Understanding RESTful APIs: A Comprehensive Guide for Developers
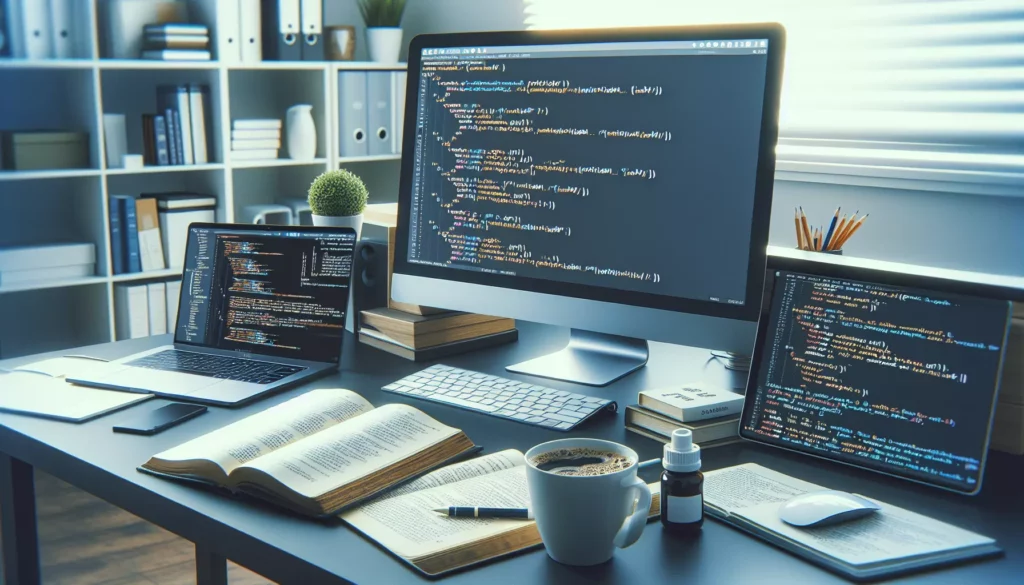
In today’s interconnected digital world, APIs (Application Programming Interfaces) play a crucial role in allowing different software systems to communicate with each other. Among the various types of APIs, RESTful APIs have emerged as a popular and widely adopted architectural style for designing networked applications. Whether you’re a beginner programmer or an experienced developer looking to enhance your skills, understanding RESTful APIs is essential for modern software development.
In this comprehensive guide, we’ll dive deep into the world of RESTful APIs, exploring their principles, benefits, and best practices. By the end of this article, you’ll have a solid foundation in RESTful API design and implementation, which will prove invaluable as you progress in your coding journey and prepare for technical interviews at major tech companies.
Table of Contents
- What is REST?
- Key Principles of REST
- RESTful APIs: The Basics
- HTTP Methods in RESTful APIs
- HTTP Status Codes
- Resource Naming Conventions
- API Versioning
- Authentication and Security
- Best Practices for RESTful API Design
- RESTful API Examples
- Tools for Working with RESTful APIs
- Conclusion
1. What is REST?
REST, which stands for Representational State Transfer, is an architectural style for designing networked applications. It was first introduced by Roy Fielding in his doctoral dissertation in 2000. REST is not a protocol or a standard, but rather a set of constraints that, when applied as a whole, provides a scalable and maintainable architecture for system-to-system communication.
The key idea behind REST is to treat server-side resources as uniquely identifiable by URLs. These resources can be manipulated using a standard set of operations, typically corresponding to the HTTP methods GET, POST, PUT, DELETE, and others.
2. Key Principles of REST
To fully understand RESTful APIs, it’s crucial to grasp the fundamental principles that define the REST architectural style:
- Client-Server Architecture: The client and server are separated, allowing them to evolve independently.
- Statelessness: Each request from the client to the server must contain all the information needed to understand and process the request. The server should not store any client context between requests.
