The Role of Test-Driven Development in Modern Software Engineering
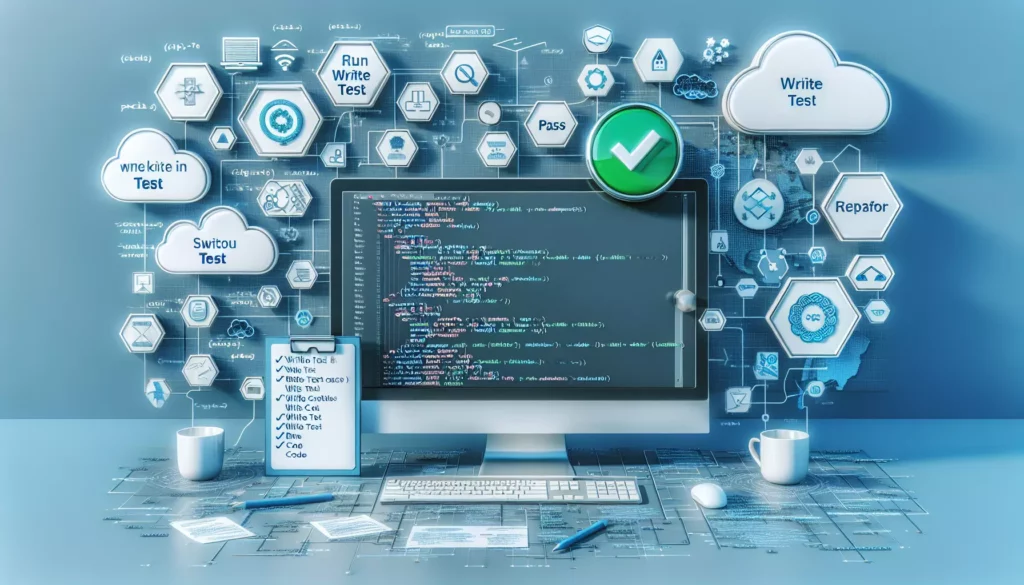
In the ever-evolving landscape of software development, methodologies and practices come and go. However, one approach that has stood the test of time and continues to gain traction is Test-Driven Development (TDD). This powerful technique not only improves code quality but also enhances the overall development process. In this comprehensive guide, we’ll explore the role of TDD in modern software engineering, its benefits, challenges, and how it fits into the broader context of coding education and skill development.
What is Test-Driven Development?
Test-Driven Development is a software development process that relies on the repetition of a very short development cycle. It begins with the developer writing a failing automated test case that defines a desired improvement or new function. This is followed by producing the minimum amount of code to pass that test, and then refactoring the new code to acceptable standards.
The TDD cycle typically follows these steps:
- Write a test
- Run the test (which should fail because the feature hasn’t been implemented)
- Write the minimal amount of code to make the test pass
- Run the test again (which should now pass)
- Refactor the code
- Repeat
This approach is often summarized as “Red-Green-Refactor,” where red represents the failing test, green represents the passing test, and refactor represents the optimization of the code.
The Benefits of Test-Driven Development
1. Improved Code Quality
One of the primary benefits of TDD is the improvement in code quality. By writing tests before the actual code, developers are forced to think about the design and functionality of their code from the outset. This leads to more modular, loosely coupled, and easily maintainable code.
2. Better Documentation
Tests serve as a form of documentation. They describe what the code is supposed to do in a clear, executable format. This is particularly useful for new team members or when revisiting code after a long period.
3. Faster Debugging
When a test fails, it immediately pinpoints where the problem is. This significantly reduces debugging time, as developers can quickly identify and fix issues.
4. Encourages Simplicity
TDD encourages developers to write only the code necessary to pass the tests. This naturally leads to simpler, more focused code that does exactly what it’s supposed to do and nothing more.
5. Facilitates Refactoring
With a comprehensive test suite in place, developers can refactor code with confidence. They can make changes and immediately see if anything breaks, allowing for continuous improvement of the codebase.
TDD in Practice: A Simple Example
Let’s walk through a simple example of TDD in practice. Suppose we want to create a function that adds two numbers. Here’s how we might approach this using TDD:
Step 1: Write a Test
First, we write a test for our yet-to-be-implemented function:
def test_add_numbers():
assert add_numbers(2, 3) == 5
assert add_numbers(-1, 1) == 0
assert add_numbers(0, 0) == 0
Step 2: Run the Test
When we run this test, it will fail because we haven’t implemented the add_numbers
function yet.
