Top 20 Twilio Interview Questions: Master Your Tech Interview
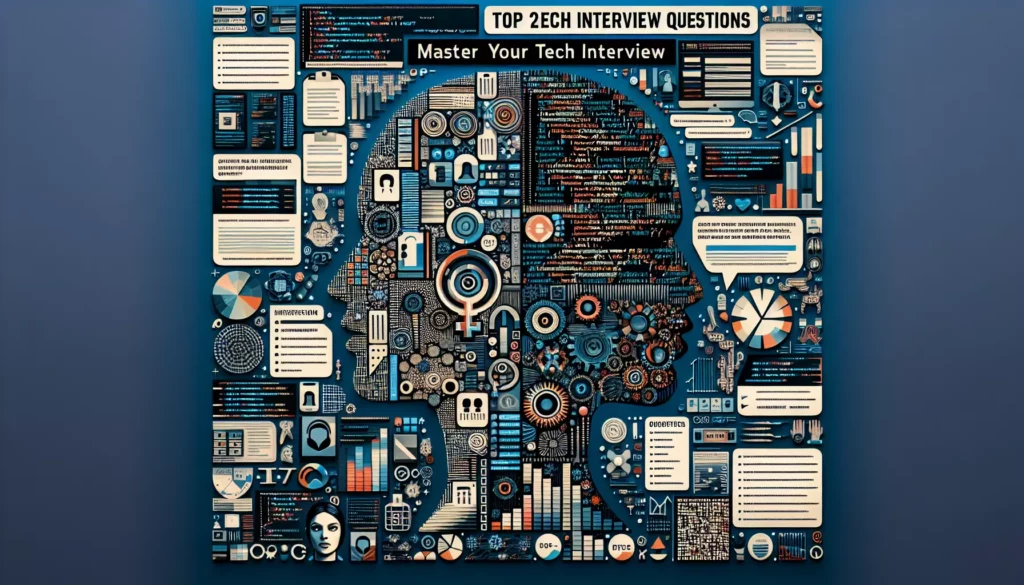
Are you preparing for a Twilio interview? Whether you’re a seasoned developer or just starting your journey in the world of cloud communications, it’s crucial to be well-prepared. In this comprehensive guide, we’ll explore the top 20 Twilio interview questions you might encounter, along with detailed answers and explanations. By the end of this article, you’ll be better equipped to showcase your knowledge and skills in your Twilio interview.
Understanding Twilio and Its Importance
Before we dive into the specific interview questions, let’s briefly discuss what Twilio is and why it’s such an important player in the tech industry.
Twilio is a cloud communications platform that allows developers to integrate various communication channels (such as voice, messaging, and video) into their applications. It provides APIs that enable businesses to build powerful communication experiences for their customers. As more companies seek to enhance their customer engagement strategies, Twilio has become an increasingly valuable tool in the developer’s toolkit.
Top 20 Twilio Interview Questions and Answers
1. What is Twilio, and how does it work?
Answer: Twilio is a cloud communications platform that provides APIs for developers to integrate voice, video, and messaging capabilities into their applications. It works by offering a set of web service APIs that developers can use to programmatically make and receive phone calls, send and receive text messages, and perform other communication functions. Twilio handles the complex telecommunications infrastructure, allowing developers to focus on building their applications.
2. What are some key features of Twilio?
Answer: Some key features of Twilio include:
- Programmable Voice: Make and receive phone calls programmatically
- Programmable SMS: Send and receive text messages
- Programmable Video: Build video chat applications
- Elastic SIP Trunking: Connect VoIP systems to the public switched telephone network (PSTN)
