10 Essential Tips for Acing Your Technical Coding Interview
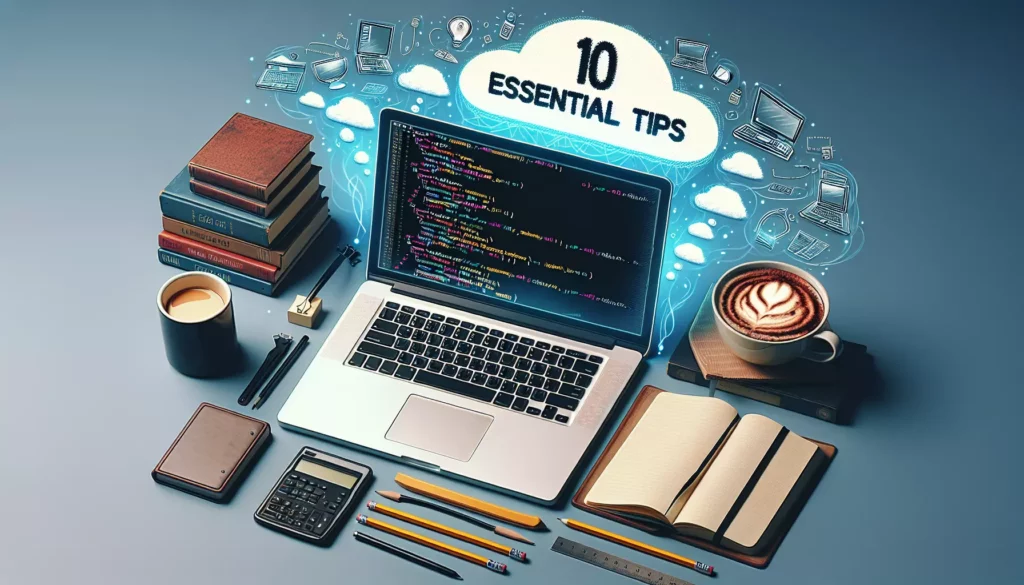
Are you preparing for a technical coding interview with a top tech company? Whether you’re eyeing a position at a FAANG (Facebook, Amazon, Apple, Netflix, Google) company or any other tech giant, mastering the art of the technical interview is crucial. In this comprehensive guide, we’ll explore ten essential tips to help you ace your coding interview and land your dream job in the competitive world of software development.
1. Master the Fundamentals of Data Structures and Algorithms
The foundation of any successful technical interview lies in a solid understanding of data structures and algorithms. These concepts are the building blocks of efficient problem-solving in computer science. Here’s why they’re so important:
- Efficiency: Knowing the right data structure or algorithm can dramatically improve the performance of your code.
- Problem-solving: Many interview questions are designed to test your ability to apply these concepts to real-world scenarios.
- Code organization: Proper use of data structures leads to cleaner, more maintainable code.
Focus on mastering these key areas:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Sorting and Searching Algorithms
- Dynamic Programming
- Recursion
Platforms like AlgoCademy offer structured learning paths and interactive tutorials to help you build a strong foundation in these areas. Make use of these resources to strengthen your understanding and practice implementing these concepts in code.
2. Practice, Practice, Practice
There’s no substitute for hands-on coding practice when preparing for a technical interview. The more problems you solve, the more comfortable you’ll become with different problem types and solution patterns. Here’s how to make the most of your practice sessions:
- Consistency is key: Aim to solve at least one coding problem every day.
- Variety matters: Expose yourself to a wide range of problem types and difficulty levels.
- Time yourself: Practice solving problems under time constraints to simulate interview conditions.
- Review and reflect: After solving a problem, take time to understand alternative solutions and optimize your approach.
Utilize coding platforms that offer a vast array of practice problems, such as LeetCode, HackerRank, or AlgoCademy’s interactive coding challenges. These platforms often categorize problems by difficulty and topic, allowing you to focus on areas where you need improvement.
3. Develop a Problem-Solving Approach
Having a structured approach to problem-solving can help you tackle even the most challenging interview questions. Follow these steps to develop a robust problem-solving methodology:
- Understand the problem: Carefully read the question and clarify any ambiguities with the interviewer.
- Identify the inputs and outputs: Clearly define what information you’re given and what you need to produce.
- Break down the problem: Divide complex problems into smaller, manageable sub-problems.
- Consider edge cases: Think about potential edge cases and how your solution will handle them.
- Develop a high-level approach: Outline your solution strategy before diving into coding.
- Implement the solution: Write clean, readable code to implement your approach.
- Test and debug: Walk through your code with sample inputs and identify any issues.
- Optimize: Consider ways to improve the time and space complexity of your solution.
Practice applying this approach to every problem you solve. Over time, it will become second nature, allowing you to tackle interview questions with confidence and clarity.
4. Learn to Communicate Your Thought Process
Technical interviews aren’t just about writing code; they’re also an opportunity to showcase your problem-solving skills and ability to communicate complex ideas. Here’s how to effectively articulate your thought process:
- Think out loud: Verbalize your thoughts as you work through the problem.
- Explain your reasoning: Justify your choices of data structures, algorithms, and approaches.
- Ask clarifying questions: Don’t hesitate to seek clarification on problem details or requirements.
- Discuss trade-offs: Explain the pros and cons of different solution approaches.
- Be open to feedback: Listen to the interviewer’s suggestions and be willing to adapt your approach.
Practice explaining your solutions to a friend or mentor. This will help you become more comfortable verbalizing your thoughts and improve your ability to communicate technical concepts clearly.
