10 Biggest Mistakes Programmers Make in Technical Interviews (And How to Avoid Them)
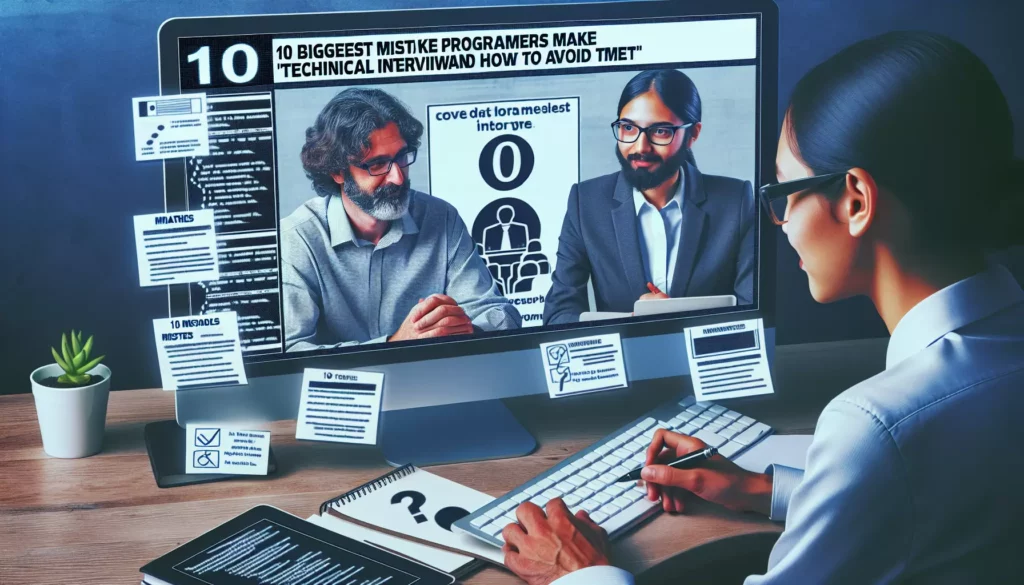
Technical interviews can be daunting, even for experienced programmers. Whether you’re a fresh graduate or a seasoned developer, the pressure to perform well in these high-stakes situations can lead to common pitfalls. In this comprehensive guide, we’ll explore the ten biggest mistakes programmers often make during technical interviews and provide actionable advice on how to avoid them. By understanding these potential pitfalls, you’ll be better prepared to showcase your skills and land your dream job in the competitive world of software development.
1. Failing to Clarify the Problem
One of the most critical mistakes programmers make is diving into coding without fully understanding the problem at hand. This eagerness to start writing code can lead to wasted time and incorrect solutions.
Why it’s a problem:
- Misinterpreting the question can result in solving the wrong problem entirely.
- Overlooking important details or constraints can lead to an incomplete or inefficient solution.
- It may give the impression that you lack attention to detail or don’t value clear communication.
How to avoid it:
- Take a moment to carefully read and analyze the problem statement.
- Ask clarifying questions about input formats, expected outputs, and any constraints or edge cases.
- Repeat your understanding of the problem back to the interviewer to ensure you’re on the same page.
- Discuss potential approaches before starting to code, allowing the interviewer to provide guidance if needed.
Remember, interviewers often intentionally leave out certain details to see if you’ll ask the right questions. Demonstrating your ability to gather requirements effectively is a valuable skill in real-world programming scenarios.
2. Poor Time Management
Technical interviews often come with time constraints, and poor time management can prevent you from showcasing your full potential.
Why it’s a problem:
- Spending too much time on a single aspect of the problem can leave you rushed or unable to complete the entire task.
- Failing to allocate time for testing and optimization can result in a suboptimal or buggy solution.
- It may give the impression that you struggle with prioritization and meeting deadlines.
How to avoid it:
- Ask about the time limit at the beginning of the interview if it’s not clearly stated.
- Break down the problem into smaller tasks and estimate the time needed for each.
- Set mental checkpoints for yourself (e.g., “I should have the basic algorithm implemented by the halfway mark”).
