The Future of Coding: Trends to Watch
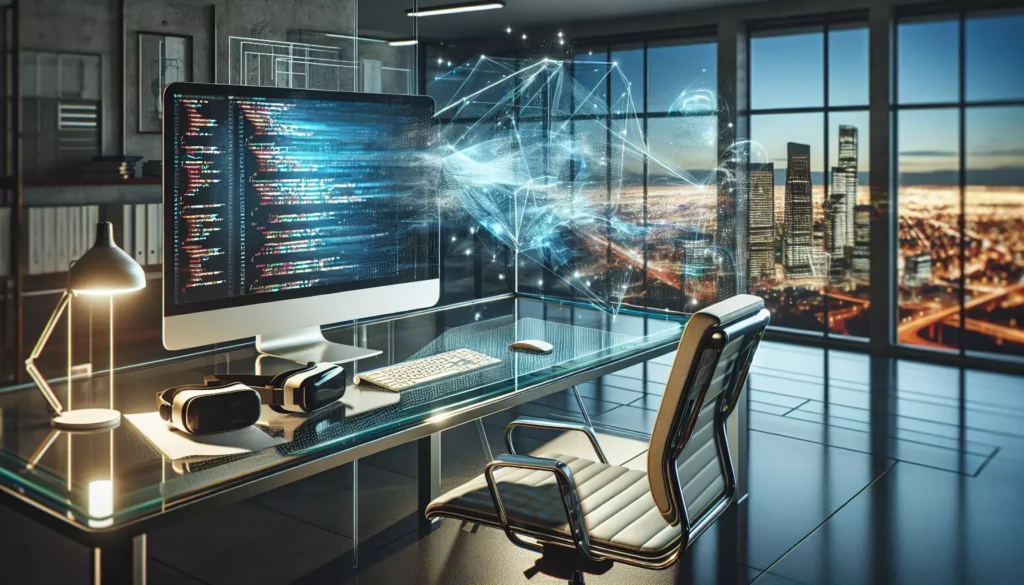
As we stand on the cusp of a new technological era, the world of coding is evolving at an unprecedented pace. For aspiring programmers and seasoned developers alike, staying ahead of the curve is not just beneficial—it’s essential. In this comprehensive guide, we’ll explore the emerging trends that are shaping the future of coding, and how platforms like AlgoCademy are adapting to prepare the next generation of tech innovators.
1. Artificial Intelligence and Machine Learning Integration
Artificial Intelligence (AI) and Machine Learning (ML) are no longer just buzzwords; they’re becoming integral parts of modern software development. As these technologies continue to advance, we’re seeing a growing demand for programmers who can work with AI and ML frameworks.
Key Developments:
- Increased adoption of AI-powered coding assistants
- Machine learning models for code prediction and auto-completion
- AI-driven bug detection and code optimization
AlgoCademy is at the forefront of this trend, incorporating AI-powered assistance into its platform to help learners grasp complex coding concepts more efficiently. This integration not only aids in learning but also prepares students for a future where AI collaboration in coding will be commonplace.
Practical Application:
Consider a scenario where you’re working on a complex algorithm. An AI assistant could suggest optimizations or point out potential edge cases you might have missed. Here’s a simple example of how an AI might assist in improving a sorting algorithm:
# Original code
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
# AI-suggested optimization
def optimized_bubble_sort(arr):
n = len(arr)
for i in range(n):
swapped = False
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
swapped = True
if not swapped:
break
return arr
In this example, the AI has suggested an optimization that can significantly improve the performance of the bubble sort algorithm for nearly sorted arrays.
2. Low-Code and No-Code Platforms
The rise of low-code and no-code platforms is democratizing software development, allowing individuals with limited programming experience to create applications. While this trend might seem to threaten traditional coding jobs, it actually creates new opportunities for developers to focus on more complex, high-value tasks.
Implications for Developers:
- Increased focus on system architecture and integration
- Need for skills in customizing and extending low-code platforms
- Greater emphasis on business logic and user experience design
AlgoCademy recognizes this shift and incorporates modules that teach not just coding, but also the principles of software design and architecture. This holistic approach ensures that learners are prepared for a future where understanding the broader context of software development is as crucial as writing code.
Example Scenario:
Imagine you’re tasked with creating a customer relationship management (CRM) system. Instead of building it from scratch, you might use a low-code platform to rapidly develop the core functionality, and then write custom code for specific, complex features:
// Low-code platform generated base structure
class CRMSystem {
constructor() {
this.customers = [];
this.orders = [];
}
addCustomer(customer) { /* ... */ }
placeOrder(order) { /* ... */ }
}
// Custom code for advanced analytics
class AdvancedAnalytics extends CRMSystem {
constructor() {
super();
this.mlModel = new MachineLearningModel();
}
predictChurn() {
return this.mlModel.analyze(this.customers);
}
recommendProducts(customerId) {
const customer = this.customers.find(c => c.id === customerId);
return this.mlModel.getRecommendations(customer);
}
}
This example demonstrates how developers can leverage low-code platforms for rapid development while still applying their coding skills for advanced features.