5. Familiarize Yourself with Common Interview Patterns
While every interview is unique, certain problem-solving patterns tend to appear frequently in technical interviews. Familiarizing yourself with these patterns can give you a significant advantage. Some common patterns include:
- Two Pointers
- Sliding Window
- Fast and Slow Pointers
- Merge Intervals
- Cyclic Sort
- In-place Reversal of a Linked List
- Tree Breadth-First Search
- Tree Depth-First Search
- Two Heaps
- Subsets
- Modified Binary Search
- Top K Elements
- K-way Merge
- Topological Sort
Study these patterns and practice applying them to various problems. Platforms like AlgoCademy often group problems by pattern, making it easier to focus your practice on specific techniques.
6. Master Your Preferred Programming Language
While most companies allow you to code in the language of your choice, it’s crucial to have a deep understanding of your preferred programming language. Here’s what you should focus on:
- Syntax mastery: Be comfortable with the language’s syntax and common idioms.
- Standard library knowledge: Familiarize yourself with built-in functions and data structures.
- Language-specific optimizations: Understand how to write efficient code in your chosen language.
- Common pitfalls: Be aware of language-specific quirks or common mistakes.
For example, if you’re using Python, you should be comfortable with list comprehensions, lambda functions, and the collections module. If you’re using Java, make sure you understand the intricacies of object-oriented programming and the Java Collections Framework.
Here’s a simple example of how language proficiency can make a difference in coding interviews:
// Less efficient way to check if a number is even in Python
def is_even(num):
if num % 2 == 0:
return True
else:
return False
# More pythonic and efficient way
def is_even(num):
return num % 2 == 0
Being able to write concise, idiomatic code can save you time and demonstrate your expertise during the interview.
7. Understand Time and Space Complexity
Analyzing the efficiency of your algorithms is a crucial skill in technical interviews. Interviewers often ask about the time and space complexity of your solutions. Here’s what you need to know:
- Big O notation: Understand how to express the worst-case time and space complexity using Big O notation.
- Common time complexities: Be familiar with O(1), O(log n), O(n), O(n log n), O(n^2), and O(2^n).
- Space complexity analysis: Consider both the input size and additional space used by your algorithm.
- Trade-offs: Understand the relationship between time and space complexity and when to prioritize one over the other.
Practice analyzing the complexity of your solutions for every problem you solve. This will help you develop an intuition for efficiency and prepare you to discuss complexity during interviews.
8. Mock Interviews and Peer Programming
Simulating the interview environment can significantly boost your confidence and performance. Here’s how to make the most of mock interviews and peer programming sessions:
- Find a practice partner: Team up with a friend or use platforms that offer mock interview services.
- Simulate real conditions: Use a whiteboard or a simple text editor without auto-completion or syntax highlighting.
- Time your sessions: Stick to typical interview durations (usually 45-60 minutes).
- Give and receive feedback: After each session, discuss what went well and areas for improvement.
- Practice different roles: Take turns being the interviewer and the interviewee to gain perspective from both sides.
Platforms like AlgoCademy often offer features for peer programming and mock interviews, allowing you to practice in a realistic setting with other learners or mentors.
9. Review Your Past Projects and Experiences
While technical skills are crucial, interviewers are also interested in your practical experience and problem-solving abilities in real-world scenarios. Prepare to discuss your past projects and experiences:
- Highlight key projects: Choose 2-3 significant projects you’ve worked on and be prepared to discuss them in detail.
- Focus on your contributions: Clearly articulate your role and the impact of your work on each project.
- Discuss challenges: Prepare to talk about obstacles you faced and how you overcame them.
- Technical decisions: Be ready to explain the reasoning behind key technical choices in your projects.
- Lessons learned: Reflect on what you learned from each experience and how it has shaped your skills as a developer.
Having a clear narrative about your experiences can help you stand out and demonstrate your ability to apply your skills in practical situations.
10. Stay Calm and Manage Your Stress
Technical interviews can be stressful, but maintaining composure is crucial for peak performance. Here are some strategies to help you stay calm and focused:
- Practice mindfulness: Incorporate relaxation techniques like deep breathing or meditation into your preparation routine.
- Positive self-talk: Cultivate a growth mindset and remind yourself of your capabilities.
- Prepare physically: Get enough sleep, eat well, and consider light exercise before the interview to boost your energy and mood.
- Arrive early: Give yourself plenty of time to arrive at the interview location or set up for a virtual interview.
- Remember it’s a conversation: View the interview as a collaborative problem-solving session rather than an interrogation.
- Learn from setbacks: If you struggle with a question, stay positive and try to learn from the experience.
Remember, even experienced developers sometimes struggle in interviews. The key is to stay calm, demonstrate your problem-solving process, and show your willingness to learn and improve.
Conclusion
Mastering technical coding interviews is a journey that requires dedication, practice, and a strategic approach. By following these ten tips, you’ll be well-equipped to showcase your skills and land your dream job in the tech industry. Remember to:
- Build a strong foundation in data structures and algorithms
- Practice consistently and strategically
- Develop a structured problem-solving approach
- Communicate your thoughts clearly
- Learn common interview patterns
- Master your preferred programming language
- Understand time and space complexity
- Engage in mock interviews and peer programming
- Prepare to discuss your past projects and experiences
- Manage your stress and stay calm during the interview
With platforms like AlgoCademy offering comprehensive resources, interactive coding challenges, and AI-powered assistance, you have all the tools you need to prepare effectively. Embrace the learning process, stay persistent, and approach each interview as an opportunity to grow as a developer. Good luck with your technical interviews!