- If you’re stuck on a particular part, consider moving on and coming back to it later if time allows.
- Leave time at the end for testing, optimization, and discussing potential improvements.
Effective time management not only helps you complete the task but also demonstrates your ability to work efficiently under pressure – a valuable skill in any development role.
3. Neglecting to Think Aloud
Many candidates make the mistake of coding in silence, leaving the interviewer in the dark about their thought process.
Why it’s a problem:
- Interviewers can’t assess your problem-solving approach if they don’t know what you’re thinking.
- Silent coding makes it difficult for interviewers to provide hints or guidance when you’re stuck.
- It may give the impression that you struggle with communication or teamwork.
How to avoid it:
- Verbalize your thought process as you work through the problem.
- Explain the rationale behind your chosen approach and any trade-offs you’re considering.
- If you’re unsure about something, voice your concerns and ask for clarification.
- Discuss alternative solutions you’ve considered, even if you don’t implement them.
Thinking aloud not only helps the interviewer understand your reasoning but also demonstrates your ability to communicate technical concepts effectively – a crucial skill for working in a team environment.
4. Ignoring Edge Cases and Error Handling
A common pitfall is focusing solely on the “happy path” and neglecting to consider edge cases or implement proper error handling.
Why it’s a problem:
- Real-world applications need to be robust and handle unexpected inputs or scenarios.
- Failing to address edge cases can lead to bugs and system vulnerabilities.
- It may indicate a lack of thoroughness or attention to detail in your coding practices.
How to avoid it:
- After implementing the main solution, systematically consider potential edge cases (e.g., empty inputs, extremely large values, negative numbers).
- Implement input validation and error handling where appropriate.
- Discuss with the interviewer any assumptions you’re making about the input or system constraints.
- If time allows, write test cases that cover both typical scenarios and edge cases.
Here’s a simple example of how you might handle an edge case in a function that calculates the average of an array of numbers:
function calculateAverage(numbers) {
if (!Array.isArray(numbers) || numbers.length === 0) {
throw new Error("Input must be a non-empty array of numbers");
}
const sum = numbers.reduce((acc, num) => acc + num, 0);
return sum / numbers.length;
}
// Usage:
try {
console.log(calculateAverage([1, 2, 3, 4, 5])); // Output: 3
console.log(calculateAverage([])); // Throws an error
} catch (error) {
console.error(error.message);
}
By demonstrating your ability to anticipate and handle edge cases, you show that you write code with reliability and maintainability in mind.
5. Overlooking Code Efficiency and Optimization
While getting a working solution is important, many candidates stop there without considering the efficiency of their code or potential optimizations.
Why it’s a problem:
- Inefficient solutions may not scale well with larger inputs, leading to performance issues.
- Overlooking optimization opportunities can result in unnecessary resource consumption.
- It may suggest a lack of understanding of algorithmic complexity and performance considerations.
How to avoid it:
- After implementing a working solution, analyze its time and space complexity.
- Consider whether there are more efficient data structures or algorithms you could use.
- Look for opportunities to reduce redundant calculations or unnecessary iterations.
- Discuss the trade-offs between different approaches in terms of time complexity, space complexity, and readability.
- If time allows, implement or at least discuss potential optimizations.
Here’s an example of optimizing a function that finds the nth Fibonacci number:
// Inefficient recursive solution
function fibonacciRecursive(n) {
if (n <= 1) return n;
return fibonacciRecursive(n - 1) + fibonacciRecursive(n - 2);
}
// Optimized iterative solution
function fibonacciIterative(n) {
if (n <= 1) return n;
let a = 0, b = 1;
for (let i = 2; i <= n; i++) {
[a, b] = [b, a + b];
}
return b;
}
// Usage:
console.log(fibonacciIterative(10)); // Output: 55
The recursive solution has an exponential time complexity of O(2^n), while the iterative solution has a linear time complexity of O(n) and constant space complexity. By discussing and implementing such optimizations, you demonstrate your ability to write efficient and scalable code.