3. Quantum Computing
As quantum computing moves from theoretical concept to practical reality, it’s poised to revolutionize certain areas of computation. While not all developers will need to become quantum computing experts, understanding its principles and potential applications will be increasingly valuable.
Areas of Impact:
- Cryptography and security
- Optimization problems
- Machine learning and AI
AlgoCademy is preparing for this shift by introducing foundational concepts of quantum computing in its advanced courses. This forward-thinking approach ensures that students are aware of emerging technologies that could shape their future careers.
Quantum Computing Concept Example:
While actual quantum code is highly specialized, understanding quantum concepts can influence how we approach certain problems. Here’s a simplified example of how quantum thinking might influence a classical algorithm:
import random
def quantum_inspired_search(items, target):
n = len(items)
iterations = int(math.sqrt(n)) # Grover's algorithm inspiration
for _ in range(iterations):
# "Superposition" - check multiple items at once
sample = random.sample(items, int(math.sqrt(n)))
if target in sample:
return items.index(target)
# If not found in "quantum" search, fall back to classical
return items.index(target) if target in items else -1
This example doesn’t use actual quantum computing, but it’s inspired by quantum concepts like superposition and the speedup provided by Grover’s algorithm.
4. Edge Computing and IoT
With the proliferation of Internet of Things (IoT) devices and the need for real-time processing, edge computing is becoming increasingly important. This shift is creating new challenges and opportunities for developers.
Key Considerations:
- Optimizing code for resource-constrained devices
- Developing for distributed systems
- Handling real-time data processing and analytics
AlgoCademy is adapting its curriculum to include modules on efficient coding practices and distributed systems, preparing students for the unique challenges of edge computing environments.
Edge Computing Example:
Consider a scenario where you’re developing software for a smart home device that needs to process sensor data locally before sending aggregated information to the cloud:
class EdgeDevice:
def __init__(self):
self.sensors = []
self.data_buffer = []
def add_sensor(self, sensor):
self.sensors.append(sensor)
def collect_data(self):
for sensor in self.sensors:
reading = sensor.get_reading()
self.data_buffer.append(reading)
def process_locally(self):
if len(self.data_buffer) >= 100:
avg = sum(self.data_buffer) / len(self.data_buffer)
self.send_to_cloud(avg)
self.data_buffer.clear()
def send_to_cloud(self, data):
# Simulated cloud send
print(f"Sending aggregated data to cloud: {data}")
# Usage
edge_device = EdgeDevice()
edge_device.add_sensor(TemperatureSensor())
edge_device.add_sensor(HumiditySensor())
while True:
edge_device.collect_data()
edge_device.process_locally()
time.sleep(1)
This example demonstrates how an edge device might collect and process data locally, only sending aggregated information to the cloud to reduce bandwidth and improve response times.
5. Cybersecurity and Ethical Hacking
As our reliance on digital systems grows, so does the importance of cybersecurity. The future of coding will see an increased focus on security-first development practices and ethical hacking skills.
Emerging Focus Areas:
- Secure coding practices
- Penetration testing and vulnerability assessment
- Blockchain technology for secure transactions
AlgoCademy is incorporating cybersecurity principles into its core curriculum, ensuring that all students, regardless of their specialization, have a solid foundation in secure coding practices.
Secure Coding Example:
Here’s an example of how secure coding practices might be applied to a simple user authentication system:
import bcrypt
import re
class SecureAuthSystem:
def __init__(self):
self.users = {}
def register_user(self, username, password):
if not self._is_valid_username(username):
raise ValueError("Invalid username format")
if not self._is_strong_password(password):
raise ValueError("Password does not meet security requirements")
salt = bcrypt.gensalt()
hashed_password = bcrypt.hashpw(password.encode('utf-8'), salt)
self.users[username] = hashed_password
def authenticate_user(self, username, password):
if username not in self.users:
return False
stored_hash = self.users[username]
return bcrypt.checkpw(password.encode('utf-8'), stored_hash)
def _is_valid_username(self, username):
return re.match(r'^[a-zA-Z0-9_]{3,20}$', username) is not None
def _is_strong_password(self, password):
return (len(password) >= 12 and
re.search(r"[A-Z]", password) and
re.search(r"[a-z]", password) and
re.search(r"[0-9]", password) and
re.search(r"[!@#$%^&*(),.?":{}|<>]", password))
# Usage
auth_system = SecureAuthSystem()
auth_system.register_user("john_doe", "Str0ngP@ssw0rd!")
is_authenticated = auth_system.authenticate_user("john_doe", "Str0ngP@ssw0rd!")
print(f"Authentication successful: {is_authenticated}")
This example demonstrates several security best practices, including password hashing, input validation, and enforcing strong password policies.
