10 Essential Tips for Solving Coding Challenges Under Pressure
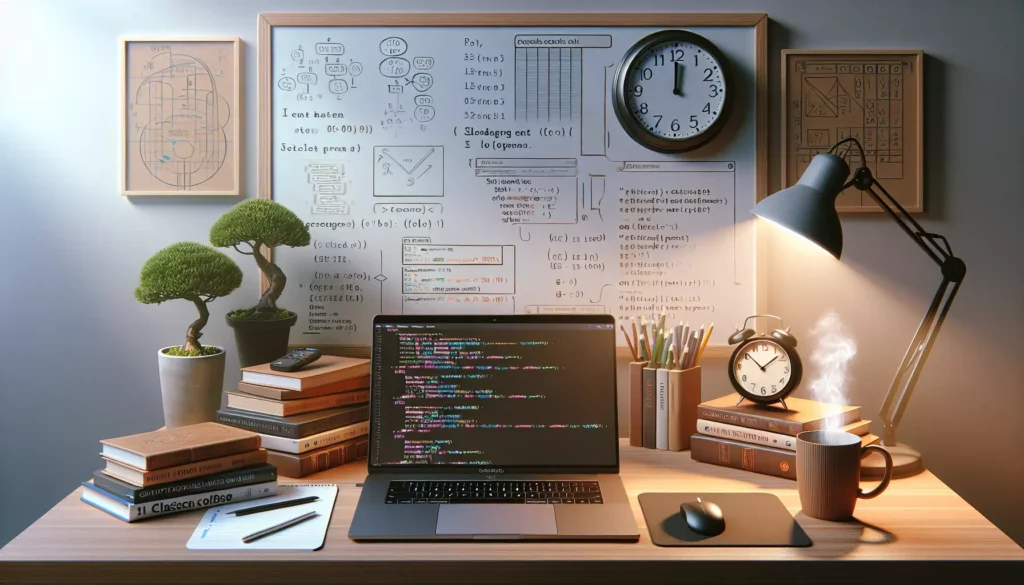
In the world of software development, the ability to solve coding challenges under pressure is a valuable skill that can make or break your career. Whether you’re preparing for a technical interview at a FAANG company (Facebook, Amazon, Apple, Netflix, Google) or participating in a coding competition, being able to think clearly and code efficiently when the clock is ticking is crucial. In this comprehensive guide, we’ll explore ten essential tips to help you excel at solving coding challenges under pressure.
1. Practice, Practice, Practice
The old adage “practice makes perfect” holds especially true when it comes to coding challenges. Regular practice is the foundation of success in any skill-based activity, and coding is no exception. Here’s how you can make the most of your practice sessions:
- Consistent Schedule: Set aside dedicated time each day or week for solving coding problems. Consistency is key to building and maintaining your skills.
- Varied Difficulty: Work on problems of varying difficulty levels. Start with easier ones to build confidence, then gradually increase the complexity.
- Timed Practice: Simulate real interview conditions by setting time limits for your practice sessions. This will help you get used to working under pressure.
- Use Platforms: Utilize coding platforms like LeetCode, HackerRank, or CodeSignal that offer a wide range of problems and track your progress.
Remember, the goal is not just to solve problems, but to understand the underlying concepts and patterns. After solving a problem, take time to reflect on your approach and explore alternative solutions.
2. Master the Fundamentals
Before diving into complex algorithms and data structures, ensure you have a solid grasp of the fundamentals. This includes:
- Basic data structures (arrays, linked lists, stacks, queues, trees, graphs)
- Common algorithms (sorting, searching, traversal)
- Time and space complexity analysis
- Basic programming constructs (loops, conditionals, functions)
Having a strong foundation will make it easier to tackle more advanced problems and recognize patterns in new challenges. Spend time reviewing these concepts regularly, and try to understand how they can be applied in different scenarios.
3. Develop a Problem-Solving Framework
Having a structured approach to problem-solving can significantly reduce stress and improve your efficiency during coding challenges. Here’s a framework you can follow:
- Understand the Problem: Read the problem statement carefully. Identify the inputs, outputs, and constraints.
- Ask Clarifying Questions: Don’t hesitate to ask for clarification if anything is unclear. In an interview setting, this shows your attention to detail and communication skills.
- Break Down the Problem: Divide the problem into smaller, manageable sub-problems.
- Plan Your Approach: Outline your solution before coding. Consider different algorithms and data structures that could be useful.
- Implement the Solution: Write clean, readable code. Start with a basic implementation and optimize later if time allows.
- Test and Debug: Use sample inputs to test your code. Debug any issues you encounter.
- Optimize: If time permits, consider ways to improve the time or space complexity of your solution.
By following a consistent framework, you’ll be less likely to get overwhelmed and more likely to approach problems systematically, even under pressure.
4. Learn to Manage Your Time Effectively
Time management is crucial when solving coding challenges under pressure. Here are some strategies to help you make the most of your time:
- Read the Problem Carefully: Spend the first few minutes thoroughly understanding the problem. This investment upfront can save you time in the long run.
- Allocate Time Wisely: Divide your available time between understanding the problem, planning your approach, coding, and testing.
- Start with a Brute Force Solution: If you’re struggling to find an optimal solution, start with a brute force approach. Having a working solution, even if it’s not the most efficient, is better than having no solution at all.
- Know When to Move On: If you’re stuck on a problem for too long, consider moving on to the next one and coming back later if time allows.
- Leave Time for Testing: Always reserve some time at the end to test your code with various inputs and edge cases.
Remember, it’s not just about solving the problem quickly, but about delivering a correct and well-thought-out solution within the given time constraints.