Step 3: Write the Minimal Code
Now, we write the minimal code to make the test pass:
def add_numbers(a, b):
return a + b
Step 4: Run the Test Again
We run the test again, and it should now pass.
Step 5: Refactor (if necessary)
In this simple example, there’s not much to refactor. However, in more complex scenarios, this is where we would optimize our code while ensuring the tests still pass.
TDD and Its Role in Coding Education
Test-Driven Development plays a crucial role in coding education and skill development. Here’s how:
1. Encourages Analytical Thinking
TDD forces learners to think critically about what they want their code to accomplish before they start writing it. This analytical approach is crucial for developing problem-solving skills.
2. Builds Confidence
For beginners, seeing tests pass can be incredibly motivating. It provides immediate feedback and a sense of accomplishment, which can boost confidence and encourage further learning.
3. Teaches Best Practices
TDD inherently teaches several best practices in software development, such as writing modular code, thinking about edge cases, and the importance of testing.
4. Prepares for Real-World Development
Many professional development teams use TDD or similar testing practices. By learning TDD early, students are better prepared for real-world software development scenarios.
TDD and Technical Interviews
When it comes to preparing for technical interviews, particularly for major tech companies, understanding and practicing TDD can be a significant advantage:
1. Demonstrates Thoroughness
By writing tests before implementing a solution, candidates demonstrate a thorough approach to problem-solving. This attention to detail is highly valued in technical interviews.
2. Showcases Testing Knowledge
Many companies place a high value on testing. Demonstrating familiarity with TDD shows that a candidate understands the importance of writing testable, maintainable code.
3. Improves Code Quality Under Pressure
Technical interviews can be stressful, and it’s easy to make mistakes. By following TDD principles, candidates can write more robust solutions even under pressure.
4. Facilitates Clear Communication
Writing tests first allows candidates to clearly communicate their understanding of the problem and their approach to solving it. This can lead to better discussions with interviewers.
Challenges of Test-Driven Development
While TDD offers numerous benefits, it’s not without its challenges:
1. Initial Time Investment
Writing tests before code can feel time-consuming, especially for developers new to the practice. It requires a shift in mindset and workflow.
2. Learning Curve
TDD requires knowledge of testing frameworks and best practices. This can be an additional learning curve for beginners.
3. Maintaining Test Suites
As projects grow, maintaining a comprehensive test suite can become challenging. Tests need to be updated as requirements change, which can be time-consuming.
4. Overemphasis on Testing
Sometimes, developers can become too focused on achieving test coverage at the expense of other important aspects of development, like user experience or performance.
Integrating TDD into Your Development Workflow
If you’re interested in adopting TDD, here are some steps to integrate it into your development workflow:
1. Start Small
Begin by applying TDD to small, isolated parts of your project. This allows you to get comfortable with the process without feeling overwhelmed.
2. Use Testing Frameworks
Familiarize yourself with testing frameworks for your programming language. For example, JUnit for Java, pytest for Python, or Jest for JavaScript. These frameworks make it easier to write and run tests.
3. Practice Regularly
Like any skill, TDD improves with practice. Try to incorporate it into your daily coding routine.
4. Pair Programming
If possible, practice TDD with a partner. One person can write the tests while the other writes the code to pass them. This can help reinforce the TDD mindset.
5. Refactor Fearlessly
With a solid test suite in place, you can refactor your code with confidence. Take advantage of this to continuously improve your codebase.
TDD and Continuous Integration/Continuous Deployment (CI/CD)
Test-Driven Development aligns well with modern CI/CD practices. Here’s how:
1. Automated Testing
TDD naturally produces a comprehensive suite of automated tests. These can be easily integrated into CI/CD pipelines to ensure code quality at every stage of development.
2. Early Bug Detection
By running tests automatically with each code change, bugs are caught early in the development process, reducing the cost and time of fixes.
3. Confidence in Deployments
With a robust test suite, teams can have more confidence in their deployments. This can lead to more frequent and reliable releases.
4. Documentation for CI/CD
Tests serve as living documentation of the system’s behavior, which can be invaluable for maintaining and updating CI/CD pipelines.