- Two-Factor Authentication: Implement secure user authentication
- Programmable Chat: Build real-time chat applications
- TaskRouter: Intelligently route tasks to agents or processes
- Lookup API: Validate and format phone numbers
3. How do you send an SMS using Twilio’s API?
Answer: To send an SMS using Twilio’s API, you typically follow these steps:
- Sign up for a Twilio account and obtain your Account SID and Auth Token
- Purchase a Twilio phone number capable of sending SMS
- Use a programming language of your choice and Twilio’s SDK or REST API
Here’s a basic example using Python:
from twilio.rest import Client
account_sid = 'your_account_sid'
auth_token = 'your_auth_token'
client = Client(account_sid, auth_token)
message = client.messages.create(
body='Hello from Twilio!',
from_='your_twilio_number',
to='recipient_number'
)
print(message.sid)
4. What is a Twilio TwiML, and how is it used?
Answer: TwiML (Twilio Markup Language) is an XML-based language used to instruct Twilio on how to handle phone calls or SMS messages. When Twilio receives an incoming call or message, it looks for TwiML instructions to determine how to respond. TwiML consists of verbs (actions) and nouns (properties of those actions).
For example, a simple TwiML to respond to a voice call with a message might look like this:
<?xml version="1.0" encoding="UTF-8"?>
<Response>
<Say>Hello, thank you for calling. This is a Twilio response.</Say>
</Response>
5. What is a Twilio Webhook, and why is it important?
Answer: A Twilio Webhook is a URL that Twilio sends HTTP requests to when certain events occur, such as receiving an incoming call or message. Webhooks are important because they allow your application to receive real-time notifications and respond dynamically to events in the Twilio ecosystem. This enables you to build interactive and responsive communication applications.
6. How do you handle incoming calls using Twilio?
Answer: To handle incoming calls with Twilio, you typically follow these steps:
- Set up a webhook URL in your Twilio account for incoming calls
- Create a server endpoint that responds to Twilio’s webhook requests
- Use TwiML to instruct Twilio on how to handle the call
Here’s a simple example using Python and Flask:
from flask import Flask, request
from twilio.twiml.voice_response import VoiceResponse
app = Flask(__name__)
@app.route("/voice", methods=['POST'])
def handle_incoming_call():
resp = VoiceResponse()
resp.say("Thank you for calling. This is a Twilio response.")
return str(resp)
if __name__ == "__main__":
app.run(debug=True)
7. What is Twilio Programmable Voice, and how is it different from regular phone calls?
Answer: Twilio Programmable Voice is a set of APIs that allow developers to build custom voice experiences into their applications. It differs from regular phone calls in several ways:
- Programmability: You can control call flow, routing, and features programmatically
- Scalability: It can handle a large volume of concurrent calls
- Advanced features: Supports features like call recording, transcription, and conference calling
- Integration: Can be easily integrated with other systems and applications
- Global reach: Allows for making and receiving calls worldwide
8. How do you implement two-factor authentication using Twilio?
Answer: To implement two-factor authentication (2FA) using Twilio, you can follow these general steps:
- Generate a random verification code when a user attempts to log in
- Use Twilio’s SMS API to send the code to the user’s phone number
- Prompt the user to enter the code on your application
- Verify the entered code against the generated code
Here’s a basic example using Python:
import random
from twilio.rest import Client
def generate_verification_code():
return str(random.randint(100000, 999999))
def send_verification_code(phone_number, code):
account_sid = 'your_account_sid'
auth_token = 'your_auth_token'
client = Client(account_sid, auth_token)
message = client.messages.create(
body=f'Your verification code is: {code}',
from_='your_twilio_number',
to=phone_number
)
return message.sid
# Usage
user_phone = '+1234567890'
verification_code = generate_verification_code()
send_verification_code(user_phone, verification_code)
# Then, verify the code entered by the user
entered_code = input("Enter the verification code: ")
if entered_code == verification_code:
print("Verification successful!")
else:
print("Verification failed.")