- Cacheability: Responses must define themselves as cacheable or non-cacheable to prevent clients from reusing stale or inappropriate data in response to further requests.
- Uniform Interface: A uniform way of interacting with a given server irrespective of device or application type. This simplifies and decouples the architecture.
- Layered System: A client cannot ordinarily tell whether it is connected directly to the end server or an intermediary along the way.
- Code on Demand (optional): Servers can temporarily extend or customize the functionality of a client by transferring executable code.
3. RESTful APIs: The Basics
A RESTful API is an API that adheres to the principles of REST. It uses HTTP requests to access and manipulate data. RESTful APIs are designed around resources, which are any kind of object, data, or service that can be accessed by the client.
Here are some key characteristics of RESTful APIs:
- They use HTTP methods explicitly.
- They are stateless.
- They expose directory structure-like URIs.
- They transfer data in one of several formats, often JSON or XML.
4. HTTP Methods in RESTful APIs
RESTful APIs typically use the following HTTP methods to perform operations on resources:
- GET: Retrieve a resource
- POST: Create a new resource
- PUT: Update an existing resource
- DELETE: Remove a resource
- PATCH: Partially modify a resource
- HEAD: Similar to GET but retrieves only headers, not the body
- OPTIONS: Describes the communication options for the target resource
Here’s an example of how these methods might be used in a RESTful API for a blog application:
GET /posts # Retrieve all posts
POST /posts # Create a new post
GET /posts/123 # Retrieve post with ID 123
PUT /posts/123 # Update post with ID 123
DELETE /posts/123 # Delete post with ID 123
PATCH /posts/123 # Partially update post with ID 123
5. HTTP Status Codes
HTTP status codes are an integral part of RESTful APIs. They indicate the result of the HTTP request. Here are some common status codes you’ll encounter:
- 200 OK: The request was successful
- 201 Created: The request was successful and a new resource was created
- 204 No Content: The request was successful but there’s no content to send back
- 400 Bad Request: The request was invalid or cannot be served
- 401 Unauthorized: The request requires authentication
- 403 Forbidden: The server understood the request but refuses to authorize it
- 404 Not Found: The requested resource could not be found
- 500 Internal Server Error: The server encountered an unexpected condition
Using appropriate status codes helps clients understand the outcome of their requests and handle different scenarios accordingly.
6. Resource Naming Conventions
Proper naming of resources is crucial for creating intuitive and easy-to-use APIs. Here are some best practices for naming resources:
- Use nouns to represent resources
- Use plural nouns for collections
- Use hyphens (-) to improve readability
- Avoid using verbs in URIs
- Use lowercase letters
Examples of good resource naming:
/users
/user-profiles
/blog-posts
/categories/5/posts
7. API Versioning
As your API evolves, you may need to make changes that could break existing client integrations. Versioning your API allows you to introduce changes while maintaining backward compatibility. There are several approaches to API versioning:
- URI Versioning: Include the version in the URI
/api/v1/users
- Header Versioning: Use a custom header to specify the version
Accept-version: v1
- Parameter Versioning: Include the version as a query parameter
/api/users?version=1
- Media Type Versioning: Use content negotiation and custom media types
Accept: application/vnd.myapp.v1+json
Each approach has its pros and cons, and the choice often depends on your specific requirements and constraints.
8. Authentication and Security
Securing your RESTful API is crucial to protect sensitive data and ensure that only authorized clients can access your resources. Here are some common authentication methods used in RESTful APIs:
- API Keys: A simple approach where clients include a unique key in each request.
- Basic Authentication: Clients send a username and password with each request.
- Token-based Authentication: Clients obtain a token (e.g., JWT) and include it in the request headers.
- OAuth 2.0: A more complex but flexible authorization framework.
In addition to authentication, consider implementing the following security measures:
- Use HTTPS to encrypt data in transit
- Implement rate limiting to prevent abuse
- Validate and sanitize input data
- Use appropriate error messages that don’t reveal sensitive information
9. Best Practices for RESTful API Design
To create high-quality RESTful APIs, consider following these best practices:
- Use JSON as the primary data format: JSON is lightweight and easy to read and write for both humans and machines.
