10 Common Coding Mistakes That Hinder Your Growth as a Programmer
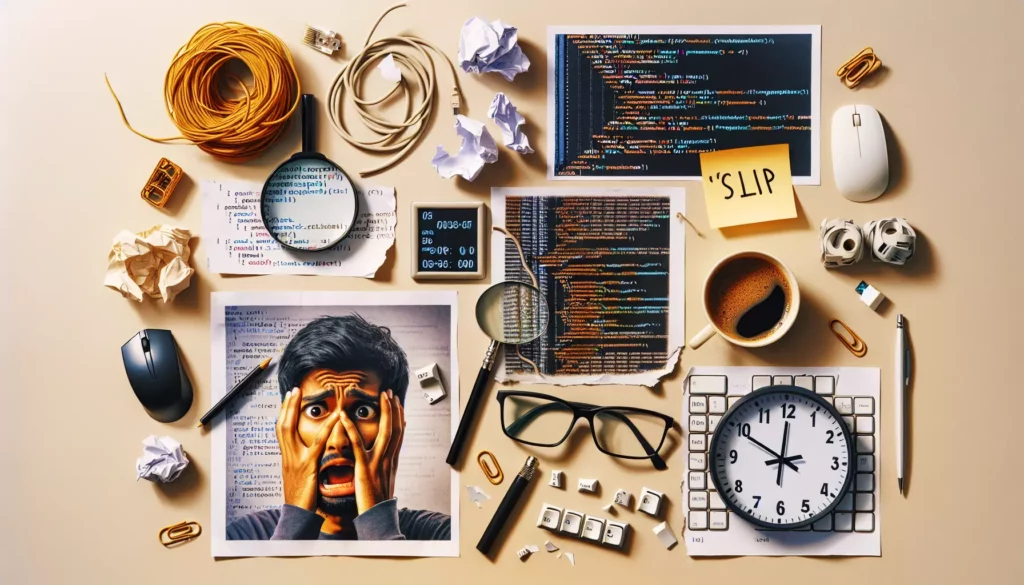
As aspiring programmers and seasoned developers alike, we all strive to improve our coding skills and advance our careers. However, certain coding habits and mistakes can significantly hinder our growth and progress. In this comprehensive guide, we’ll explore ten common coding mistakes that may be holding you back from reaching your full potential as a programmer. By identifying and addressing these issues, you’ll be better equipped to tackle complex problems, write cleaner code, and accelerate your journey towards becoming a proficient developer.
1. Neglecting to Plan Before Coding
One of the most prevalent mistakes among programmers, especially beginners, is diving into coding without proper planning. While it may seem time-consuming initially, taking the time to plan your approach can save you hours of debugging and refactoring later on.
Why it’s a problem:
- Leads to disorganized and inefficient code
- Makes it difficult to scale or modify your project later
- Increases the likelihood of bugs and errors
How to avoid it:
- Start with pseudocode to outline your algorithm
- Create a basic flowchart or diagram of your program’s structure
- Break down complex problems into smaller, manageable tasks
By implementing a planning phase before you start coding, you’ll have a clearer vision of your project’s structure and flow, leading to more organized and maintainable code.
2. Ignoring Code Readability and Documentation
Writing code that works is only half the battle. Ensuring that your code is readable and well-documented is crucial for long-term maintainability and collaboration with other developers.
Why it’s a problem:
- Makes it difficult for others (and your future self) to understand and modify the code
- Increases the time needed for debugging and maintenance
- Hinders collaboration in team projects
How to avoid it:
- Use meaningful variable and function names
- Write clear, concise comments explaining complex logic
- Follow consistent formatting and indentation practices
- Document your code using tools like JSDoc or Sphinx
Here’s an example of poorly documented code versus well-documented code:
// Poorly documented
function calc(a, b) {
return a * b + 100;
}
// Well-documented
/**
* Calculates the total cost of a product including tax.
* @param {number} price - The base price of the product.
* @param {number} taxRate - The tax rate as a decimal (e.g., 0.08 for 8%).
