10 Common Mistakes That Sabotage Your Coding Interview and How to Avoid Them
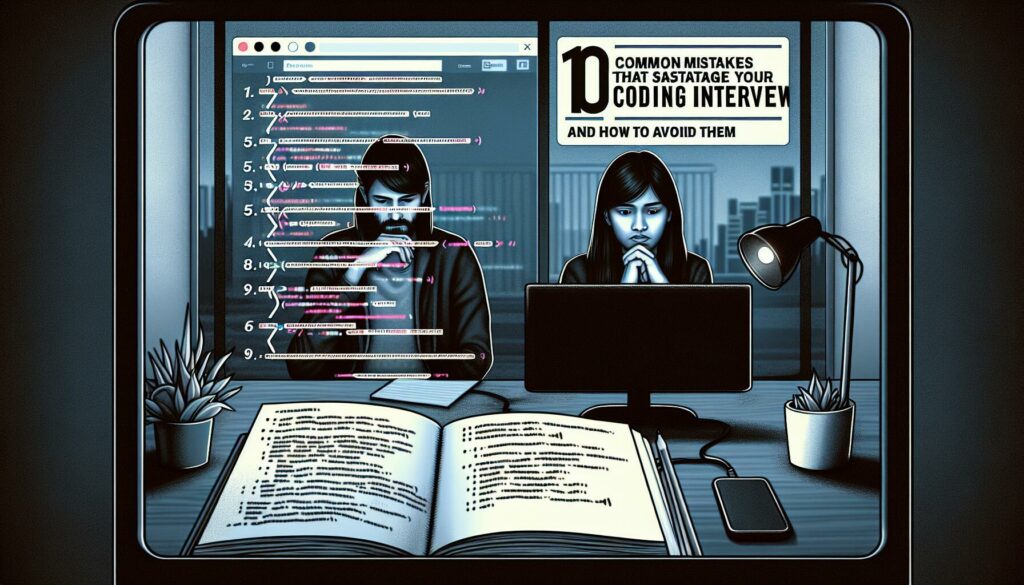
Landing your dream job in software development often hinges on your performance during the coding interview. This crucial step in the hiring process can be nerve-wracking, and even the most skilled developers can stumble if they’re not prepared. In this comprehensive guide, we’ll explore the 10 most common mistakes that can sabotage your coding interview and provide actionable advice on how to avoid them. By understanding these pitfalls and implementing the right strategies, you’ll be better equipped to showcase your skills and impress your potential employers.
1. Failing to Clarify the Problem
One of the biggest mistakes candidates make is diving into coding without fully understanding the problem at hand. This can lead to wasted time and incorrect solutions.
How to Avoid:
- Take a moment to read the problem statement carefully
- Ask clarifying questions about requirements, constraints, and edge cases
- Discuss potential approaches with the interviewer before starting to code
- Confirm your understanding by restating the problem in your own words
Remember, interviewers often intentionally leave out details to see if you’ll ask for clarification. Don’t be afraid to seek more information – it shows you’re thorough and communicative.
2. Poor Time Management
Coding interviews often have time constraints, and poor time management can prevent you from completing the task or demonstrating your full capabilities.
How to Avoid:
- Practice solving problems within time limits before the interview
- Allocate time for problem-solving, coding, and testing
- If you’re stuck, move on to other parts of the problem you can solve
- Keep an eye on the clock and pace yourself accordingly
It’s better to have a partially complete solution with good explanations than to run out of time while stuck on one part of the problem.
3. Neglecting to Think Aloud
Interviewers want to understand your thought process, not just see the final code. Staying silent throughout the coding process can be a missed opportunity to showcase your problem-solving skills.
How to Avoid:
- Verbalize your thought process as you work through the problem
- Explain the rationale behind your chosen approach
- Discuss trade-offs between different solutions
- Share any assumptions you’re making along the way
By thinking aloud, you give the interviewer insight into your reasoning and allow them to provide hints if you’re heading in the wrong direction.
4. Ignoring Edge Cases
Failing to consider and handle edge cases can make your solution incomplete and show a lack of attention to detail.
How to Avoid:
- Always consider extreme inputs (e.g., empty arrays, null values, very large numbers)
- Think about boundary conditions and special cases
- Discuss potential edge cases with the interviewer
- Include error handling and input validation in your code
Demonstrating that you think about edge cases shows that you write robust and reliable code.
5. Writing Messy, Unreadable Code
Even if your solution works, messy and unreadable code can leave a poor impression and make it difficult for the interviewer to follow your logic.
How to Avoid:
- Use consistent indentation and formatting
- Choose meaningful variable and function names
- Break down complex logic into smaller, well-named functions
- Add comments to explain non-obvious parts of your code
Here’s an example of clean, readable code:
def is_palindrome(s: str) -> bool:
# Remove non-alphanumeric characters and convert to lowercase
cleaned_s = ''.join(c.lower() for c in s if c.isalnum())
# Compare the string with its reverse
return cleaned_s == cleaned_s[::-1]
def find_longest_palindrome(text: str) -> str:
longest_palindrome = ""
for i in range(len(text)):
for j in range(i + 1, len(text) + 1):
substring = text[i:j]
if is_palindrome(substring) and len(substring) > len(longest_palindrome):
longest_palindrome = substring
return longest_palindrome
Writing clean, well-organized code demonstrates your professionalism and makes it easier for others to understand and maintain your work.