TDD and Agile Methodologies
Test-Driven Development aligns well with Agile methodologies:
1. Iterative Development
TDD’s short development cycles fit perfectly with Agile’s iterative approach to software development.
2. Emphasis on Quality
Both TDD and Agile methodologies prioritize delivering high-quality, working software.
3. Adaptability
TDD makes it easier to adapt to changing requirements, a key principle of Agile development.
4. Continuous Improvement
The refactoring step in TDD aligns with Agile’s focus on continuous improvement and technical excellence.
TDD and Different Programming Paradigms
While TDD is often associated with object-oriented programming, it can be applied to various programming paradigms:
1. Functional Programming
In functional programming, TDD can be used to test pure functions and ensure they produce the expected output for given inputs.
2. Procedural Programming
TDD can be applied to test individual procedures or functions in procedural programming.
3. Object-Oriented Programming
In OOP, TDD is often used to test individual methods, class behaviors, and interactions between objects.
Tools and Frameworks for TDD
There are numerous tools and frameworks available to support Test-Driven Development across various programming languages:
1. JUnit (Java)
JUnit is a popular testing framework for Java. It provides annotations to identify test methods and assertions to verify expected results.
2. pytest (Python)
pytest is a powerful testing framework for Python that makes it easy to write simple and scalable test cases.
3. Jest (JavaScript)
Jest is a delightful JavaScript Testing Framework with a focus on simplicity. It works with projects using Babel, TypeScript, Node.js, React, Angular, Vue.js and more.
4. RSpec (Ruby)
RSpec is a testing tool for Ruby, created for behavior-driven development (BDD). It is the most frequently used testing library for Ruby in production applications.
5. NUnit (.NET)
NUnit is a unit-testing framework for all .NET languages. It is written entirely in C# and has been completely redesigned to take advantage of many .NET language features.
Best Practices for Test-Driven Development
To get the most out of TDD, consider these best practices:
1. Keep Tests Simple
Write clear, concise tests that focus on one specific behavior or functionality.
2. Follow the AAA Pattern
Structure your tests using the Arrange-Act-Assert pattern: set up the test conditions, perform the action, and verify the results.
3. Test Behavior, Not Implementation
Focus on testing what your code does, not how it does it. This allows for more flexibility in refactoring.
4. Maintain Test Independence
Ensure that your tests can run in any order and don’t depend on the state created by other tests.
5. Embrace Red-Green-Refactor
Don’t skip steps in the TDD cycle. Write a failing test, make it pass with the simplest code possible, then refactor.
6. Use Descriptive Test Names
Your test names should clearly describe the behavior being tested. This improves readability and serves as documentation.
The Future of TDD
As software development continues to evolve, so too does the practice of Test-Driven Development. Here are some trends that may shape the future of TDD:
1. AI-Assisted TDD
Artificial Intelligence could potentially assist in generating test cases or even suggesting code implementations based on written tests.
2. TDD in Machine Learning
As machine learning becomes more prevalent, new approaches to TDD are being developed to handle the unique challenges of testing ML models.
3. TDD for Microservices
With the rise of microservices architecture, TDD practices are adapting to handle distributed systems and service-to-service interactions.
4. Property-Based Testing
This approach, where tests are written to verify properties of the code rather than specific examples, is gaining popularity and could influence the future of TDD.
Conclusion
Test-Driven Development is more than just a coding practice; it’s a fundamental shift in how we approach software development. By emphasizing upfront design, continuous testing, and iterative improvement, TDD aligns perfectly with the goals of producing high-quality, maintainable code.
For those learning to code or preparing for technical interviews, TDD offers a structured approach to problem-solving that can significantly enhance your skills. It encourages careful thinking about requirements, edge cases, and code structure before diving into implementation.
While TDD does come with its challenges, particularly in terms of the initial learning curve and time investment, the long-term benefits in code quality, maintainability, and developer confidence are well worth the effort. As software systems continue to grow in complexity, practices like TDD become increasingly valuable in managing that complexity and ensuring robust, reliable software.
Whether you’re a beginner just starting your coding journey or an experienced developer looking to improve your practices, incorporating Test-Driven Development into your workflow can be a game-changer. It not only improves the quality of your code but also enhances your overall approach to problem-solving and software design.
As you continue to develop your programming skills, consider making TDD a core part of your practice. Start small, be consistent, and you’ll soon see the benefits in your code quality, your confidence as a developer, and your ability to tackle complex programming challenges.