6. Neglecting to Test Your Code
In the rush to complete the problem, many candidates forget to thoroughly test their code or rely solely on the provided test cases.
Why it’s a problem:
- Untested code may contain bugs or fail to handle certain scenarios.
- Relying only on provided test cases may miss edge cases or important scenarios.
- It can give the impression that you don’t prioritize code quality and reliability.
How to avoid it:
- Allocate time for testing in your problem-solving approach.
- Write your own test cases, including edge cases and typical scenarios.
- Use a methodical approach to testing, such as boundary value analysis or equivalence partitioning.
- If writing formal unit tests isn’t feasible due to time constraints, at least walk through your code with sample inputs.
- Explain your testing strategy to the interviewer, showcasing your commitment to code quality.
Here’s an example of how you might include some basic testing in your solution:
function isPalindrome(str) {
const cleanStr = str.toLowerCase().replace(/[^a-z0-9]/g, '');
return cleanStr === cleanStr.split('').reverse().join('');
}
// Test cases
function runTests() {
const testCases = [
{ input: "A man, a plan, a canal: Panama", expected: true },
{ input: "race a car", expected: false },
{ input: "", expected: true },
{ input: "Madam, I'm Adam", expected: true },
{ input: "12321", expected: true },
{ input: "Not a palindrome", expected: false }
];
testCases.forEach((testCase, index) => {
const result = isPalindrome(testCase.input);
console.log(`Test case ${index + 1}: ${result === testCase.expected ? 'PASS' : 'FAIL'}`);
console.log(` Input: "${testCase.input}"`);
console.log(` Expected: ${testCase.expected}, Got: ${result}\n`);
});
}
runTests();
By including tests and discussing your testing approach, you demonstrate your commitment to producing reliable and well-verified code.
7. Poor Code Organization and Readability
In the pressure of an interview, it’s easy to focus solely on functionality and neglect code organization and readability.
Why it’s a problem:
- Messy or poorly organized code is harder to understand, debug, and maintain.
- It can make it difficult for the interviewer to follow your logic and thought process.
- Poor code organization may suggest that you struggle with writing clean, professional-quality code.
How to avoid it:
- Use meaningful variable and function names that clearly convey their purpose.
- Break down complex logic into smaller, well-named functions.
- Use consistent indentation and formatting.
- Add comments to explain complex logic or non-obvious decisions.
- Follow established coding conventions for the language you’re using.
Here’s an example of refactoring poorly organized code into a more readable and maintainable version:
// Poor organization
function x(a,b,c){let d=0;for(let i=0;i<a.length;i++){if(a[i]>b&&a[i]<c){d+=a[i];}}return d;}
// Improved organization and readability
function sumNumbersInRange(numbers, lowerBound, upperBound) {
let sum = 0;
for (const number of numbers) {
if (isInRange(number, lowerBound, upperBound)) {
sum += number;
}
}
return sum;
}
function isInRange(number, min, max) {
return number > min && number < max;
}
// Usage
const numbers = [1, 5, 3, 9, 2, 7];
const result = sumNumbersInRange(numbers, 2, 8);
console.log(result); // Output: 15
By writing clean, well-organized code, you demonstrate your ability to produce maintainable solutions and work effectively in a team environment.
8. Failing to Communicate When Stuck
Many candidates freeze up or become silent when they encounter a challenging part of the problem, afraid to admit they’re stuck.
Why it’s a problem:
- Silence can be interpreted as a lack of problem-solving skills or giving up.
- It prevents the interviewer from providing helpful hints or guidance.
- It misses an opportunity to demonstrate your ability to work through challenges collaboratively.
How to avoid it:
- If you’re stuck, verbalize what you’re thinking and where you’re having difficulty.
- Explain the approaches you’ve considered and why they might not work.
- Ask for clarification or hints if needed, framing it as a collaborative problem-solving exercise.
- Break down the problem into smaller parts and tackle them one at a time.
- Consider working through a simpler version of the problem first to gain insights.
