statements, and user input. This project will help you understand how to organize and present information to users, as well as how to keep track of scores.
Key Features:
- Multiple-choice questions
- Score tracking
- Randomized question order
Advanced Features (Optional):
- Timer for each question
- Different difficulty levels
- Saving high scores
- Adding new questions through user input
Sample Python Code for a Basic Quiz Game:
# Simple Quiz Game in Python
import random
questions = [
{
"question": "What is the capital of France?",
"options": ["London", "Berlin", "Paris", "Madrid"],
"correct_answer": "Paris"
},
{
"question": "Which planet is known as the Red Planet?",
"options": ["Venus", "Mars", "Jupiter", "Saturn"],
"correct_answer": "Mars"
},
{
"question": "What is the largest mammal in the world?",
"options": ["African Elephant", "Blue Whale", "Giraffe", "Hippopotamus"],
"correct_answer": "Blue Whale"
}
]
def run_quiz(questions):
score = 0
random.shuffle(questions)
for question in questions:
print(question["question"])
for i, option in enumerate(question["options"], 1):
print(f"{i}. {option}")
user_answer = input("Enter your answer (1-4): ")
if question["options"][int(user_answer) - 1] == question["correct_answer"]:
print("Correct!")
score += 1
else:
print(f"Sorry, the correct answer was: {question['correct_answer']}")
print()
print(f"Quiz completed! Your score: {score}/{len(questions)}")
run_quiz(questions)
This project introduces you to working with dictionaries, lists, and randomization. As you expand on this project, you can add more questions, implement a scoring system, or even create a GUI for a more interactive experience.
4. Weather App
Building a weather app is an excellent way to learn about working with APIs (Application Programming Interfaces) and parsing JSON data. This project will introduce you to making HTTP requests and handling external data in your applications.
Key Features:
- Fetch weather data for a given location
- Display current temperature and conditions
- Show basic forecast information
Advanced Features (Optional):
- Graphical representation of weather data
- Multiple day forecasts
- User location detection
- Weather alerts and notifications
Sample Python Code for a Basic Weather App:
# Simple Weather App in Python using OpenWeatherMap API
# Note: You need to sign up for a free API key at OpenWeatherMap.org
import requests
API_KEY = "YOUR_API_KEY_HERE"
BASE_URL = "http://api.openweathermap.org/data/2.5/weather"
def get_weather(city):
params = {
"q": city,
"appid": API_KEY,
"units": "metric"
}
response = requests.get(BASE_URL, params=params)
data = response.json()
if response.status_code == 200:
temperature = data["main"]["temp"]
description = data["weather"][0]["description"]
humidity = data["main"]["humidity"]
print(f"Weather in {city}:")
print(f"Temperature: {temperature}°C")
print(f"Description: {description.capitalize()}")
print(f"Humidity: {humidity}%")
else:
print("Error fetching weather data. Please check your city name or API key.")
city = input("Enter a city name: ")
get_weather(city)
This project will teach you how to work with external APIs, handle JSON data, and present information to users. As you expand on this project, you can add more detailed weather information, create a graphical interface, or even integrate maps to show weather patterns.
5. Hangman Game
Hangman is a classic word-guessing game that’s perfect for practicing string manipulation, loops, and conditional statements. This project will help you understand how to work with user input and update game state based on that input.
Key Features:
- Random word selection
- Display of correct and incorrect guesses
- Limited number of attempts
Advanced Features (Optional):
- Categories for words
- Difficulty levels
- Graphical representation of the hangman
- Hint system
Sample Python Code for a Basic Hangman Game:
# Simple Hangman Game in Python
import random
words = ["python", "programming", "computer", "science", "algorithm", "database", "network"]
def choose_word():
return random.choice(words)
def play_hangman():
word = choose_word()
word_letters = set(word)
alphabet = set('abcdefghijklmnopqrstuvwxyz')
used_letters = set()
lives = 6
while len(word_letters) > 0 and lives > 0:
print("You have", lives, "lives left and you have used these letters: ", ' '.join(used_letters))
word_list = [letter if letter in used_letters else '_' for letter in word]
print("Current word: ", ' '.join(word_list))
user_letter = input("Guess a letter: ").lower()
if user_letter in alphabet - used_letters:
used_letters.add(user_letter)
if user_letter in word_letters:
word_letters.remove(user_letter)
else:
lives = lives - 1
print("Letter is not in the word.")
elif user_letter in used_letters:
print("You have already used that letter. Please try again.")
else:
print("Invalid character. Please try again.")
if lives == 0:
print("Sorry, you died. The word was", word)
else:
print("Congratulations! You guessed the word", word, "!!")
play_hangman()
This project will help you practice working with sets, lists, and string manipulation. As you expand on this project, you can add a graphical interface, implement different difficulty levels, or even create a two-player version of the game.