9. What is Twilio Studio, and how can it be used in application development?
Answer: Twilio Studio is a visual development environment that allows you to build communication workflows without writing code. It provides a drag-and-drop interface for creating voice and messaging applications. Studio can be used in application development to:
- Quickly prototype and test communication flows
- Build interactive voice response (IVR) systems
- Create SMS-based chatbots
- Design customer support workflows
- Implement automated appointment reminders
Studio integrates with other Twilio products and can be extended with custom functions, making it a powerful tool for both rapid prototyping and production-ready applications.
10. How do you handle errors and exceptions when working with Twilio APIs?
Answer: When working with Twilio APIs, it’s important to implement proper error handling to ensure your application remains stable. Here are some best practices:
- Use try-except blocks to catch Twilio-specific exceptions
- Handle common errors like authentication failures, invalid phone numbers, or rate limiting
- Log errors for debugging and monitoring purposes
- Implement retries for transient errors
- Provide meaningful error messages to users
Here’s an example of error handling in Python:
from twilio.rest import Client
from twilio.base.exceptions import TwilioRestException
try:
client = Client(account_sid, auth_token)
message = client.messages.create(
body='Hello from Twilio!',
from_='your_twilio_number',
to='recipient_number'
)
print(f"Message sent successfully. SID: {message.sid}")
except TwilioRestException as e:
print(f"An error occurred: {e}")
# Handle specific error codes
if e.code == 21614:
print("Invalid phone number")
elif e.code == 20003:
print("Authentication failed")
except Exception as e:
print(f"An unexpected error occurred: {e}")
# Log the error for further investigation
11. What is Twilio Flex, and how does it differ from other Twilio products?
Answer: Twilio Flex is a programmable contact center platform that allows businesses to create customized customer engagement solutions. It differs from other Twilio products in several ways:
- Comprehensive solution: Flex provides a full-featured contact center out of the box, while other Twilio products are more focused on specific communication channels
- Customizability: Flex offers a high degree of customization, allowing businesses to tailor the platform to their specific needs
- Omnichannel support: Flex natively supports voice, video, chat, SMS, and social media channels in a single interface
- Agent interface: Flex includes a pre-built but customizable agent desktop interface
- Integration capabilities: Flex can easily integrate with CRM systems and other business tools
12. How do you implement a conference call using Twilio?
Answer: To implement a conference call using Twilio, you can use the <Conference> TwiML verb. Here’s a basic example of how to set up a conference call:
from flask import Flask, request
from twilio.twiml.voice_response import VoiceResponse, Dial
app = Flask(__name__)
@app.route("/conference", methods=['POST'])
def conference():
response = VoiceResponse()
dial = Dial()
dial.conference('MyConference')
response.append(dial)
return str(response)
if __name__ == "__main__":
app.run(debug=True)
In this example, when a caller reaches this endpoint, they will be added to a conference room named ‘MyConference’. Multiple callers can join the same conference by calling this endpoint.
13. What is Twilio’s Lookup API, and how can it be used?
Answer: Twilio’s Lookup API is a service that allows you to validate and obtain information about phone numbers. It can be used for various purposes, including:
- Validating phone numbers before sending messages or making calls
- Formatting phone numbers to ensure consistency
- Determining the carrier associated with a phone number
- Checking if a number is capable of receiving SMS messages
- Identifying the country and region of a phone number
Here’s an example of how to use the Lookup API in Python:
from twilio.rest import Client
account_sid = 'your_account_sid'
auth_token = 'your_auth_token'
client = Client(account_sid, auth_token)
phone_number = '+1234567890'
try:
number = client.lookups.phone_numbers(phone_number).fetch(type=['carrier'])
print(f"Phone number: {number.phone_number}")
print(f"Carrier: {number.carrier['name']}")
print(f"Type: {number.carrier['type']}")
except Exception as e:
print(f"An error occurred: {e}")
14. How do you implement call forwarding using Twilio?
Answer: To implement call forwarding with Twilio, you can use the <Dial> verb in TwiML. Here’s an example of how to forward an incoming call to another number:
from flask import Flask, request
from twilio.twiml.voice_response import VoiceResponse, Dial
app = Flask(__name__)
@app.route("/forward", methods=['POST'])
def forward_call():
response = VoiceResponse()
dial = Dial()
dial.number('+1987654321') # Replace with the forwarding number
response.append(dial)
return str(response)
if __name__ == "__main__":
app.run(debug=True)
In this example, when an incoming call reaches this endpoint, it will be forwarded to the number specified in the dial.number() method.