Here’s an example of how you might communicate when stuck:
“I’m trying to figure out how to efficiently find the longest palindromic substring. I’ve considered using a brute force approach of checking every possible substring, but that would be O(n^3) time complexity, which seems inefficient. I’m wondering if there’s a way to use dynamic programming here, but I’m not sure how to structure the subproblems. Do you have any suggestions on what direction I should explore next?”
By communicating openly when you’re stuck, you demonstrate resilience, problem-solving skills, and the ability to work collaboratively – all valuable traits in a professional setting.
9. Neglecting to Consider Space Complexity
While many candidates focus on optimizing time complexity, they often overlook the importance of space complexity in their solutions.
Why it’s a problem:
- Excessive memory usage can be as problematic as slow execution in real-world applications.
- Overlooking space complexity may indicate a lack of understanding of system resources and constraints.
- It misses an opportunity to demonstrate a comprehensive understanding of algorithmic efficiency.
How to avoid it:
- Consider both time and space complexity when designing your solution.
- Analyze the memory usage of your algorithm, including any additional data structures you introduce.
- Discuss trade-offs between time and space complexity with the interviewer.
- Look for opportunities to optimize space usage, such as in-place algorithms or reusing existing data structures.
Here’s an example comparing two approaches to reversing a string, one with O(n) space complexity and another with O(1) space complexity:
// O(n) space complexity
function reverseStringExtraSpace(s) {
return s.split('').reverse().join('');
}
// O(1) space complexity (assuming string is mutable)
function reverseStringInPlace(s) {
const chars = s.split('');
let left = 0;
let right = chars.length - 1;
while (left < right) {
[chars[left], chars[right]] = [chars[right], chars[left]];
left++;
right--;
}
return chars.join('');
}
// Usage
const str = "Hello, World!";
console.log(reverseStringExtraSpace(str)); // Output: "!dlroW ,olleH"
console.log(reverseStringInPlace(str)); // Output: "!dlroW ,olleH"
By considering and discussing space complexity, you demonstrate a more comprehensive understanding of algorithmic efficiency and resource management.
10. Forgetting to Ask Questions About the Role or Company
While not strictly a technical mistake, many candidates forget that an interview is a two-way process and miss the opportunity to ask insightful questions about the role or company.
Why it’s a problem:
- It can give the impression that you’re not genuinely interested in the position or company.
- You miss valuable information that could help you decide if the role is a good fit for you.
- It’s a missed opportunity to demonstrate your enthusiasm and preparedness.
How to avoid it:
- Prepare a list of thoughtful questions about the role, team, and company before the interview.
- Ask about the technical challenges the team is facing and how your role would contribute to solving them.
- Inquire about the company’s engineering culture, development processes, and opportunities for growth.
- Show interest in the interviewer’s experience at the company and what they find most rewarding about their work.
Here are some example questions you might ask:
- “Can you tell me more about the current projects your team is working on and the technologies you’re using?”
- “How does your team approach continuous learning and staying up-to-date with new technologies?”
- “What are the biggest technical challenges your team has faced recently, and how did you overcome them?”
- “How does the company support professional development and career growth for engineers?”
- “Can you describe the typical development lifecycle for a feature, from ideation to deployment?”
By asking thoughtful questions, you not only gather valuable information but also demonstrate your genuine interest in the role and your potential as a proactive team member.
Conclusion
Technical interviews can be challenging, but by avoiding these common mistakes, you can significantly improve your chances of success. Remember to:
- Clarify the problem thoroughly before starting.
- Manage your time effectively.
- Think aloud and communicate your thought process.
- Consider edge cases and implement proper error handling.
- Look for opportunities to optimize your code.
- Test your solution thoroughly.
- Write clean, well-organized code.
- Communicate openly when you’re stuck.
- Consider both time and space complexity.
- Ask insightful questions about the role and company.
By focusing on these areas, you’ll not only perform better in technical interviews but also demonstrate the skills and qualities that make for an excellent software developer. Remember, practice is key to improving your interview performance. Platforms like AlgoCademy offer interactive coding tutorials and resources to help you prepare effectively for technical interviews, with a focus on algorithmic thinking and problem-solving skills.
Good luck with your next technical interview!