5. Improve Your Coding Speed
While speed should never come at the cost of accuracy, being able to code quickly can give you a significant advantage in time-pressured situations. Here are some tips to improve your coding speed:
- Master Your IDE or Text Editor: Learn keyboard shortcuts and utilize features like code completion and snippets.
- Practice Typing: Improve your typing speed and accuracy. There are many online typing tutors available.
- Use Code Templates: Prepare templates for common algorithms and data structures that you can quickly adapt to specific problems.
- Write Clean Code from the Start: It’s faster to write clean, readable code from the beginning than to write messy code and clean it up later.
Here’s an example of a simple code template for a binary search in Python:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
# Usage
arr = [1, 3, 5, 7, 9, 11, 13]
target = 7
result = binary_search(arr, target)
print(f"Target {target} found at index: {result}")
Having templates like this ready can save you valuable time during a coding challenge.
6. Learn to Recognize Common Patterns
Many coding challenges are variations of common problem patterns. Learning to recognize these patterns can help you quickly identify the appropriate approach to solving a problem. Some common patterns include:
- Two Pointers
- Sliding Window
- Fast and Slow Pointers
- Merge Intervals
- Cyclic Sort
- In-place Reversal of a LinkedList
- Tree Breadth First Search
- Tree Depth First Search
- Two Heaps
- Subsets
- Modified Binary Search
- Top ‘K’ Elements
- K-way Merge
- Topological Sort
For each pattern, study multiple problems that use that pattern. This will help you recognize when to apply a particular approach to a new problem.
7. Develop Strong Debugging Skills
When working under pressure, it’s easy to make small mistakes that can lead to frustrating bugs. Strong debugging skills can help you quickly identify and fix issues in your code. Here are some tips for effective debugging:
- Use Print Statements: Strategically place print statements to track the flow of your program and the values of key variables.
- Leverage Debugger Tools: Learn to use the debugger in your IDE or coding environment. It can help you step through your code line by line and inspect variable values.
- Test with Edge Cases: Always consider edge cases (empty input, single element, extremely large input, etc.) when testing your code.
- Rubber Duck Debugging: Explain your code out loud as if you’re explaining it to someone else (or a rubber duck). This can often help you spot logical errors.
Here’s an example of how you might use print statements to debug a function:
def find_max(arr):
if not arr:
return None
max_val = arr[0]
print(f"Initial max: {max_val}") # Debug print
for num in arr[1:]:
print(f"Comparing {num} with current max {max_val}") # Debug print
if num > max_val:
max_val = num
print(f"New max: {max_val}") # Debug print
return max_val
# Test the function
test_arr = [3, 7, 2, 9, 1, 5]
result = find_max(test_arr)
print(f"Final result: {result}")
These print statements can help you track the function’s progress and identify where things might be going wrong.
8. Learn to Communicate Your Thought Process
In many coding interviews, the interviewer is just as interested in your problem-solving process as they are in your final solution. Being able to clearly communicate your thoughts as you work through a problem is a valuable skill. Here’s how you can improve:
- Think Aloud: Practice verbalizing your thoughts as you solve problems. This includes explaining your reasoning for choosing a particular approach, discussing potential trade-offs, and walking through your code.
- Explain Your Approach Before Coding: Before you start writing code, explain your high-level approach to the interviewer. This gives them a chance to provide feedback or guidance if needed.
- Discuss Complexity: Be prepared to discuss the time and space complexity of your solution. This shows that you’re thinking about efficiency and scalability.
- Ask for Feedback: Don’t be afraid to ask the interviewer if your approach makes sense or if they have any suggestions. This shows that you’re open to learning and collaboration.
Remember, clear communication can sometimes compensate for small mistakes in your code or approach.
9. Stay Calm and Manage Stress
Maintaining composure under pressure is crucial for clear thinking and problem-solving. Here are some strategies to help you stay calm during coding challenges:
- Deep Breathing: Take a few deep breaths before starting the challenge and during if you feel stress building up.
- Positive Self-Talk: Remind yourself of your preparation and capabilities. Replace negative thoughts with positive affirmations.
- Focus on the Present: Concentrate on the current problem rather than worrying about the outcome or future challenges.
- Take Short Breaks: If allowed, take brief mental breaks to reset your focus.
- Practice Mindfulness: Regular mindfulness or meditation practice can help improve your ability to stay calm under pressure.
Remember, it’s normal to feel some level of stress. The key is to manage it effectively so it doesn’t impair your performance.
10. Learn from Your Mistakes
Every coding challenge, whether you succeed or not, is an opportunity to learn and improve. After each challenge:
- Review Your Solution: Even if you solved the problem, look for ways to improve your code or approach.
- Study Optimal Solutions: If there are more efficient solutions available, study and understand them.
- Analyze Your Mistakes: If you made errors, identify what went wrong and how you can prevent similar mistakes in the future.
- Keep a Learning Journal: Document key learnings, common pitfalls, and strategies that worked well for you.
Continuous learning and self-reflection are key to long-term improvement in your coding skills.
Conclusion
Solving coding challenges under pressure is a skill that improves with practice and the right strategies. By following these ten tips – practicing regularly, mastering fundamentals, developing a problem-solving framework, managing time effectively, improving coding speed, recognizing patterns, honing debugging skills, communicating clearly, managing stress, and learning from mistakes – you’ll be well-equipped to tackle coding challenges with confidence.
Remember, the goal is not just to solve problems quickly, but to develop a robust problem-solving approach that you can rely on in any situation. With consistent effort and the right mindset, you can significantly enhance your ability to perform well in high-pressure coding scenarios, whether in interviews, competitions, or real-world development tasks.
Keep practicing, stay curious, and embrace each challenge as an opportunity to grow. Your journey in mastering coding challenges is ongoing, and each problem you face is a stepping stone towards becoming a more skilled and confident programmer.