- Implement HATEOAS (Hypertext As The Engine Of Application State): Include links in API responses to guide clients through the application.
- Use plural nouns for resource collections: For consistency and clarity, use plural nouns for resource collections (e.g., /users instead of /user).
- Provide comprehensive documentation: Clear and detailed documentation helps developers understand and integrate your API quickly.
- Implement pagination for large datasets: Use query parameters like limit and offset to allow clients to request specific subsets of data.
- Use appropriate HTTP status codes: Respond with the correct status code to indicate the result of the request.
- Handle errors gracefully: Provide clear error messages and use appropriate status codes for different types of errors.
- Allow filtering, sorting, and searching: Implement these features to give clients more control over the data they receive.
- Use SSL/TLS: Secure your API by using HTTPS to encrypt data in transit.
- Implement caching: Use HTTP caching headers to improve performance and reduce server load.
10. RESTful API Examples
To better understand RESTful APIs, let’s look at some examples of API endpoints and their corresponding HTTP methods:
User Management API
GET /users # Retrieve all users
POST /users # Create a new user
GET /users/{id} # Retrieve a specific user
PUT /users/{id} # Update a specific user
DELETE /users/{id} # Delete a specific user
GET /users/{id}/posts # Retrieve posts for a specific user
E-commerce API
GET /products # Retrieve all products
POST /products # Create a new product
GET /products/{id} # Retrieve a specific product
PUT /products/{id} # Update a specific product
DELETE /products/{id} # Delete a specific product
GET /orders # Retrieve all orders
POST /orders # Create a new order
GET /orders/{id} # Retrieve a specific order
PATCH /orders/{id} # Update the status of an order
Social Media API
GET /posts # Retrieve all posts
POST /posts # Create a new post
GET /posts/{id} # Retrieve a specific post
PUT /posts/{id} # Update a specific post
DELETE /posts/{id} # Delete a specific post
POST /posts/{id}/likes # Like a specific post
DELETE /posts/{id}/likes # Unlike a specific post
GET /users/{id}/followers # Retrieve followers of a user
POST /users/{id}/follow # Follow a user
DELETE /users/{id}/follow # Unfollow a user
11. Tools for Working with RESTful APIs
There are numerous tools available to help developers work with RESTful APIs. Here are some popular ones:
- Postman: A powerful API development environment for testing, documenting, and sharing APIs.
- Swagger/OpenAPI: A set of tools for designing, building, and documenting RESTful APIs.
- cURL: A command-line tool for making HTTP requests, useful for quick API testing.
- Insomnia: A cross-platform HTTP client with a clean interface for organizing API requests.
- REST Client for Visual Studio Code: An extension that allows you to send HTTP requests and view responses directly in VS Code.
- Axios: A popular JavaScript library for making HTTP requests from both browser and Node.js environments.
- Paw (for macOS): A full-featured HTTP client for testing and describing APIs.
These tools can significantly streamline your workflow when developing, testing, and documenting RESTful APIs.
12. Conclusion
RESTful APIs have become a cornerstone of modern web development, enabling seamless communication between different software systems. By adhering to REST principles and following best practices, you can create APIs that are scalable, maintainable, and easy to use.
As you continue your journey in coding education and skill development, mastering RESTful API design and implementation will prove invaluable. Whether you’re building your own applications or preparing for technical interviews at major tech companies, a solid understanding of RESTful APIs will set you apart as a skilled developer.
Remember that practice is key to mastering any programming concept. Try building your own RESTful APIs, experiment with different design patterns, and use the tools mentioned in this guide to refine your skills. As you gain more experience, you’ll develop an intuition for creating elegant and efficient API designs that solve real-world problems.
Keep learning, stay curious, and happy coding!