6. Overlooking Test Cases
Failing to test your code or only testing with basic inputs can lead to overlooking bugs or edge case failures.
How to Avoid:
- Develop a set of test cases before you start coding
- Include both normal and edge cases in your tests
- Test your code as you go, not just at the end
- Walk through your code with a small example to verify its correctness
Here’s an example of how you might include test cases in your code:
def test_find_longest_palindrome():
assert find_longest_palindrome("babad") in ["bab", "aba"]
assert find_longest_palindrome("cbbd") == "bb"
assert find_longest_palindrome("a") == "a"
assert find_longest_palindrome("") == ""
assert find_longest_palindrome("A man, a plan, a canal: Panama") == "amanaplanacanalpanama"
print("All tests passed!")
test_find_longest_palindrome()
By demonstrating that you test your code thoroughly, you show that you’re committed to producing reliable and correct solutions.
7. Failing to Optimize
While it’s important to get a working solution, failing to consider and implement optimizations can show a lack of understanding of efficiency and scalability.
How to Avoid:
- Start with a brute-force solution if necessary, but always mention that you’re aware it’s not optimal
- Analyze the time and space complexity of your initial solution
- Consider ways to improve efficiency, such as using appropriate data structures or algorithms
- Discuss trade-offs between different optimizations
For example, you might optimize the palindrome finder like this:
def find_longest_palindrome_optimized(s: str) -> str:
if not s:
return ""
def expand_around_center(left: int, right: int) -> str:
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return s[left + 1:right]
longest = s[0]
for i in range(len(s)):
# Odd length palindromes
palindrome1 = expand_around_center(i, i)
# Even length palindromes
palindrome2 = expand_around_center(i, i + 1)
if len(palindrome1) > len(longest):
longest = palindrome1
if len(palindrome2) > len(longest):
longest = palindrome2
return longest
This optimized version has a time complexity of O(n^2) instead of O(n^3), demonstrating your ability to improve algorithm efficiency.
8. Not Asking for Help When Stuck
Some candidates feel that asking for help during an interview shows weakness. In reality, knowing when to seek assistance is a valuable skill in software development.
How to Avoid:
- If you’re stuck, explain your current thought process and where you’re having difficulty
- Ask for hints or clarification if you’ve been struggling for more than a few minutes
- Be receptive to suggestions from the interviewer
- Use the interviewer’s hints as a learning opportunity
Remember, the interview is also an opportunity to demonstrate how you collaborate and handle challenges in a team setting.
9. Neglecting to Explain Design Decisions
Failing to explain why you chose certain approaches or data structures can make it seem like you’re coding without careful consideration.
How to Avoid:
- Explain your choice of data structures and algorithms
- Discuss the pros and cons of different approaches you considered
- Mention any assumptions or constraints that influenced your design
- Be prepared to defend your choices if the interviewer questions them
For instance, you might explain your choice of a hash table like this:
def two_sum(nums: List[int], target: int) -> List[int]:
# Using a hash table for O(1) lookup time
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return [] # No solution found
Explanation: “I chose to use a hash table (dictionary in Python) because it allows for O(1) lookup time, which helps us achieve an overall O(n) time complexity for this problem. The space complexity is also O(n) in the worst case, but this trade-off is worth it for the improved time efficiency.”
10. Giving Up Too Easily
Some candidates give up when they encounter a difficult problem or make a mistake. This can be interpreted as a lack of perseverance or problem-solving skills.
How to Avoid:
- Stay calm and composed when faced with challenges
- If your initial approach doesn’t work, be willing to start over with a new strategy
- Break down complex problems into smaller, manageable steps
- Demonstrate your problem-solving process, even if you don’t reach the final solution
Remember, interviewers are often more interested in seeing how you approach problems than whether you get the perfect answer immediately.
Conclusion
Coding interviews can be challenging, but by avoiding these common mistakes, you can significantly improve your chances of success. Remember to:
- Clarify the problem before coding
- Manage your time effectively
- Think aloud and explain your reasoning
- Consider edge cases and test your code
- Write clean, readable code
- Optimize your solutions when possible
- Ask for help when needed
- Explain your design decisions
- Persevere through challenges
By implementing these strategies, you’ll not only perform better in coding interviews but also develop habits that will serve you well throughout your software development career. Remember, practice is key – the more coding problems you solve and the more mock interviews you participate in, the more comfortable and proficient you’ll become.
Lastly, don’t forget that an interview is a two-way street. While you’re showcasing your skills, you’re also evaluating whether the company and role are a good fit for you. Stay confident, be yourself, and let your passion for coding shine through. With the right preparation and mindset, you’ll be well on your way to acing your next coding interview and landing your dream job in software development.