15. What is Twilio’s TaskRouter, and how can it be used in a customer service application?
Answer: Twilio’s TaskRouter is a powerful tool for intelligently distributing tasks (such as incoming calls, messages, or support tickets) to the most appropriate agents or processes. In a customer service application, TaskRouter can be used to:
- Route incoming customer inquiries to the most qualified agent based on skills, language, or other attributes
- Manage agent availability and workload
- Implement priority queuing for VIP customers or urgent issues
- Create workflows for different types of customer inquiries
- Provide real-time analytics on queue status and agent performance
Here’s a basic example of how to create a task using TaskRouter:
from twilio.rest import Client
account_sid = 'your_account_sid'
auth_token = 'your_auth_token'
workspace_sid = 'your_workspace_sid'
workflow_sid = 'your_workflow_sid'
client = Client(account_sid, auth_token)
task = client.taskrouter.workspaces(workspace_sid).tasks.create(
workflow_sid=workflow_sid,
attributes='{"type": "support", "language": "en"}',
timeout=3600
)
print(f"Created task: {task.sid}")
16. How do you implement a chatbot using Twilio’s Programmable Chat?
Answer: To implement a chatbot using Twilio’s Programmable Chat, you can follow these general steps:
- Set up a Twilio Programmable Chat service
- Create a chat channel for the bot
- Implement a webhook to receive incoming messages
- Process the incoming messages and generate appropriate responses
- Send the bot’s responses back to the chat channel
Here’s a simple example of a chatbot webhook using Python and Flask:
from flask import Flask, request, jsonify
from twilio.rest import Client
app = Flask(__name__)
account_sid = 'your_account_sid'
auth_token = 'your_auth_token'
client = Client(account_sid, auth_token)
@app.route("/chatbot", methods=['POST'])
def chatbot():
data = request.json
channel_sid = data['ChannelSid']
message_body = data['Body']
# Process the message and generate a response
response = generate_bot_response(message_body)
# Send the response back to the chat channel
message = client.chat.services('your_chat_service_sid').channels(channel_sid).messages.create(
body=response
)
return jsonify({'status': 'success'})
def generate_bot_response(message):
# Implement your chatbot logic here
if 'hello' in message.lower():
return "Hello! How can I assist you today?"
else:
return "I'm sorry, I didn't understand that. Can you please rephrase?"
if __name__ == "__main__":
app.run(debug=True)
17. What are Twilio Functions, and how can they be used to extend Twilio’s capabilities?
Answer: Twilio Functions are serverless JavaScript functions that run in Twilio’s cloud environment. They allow you to write custom logic to extend Twilio’s capabilities without managing your own server infrastructure. Twilio Functions can be used to:
- Create custom webhooks for handling Twilio events
- Implement complex call flows or messaging logic
- Integrate with external APIs or databases
- Process and transform data before sending it to other Twilio services
- Build custom IVR systems
Here’s an example of a simple Twilio Function that responds to an incoming SMS:
exports.handler = function(context, event, callback) {
let twiml = new Twilio.twiml.MessagingResponse();
twiml.message('Thank you for your message! We'll get back to you soon.');
callback(null, twiml);
};
18. How do you implement call recording using Twilio?
Answer: To implement call recording with Twilio, you can use the <Record> verb in TwiML or enable recording programmatically. Here’s an example of how to record a call using TwiML:
from flask import Flask, request
from twilio.twiml.voice_response import VoiceResponse, Record
app = Flask(__name__)
@app.route("/record", methods=['POST'])
def record_call():
response = VoiceResponse()
response.say("This call will be recorded. Please leave a message after the beep.")