6. Cross-Platform Development
The proliferation of devices and platforms has made cross-platform development more important than ever. Developers of the future will need to be adept at creating applications that work seamlessly across multiple environments.
Key Technologies:
- Progressive Web Apps (PWAs)
- Cross-platform frameworks like React Native and Flutter
- Universal Windows Platform (UWP) for Microsoft ecosystems
AlgoCademy recognizes this trend and includes modules on cross-platform development techniques, ensuring that students are prepared to create applications that reach the widest possible audience.
Cross-Platform Development Example:
Here’s a simple example of how you might structure a cross-platform mobile app using React Native:
import React from 'react';
import { View, Text, Platform, StyleSheet } from 'react-native';
const CrossPlatformApp = () => {
return (
<View style={styles.container}>
<Text style={styles.text}>
Welcome to my {Platform.OS === 'ios' ? 'iOS' : 'Android'} app!
</Text>
{Platform.OS === 'ios' ? (
<Text style={styles.platformSpecific}>iOS-specific feature</Text>
) : (
<Text style={styles.platformSpecific}>Android-specific feature</Text>
)}
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: Platform.select({
ios: '#FFFFFF',
android: '#E8E8E8',
}),
},
text: {
fontSize: 20,
color: Platform.select({
ios: '#007AFF',
android: '#4CAF50',
}),
},
platformSpecific: {
marginTop: 20,
fontStyle: 'italic',
},
});
export default CrossPlatformApp;
This example shows how you can create a single codebase that adapts to different platforms, providing a consistent user experience while still allowing for platform-specific customizations.
7. Sustainability and Green Coding
As awareness of environmental issues grows, there’s an increasing focus on sustainable and energy-efficient coding practices. The future of coding will likely see a greater emphasis on optimizing software for energy consumption.
Areas of Focus:
- Energy-efficient algorithms
- Optimizing cloud resource usage
- Sustainable software design principles
AlgoCademy is incorporating these principles into its curriculum, teaching students not just how to write functional code, but how to write code that is environmentally responsible.
Green Coding Example:
Here’s an example of how you might optimize a computationally intensive task for energy efficiency:
import time
def energy_intensive_task(data):
# Simulating a computationally expensive operation
time.sleep(0.1)
return sum(data)
def batch_process(data, batch_size=1000):
results = []
for i in range(0, len(data), batch_size):
batch = data[i:i+batch_size]
results.append(energy_intensive_task(batch))
return sum(results)
# Inefficient approach
def inefficient_approach(data):
return sum(energy_intensive_task([x]) for x in data)
# Energy-efficient approach
def efficient_approach(data):
return batch_process(data)
# Compare the two approaches
data = list(range(10000))
start = time.time()
inefficient_result = inefficient_approach(data)
inefficient_time = time.time() - start
start = time.time()
efficient_result = efficient_approach(data)
efficient_time = time.time() - start
print(f"Inefficient approach time: {inefficient_time:.2f} seconds")
print(f"Efficient approach time: {efficient_time:.2f} seconds")
print(f"Energy savings: {(inefficient_time - efficient_time) / inefficient_time * 100:.2f}%")
This example demonstrates how batching operations can significantly reduce processing time and, consequently, energy consumption, especially for cloud-based or large-scale applications.
Conclusion: Embracing the Future of Coding
As we’ve explored in this comprehensive guide, the future of coding is dynamic, multifaceted, and full of exciting possibilities. From the integration of AI and machine learning to the rise of quantum computing and the emphasis on sustainable coding practices, the landscape of software development is evolving rapidly.
Platforms like AlgoCademy are playing a crucial role in preparing the next generation of developers for these changes. By offering a curriculum that not only covers the fundamentals of coding but also incorporates emerging trends and technologies, AlgoCademy ensures that its students are well-equipped to face the challenges and opportunities of tomorrow’s tech landscape.
For aspiring developers and seasoned professionals alike, staying ahead in this fast-paced field requires a commitment to lifelong learning and adaptation. The key to success lies not just in mastering today’s technologies, but in developing the flexibility and curiosity to embrace whatever new innovations the future may bring.
As we stand on the brink of these exciting developments, one thing is clear: the future of coding is bright, and those who are prepared to learn, adapt, and innovate will find themselves at the forefront of the next technological revolution.
Whether you’re just starting your coding journey or looking to expand your existing skills, platforms like AlgoCademy offer the resources and guidance needed to navigate this evolving landscape. By focusing on algorithmic thinking, problem-solving skills, and practical coding experience, while also introducing cutting-edge concepts, AlgoCademy is helping to shape the developers who will define the future of technology.
Embrace the changes, stay curious, and never stop learning. The future of coding is in your hands, and it’s full of endless possibilities.