6. Password Generator
A password generator is a useful tool that can teach you about randomization, string manipulation, and basic security concepts. This project will help you understand how to create secure passwords and work with different types of characters.
Key Features:
- Generate random passwords of specified length
- Include a mix of uppercase, lowercase, numbers, and special characters
- Option to exclude similar-looking characters
Advanced Features (Optional):
- Password strength meter
- Generate multiple passwords at once
- Save generated passwords to a file
- GUI for easier user interaction
Sample Python Code for a Basic Password Generator:
# Simple Password Generator in Python
import random
import string
def generate_password(length, use_uppercase, use_lowercase, use_numbers, use_special):
characters = ""
if use_uppercase:
characters += string.ascii_uppercase
if use_lowercase:
characters += string.ascii_lowercase
if use_numbers:
characters += string.digits
if use_special:
characters += string.punctuation
if not characters:
return "Error: No character types selected."
password = ''.join(random.choice(characters) for _ in range(length))
return password
def main():
print("Welcome to the Password Generator!")
length = int(input("Enter the desired password length: "))
use_uppercase = input("Include uppercase letters? (y/n): ").lower() == 'y'
use_lowercase = input("Include lowercase letters? (y/n): ").lower() == 'y'
use_numbers = input("Include numbers? (y/n): ").lower() == 'y'
use_special = input("Include special characters? (y/n): ").lower() == 'y'
password = generate_password(length, use_uppercase, use_lowercase, use_numbers, use_special)
print(f"\nGenerated Password: {password}")
if __name__ == "__main__":
main()
This project introduces you to working with random selections, string operations, and user input validation. As you expand on this project, you can add features like a password strength checker, or create a graphical interface for a more user-friendly experience.
7. Rock Paper Scissors Game
Rock Paper Scissors is a simple game that’s perfect for practicing conditional statements, user input, and basic AI concepts. This project will help you understand how to implement game logic and create a computer opponent.
Key Features:
- User input for player’s choice
- Random computer choice
- Game result determination
- Score tracking
Advanced Features (Optional):
- Best of N games
- GUI with images for choices
- AI opponent that learns from player’s choices
- Multiplayer mode
Sample Python Code for a Basic Rock Paper Scissors Game:
# Simple Rock Paper Scissors Game in Python
import random
def get_user_choice():
while True:
choice = input("Enter your choice (rock/paper/scissors): ").lower()
if choice in ["rock", "paper", "scissors"]:
return choice
print("Invalid choice. Please try again.")
def get_computer_choice():
return random.choice(["rock", "paper", "scissors"])
def determine_winner(user_choice, computer_choice):
if user_choice == computer_choice:
return "It's a tie!"
elif (
(user_choice == "rock" and computer_choice == "scissors") or
(user_choice == "paper" and computer_choice == "rock") or
(user_choice == "scissors" and computer_choice == "paper")
):
return "You win!"
else:
return "Computer wins!"
def play_game():
user_score = 0
computer_score = 0
while True:
user_choice = get_user_choice()
computer_choice = get_computer_choice()
print(f"\nYou chose {user_choice}")
print(f"Computer chose {computer_choice}")
result = determine_winner(user_choice, computer_choice)
print(result)
if result == "You win!":
user_score += 1
elif result == "Computer wins!":
computer_score += 1
print(f"Score - You: {user_score}, Computer: {computer_score}")
play_again = input("Do you want to play again? (y/n): ").lower()
if play_again != "y":
break
print("\nThanks for playing!")
if __name__ == "__main__":
play_game()
This project will help you practice working with random selections, conditional statements, and game logic. As you expand on this project, you can add a graphical interface, implement different game modes, or even create an AI opponent that learns from the player’s choices.
8. Countdown Timer
A countdown timer is a practical project that introduces you to working with time in programming. This project will help you understand how to manipulate time objects, use loops, and create a simple user interface.
Key Features:
- Set a countdown duration
- Display remaining time
- Alert when time is up
Advanced Features (Optional):
- Pause and resume functionality
- Multiple timers running simultaneously
- Custom alert sounds
- GUI with a visual countdown
Sample Python Code for a Basic Countdown Timer:
# Simple Countdown Timer in Python
import time
def countdown(t):
while t:
mins, secs = divmod(t, 60)
timer = '{:02d}:{:02d}'.format(mins, secs)
print(timer, end="\r")
time.sleep(1)
t -= 1
print("Time's up!")
def main():
print("Welcome to the Countdown Timer!")
minutes = int(input("Enter the number of minutes: "))
seconds = int(input("Enter the number of seconds: "))
total_seconds = minutes * 60 + seconds
print(f"\nStarting countdown for {minutes} minutes and {seconds} seconds...")
countdown(total_seconds)
if __name__ == "__main__":
main()
This project introduces you to working with time functions, loops, and formatting output. As you expand on this project, you can add features like multiple timers, a graphical interface, or even integrate it with other applications to create a productivity tool.