response.record(max_length=30, play_beep=True)
response.say("Thank you for your message. Goodbye.")
return str(response)
if __name__ == "__main__":
app.run(debug=True)
You can also start, stop, or pause recordings programmatically using the Twilio API:
from twilio.rest import Client
account_sid = 'your_account_sid'
auth_token = 'your_auth_token'
client = Client(account_sid, auth_token)
recording = client.calls('CAXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX').recordings.create()
print(f"Recording started: {recording.sid}")
19. What is Twilio’s Verify API, and how does it differ from implementing 2FA manually?
Answer: Twilio’s Verify API is a service that simplifies the implementation of phone verification and two-factor authentication (2FA). It differs from manual 2FA implementation in several ways:
- Simplified integration: Verify API handles the entire verification process, reducing the amount of code you need to write
- Multiple channels: Supports SMS, voice, email, and push notifications for verification
- Global coverage: Automatically handles international phone number formatting and regulations
- Fraud prevention: Includes built-in security features to prevent abuse
- Analytics and reporting: Provides insights into verification attempts and success rates
Here’s an example of how to use the Verify API:
from twilio.rest import Client
account_sid = 'your_account_sid'
auth_token = 'your_auth_token'
client = Client(account_sid, auth_token)
verification = client.verify.services('VAXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX') \
.verifications \
.create(to='+1234567890', channel='sms')
print(verification.status)
# Later, verify the code entered by the user
verification_check = client.verify.services('VAXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX') \
.verification_checks \
.create(to='+1234567890', code='123456')
print(verification_check.status)
20. How do you ensure security and compliance when working with Twilio, especially regarding sensitive customer data?
Answer: When working with Twilio and handling sensitive customer data, it’s crucial to implement proper security measures and ensure compliance with relevant regulations. Here are some best practices:
- Use HTTPS for all communications with Twilio APIs
- Securely store and manage Twilio credentials (Account SID and Auth Token)
- Implement proper authentication and authorization in your application
- Use Twilio’s security features, such as request validation
- Encrypt sensitive data at rest and in transit
- Implement proper logging and auditing
- Regularly update your Twilio SDK and dependencies
- Follow data privacy regulations (e.g., GDPR, CCPA) when handling customer data
- Use Twilio’s compliance features, such as redacting sensitive information from logs
- Implement proper error handling to prevent information leakage
Here’s an example of how to validate incoming Twilio requests in Python:
from flask import Flask, request
from twilio.request_validator import RequestValidator
app = Flask(__name__)
@app.route("/webhook", methods=['POST'])
def webhook():
# Your Twilio Auth Token
auth_token = 'your_auth_token'
# The Twilio request validator
validator = RequestValidator(auth_token)
# The Twilio signature from the request header
twilio_signature = request.headers.get('X-Twilio-Signature', '')
# The full URL of the request URL
url = request.url
# Get the request parameters
params = request.form.to_dict()
# Validate the request
if validator.validate(url, params, twilio_signature):
# Request is valid, process it
return "Valid Twilio request", 200
else:
# Invalid request, reject it
return "Invalid Twilio request", 403
if __name__ == "__main__":
app.run(debug=True)
Conclusion
Mastering these Twilio interview questions will significantly boost your confidence and preparedness for your upcoming interview. Remember that Twilio is a powerful and versatile platform, and interviewers are often looking for candidates who not only understand its technical aspects but also grasp its potential for solving real-world communication challenges.
As you prepare, consider working on small projects or demos that showcase your Twilio skills. This hands-on experience will not only reinforce your understanding but also provide concrete examples to discuss during your interview.
Good luck with your Twilio interview! With thorough preparation and a solid understanding of these concepts, you’ll be well-equipped to impress your interviewers and take the next step in your career in cloud communications development.