* @returns {number} The total cost including tax.
*/
function calculateTotalCost(price, taxRate) {
const taxAmount = price * taxRate;
return price + taxAmount;
}
3. Neglecting Error Handling
Proper error handling is essential for creating robust and reliable software. Many developers, especially those new to programming, often overlook this crucial aspect of coding.
Why it’s a problem:
- Makes your program vulnerable to crashes and unexpected behavior
- Difficult to debug and identify the source of problems
- Poor user experience when errors occur
How to avoid it:
- Use try-catch blocks to handle exceptions
- Implement proper input validation
- Provide meaningful error messages to users and log detailed error information for debugging
Here’s an example of improved error handling in JavaScript:
function divideNumbers(a, b) {
try {
if (typeof a !== 'number' || typeof b !== 'number') {
throw new Error('Both arguments must be numbers');
}
if (b === 0) {
throw new Error('Cannot divide by zero');
}
return a / b;
} catch (error) {
console.error('An error occurred:', error.message);
// You might want to re-throw the error or return a default value
return null;
}
}
4. Overcomplicating Solutions
As programmers, we often fall into the trap of creating overly complex solutions to problems that could be solved more simply. This tendency can lead to code that’s difficult to maintain and prone to bugs.
Why it’s a problem:
- Increases the likelihood of introducing bugs
- Makes code harder to understand and maintain
- Can lead to performance issues due to unnecessary complexity
How to avoid it:
- Follow the KISS principle (Keep It Simple, Stupid)
- Regularly refactor your code to simplify complex sections
- Seek feedback from peers on your code’s complexity
- Use design patterns appropriately, but don’t force them where they’re not needed
Here’s an example of simplifying a complex solution:
// Overcomplicated solution
function isEven(num) {
let result;
if (num % 2 === 0) {
result = true;
} else {
result = false;
}
return result;
}
// Simplified solution
function isEven(num) {
return num % 2 === 0;
}
5. Ignoring Code Reusability
Writing reusable code is a fundamental principle of good programming practice. Failing to create modular, reusable components can lead to duplicated code and increased maintenance efforts.
Why it’s a problem:
- Leads to code duplication, violating the DRY (Don’t Repeat Yourself) principle
- Makes it difficult to update and maintain your codebase
- Increases the likelihood of inconsistencies and bugs
How to avoid it:
- Create functions and classes for commonly used operations
- Use object-oriented programming principles to create reusable components
- Implement design patterns that promote code reuse
- Consider creating libraries or modules for frequently used functionality
Here’s an example of improving code reusability:
// Non-reusable code
function calculateCircleArea(radius) {
return Math.PI * radius * radius;
}
function calculateCircleCircumference(radius) {
return 2 * Math.PI * radius;
}
// Reusable code
class Circle {
constructor(radius) {
this.radius = radius;
}
area() {
return Math.PI * this.radius ** 2;
}
circumference() {
return 2 * Math.PI * this.radius;
}
}
// Usage
const myCircle = new Circle(5);
console.log(myCircle.area());
console.log(myCircle.circumference());
6. Neglecting Version Control
Version control systems like Git are essential tools for modern software development. Failing to use version control effectively can lead to lost work, difficulty collaborating, and challenges in managing different versions of your code.
Why it’s a problem:
- Increases the risk of losing important code changes
- Makes it difficult to collaborate with other developers
- Complicates the process of managing different versions and features
How to avoid it:
- Learn and use a version control system like Git
- Commit your changes regularly with meaningful commit messages
- Use branches for developing new features or experimenting
- Familiarize yourself with common Git workflows, such as GitFlow
Here’s an example of a good Git commit message:
feat: Add user authentication functionality
- Implement login and registration forms
- Create user model and database schema
- Set up JWT for secure authentication
- Add middleware for protected routes
Closes #123
7. Ignoring Performance Considerations
While premature optimization is often discouraged, completely ignoring performance considerations can lead to slow and inefficient code that doesn’t scale well.