9. Currency Converter
A currency converter is a practical application that teaches you how to work with APIs, handle real-time data, and perform calculations. This project will help you understand how to fetch and use external data in your programs.
Key Features:
- Convert between different currencies
- Fetch real-time exchange rates
- Support for multiple currencies
Advanced Features (Optional):
- Historical exchange rates
- Currency trend visualization
- Offline mode with cached exchange rates
- GUI for easier user interaction
Sample Python Code for a Basic Currency Converter:
# Simple Currency Converter in Python using ExchangeRate-API
# Note: You need to sign up for a free API key at https://www.exchangerate-api.com/
import requests
API_KEY = "YOUR_API_KEY_HERE"
BASE_URL = f"https://v6.exchangerate-api.com/v6/{API_KEY}/latest/USD"
def get_exchange_rates():
response = requests.get(BASE_URL)
data = response.json()
if data["result"] == "success":
return data["conversion_rates"]
else:
return None
def convert_currency(amount, from_currency, to_currency, rates):
if from_currency != "USD":
amount = amount / rates[from_currency]
return amount * rates[to_currency]
def main():
rates = get_exchange_rates()
if not rates:
print("Failed to fetch exchange rates. Please try again later.")
return
print("Available currencies:")
for currency in rates.keys():
print(currency)
from_currency = input("\nEnter the currency you want to convert from: ").upper()
to_currency = input("Enter the currency you want to convert to: ").upper()
amount = float(input("Enter the amount to convert: "))
if from_currency not in rates or to_currency not in rates:
print("Invalid currency. Please choose from the available currencies.")
return
result = convert_currency(amount, from_currency, to_currency, rates)
print(f"\n{amount} {from_currency} = {result:.2f} {to_currency}")
if __name__ == "__main__":
main()
This project will teach you how to work with APIs, handle JSON data, and perform currency calculations. As you expand on this project, you can add features like historical data analysis, a graphical interface, or even create a mobile app version of the converter.
10. Tic-Tac-Toe Game
Tic-Tac-Toe is a classic game that’s perfect for practicing 2D arrays, game logic, and basic AI concepts. This project will help you understand how to implement game rules, handle user input, and create a simple computer opponent.
Key Features:
- 2-player mode
- Display game board
- Check for win or draw conditions
Advanced Features (Optional):
- AI opponent with different difficulty levels
- GUI for the game board
- Score tracking across multiple games
- Networked multiplayer mode
Sample Python Code for a Basic Tic-Tac-Toe Game:
# Simple Tic-Tac-Toe Game in Python
def print_board(board):
for row in board:
print(" | ".join(row))
print("---------")
def check_winner(board, player):
# Check rows, columns, and diagonals
for i in range(3):
if all(board[i][j] == player for j in range(3)) or \
all(board[j][i] == player for j in range(3)):
return True
if all(board[i][i] == player for i in range(3)) or \
all(board[i][2-i] == player for i in range(3)):
return True
return False
def is_board_full(board):
return all(cell != " " for row in board for cell in row)
def play_game():
board = [[" " for _ in range(3)] for _ in range(3)]
current_player = "X"
while True:
print_board(board)
row = int(input(f"Player {current_player}, enter row (0-2): "))
col = int(input(f"Player {current_player}, enter column (0-2): "))
if board[row][col] == " ":
board[row][col] = current_player
if check_winner(board, current_player):
print_board(board)
print(f"Player {current_player} wins!")
break
elif is_board_full(board):
print_board(board)
print("It's a draw!")
break
current_player = "O" if current_player == "X" else "X"
else:
print("That cell is already occupied. Try again.")
if __name__ == "__main__":
play_game()
This project will help you practice working with 2D arrays, game logic, and user input validation. As you expand on this project, you can add a graphical interface, implement an AI opponent with different difficulty levels, or even create a networked multiplayer version of the game.
Conclusion
These 10 beginner coding projects offer a great starting point for new programmers to practice their skills and build confidence. Each project introduces different programming concepts and challenges, helping you develop a well-rounded understanding of software development.
Remember, the key to improving your coding skills is practice and persistence. Don’t be afraid to experiment, make mistakes, and learn from them. As you work through these projects, try to add your own features and improvements to make them unique.
Once you’ve completed these projects, you’ll have a solid foundation in programming basics and be ready to tackle more complex challenges. Keep exploring, learning, and building, and you’ll be well on your way to becoming a proficient programmer!