Why it’s a problem:
- Results in slow and unresponsive applications
- Can lead to poor user experience
- Makes it difficult to scale your application as it grows
How to avoid it:
- Learn about time and space complexity (Big O notation)
- Use appropriate data structures for your use case
- Avoid unnecessary computations and redundant operations
- Profile your code to identify performance bottlenecks
- Optimize critical paths in your application
Here’s an example of improving performance by using a more efficient algorithm:
// Inefficient approach (O(n^2) time complexity)
function hasDuplicates(arr) {
for (let i = 0; i
8. Failing to Write Tests
Writing tests for your code is crucial for ensuring its reliability and making it easier to refactor and maintain. Many developers, especially those new to the field, often neglect this important practice.
Why it’s a problem:
- Increases the likelihood of introducing bugs when making changes
- Makes it difficult to refactor code with confidence
- Can lead to reduced code quality over time
How to avoid it:
- Learn and practice Test-Driven Development (TDD)
- Write unit tests for individual functions and methods
- Implement integration tests for larger components
- Use testing frameworks appropriate for your programming language
- Aim for good test coverage in your codebase
Here’s an example of a simple unit test using Jest for JavaScript:
// Function to test
function sum(a, b) {
return a + b;
}
// Test suite
describe('sum function', () => {
test('adds 1 + 2 to equal 3', () => {
expect(sum(1, 2)).toBe(3);
});
test('adds -1 + 1 to equal 0', () => {
expect(sum(-1, 1)).toBe(0);
});
test('adds 0.1 + 0.2 to be close to 0.3', () => {
expect(sum(0.1, 0.2)).toBeCloseTo(0.3);
});
});
9. Not Staying Updated with New Technologies
The field of software development is constantly evolving, with new technologies, frameworks, and best practices emerging regularly. Failing to stay updated can lead to outdated skills and missed opportunities.
Why it’s a problem:
- Can limit your career growth and job opportunities
- May result in using outdated or inefficient techniques
- Makes it challenging to work on modern projects
How to avoid it:
- Regularly read tech blogs, articles, and newsletters
- Attend conferences, webinars, and meetups
- Experiment with new technologies in side projects
- Participate in online coding communities and forums
- Take online courses or pursue certifications in emerging technologies
While it’s important to stay updated, it’s equally crucial to strike a balance and not chase every new trend. Focus on technologies that align with your career goals and have proven longevity.
10. Neglecting Soft Skills
While technical skills are crucial for programmers, soft skills like communication, teamwork, and problem-solving are equally important for career growth. Many developers focus solely on improving their coding skills while neglecting these essential soft skills.
Why it’s a problem:
- Can hinder effective collaboration in team settings
- May limit your ability to understand and meet client or user needs
- Can impact your career progression, especially into leadership roles
How to avoid it:
- Practice clear and concise communication, both written and verbal
- Actively participate in code reviews and team discussions
- Develop empathy for users and stakeholders
- Learn to give and receive constructive feedback
- Work on your time management and prioritization skills
Remember that being a great programmer isn’t just about writing excellent code; it’s also about being able to work effectively with others and solve real-world problems.
Conclusion
Avoiding these common coding mistakes can significantly accelerate your growth as a programmer. By focusing on writing clean, efficient, and maintainable code, staying updated with new technologies, and developing your soft skills, you’ll be well-equipped to tackle complex programming challenges and advance in your career.
Remember that becoming a proficient programmer is a continuous journey of learning and improvement. Don’t be discouraged if you find yourself making some of these mistakes – awareness is the first step towards improvement. Keep practicing, seek feedback from more experienced developers, and always strive to write better code.
As you continue your coding journey, consider using platforms like AlgoCademy to enhance your skills. With interactive coding tutorials, AI-powered assistance, and a focus on algorithmic thinking and problem-solving, AlgoCademy can help you avoid these common pitfalls and develop the skills needed to excel in technical interviews and real-world programming challenges.
Happy coding, and may your future projects be bug-free and efficient!